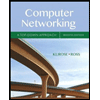
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
![Write a well-documented, Python program, hmwk3Q4.py that implements the Bubble-Sort algorithm. Bubble-Sort
iterates over a list of numbers, comparing the elements in pairs and swaps them if the first element in the pair is
larger than the second. Swapping continues until the largest number "bubbles" to the top of the list, while the smallest
element stays at the bottom. Implement the algorithm that accepts an unsorted list and returns a sorted one - in
ascending order.
5 3
لنا
8
3 5 8
3 5 8
3
3 5 4
5 4
4
4
8
4 6
LO
6
6
6
6
8
Initial List
Swap
Do Not Swap
Swap
Swap
3
3
5 4
5
●●●
Bubble Sort Algorithm
6
6
8
8
2nd Pass
Swap
●●●
Nth Pass
Hints:
Convince yourself that the algorithm and Python implementation is correct by calling the sorting function with a list
[50,20,10,80,40], and printing the sorted result.
Your algorithm should use a while-loop to ensure that the ascending order is achieved.](https://content.bartleby.com/qna-images/question/d8cbd1f0-fbe3-46ea-b49e-77385d6c8a18/95afc0ef-937b-4b16-8bd9-dda5d107a1a6/0v0ubyu_thumbnail.png)
Transcribed Image Text:Write a well-documented, Python program, hmwk3Q4.py that implements the Bubble-Sort algorithm. Bubble-Sort
iterates over a list of numbers, comparing the elements in pairs and swaps them if the first element in the pair is
larger than the second. Swapping continues until the largest number "bubbles" to the top of the list, while the smallest
element stays at the bottom. Implement the algorithm that accepts an unsorted list and returns a sorted one - in
ascending order.
5 3
لنا
8
3 5 8
3 5 8
3
3 5 4
5 4
4
4
8
4 6
LO
6
6
6
6
8
Initial List
Swap
Do Not Swap
Swap
Swap
3
3
5 4
5
●●●
Bubble Sort Algorithm
6
6
8
8
2nd Pass
Swap
●●●
Nth Pass
Hints:
Convince yourself that the algorithm and Python implementation is correct by calling the sorting function with a list
[50,20,10,80,40], and printing the sorted result.
Your algorithm should use a while-loop to ensure that the ascending order is achieved.
Expert Solution

arrow_forward
Step 1
CODE IN PYTHON:
def bubbleSort(inputList): n = len(inputList) for i in range(n-1): isSorted = True for j in range(n-1): if inputList[j] > inputList[j+1]: temp = inputList[j] inputList[j] = inputList[j+1] inputList[j+1] = temp isSorted = False if isSorted: break return inputList inputList = [50, 20, 10, 80, 40] print("Before sorting : ", inputList) inputList = bubbleSort(inputList) print("After sorting: ", inputList)
INDENTATION:
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

Knowledge Booster
Similar questions
- Implement a simple linked list in Python (Write source code and show output) with basic linked list operations like: (a) create a sequence of nodes and construct a linear linked list. (b) insert a new node in the linked list. (b) delete a particular node in the linked list. (c) modify the linear linked list into a circular linked list. Use this template: class Node:def __init__(self, val=None):self.val = valself.next = Noneclass LinkedList:"""TODO: Remove the "pass" statements and implement each methodAdd any methods if necessaryDON'T use a builtin list to keep all your nodes."""def __init__(self):self.head = None # The head of your list, don't change its name. It shouldbe "None" when the list is empty.def append(self, num): # append num to the tail of the listpassdef insert(self, index, num): # insert num into the given indexpassdef delete(self, index): # remove the node at the given index and return the deleted value as an integerpassdef circularize(self): # Make your list circular.…arrow_forwardJavaarrow_forwardORIGINAL Q: Instead of reading in an entire list N1,N2,⋯N1,N2,⋯ all at once, some algorithms (depending on the task to be done) can read in only one element at a time and process that single element completely before inputting the next one. This can be a useful technique when the list is very big (e.g., billions of elements) and there might not be enough memory in the computer to store it in its entirety. Write an algorithm that reads in a sequence of values v≥0, one at a time, and computes the average of all the numbers. You should stop the computation when you input a value of V = −1. Do not include this negative value in your computations; it is not a piece of data but only a marker to identify the end of the list. PLEASE MODIFY THE FOLLOWING CODE SO THE USER INPUTS THE NUMBERS ONE BY ONE: ORIGINAL Q: Instead of reading in an entire list N1,N2,⋯N1 ,N2,⋯ all at once, some algorithms (depending on the task to be done) can read in only one element at a time and process that single…arrow_forward
- Write a Python program to create a list by asking the user the list size and listelements. Remove all duplicates elements from a list. : Let’s the input list is- A= [4, 7, 9, 12, 32, 4, 67, 9], output will be [7, 12, 32, 67]arrow_forwardPython Code Create a code that can plot a distance versus time graph by importing matplotlib and appending data from a text file to a list. Follow the algorithm: Import matplotlib. Create two empty lists: Time = [ ] and Distance = [ ] Open text file named Motion.txt (content attached). Append data from Motion.txt such that the first column is placed in Time list and the second column is placed in Distance list. Plot the lists (Distance vs Time Graph). You may use this following link as a source for matplotlib functions: https://datatofish.com/line-chart-python-matplotlib/ Show Plot.arrow_forwardWrite a program that implements a sorted list using dynamic allocated arrays. DataFile.txt contains the information of poker cards. C: clubs(lowest),D: diamonds, H: hearts, S:spades(highest) 2 (lowest), 3, 4, 5, 6, 7, 8, 9, 10, J, Q, K, A No Joker cards Any C cards are lower than any D cards. DataFile Content(You can write the file specification into your program.): H4,C8,HJ,C9,D10,D5,DK,D2,S7,DJ,H3,H6,S10,HK,DQ,C2,CJ,C4,CQ,D8,C3,SA,S2,HQ,S8,C6,D9,S3,SQ ,C5,S4,H5,SJ,D3,H8,CK,S6,D7,S9,H2,CA,C7,H7,DA,D4,H9,D6,HA,H10,S5,C10 H4, D5, HK, D2 H4, HK, SK C9,C10 For examples, DJ means J of Diamonds;H7 means 7 of hearts. Your job Create a list by dynamic allocated array and set the size to 20 Read the first 20 cards in the first line of the file, the put them one by one into the list by implementing and using putItem(). The list must be kept sorted in ascending order. Then print out all the cards in the list in one line separating by commas. Then delete the cards indicated in the second…arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
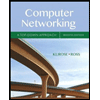
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
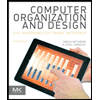
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
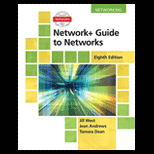
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
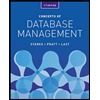
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
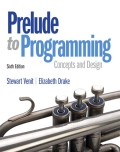
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
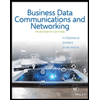
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY