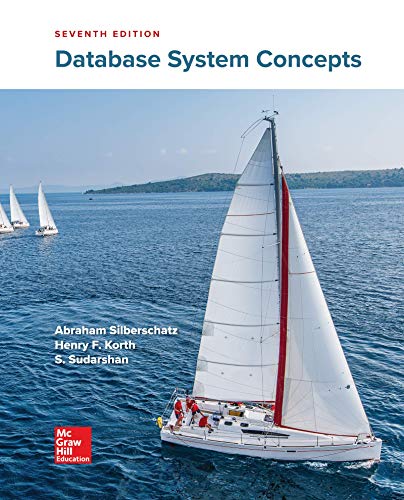
Python (this task is NOT graded, this is work for practice)
write two functions with the following tasks:
-Function 1 (called function):
accept two arguments: a low acceptable limit, and a high acceptable limit. The low
limit MUST be a negative number, while the high limit MUST be a positive
number, and your code must verify those two conditions.
if the arguments do not meet the conditions above, the function should return the
message “Wrong! try again” to the calling function.
if the arguments are accepted, the function will ask the user to enter a single number
between the low limit and the high limit.
if the user enters a single number that falls outside the specified range, the function
should emit the message Error: the value is not within permitted range (min..max) and
ask the user to input the value again;
if the input value is valid, return it as a result.
-Function 2 (caller function)
No argument is passed to this function
The function prompts the user to enter the two numbers to be passed to the called
functions.
If the return value is “Wrong! try again” then the user is prompted to enter two other
numbers.
If the return value is the single number the user entered, then the user is asked if he or
she wants to continue. If yes, the function should continue running until the user
inputs -1, -1 as number to end the loop

Step by stepSolved in 3 steps with 1 images

- Code is in Pythonarrow_forwardInclude pseudocode that describes all steps required to solve the problem. Employ variable names that describe the values they store and adhere to Python naming conventions. Include additional comments as needed to annotate your code. Use correct spelling and grammar. Use f_strings to output variable values. Use the "dunders test" for __name__ equals __main__ 1. Write a program that contains a main function and a custom, void function named show_larger that takes two random integers as parameters. This function should display which integer is larger and by how much. The difference must be expressed as a positive number if the random integers differ. If the random integers are the same, show_larger should handle that, too. See example outputs. In the main function, generate two random integers both in the range from 1 to 5 inclusive, and call show_larger with the integers as arguments.EXAMPLE OUTPUT 13 is larger than 1 by 2EXAMPLE OUTPUT 2The integers are equal, both are 3 2. Write a…arrow_forwardAn automated donation machine accepts donations via 10 or 20 bills to a maximum of $990. The machine needs to print a receipt with the donation amount in English, rather than numerically. Complete the following function which converts any number between 10 to 990 dollars to English.NOTE: You may define and use another function if necessary.NOTE: You should use arrays for this task. Use of switch statements or very long if/else if statements for printing is not allowed. However, using if/else if/else statements for the logic of your algorithm is permitted.NOTE: This function returns the result, and does not print it. Make sure the result is not lost when you return. Example function input and output (donation is 110):Input: 110Output: “one hundred ten dollars” string convertToEnglish (int donation); in C++ #include <iostream>#include <string>using namespace std; string convertToEnglish (int donation); int main() {int donation;cin>>donation;…arrow_forward
- The prompt asks: Write a function that calculates the amount of money a person would earn over a period of years if his or her salary is one penny the first day, two pennies the second day, and continues to double each day. The program should ask the user for the number of years and call the function which will return the total money earned in dollars and cents, not pennies. Assume there are 365 days in a year. function totalEarned(years) { } var yearsWorked = parseInt(prompt('How many years will you work for pennies a day? ')); alert('Over a total of ' + yearsWorked + ', you will have earned $' + totalEarned(yearsWorked));arrow_forwardJS You are writing a number guessing program where the player must try to guess the secret number 17. This function will take a player's guess and tell them if they are either right or whether they should guess higher or lower on their next turn Write a function named "higher_lower" that takes an int as a parameter and returns "higher" if 17 is greater than the input and "lower" if 17 is less than the input, and "correct" if 17 is equal to the input.arrow_forwardWhy would you want to use a return statement in a void function, you may wonder.arrow_forward
- def truth_value(integer): """Convert an integer into a truth value.""" # Convert 0 into False and all other integers, # including 1, into True if integer == 0: truth_value = False else: truth_value = True return truth_value Now, write a function that goes in the opposite direction of the truth_value() function. This new function should convert a truth value into an integer. In particular, True should be converted into 1 and False into 0. Name the new function integer_0_or_1(). All you need to include below is your definition of the function integer_0_or_1(). It will be tested automatically against relevant inputs. So include your function and nothing more.arrow_forwardPlease tell me where I get wrong.arrow_forwardFunctions like print which perform an action but don’t return a value are called: Select one: a. recursive functions b. built-in functions c. simple functions d. utility functions e. void functionsarrow_forward
- Create a function that takes a number as an argument and returns "Fizz", "Buzz" or "FizzBuzz" If the number is a multiple of 3 the output should be "Fizz" • If the number given is a multiple of 5, the output should be "Buzz" If the number given is a multiple of both 3 and 5, the output should be "FizzBuzz" ● • If the number is not a multiple of either 3 or 5, the number should be output on its own as shown in the examples below. • The output should always be a string even if it is not a multiple of 3 or 5. Examples fizz buzz (3) → "Fizz" fizz buzz (5) → "Buzz" fizz buzz (15) ➡ "FizzBuzz" fizz buzz (4) "4" (Ctrl)arrow_forwardPython Programming Only (PLEASE INCLUDE INPUT VALIDATION IF NEEDED) Write a function named maximum that accepts two integer values as arguments and returns the value that is the greater of the two. For example, if two numbers are one big and one small the function should return the bigger number. Use the function in a program that prompts the user to enter two integer values. The program should display the value that is the greater of the two.arrow_forwardPython code Screenshot and output is mustarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
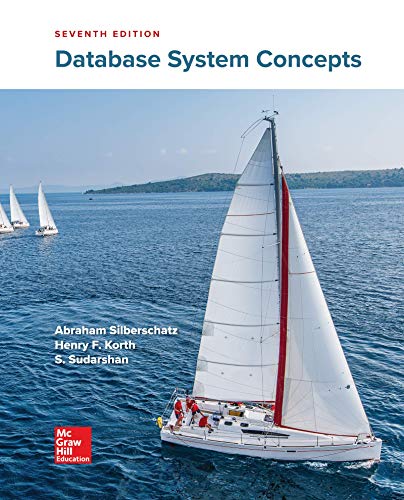
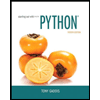
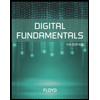
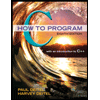
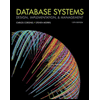
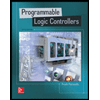