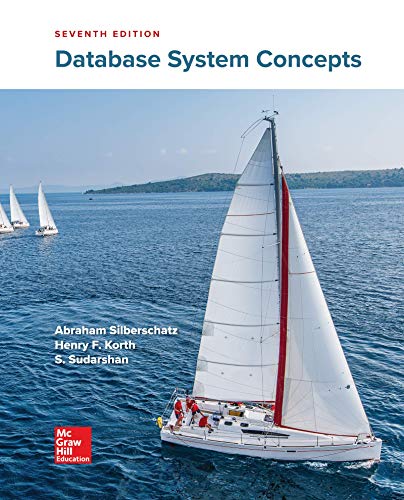
Write Python commands, within a print command, that output the following. (E.g. print(list(range(10))) ), in spyder
(i) The penultimate element of the list b where b = list(range(-41,10))
(ii) The middle element of the list c where c = 10*a+5*b

Algorithm to Calculate and Print Penultimate and Middle Elements of Lists
1. Create a list 'a' containing numbers from 0 to 9.
2. Create a list 'b' containing numbers from -41 to 9.
3. Initialize an empty list 'c' to store the results.
4. Iterate through the elements of 'a' and 'b' in parallel:
- For each pair of corresponding elements (a_i, b_i), calculate 10 * a_i + 5 * b_i.
- Append the result to the 'c' list.
5. Calculate the penultimate element of list 'b' by accessing 'b[-2]'.
6. Calculate the middle element of list 'c' by finding the index 'len(c) // 2' and accessing the element at that index.
7. Print the penultimate element of list 'b' and the middle element of list 'c'.
Step by stepSolved in 4 steps with 2 images

- Written in python with docstrings if applicable. Thanksarrow_forwardAnswer fast…. create a python functions outlined below reverse_number_in_list(number_list:list)-> list This function will be given a list of numbers your job is to reverse all the numbers in the list and return a list with the reversed numbers. If a number ends with 0 you need to remove all the trailing zeros before reversing the number. An example of reversing numbers with trailing zeros: 10 -> 1, 590 -> 95. None of the numbers in the number_list will be less than 1. Example: number_list = [13, 45, 690, 57] output = [31, 54, 96, 75] tails_same(number_list:list) -> bool This function should return true if the value at the beginning and the end of the list are equal. False otherwise. Example: number_list = [1, 239, 949, 0, 84, 0, 1] output: True number_list = [1, 239, 949, 0, 84, 0, 13] output: False remove_char(str_list:list, char:str) -> list This function will be given a list of strings and a character. You must remove all occurrences of the character…arrow_forwardpython: In the Python terminal, the command dir(list) returns a list of operations that can be performed on lists. choose three (3) operations from this list and, in your own words, describe what it does, how to use it, and give your own example. Try to choose operations that have not been completed yet.arrow_forward
- However, the number of integers must be a power of 2. In main.c, key[ ] has 16 integers. But as you should alreadyknow, the program will not sort if one integers is removed or added. What you are going to do is modify the mergesort.c module so that a non-power of 2 number of integers can be sorted. When you modify a module, indicate by way of comment, what was changed, the date the change was made. You will also need to add or remove an integer to/from key[] in module sort158a.c.You are NOT allowed to change merge.c, mergesort.h or wrt.c filesThe mergesort function in file mergesort.c MUST call the merge function which it currently does.The main function in sort158a.c you can only change the number of integers in the key[] arrayBelow are 3 examples of output. The first with an array of 15 integers. The second with an array of 16 (power of 2) integers and the third with 17 integers.Example of Output with 15 integersBefore mergesort: 4 3 1 67 55 4 -5 37 7 4 2 9 1 -1 6After mergesort: -5…arrow_forwardPYTHON!!! Write a function that will filter course grades that are stored in a list, creating and returning a new list. Your implementation must have the following rules: - The function must accept two (and only two) parameters: The first is: the maximum integer value for any grade to remain in the list (any value above the maximum will be filtered and removed from the list). The second is: the list of grades (you can assume the list contains only integer values) - The function must return a new list and not modify the existing list. - The function must use a list comprehension to filter the existing list and create the new list that is returned. - DO NOT USE THE: built-in-"filter" function.arrow_forwardCreate a Python program using the following: -Create list[ "Parking:ID123", "Speeding:"ID455", "Running:ID346"] -Slice the list by ":" -Create a search such that when the user inputs a name, if the name is on the list, print the name and the corresponding ID number or return that there is no match found. -Use control statements (break/continue)arrow_forward
- Write a Python program that will read in an input file named RandomNumbers.txt and place all the positive values in a list. From there, display this list sorted in ascending order. Use at least 4 functions within the program. Remember, main() should become a to-do list calling the functions in the order in which they should execute. Each function should perform 1 task only. In addition to the above, be sure your code handles the following exception: any IOError exception that is thrown when the file is opened and data is read from it REQUIREMENTS: Use functions throughout. The function main should only call functions in the order needed. Keep the functions modular and performing only one task. Add a beginning and ending statement so the user will know when the program begins and ends. Comment throughout the code. The program should look for the file in the current directory. Example: If the input file contains the following data: 99-11044-22 The program output should be:…arrow_forwardPlease write a program that checks if the last data item entered appears earlier in the list. The input is a sequence of non-negative numbers, which is terminated by a user entering a -1. The code needs to output ONLY ONE of these 3 messages: Yes the last data item appears earlier, No the last data item appears earlier, No Data Provided The code is also not allowed to compare the last number with itself. Thanks!arrow_forwardWrite a python program that stores current grades in a dictionary, with course codes as keys and percent grades as values. Start with an empty dictionary and then use a while loop to enable input of course codes and percent grades from the keyboard. Enter data for at least five courses. After entering the data, use a for loop and the keys to show the current status of all courses. This same loop should include code that enables determination of the worst course and the average of all courses. Both of these findings should be printed when the loop ends. The worst course should be dropped and reported. This being done, the program should use another loop and the items method to display the revised courses and grades and report the revised term average.arrow_forward
- This is the questions and answers for the first parts Questions: Using Python Create a list with building names 'Richard Weeks Hall’, ’Busch Student Center’, ‘Environmental & Natural Resource Sciences Building’ Replace the list to 'Richard Weeks’, ’Busch Student Center’, ‘Environmental & Natural Resource Sciences Bldg’ Code for the total points of each name by setting• The base points as the length of the building name.• The bonus points: as ‘Bldg’ being equal to 10 points Meaning, 'Academic Building' which would be 'Academic Bldg' would have a base point of 13 and a bonus point of 10 for a total of 23. #1 Create a list of buildings Buildings=[ 'Richard Weeks Hall', 'Busch Student Center', 'Environmental & Natural Resource Sciences Building' ] print("The Building names in the list are: ") print(Buildings) #2 Process the name of each building res = [sub.replace(' Hall', '').replace('Building', 'Bldg') for sub in Buildings] print("The names have been replaced to :…arrow_forwardComplete the Python program below to list all language names and number of related articles in the order they appear in the URL below from urllib.request import urlopenfrom bs4 import BeautifulSouphtml = urlopen('https://www.wikipedia.org/')bs = BeautifulSoup(html, "html.parser") nameList = #TO DO -- Complete the Codefor name in nameList: print(name.get_text())arrow_forwardpythonarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
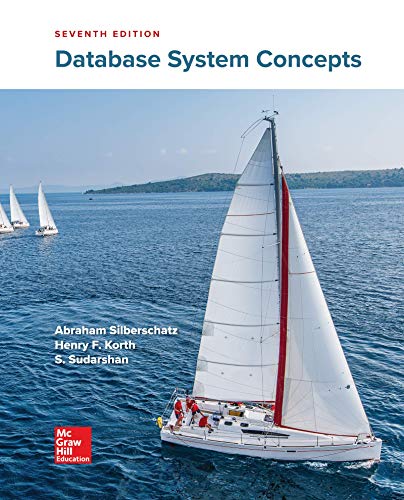
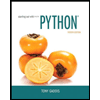
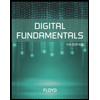
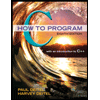
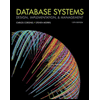
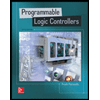