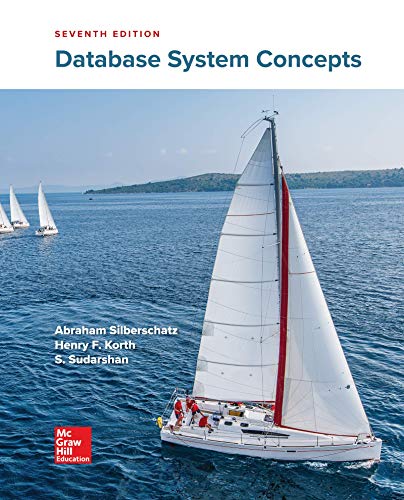
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
Please solve both parts of the question(the entire problem). Thank you!
![Part I
Write a recursive function named merge_sort that sorts a given list using the recursive algorithm of merge sort. Implement and use the helper function
_merge , which merges two sorted arrays.
Sample Output
> merge_sort([10, 32, 83, 2, 72, 100, 32])
[2, 10, 32, 32, ]
> merge_sort ( ['a','g','a', 't','e'])
['a','a','g','e', 't']
In [ ]: def _merge(P, Q):
""merges two sorted arrays
INPUT:
* P, Q - the two sorted arrays
OUTPUT: a sorted list consisting of elements in P and Q
pass
In [ ]: def merge_sort(array):
""sorts a given list
INPUT:
* array - the list
OUTPUT: a sorted copy of the list.
pass
Part II
Use a proof by induction to show that the function merge_sort will correctly sort any array of size n.
Proof
Type your answer here. Use to type LaTeX math expressions, e.g. p A q](https://content.bartleby.com/qna-images/question/7a565b17-6d0d-4aeb-8757-7c825aa401c7/022e7f7c-47f0-41b3-a54c-5cb72a0dc412/gbzju5_thumbnail.png)
Transcribed Image Text:Part I
Write a recursive function named merge_sort that sorts a given list using the recursive algorithm of merge sort. Implement and use the helper function
_merge , which merges two sorted arrays.
Sample Output
> merge_sort([10, 32, 83, 2, 72, 100, 32])
[2, 10, 32, 32, ]
> merge_sort ( ['a','g','a', 't','e'])
['a','a','g','e', 't']
In [ ]: def _merge(P, Q):
""merges two sorted arrays
INPUT:
* P, Q - the two sorted arrays
OUTPUT: a sorted list consisting of elements in P and Q
pass
In [ ]: def merge_sort(array):
""sorts a given list
INPUT:
* array - the list
OUTPUT: a sorted copy of the list.
pass
Part II
Use a proof by induction to show that the function merge_sort will correctly sort any array of size n.
Proof
Type your answer here. Use to type LaTeX math expressions, e.g. p A q
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Task 7 - Task 10 Task 7: List the square footage, owner number, owner last name, and owner first name for each property managed by the StayWell-Columbia City office. Task 8: Repeat Task 7, but this time include only those properties with three bedrooms. Task 9: List the office number, address, and monthly rent for properties whose owners live in Washington State or own two-bedroom properties. Task 10: List the office number, address, and monthly rent for properties whose owners live in Washington State and own a two-bedroom property.arrow_forwardTools for validators should be specified.arrow_forwardYou may use the Boolean operator to search for data on two separate topics at once. To get the most relevant results, Kevin should utilize which of the following Boolean operators?arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
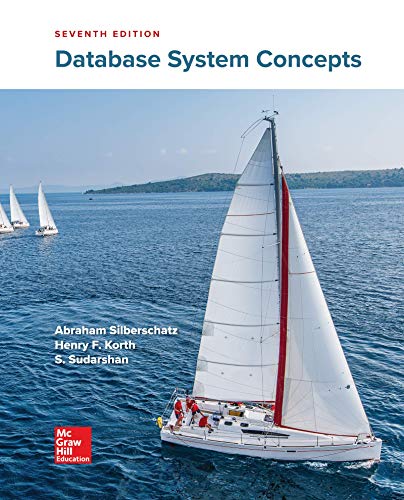
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
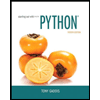
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
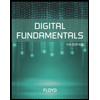
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
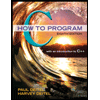
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
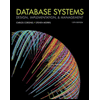
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
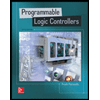
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education