Write the function readSalesData. #include #include #include #include #include using namespace std; // structure definition struct Sales { string name; int amount; }; // function prototypes double detCommissionPercent(int sales); double detAmountEarned(int sales); Sales* readSalesData(string filename, int& noPeople); int calculateTotal(Sales* list, int noPeople); void showSalesData(Sales* list, int noPeople, int total); void saveSalesData(string filename, Sales* list, int noPeople, int total); int main() { // pointer to be used to dynamically allocate an array of Sales structures Sales *list; // other variables int noPeople; // number of sales people string filename; // input file name int total; // function calls cout << "Enter input file name: "; // without extension cin >> filename; filename += ".txt"; // call readSalesData ifstream inFile; //Open the file inFile.open(filename.c_str()); //Test for file open errors if (!inFile) { cout << "Error opening the input file: " << filename << "." << endl; exit (101); }//if inFile >> noPeople; //Display error when number of salesperson is 0 or negaative if (noPeople <= 0) { cout << "The array size is invalid." << endl; cout << "The program ends here!" << endl; return 0; } else { list = new Sales[noPeople]; readSalesData (filename, noPeople); } // call calculateTotal total = calculateTotal (list, noPeople); string answer; cout << "\nShow Report(Y/N)? "; cin >> answer; if (answer == "y" || answer == "Y") { // call showSalesData showSalesData (list, noPeople, total); } // call saveSalesData saveSalesData (filename, list, noPeople, total); delete [] list; return 0; } /* Write your code here. For each function definition write a short comment too, as demonstrated in previous assignments */ /*~*~*~*~*~*~ This function determines the commision percent based on the following table: Sales Commission $0 - 1000 3% 1001 - 5000 4.5% 5001 - 10,000 5.25% over 10,000 6% *~*/ double detCommissionPercent(int sales) { double commission = 0; /*Write your code here */ if (sales <= 1000) { commission = 3; } else if (sales <= 5000) { commission = 4.5; } else if (sales <= 10000) { commission = 5.25; } else { commission = 6; } return commission; } /*~*~*~*~*~*~ This function determines the amount earned: it calls the detCommisionPercent) function. *~*/ double detAmountEarned(int sales) { double amountEarned = 0; /* Write your code here */ amountEarned = detCommissionPercent(sales) * sales / 100.0; return amountEarned; } ///////////////////////////////////// //Calculate all data and add to the total int calculateTotal(Sales *list, int noPeople) { int total = 0; for(int i = 0; i < noPeople; i++) { total += list[i].amount; } return total; }//calculateTotal ///////////////////////////////////// //Read the sales data from file and store it in an array of structures Sales* readSalesData(string filename, int& noPeople) { }//readSalesData /////////////////////////////////////////// /*Display the amount sold, names, comission percent, and amount earned for each person on screen. It also shows the total amount earned by all sales persons.*/ void showSalesData(Sales* list, int noPeople, int total) { cout << "ABC Company Report" << endl; for(int i = 0; i < noPeople; i++) { cout << "$"; cout << right << setw(6) << list[i].amount; cout << " " << list[i].name << ","; cout << fixed << setprecision(2) << " " << detCommissionPercent(list[i].amount); cout << "% ($" << detAmountEarned(list[i].amount) << ")" << endl; } cout << "Total Sales: $" << total << endl; }//showSalesData ///////////////////////////////////////////// //Save data void saveSalesData(string filename, Sales* list, int noPeople, int total) { string out_file_name = ""; for(int i = 0; filename[i]; i++) { if(filename[i] == '.') break; out_file_name += filename[i]; } out_file_name += "_report.txt"; ofstream fout(out_file_name); fout << "ABC Company Report" << endl; for(int i = 0; i < noPeople; i++) { fout << "$"; fout << right << setw(6) << list[i].amount; fout << " " << list[i].name << ","; fout << fixed << setprecision(2) << " " << detCommissionPercent(list[i].amount) << "%"; fout << " ($" << detAmountEarned(list[i].amount) << ")" << endl; } fout << "Total Sales: $" << total; cout << "The Sales Report has been saved to \"" << out_file_name << "\"" << endl; }
Write the function readSalesData.
#include <iostream>
#include <iomanip>
#include <fstream>
#include <sstream>
#include <string>
using namespace std;
// structure definition
struct Sales {
string name;
int amount;
};
// function prototypes
double detCommissionPercent(int sales);
double detAmountEarned(int sales);
Sales* readSalesData(string filename, int& noPeople);
int calculateTotal(Sales* list, int noPeople);
void showSalesData(Sales* list, int noPeople, int total);
void saveSalesData(string filename, Sales* list, int noPeople, int total);
int main()
{
// pointer to be used to dynamically allocate an array of Sales structures
Sales *list;
// other variables
int noPeople; // number of sales people
string filename; // input file name
int total;
// function calls
cout << "Enter input file name: "; // without extension
cin >> filename;
filename += ".txt";
// call readSalesData
ifstream inFile;
//Open the file
inFile.open(filename.c_str());
//Test for file open errors
if (!inFile)
{
cout << "Error opening the input file: " << filename << "." << endl;
exit (101);
}//if
inFile >> noPeople;
//Display error when number of salesperson is 0 or negaative
if (noPeople <= 0) {
cout << "The array size is invalid." << endl;
cout << "The program ends here!" << endl;
return 0;
}
else {
list = new Sales[noPeople];
readSalesData (filename, noPeople);
}
// call calculateTotal
total = calculateTotal (list, noPeople);
string answer;
cout << "\nShow Report(Y/N)? ";
cin >> answer;
if (answer == "y" || answer == "Y") {
// call showSalesData
showSalesData (list, noPeople, total);
}
// call saveSalesData
saveSalesData (filename, list, noPeople, total);
delete [] list;
return 0;
}
/* Write your code here. For each function definition write a short comment too,
as demonstrated in previous assignments */
/*~*~*~*~*~*~
This function determines the commision percent based on the following table:
Sales Commission
$0 - 1000 3%
1001 - 5000 4.5%
5001 - 10,000 5.25%
over 10,000 6%
*~*/
double detCommissionPercent(int sales)
{
double commission = 0;
/*Write your code here */
if (sales <= 1000) {
commission = 3;
}
else if (sales <= 5000) {
commission = 4.5;
}
else if (sales <= 10000) {
commission = 5.25;
}
else {
commission = 6;
}
return commission;
}
/*~*~*~*~*~*~
This function determines the amount earned:
it calls the detCommisionPercent) function.
*~*/
double detAmountEarned(int sales)
{
double amountEarned = 0;
/* Write your code here */
amountEarned = detCommissionPercent(sales) * sales / 100.0;
return amountEarned;
}
/////////////////////////////////////
//Calculate all data and add to the total
int calculateTotal(Sales *list, int noPeople)
{
int total = 0;
for(int i = 0; i < noPeople; i++) {
total += list[i].amount;
}
return total;
}//calculateTotal
/////////////////////////////////////
//Read the sales data from file and store it in an array of structures
Sales* readSalesData(string filename, int& noPeople)
{
}//readSalesData
///////////////////////////////////////////
/*Display the amount sold, names, comission percent,
and amount earned for each person on screen.
It also shows the total amount earned by all sales persons.*/
void showSalesData(Sales* list, int noPeople, int total)
{
cout << "ABC Company Report" << endl;
for(int i = 0; i < noPeople; i++)
{
cout << "$";
cout << right << setw(6) << list[i].amount;
cout << " " << list[i].name << ",";
cout << fixed << setprecision(2) << " " << detCommissionPercent(list[i].amount);
cout << "% ($" << detAmountEarned(list[i].amount) << ")" << endl;
}
cout << "Total Sales: $" << total << endl;
}//showSalesData
/////////////////////////////////////////////
//Save data
void saveSalesData(string filename, Sales* list, int noPeople, int total)
{
string out_file_name = "";
for(int i = 0; filename[i]; i++)
{
if(filename[i] == '.')
break;
out_file_name += filename[i];
}
out_file_name += "_report.txt";
ofstream fout(out_file_name);
fout << "ABC Company Report" << endl;
for(int i = 0; i < noPeople; i++)
{
fout << "$";
fout << right << setw(6) << list[i].amount;
fout << " " << list[i].name << ",";
fout << fixed << setprecision(2) << " " << detCommissionPercent(list[i].amount) << "%";
fout << " ($" << detAmountEarned(list[i].amount) << ")" << endl;
}
fout << "Total Sales: $" << total;
cout << "The Sales Report has been saved to \"" << out_file_name << "\"" << endl;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 9 images

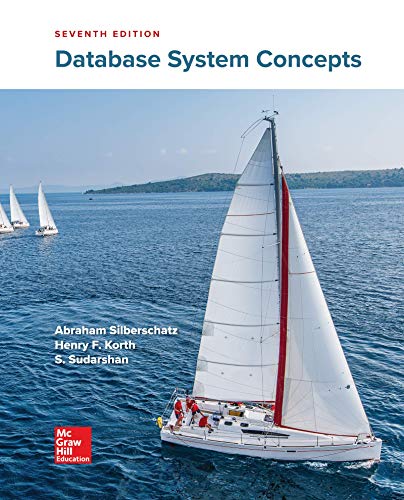
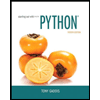
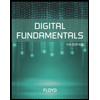
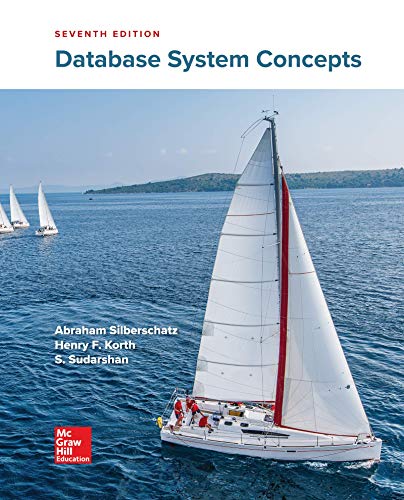
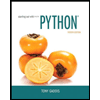
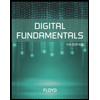
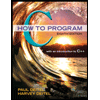
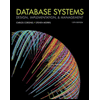
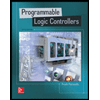