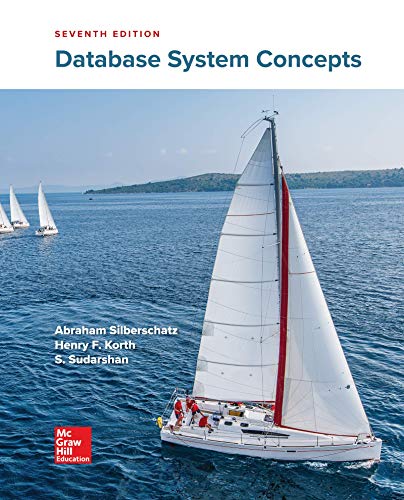
You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment.
In this problem, you must implement a tokenizer for the Assembly instruction format: an operation type (e.g. SUBI) followed by a comma-separated list of parameters (e.g. R0, R1, 6). If a line has an invalid syntax, it should be skipped in the output. You do not need to check whether the operations and arguments are valid Assembly instructions: you just need to separate them into tokens. Input Format The input to the program will consist of some number of lines. Each line is of the following form: "op arg1, arg2, ..., argn". Your program should terminate upon receiving a blank line or EOF. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by the included test cases, all of which are public. This is not a performance-centric problem. Output Format The tokenized output from each input line should be given as a series of space-separated tokens, without commas or other symbols. Invalid lines should be skipped. Input 0 ADD R0, R6, R7 SUB R3, R4, R5 Output 0 ADD R0 R6 R7 SUB R3 R4 R5 Input 1 ADDI R8, R9, 6 JMP R3 Output 1 ADDI R8 R9 6 JMP R3 Input 2 TEST a TEST a, b, c, d, e, f, g Output 2 TEST a TEST a b c d e f g Input 3 this file, tests TEST a, b c erroneous cases TEST, a, b there should, only, be TEST, a b six lines, in, the, output TEST a b and it, should TEST a , b, c include this, one TEST a, b,, c Output 3 this file tests erroneous cases there should only be six lines in the output and it should include this one All of the lines beginning with TEST have some sort of syntax error, so they should be omitted from the output. The other lines have no errors, so they should be tokenized and output. The errors are as follows: TEST a, b c: the comma between b and c is missing TEST, a, b: there is an extra comma after TEST TEST, a b: the comma between a and b is missing, and there is an extra comma after TEST TEST a b: the comma between a and b is missing TEST a , b, c: there is a space before the comma between a and b TEST a, b,, c: there is an extra comma between b and c

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 2 images

I have something similar but I having difficulty for it passing the final test case
Sample Input 3
Sample Output 3
Explanation 3
All of the lines beginning with TEST have some sort of syntax error, so they should be omitted from the output. The other lines have no errors, so they should be tokenized and output. The errors are as follows:
- TEST a, b c: the comma between b and c is missing
- TEST, a, b: there is an extra comma after TEST
- TEST, a b: the comma between a and b is missing, and there is an extra comma after TEST
- TEST a b: the comma between a and b is missing
- TEST a , b, c: there is a space before the comma between a and b
- TEST a, b,, c: there is an extra comma between b and c
My current output as of right now
this file tests
TEST a b c
erroneous cases
TEST a b
there should only be
TEST a b
six lines in the output
TEST a b
and it should
TEST a b c
include this one
TEST a b c
I have something similar but I having difficulty for it passing the final test case
Sample Input 3
Sample Output 3
Explanation 3
All of the lines beginning with TEST have some sort of syntax error, so they should be omitted from the output. The other lines have no errors, so they should be tokenized and output. The errors are as follows:
- TEST a, b c: the comma between b and c is missing
- TEST, a, b: there is an extra comma after TEST
- TEST, a b: the comma between a and b is missing, and there is an extra comma after TEST
- TEST a b: the comma between a and b is missing
- TEST a , b, c: there is a space before the comma between a and b
- TEST a, b,, c: there is an extra comma between b and c
My current output as of right now
this file tests
TEST a b c
erroneous cases
TEST a b
there should only be
TEST a b
six lines in the output
TEST a b
and it should
TEST a b c
include this one
TEST a b c
- Write a code in sim8085 for the following problem: The pressure of two boilers is monitored and controlled by a microcomputer works based on microprocessor programming. A set of 6 readings of first boiler, recorded by six pressure sensors, which are stored in the memory location starting from 2050H. A corresponding set of 6 reading from the second boiler is stored at the memory location starting from 2060H. Each reading from the first set is expected to be higher than the corresponding position in the second set of readings. Write an 8085 sequence to check whether the first set of reading is higher than the second one or not. If all the readings of first set is higher than the second set, store 00 in the ‘D’ register. If any one of the readings is lower than the corresponding reading of second set, stop the process and store FF in the register ‘D’. Data (H): First set: 78, 89, 6A, 80, 90, 85 Second Set:71, 78, 65, 89, 56, 75arrow_forwardYou are required to write a C program on Unix/Linux in which the parent process creates three child processes, lets them run concurrently, and waits for them to return and prints their exit status. The three child processes are assigned different tasks. Child one is to calculate and display the average mark of a class of twenty students for a unit. Child one is required to get the marks from the standard input (i.e. the keyboard). Child two is to load a program called “wc” (word count) to count file1. Child two is required to get file1 by the command line argument. Child three is to modify file2 by first inserting “this is the updated version.” at the beginning of this file and then replacing all the occurrences of the word “run” by “execute” and “examine” by “study”. You are required to write your OWN program for the file update. Child three is required to get file2 by the command line argument.arrow_forwardYou are expected to use python Object-Oriented programming concepts to design and implement a system in python for building a scientific calculator which inherits its basic operations from a standard calculator. The standard calculator can perform two types of operations: an addition and a subtraction The standard calculator is capable of inputting only two integers. But the scientific calculators can also interpret decimal values. The scientific calculator is capable of four operations: addition, subtraction, cosine and sine of values. The scientific calculator can accept multiple values for the addition operation. After performing an addition, the scientific calculator will send the final result to a database. (You are not expected to write the codes for the database connection and the queries. You are expected to only show how you can use your scientific calculator object to pass messages to a database object) Create 100 objects for your scientific calculator to enable…arrow_forward
- Example: The Problem Input File Using C programming language write a program that simulates a variant of the Tiny Machine Architecture. In this implementation memory (RAM) is split into Instruction Memory (IM) and Data Memory (DM). Your code must implement the basic instruction set architecture (ISA) of the Tiny Machine Architecture: //IN 5 //OUT 7 //STORE O //IN 5 //OUT 7 //STORE 1 //LOAD O //SUB 1 55 67 30 55 67 1 LOAD 2- ADD 3> STORE 4> SUB 5> IN 6> OUT 7> END 8> JMP 9> SKIPZ 31 10 41 30 //STORE O 67 //OUT 7 11 /LOAD 1 //OUT 7 //END 67 70 Output Specifications Each piece of the architecture must be accurately represented in your code (Instruction Register, Program Counter, Memory Address Registers, Instruction Memory, Data Memory, Memory Data Registers, and Accumulator). Data Memory will be represented by an integer array. Your Program Counter will begin pointing to the first instruction of the program. Your simulator should provide output according to the input file. Along with…arrow_forwardCreate a code that stores values on the stack in each iteration of the factorial label. How many values are you storing on the stack in each iteration of your factorial label? By how many bytes do you expect the stack pointer to change? using coding composer studio with a MSP432 microcontroller. In assembly language not C or C++.arrow_forwardFor this C Program, I am getting the error "Expected an identifier" on line 18.arrow_forward
- Answser must be in MIPSzy assembly language. Max of 3 - Brancharrow_forwardFor the following problem, please do not provide me any code. I will give you my code please check it and make corrections as necessary. Write a c program that will generate the safe sequence of process execution for the situationgiven below:(Use Banker’s Algorithm).Note: The code can be implemented in several different ways, but make sure the parameterremains the same as shown below.n = 6; // Number of processesm = 4; // Number of resourcesint alloc[6][4] = { { 0, 1, 0, 3 }, // P0// Allocation Matrix{ 2, 0, 0, 3 }, // P1{ 3, 0, 2, 0 }, // P2{ 2, 1, 1, 5 }, // P3{ 0, 0, 2, 2 }, // P4{1, 2 , 3, 1 } }; //P5int max[6][4] = { { 6, 4, 3, 4 }, // P0 // MAX Matrix{ 3, 2, 2, 4 }, // P1{ 9, 1, 2, 6 }, // P2{ 2, 2, 2, 8 }, // P3{ 4, 3, 3, 7 }, // P4{ 6, 2 , 6, 5 } }; //P5int avail[4] = { 2, 2, 2, 1 }; //Available resources My Code for the above problem: #include <stdio.h> int main() { int n, m; n = 6; // Number of processes m = 4; // Number of resources //…arrow_forwardIn c++ write VM translator in which it will read a program written in HACK vm from an external file and ultimatley translate each line of code into Hack asm (assembly) , so, Higher level Hack Virtual machine language to Hack level assembly language. For example make it possible to translate the following: One Arithmetic (SUB), one logic (AND), and then Memory access command POP.arrow_forward
- You must complete this in Python and the programs should not take any command-line arguments. You also need to make sure your programs will compile and run in at least a Linux environment. In this problem, you need to implement operation bit encoding for Assembly instructions. Given a line of text, your program should check whether it is a valid Assembly instruction type. If it is, your program should print out the opcode and optype of that instruction type; if the line of text is not exactly a valid Assembly instruction, your program should skip it and move to the next line of input without printing anything. Input Format The input to the program will consist of some number of lines. Each line contains some text, either a valid Assembly instruction type (with no extra whitespace or other characters, e.g. READ on a line by itself) or some other text. Constraints There are no specific constraints on the length or number of lines. They will be in a reasonable limit, as demonstrated by…arrow_forward3. Write a C program multiplication Table.c that outputs the multiplication table as follows: X Multiplication Table 1 2 7 8 9 10 2 7 8 9 10 4 14 16 18 20 6 9 12 15 18 21 24 27 30 8 12 16 20 24 28 32 36 40 35 40 45 50 18 24 30 36 42 48 54 60 42 49 35 42 49 56 63 70 64 72 80 72 81 90 70 80 90 100 3 4 5 6 3 4 5 6 1 1 2 3 3 4 4 5 5 10 15 20 25 30 6 6 12 7 7 14 21 28 35 16 24 32 40 48 8 8 99 18 10 10 20 30 40 50 60 56 27 36 45 54 63arrow_forwardI need a C program where the input is postorder. For example, if the input is postorder: x1 5.12 + x2 7.68 - * x3 / then the resulting output would be an inorder: (((x1+5.12)*(x2-7.68))/x3)arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
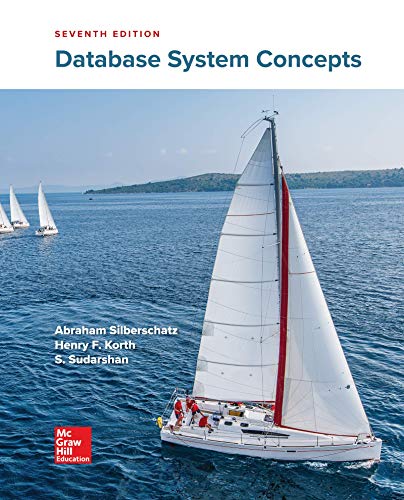
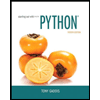
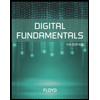
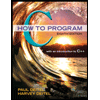
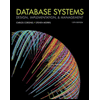
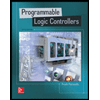