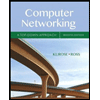
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN: 9780133594140
Author: James Kurose, Keith Ross
Publisher: PEARSON
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
X462: Arrays - Find Min Write The Method FindMin That Will Return The Smallest Number In An Array Of Integers.
![**X462: Arrays - Find Min**
Write the method `findMin` that will return the smallest number in an array of integers.
**Examples:**
```plaintext
findMin({29,2,32,12}) -> 2
```
**Your Answer:**
```java
public int findMin(int[] numbers) {
}
```
**Explanation:**
The task is to implement a method named `findMin` that takes an array of integers as input and returns the smallest integer in that array. The example provided demonstrates the expected output when the function is called with the array `{29,2,32,12}`. The method should return `2`, which is the smallest number in the array.
The code block presented under "Your Answer" is an incomplete method declaration in Java. The implementation should iterate through the array elements, compare the values, and keep track of the minimum number found so far.](https://content.bartleby.com/qna-images/question/18685f1a-91cd-4afd-8e04-fa75f11e284d/5f367c46-ee67-40eb-8e43-50c36bb4a0af/yu8vuis_thumbnail.png)
Transcribed Image Text:**X462: Arrays - Find Min**
Write the method `findMin` that will return the smallest number in an array of integers.
**Examples:**
```plaintext
findMin({29,2,32,12}) -> 2
```
**Your Answer:**
```java
public int findMin(int[] numbers) {
}
```
**Explanation:**
The task is to implement a method named `findMin` that takes an array of integers as input and returns the smallest integer in that array. The example provided demonstrates the expected output when the function is called with the array `{29,2,32,12}`. The method should return `2`, which is the smallest number in the array.
The code block presented under "Your Answer" is an incomplete method declaration in Java. The implementation should iterate through the array elements, compare the values, and keep track of the minimum number found so far.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

Knowledge Booster
Similar questions
- Q1: Write a program that creates an array of integers size 10. And do the following- a) Ask the user to enter 10 numbers (all > 0) one by one and store them in the array. If user enters a number < 0 then program should ask user to enter the number again with a message that "Pl enter a number > 0" b)Print the smallest number c) Print the largest number d)Print the averagearrow_forwardJAVA PROGRAM Chapter 7. PC #16. 2D Array Operations Write a program that creates a two-dimensional array initialized with test data. Use any primitive data type that you wish. The program should have the following methods: • getTotal. This method should accept a two-dimensional array as its argument and return the total of all the values in the array. • getAverage. This method should accept a two-dimensional array as its argument and return the average of all the values in the array. • getRowTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The method should return the total of the values in the specified row. • getColumnTotal. This method should accept a two-dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The method should return the…arrow_forwardFor Beginners Java: Write a program that ask the user to enter a list of positive scores until the user enters a negative score to terminate the input. You need to store these scores in an array. You can assume the maximum number of scores the user may enter is 50. However, you need to keep track of the actual number of scores entered. Write 2 methods: 1. calculateAverage(): this method takes the list of scores and return the average score. 2. countPerfectScores(): this method takes the list of scores and return the number of perfect scores (100). The main program reads the input and calls these methods and print the results. Please make sure you write a comment line to document what your method does.arrow_forward
- ANSWER IN JAVA: Write an application that inputs five numbers, each between 10 and 100, inclusive. As each number is read, display it only if it’s not a duplicate of a number already read. Provide for the “worst case,” in which all five numbers are different. Use the smallest possible array to solve this problem. Display the complete set of unique values input after the user enters each new value. NOTE: looking for the output to appear on three lines as shown in the results STANDARD OUTPUT: Enter·an·integer·between·10·and·100:This·is·the·first·time·100·has·been·entered↵ Enter·an·integer·between·10·and·100:Enter·an·integer·between·10·and·100:This·is·the·first·time·10·has·been·entered↵ Enter·an·integer·between·10·and·100:This·is·the·first·time·20·has·been·entered↵ Enter·an·integer·between·10·and·100:The·complete·set·of·unique·values·entered·is:↵ Unique·Value·1:·is·100↵ Unique·Value·2:·is·10↵ Unique·Value·3:·is·20↵arrow_forwardIN C++ Write a method that lets you insert a number anywhere in an array that is already full. For instance, if you have an array of size 5: 3,6,1,2,0. You should be able to insert the number 9 in some desired/specified position. E.g 3,6,1,9,2,0.arrow_forwardjavaarrow_forward
