Write a Python program that contains multiple user-defined functions which calculate storage requirements and costs. These functions will provide useful metrics in the context of data storage and backup solutions. Perform the following steps: Create a function named calculate_backup_size that takes two arguments: data_size (in gigabytes) and backup_frequency. This function should return the data_size multiplied by backup_frequency, representing the total storage needed for backups in a week. Create another function named calculate_storage_cost that takes two arguments: backup_size and cost_per_gb. This function should return the value of backup_size multiplied by cost_per_gb, representing the total cost for the backup storage. Create a third function named storage_info that takes four arguments: data_size, backup_frequency, cost_per_gb, and weeks. This function should call calculate_backup_size and calculate_storage_cost functions, taking the appropriate arguments. Then it should multiply the cost by the number of weeks. It should return a string that says "The total storage needed for backup is X GB and the total cost for Y weeks is Z dollars", replacing X, Y, and Z with the calculated values. Prompt the user to enter values for data_size, backup_frequency, cost_per_gb, and weeks. If the user does not enter a positive number, your program should catch this error and ask for the input again. Call the storage_info function with the user-provided data_size, backup_frequency, cost_per_gb, and weeks and print the returned string. Provide comments in your code to explain the logic used. Test your program with different inputs and ensure that it performs the calculations correctly.
Write a Python program that contains multiple user-defined functions which calculate storage requirements and costs. These functions will provide useful metrics in the context of data storage and backup solutions. Perform the following steps: Create a function named calculate_backup_size that takes two arguments: data_size (in gigabytes) and backup_frequency. This function should return the data_size multiplied by backup_frequency, representing the total storage needed for backups in a week. Create another function named calculate_storage_cost that takes two arguments: backup_size and cost_per_gb. This function should return the value of backup_size multiplied by cost_per_gb, representing the total cost for the backup storage. Create a third function named storage_info that takes four arguments: data_size, backup_frequency, cost_per_gb, and weeks. This function should call calculate_backup_size and calculate_storage_cost functions, taking the appropriate arguments. Then it should multiply the cost by the number of weeks. It should return a string that says "The total storage needed for backup is X GB and the total cost for Y weeks is Z dollars", replacing X, Y, and Z with the calculated values. Prompt the user to enter values for data_size, backup_frequency, cost_per_gb, and weeks. If the user does not enter a positive number, your program should catch this error and ask for the input again. Call the storage_info function with the user-provided data_size, backup_frequency, cost_per_gb, and weeks and print the returned string. Provide comments in your code to explain the logic used. Test your program with different inputs and ensure that it performs the calculations correctly.
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter6: Modularity Using Functions
Section6.1: Function And Parameter Declarations
Problem 3E
Related questions
Question
- Write a Python program that contains multiple user-defined functions which calculate storage requirements and costs. These functions will provide useful metrics in the context of data storage and backup solutions.
- Perform the following steps:
- Create a function named calculate_backup_size that takes two arguments: data_size (in gigabytes) and backup_frequency. This function should return the data_size multiplied by backup_frequency, representing the total storage needed for backups in a week.
- Create another function named calculate_storage_cost that takes two arguments: backup_size and cost_per_gb. This function should return the value of backup_size multiplied by cost_per_gb, representing the total cost for the backup storage.
- Create a third function named storage_info that takes four arguments: data_size, backup_frequency, cost_per_gb, and weeks. This function should call calculate_backup_size and calculate_storage_cost functions, taking the appropriate arguments. Then it should multiply the cost by the number of weeks. It should return a string that says "The total storage needed for backup is X GB and the total cost for Y weeks is Z dollars", replacing X, Y, and Z with the calculated values.
- Prompt the user to enter values for data_size, backup_frequency, cost_per_gb, and weeks. If the user does not enter a positive number, your program should catch this error and ask for the input again.
- Call the storage_info function with the user-provided data_size, backup_frequency, cost_per_gb, and weeks and print the returned string.
- Provide comments in your code to explain the logic used.
- Test your program with different inputs and ensure that it performs the calculations correctly.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
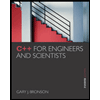
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
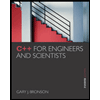
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr