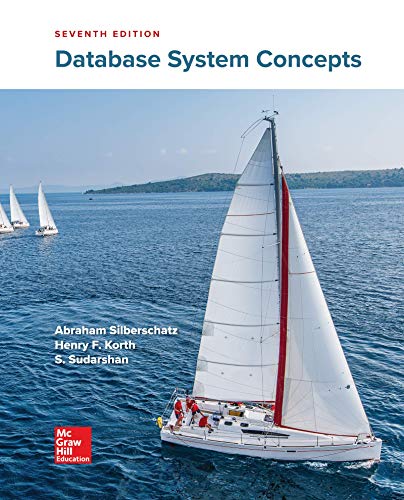
Please write the full set of logic pseudocode include all abstractions and mainline logic of the given code below:
Correct terminology used
Declarations are made with data types
create a file initial.py and write below code
#import random module
import random
def point():
#random integer stored
number = random.randint(1,100)
print("please guess number:")
print("%s number have you guessed"%number)
print("if yes please enter yes else enter no")
return number
run above code
create another file computer.py and write below code
#import random library for generating 1-100
import random
import initial
#define main function
def main():
#call module method
number = initial.point()
m = input()
if(m=="yes"):
return "computer win the game"
#no
elif(m=="no"):
for i in range(6):
print("guessing number was greater than or less than:")
print("enter g for greater than:")
print("enter l for less than:")
#for greater or less
o = input()
if(o == 'g'):
#generate random number again
number = random.randint(number,100)
print("%s number have you guessed"%number)
#for yes or no
p = input()
if(p=="yes"):
return "computer win the game after %s"+str(i+2)
else:
continue
#condition for less than
elif(o=='l'):
number = random.randint(1,number)
print("%s number have you guessed"%number)
p = input()
if(p=="yes"):
return "computer win the game after %s"+str(i+1)
else:
continue
else:
print("please enter valid keyword")
i -= 1
continue
else:
return "computer loss the game after 7 times:"
#call main function
print(main())

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- Customized step counter Learning Objectives In this lab, you will Create a function to match the specifications Use floating-point value division Instructions A pedometer treats walking 2,000 steps as walking 1 mile. It assumes that one step is a bit over 18 inches (1 mile = 36630 inches, so the pedometers assume that one step should be 18.315 inches). Let's customize this calculation to account for the size of our stride. Write a program whose input is the number of steps and the length of the step in inches, and whose output is the miles walked. Output each floating-point value with two digits after the decimal point, which can be achieved as follows: print(f'{your_value:.2f}') Ex: If the input is: 5345 18.315 the output is: You walked 5345 steps which are about 2.67 miles. Your program must define and call the following function. The function should return the number of miles walked.def steps_to_miles(user_steps, step_length) # Define your function here if __name__…arrow_forwardData Management Functions - Name format. Python*a) Define a function nameFormat() with parameters first, middle, and last.-This function prints the first name, the middle initial and the last name using proper title format.-Call the function nameFormat with these positional arguments: john stu smith-Call the function nameFormat with these keyword arguments: last=‘kennedy’, first=‘john’, middle=‘fitzgerald’ Example Output John S. SmithJohn F. Kennedyarrow_forwardC++ Coding: Arrays Implement a two-dimensional character array. Use a nested loop to store a 12x6 flag. 5 x 2 stars as * Alternate = and – to represent stripe colors Use a nested loop to output the character array to the console.arrow_forward
- can somone help me with this in c languagearrow_forwardMemory Management Programming Assignment Please if this can be coded in Java or C++ i would appreciate implement and test the GET-MEMORY algorithm This algorithm uses the Next-Fit(First-Fit-With-A-Roving-Pointer) technique. implement and test the FREE-MOMORY algorithm Implement the “GET_MEMORY” and “FREE_MEMORY” algorithms. Comprehensive testing must be done for each algorithm. Following are sample run results for each: GET_MEMORY IS RUNNING……… Initial FSB list FSB# Location Size 1 7 4 2 14 10 3 30 20 . . . . . . Rover is 14 ---------------------------------------------------------------------------- Allocation request for 5 words Allocation was successful Allocation was in location 14 FSB# Location Size 1 7 4 2 19 5 3 30 20 . . . . . . Rover is 30 ---------------------------------------------------------------------------- Allocation request for 150 words Allocation was not successful . . . __________________________________________________________ FREE_MEMORY…arrow_forwardC Programming Write function checkHorizontal to count how many discs of the opposing player would be flipped, it should do the following a. Return type integer b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. char playerCharc. If the square to the left or right is a space, stop checking d. If the square to the left or right is the same character as the player’s character, save that it was a flank, stop checking e. If the square to the left or right is not the same character as the player’s character, count the disc f. If the counted discs is greater than zero AND the player found their own character, return the counted discs, otherwise return ZERO Write function checkVertical to count how many discs of the opposing player would be flipped, it should do the following a. Return type integer b. Parameter list i. int rowii. int col iii. char board[ROW][COL] iv. char playerCharc. If the square above or below is a space, stop checking d. If the square above or below is…arrow_forward
- Python 3 NO IMPORT PICKLE Please check the picture and add one more function(def) to display menu and fix the error in the code. Write a program that keeps names and email addresses in a dictionary as key-value pairs. You Must create and use at least 5 meaningful functions. A function to display a menu A function to look up a person’s email address A function to add a new name and email address A function to change an email address A function to delete a name and email address. This is the Required Output example: Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and email address 5. Quit the program Enter your choice: 2 Enter name: John Enter email address: John@yahoo.com That name already exists Menu ---------------------------------------- 1. Look up an email address 2. Add a new name and email address 3. Change an existing email address 4. Delete a name and…arrow_forwardPYTHON QUESTION ASSIGNMENT REQUIREMENTS This assignment requires a dictionary of state abbreviations and their capital cities. The abbreviations are the keys and the state capitals are the values. Start by entering data for any four states of your choice. Then, report this count but use a function to get the count. Use a while loop to add more abbreviations and capitals. The loop should continue until the user presses Enter when prompted for an abbreviation. Inside the while loop: If the state entered is already in the dictionary, report its capital. If it is not in the dictionary, prompt the user to enter the capital for that state and add it to the dictionary After the while loop ends: Report again the count of the number of states in the dictionary. Using another loop and the items() method, display all the state abbreviations and their capitals. In the same Python program write this code, although it has nothing to do with the states code. Using a dictionary…arrow_forwardstruct employee{int ID;char name[30];int age;float salary;}; (A) Using the given structure, Help me with a C program that asks for ten employees’ name, ID, age and salary from the user. Then, it writes the data in a file named out.txt (B) For the same structure, read the contents of the file out.txt and print the name of the highest salaried employee and youngest employee names name in the outputscreen.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
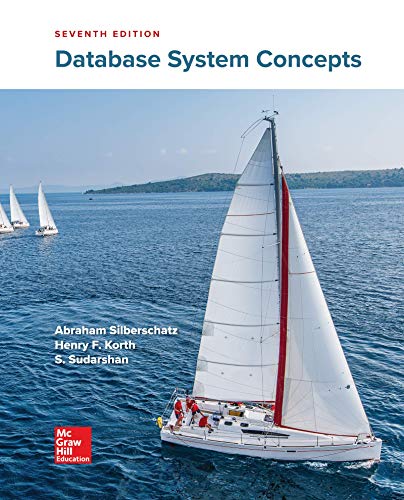
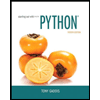
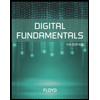
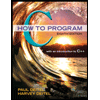
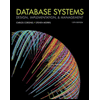
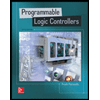