- 1) Write a method that returns the range of an array of integers. The range is the difference between the highest and lowest values in the array. The signature of the method should be as follows. public static int computeRange(int[] values)arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forward5arrow_forward
- Write java code that recursively calculates the Sum of the array cells. Program requirements: Create an array named MyArr and initialize it as follow: 19 27 81 243 88 1- Write a method named ArrSum that recursively calculates the Sum of the numbers in the Array and returns the Sum of the numbers. Hint: Method with 1 parameter (the Array) that returns 1 value (the Array's Sum). 2- Write a method named Display that displays the Array contents and Sum of the numbers. Hint: Method with 2 parameters (the Array) and Sum with no returns (void) 3- Your program output format must be the same as the following: =>> Array Summation Array: 31 19 27 81 243 88 100 6 10 5 Sum: 610 =>> End of the Program Documentation / Comments 31 100 6 10 5arrow_forward2DArray method:It will allocate a block of memory which represents a two-dimension arraybased on the input values supplied by the user. Ask the user to enter the number of rows andcolumn for 2D array that the user want to manipulate. The number of rows and columns that theuser enters may or may not define a square matrix (when the number of rows equals the numberof columns). The array will have exactly rows time columns (m * n) elements. It will notcontain any extra or empty cells. Initialize the “matrix” by rows with random number between 1to 100. Pass two arguments by out reference so that you can assign the number of row andcolumns of data to the first and second arguments. This is return 2D array method afterallocating new memory for 2D array and initialize it with random value. Generate randomnumber code in C# as following: Random randnum = new Random( );int number = randnum.Next (1, 101);SwapRow method: it will rotate the data in the matrix in an up/down method such that the…arrow_forwardcan you please write this in java.util.scanner form and can you make it so i can copy and past it, thank you.can you please write this in java.util.scanner form and can you make it so i can copy and past it, thank you. 2D Array OperationsWrite a program that creates a two-dimensional array initialized with test data. Use anyprimitive data type that you wish. The program should have the following methods:• getTotal. This method should accept a two-dimensional array as its argument andreturn the total of all the values in the array.• getAverage. This method should accept a two-dimensional array as its argument andreturn the average of all the values in the array.• getRowTotal. This method should accept a two-dimensional array as its first argumentand an integer as its second argument. The second argument should be the subscriptof a row in the array. The method should return the total of the values in thespecified row.• getColumnTotal. This method should accept a two-dimensional array…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
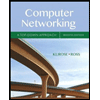
Computer Networking: A Top-Down Approach (7th Edi...
Computer Engineering
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:PEARSON
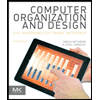
Computer Organization and Design MIPS Edition, Fi...
Computer Engineering
ISBN:9780124077263
Author:David A. Patterson, John L. Hennessy
Publisher:Elsevier Science
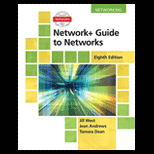
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:9781337569330
Author:Jill West, Tamara Dean, Jean Andrews
Publisher:Cengage Learning
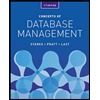
Concepts of Database Management
Computer Engineering
ISBN:9781337093422
Author:Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:Cengage Learning
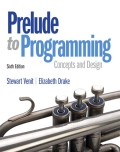
Prelude to Programming
Computer Engineering
ISBN:9780133750423
Author:VENIT, Stewart
Publisher:Pearson Education
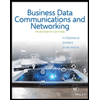
Sc Business Data Communications and Networking, T...
Computer Engineering
ISBN:9781119368830
Author:FITZGERALD
Publisher:WILEY