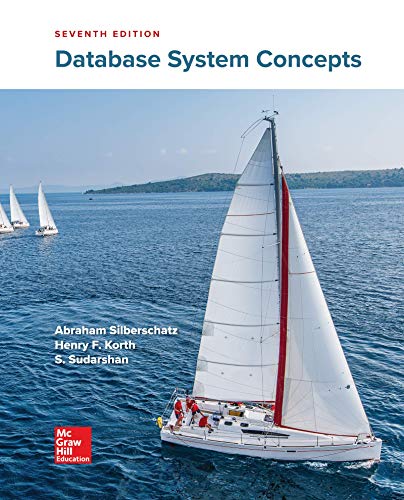
Explain the functionality of each line of code in the provided C# scripts-
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Movement2D : MonoBehaviour
{
public float moveSpeed = 3f;
public float jumpForce = 5f;
public bool isGrounded;
private bool doubleJump;
private bool canDash = true;
private bool isDashing;
private float dashingPower = 24f;
private float dashingTime = .2f;
private float dashingCooldown = 1f;
private bool isWallJumping;
private float wallJumpingDirection;
private float wallJumpingTime;
private float wallJumpingCounter;
private float wallJumpingDuration = .04f;
private Vector2 wallJumpingPower = new Vector2(8f, 16f);
[SerializeField] private Rigidbody2D rb;
[SerializeField] private TrailRenderer tr;
[SerializeField] private Transform wallCheck;
[SerializeField] private LayerMask wallLayer;
private SpriteRenderer sprite;
public void Start()
{
sprite = gameObject.GetComponent<SpriteRenderer>();
}
private void Update()
{
if (isDashing)
{
return;
}
WallJump();
Jump();
Vector3 movement = new Vector3(Input.GetAxis("Horizontal"), 0f, 0f);
transform.position += movement * Time.deltaTime * moveSpeed;
if (Input.GetAxisRaw("Horizontal") < 0)
{
sprite.flipX = true;
}
else if (Input.GetAxisRaw("Horizontal") > 0)
{
sprite.flipX = false;
}
if (Input.GetKeyDown(KeyCode.LeftShift) && canDash)
{
StartCoroutine(Dash());
}
if (!isWallJumping)
{
rb.velocity = new Vector2(Input.GetAxisRaw("Horizontal") * moveSpeed, rb.velocity.y);
}
}
void Jump()
{
if (Input.GetButtonDown("Jump") && isGrounded)
{
gameObject.GetComponent<Rigidbody2D>().velocity = (new Vector2(0f, jumpForce));
doubleJump = true;
}
else if (Input.GetButtonDown("Jump") && doubleJump)
{
gameObject.GetComponent<Rigidbody2D>().velocity = (new Vector2(0f, jumpForce));
doubleJump = false;
}
}
private IEnumerator Dash()
{
canDash = false;
isDashing = true;
float orignialGravity = rb.gravityScale;
rb.gravityScale = 0f;
rb.velocity = new Vector3(Input.GetAxis("Horizontal") * dashingPower, 0f);
tr.emitting = true;
yield return new WaitForSeconds(dashingTime);
tr.emitting = false;
rb.gravityScale = orignialGravity;
isDashing = false;
yield return new WaitForSeconds(dashingCooldown);
canDash = true;
}
private void WallJump()
{
if (Input.GetButtonDown("Jump") && wallJumpingCounter > 0f)
{
isWallJumping = true;
rb.velocity = new Vector2(wallJumpingDirection * wallJumpingPower.x, wallJumpingPower.y);
wallJumpingCounter = 0f;
if (Input.GetAxisRaw("Horizontal") != wallJumpingDirection)
{
sprite.flipX = !sprite.flipX;
}
Invoke(nameof(StopWallJumping), wallJumpingDuration);
}
}
private void StopWallJumping()
{
isWallJumping = false;
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.Video;
[RequireComponent(typeof(VideoPlayer))]
[RequireComponent(typeof(AudioSource))]
public class PlayVideo : MonoBehaviour
{
public VideoClip videoClip;
private VideoPlayer videoPlayer;
private AudioSource audioSource;
void Start()
{
videoPlayer = GetComponent<VideoPlayer>();
audioSource = GetComponent<AudioSource>();
videoPlayer.playOnAwake = false;
audioSource.playOnAwake = false;
videoPlayer.source = VideoSource.VideoClip;
videoPlayer.clip = videoClip;
videoPlayer.audioOutputMode = VideoAudioOutputMode.AudioSource;
videoPlayer.SetTargetAudioSource(0, audioSource);
videoPlayer.renderMode = VideoRenderMode.MaterialOverride;
videoPlayer.targetMaterialRenderer = GetComponent<Renderer>();
videoPlayer.targetMaterialProperty = "_MainTex";
}
void Update()
{
if (Input.GetButtonDown("Jump"))
PlayPause();
}
private void PlayPause()
{
if (videoPlayer.isPlaying)
videoPlayer.Pause();
else
videoPlayer.Play();
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement;
public class NewBehaviourScript : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
}
// Update is called once per frame
void Update()
{
}
public void LoadOnClick (int sceneIndex)
{
SceneManager.LoadScene(sceneIndex);
}
}

The first script can be defined in such a way that it controls 2D character motion, such as leaping, dashing, and wall jumping, even as dealing with consumer input and managing movement parameters.
The second script can be defined in such a way that it lets in for video playback manipulation in unity, such as playing and pausing a certain video clip using the VideoPlayer component.
The third script can be defined in such a way that it offers a simple functionality to load a brand new scene in unity based on a specified scene index.
Step by stepSolved in 5 steps

- In C++ Create a new project named lab9_1 . You will need to implement a Course class. Here is its UML diagram: Course - department : string- course_num : string- section : int- num_students : int- is_full : bool + Course()+ Course(string, string, int, int)+ setDepartment(string) : void+ setNumber(string) : void+ setSection(int) : void+ setStudents(int) : void+ getDepartment() const : string+ getNumber() const : string+ getSection() const : int+ getStudents() const : int+ print() const : void Create a sample file to read from: CSS 2A 1111 35 Additional information: The Course class has two constructors. Make sure you have default values for your default constructor. Each course maxes out at 40 students. Therefore, you need to make sure that there aren’t more than 40 students in a Course. You can choose how you handle situations where more than 40 students are added. Additionally, you should automatically set is_full to false or true, based on the number of…arrow_forwardfinal big report project for java. 1.Problem Description Student information management system is used to input, display student information records. The GUI interface for inputing ia designed as follows : The top part are the student imformation used to input student information,and the bottom part are four buttonns, each button meaning : (2) Total : add java score and C++ score ,and display the result; (3) Save: save student record into file named student.dat; (4) Clear : set all fields on GUI to empty string; (5) Close : close the winarrow_forwardIn C++ Create a new project named lab8_1. You will be implementing two classes: A Book class, and a Bookshelf class. The Bookshelf has a Book object (actually 3 of them). You will be able to choose what Book to place in each Bookshelf. Here are their UML diagrams Book class UML Book - author : string- title : string- id : int- count : static int + Book()+ Book(string, string)+ setAuthor(string) : void+ setTitle(string) : void+ print() : void+ setID() : void And the Bookshelf class UML Bookshelf - book1 : Book- book2 : Book- book3 : Book + Bookshelf()+ Bookshelf(Book, Book, Book)+ setBook1(Book) : void+ setBook2(Book) : void+ setBook3(Book) : void+ print() : void Some additional information: The Book class also has two constructors, which again offer the option of declaring a Book object with an author and title, or using the default constructor to set the author and title later, via the setters . The Book class will have a static member variable…arrow_forward
- Help me Complete the class definition and implementation for class task.h and task.cpp: Example: class Task { private: string name; DateTime startDate; DateTime endDate; int status; // value =1 means DONE! and value = 0 is pending }arrow_forwardPlease answer in C++ and give explanation LAB 17.1-B Linked List Read the internal documentation for class ItemList carefully. Fill in the missing code in the implementation file, being careful to adhere to the preconditions and postconditions. Compile your program. ------------------------------------------------------------------------------------------------------------------------------- class ItemList { private: struct ListNode { int value; ListNode * next; }; ListNode * head; public: ItemList(); // Post: List is the empty list. bool IsThere(int item) const; // Post: If item is in the list IsThere is // True; False, otherwise. void Insert(int item); // Pre: item is not already in the list. // Post: item is in the list. void Delete(int item); // Pre: item is in the list. // Post: item is no…arrow_forwardDefine and use an instance methodarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
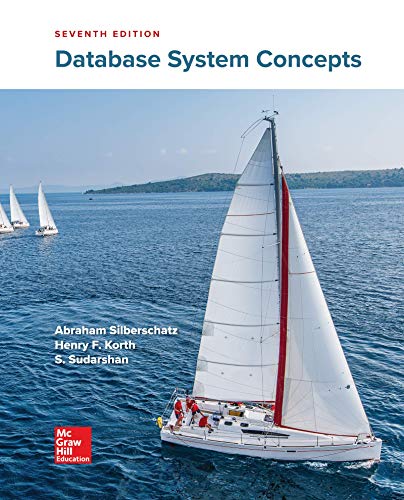
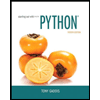
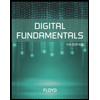
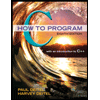
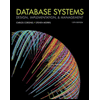
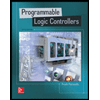