Write the resize function for the dynamic, partially-filled array below. Follow the comments to complete the function. dynamic.cpp 1 #include 2 #include 3 #include "debug_new.h" 4 #include "array.h" 5 using namespace std; 7 /** 8. Resize a dynamic chunk of memory. @param arg a pointer to the beginning of the array @param size the current allocated size @return a pointer to an initialized 2x chunk. 10 11 12 */ 13 int* resize(int* a, int size) 14 { 15 // Allocate a new array twice the size of the original 16 // Copy the original elements into the new array 17 |/ Free original array 18 |/ Return new array 19 } 20 21 int main(int argc, char* argv[]) 22 { 23 int size = 0; int capacity = 2; // initial capacity is 2 int *a = new int[capacity]; 24 25 26 27 cout « "Enter positive numbers (negative to quit): "; int n; cin >> n; while (n >= 0) 28 29 30
Write the resize function for the dynamic, partially-filled array below. Follow the comments to complete the function. dynamic.cpp 1 #include 2 #include 3 #include "debug_new.h" 4 #include "array.h" 5 using namespace std; 7 /** 8. Resize a dynamic chunk of memory. @param arg a pointer to the beginning of the array @param size the current allocated size @return a pointer to an initialized 2x chunk. 10 11 12 */ 13 int* resize(int* a, int size) 14 { 15 // Allocate a new array twice the size of the original 16 // Copy the original elements into the new array 17 |/ Free original array 18 |/ Return new array 19 } 20 21 int main(int argc, char* argv[]) 22 { 23 int size = 0; int capacity = 2; // initial capacity is 2 int *a = new int[capacity]; 24 25 26 27 cout « "Enter positive numbers (negative to quit): "; int n; cin >> n; while (n >= 0) 28 29 30
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter8: Arrays And Strings
Section: Chapter Questions
Problem 1TF: Mark the following statements as true or false. A double type is an example of a simple data type....
Related questions
Question
100%
fill in code under the comments, in C++
![Write the resize function for the dynamic, partially-filled array below. Follow the comments to
complete the function.
dynamic.cpp
1 #include <iostream>
2 #include <string>
3 #include "debug_new.h"
#include "array.h"
using namespace std;
6
4
7
/**
Resize a dynamic chunk of memory.
@param arg a pointer to the beginning of the array
@param size the current allocated size
@return a pointer to an initialized 2x chunk.
*/
8.
9
10
11
12
13
int* resize(int* a, int size)
{
// Allocate a new array twice the size of the original
// Copy the original elements into the new array
// Free original array
// Return new array
14
15
16
17
18
19
}
20
21
int main(int argc, char* argv[])
22
{
23
int size = 0;
int capacity = 2; // initial capacity is 2
int *a = new int[capacity];
24
25
26
27
cout « "Enter positive numbers (negative to quit): ";
int n;
cin >> n;
while (n >= 0)
28
29
30](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F45a9ec70-4831-4f65-a272-c2b5558c4300%2F3eae9c60-7c53-4f74-a68c-6420c08a2cc0%2F5528fs_processed.png&w=3840&q=75)
Transcribed Image Text:Write the resize function for the dynamic, partially-filled array below. Follow the comments to
complete the function.
dynamic.cpp
1 #include <iostream>
2 #include <string>
3 #include "debug_new.h"
#include "array.h"
using namespace std;
6
4
7
/**
Resize a dynamic chunk of memory.
@param arg a pointer to the beginning of the array
@param size the current allocated size
@return a pointer to an initialized 2x chunk.
*/
8.
9
10
11
12
13
int* resize(int* a, int size)
{
// Allocate a new array twice the size of the original
// Copy the original elements into the new array
// Free original array
// Return new array
14
15
16
17
18
19
}
20
21
int main(int argc, char* argv[])
22
{
23
int size = 0;
int capacity = 2; // initial capacity is 2
int *a = new int[capacity];
24
25
26
27
cout « "Enter positive numbers (negative to quit): ";
int n;
cin >> n;
while (n >= 0)
28
29
30
![29
while (n >= 0)
{
if (size ==
{
a = resize(a, size); // Write this function
capacity *= 2;
}
a[size] = n;
cin >> n;
size++;
30
31
32
сараcity)
33
34
35
36
37
38
39
40
print ("a->", a, size);
delete[] a;
41
42
43 }](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F45a9ec70-4831-4f65-a272-c2b5558c4300%2F3eae9c60-7c53-4f74-a68c-6420c08a2cc0%2Fb2wvaml_processed.png&w=3840&q=75)
Transcribed Image Text:29
while (n >= 0)
{
if (size ==
{
a = resize(a, size); // Write this function
capacity *= 2;
}
a[size] = n;
cin >> n;
size++;
30
31
32
сараcity)
33
34
35
36
37
38
39
40
print ("a->", a, size);
delete[] a;
41
42
43 }
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
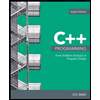
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
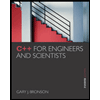
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
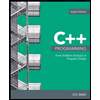
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
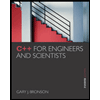
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr