Your code should work identically to the cases below. Please run each of these to check your code, in the order given. (You can try more later). HW 2: Airlines USE THESE WORDS. DO NOT BE CREATIVE. Creativity is saved for your programming. Finish the following c++ code by following the instructions Example execution: Portland, weekend, Nightflight > . /Air.out Pricing information: For Miami travel, flight prices are as follows: For weekday travel: $150 during the daytime, $100 during the nighttime For weekend travel: $180 during the daytime, $120 during the nighttime Chicago flights cost half the price of Miami flights, and Portland flights cost twice as much as Miami flights. For example, a Portland weekday flight during the nighttime is $200. A Chicago weekend flight during the daytime is $90. Welcome to Airlines! What is your destination? ([C]hicago, [M]iami, [P]ortland) P What time will you travel? (Enter time between 0-2359) 450 What type of day are you traveling? (week [E]nd or week [D]ay) E Each ticket will cost: $240.00 How many tickets do you want? 2 You owe: $480.00 Amount paid? 100.00 This is the cost per ticket for each type of travel: That is too little! No tickets ordered. WeekendNight WeekdayDay WeekdayNight WeekendDay 180 Miami 150 100 120 Another example execution Miami, Weekday, Dayflight > ./Air. out Chicago Portland 60 75 50 90 Welcome to Airlines! 360 240 300 200 What is your destination? ([C]hicago, [M]iami, [P]ortland) M What time will you travel? (Enter time between 0-2359) 1345 What type of day are you traveling? (week [E]nd or week [D]ay) D Each ticket will cost: $150.00 Your program is to work as follows: 1. Display a welcome message (e.g., "Welcome to Airlines!") 2. Prompt the user to input his/her destination: Chicago, Miami, or Portland (I recommend you use letters to represent each input) 3. Prompt the user to input what time s/he wishes to travel (in army time, e.g., 800 for 8am or 1530 for 3:30pm) 4. Prompt the user to input what type of day (s) s/he is traveling: Weekday or Weekend (I recommend you use letters to represent each input) 5. Report the price per ticket of the specified type 6. Prompt the user for the number of tickets to be purchased If the number of tickets is fewer than 0, report that the number of tickets ordered is invalid and the order has been cancelled, then exit; otherwise: 7. Compute and display the total amount due (no sales tax this time!) How many tickets do you want? 3 You owe: $450.00 Amount paid? 500.00 You will get in change: $50.00 Your tickets have been ordered! Another example execution Chicago, Weekday, NightFlight > ./Air.out Welcome to Airlines! What is your destination? ([C]hicago, [M]iami, [P]ortland) C What time will you travel? (Enter time between 0-2359) 2230 What type of day are you traveling? (week [E]nd or week [D]ay) D Each ticket will cost: $50.00 How many tickets do you want? 4 You owe: $200.00 8. Prompt the user to enter the amount s/he is paying If the amount paid is less than the amount due, report that the amount paid is too little and the order has been cancelled, then exit; otherwise: 9. Display change and confirm the order has been placed Amount paid? 300.00 You will get $100.00 in change. Your tickets have been ordered! // Add to this partially built code // fill in code where there is a TODO #include using namespace std; change (a float) // TODO: calculate the int main() cout.setf(ios:fixed): cout.setf(ios:showpoint); // show decimals even if not needed cout.precision(2); // two places to the right of the decimal // TODO: enter the missing types below int time; float ticketPrice; destination; //'c'=Chicago, 'P'=Portland, 'M'=Miami typeOfDay; //'D'=weekDay 'E'=weekEnd // TODO: If the user's payment is too little, then print this message: cout << "Welcome to Airlines!" << endl; cout <« "What is your destination? ([C]hicago, [M]iami, [P]ortland) "; cin >> destination; cout << "What time will you travel? (Enter time between 0-2359) "; cin >> time; cout << "That is too little! No tickets ordered."; // TODO: set isDayTime to true if time 5AM or later, but before 7PM cout << "What type of day are you traveling? (week[E]nd or week[D]ay) "; cin >> typeOfDay; // TODO: set isWeekend to true if typeOfDay is 'E', otherwise false // otherwise print these 2 lines: // Depending upon the destination, and whether it is weekend, day/night // set the appropriate price //I recommend using a switch // I am providing much of the Input and Output dialog to simplify this program cout << "Each ticket will cost: Ş" << ticketPrice << endl; cout << "You will get in change: $" << change << endl; int numTickets; cout << "How many tickets do you want? "; cout << "Your tickets have been ordered!"; cin >> num Tickets; // TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it cout << "You owe: $" << totalCost << endl; return 0; // TODO: declare a variable that will hold the user's payment // prompt the user for "Amount paid?" // read in the user's Payment
Your code should work identically to the cases below. Please run each of these to check your code, in the order given. (You can try more later). HW 2: Airlines USE THESE WORDS. DO NOT BE CREATIVE. Creativity is saved for your programming. Finish the following c++ code by following the instructions Example execution: Portland, weekend, Nightflight > . /Air.out Pricing information: For Miami travel, flight prices are as follows: For weekday travel: $150 during the daytime, $100 during the nighttime For weekend travel: $180 during the daytime, $120 during the nighttime Chicago flights cost half the price of Miami flights, and Portland flights cost twice as much as Miami flights. For example, a Portland weekday flight during the nighttime is $200. A Chicago weekend flight during the daytime is $90. Welcome to Airlines! What is your destination? ([C]hicago, [M]iami, [P]ortland) P What time will you travel? (Enter time between 0-2359) 450 What type of day are you traveling? (week [E]nd or week [D]ay) E Each ticket will cost: $240.00 How many tickets do you want? 2 You owe: $480.00 Amount paid? 100.00 This is the cost per ticket for each type of travel: That is too little! No tickets ordered. WeekendNight WeekdayDay WeekdayNight WeekendDay 180 Miami 150 100 120 Another example execution Miami, Weekday, Dayflight > ./Air. out Chicago Portland 60 75 50 90 Welcome to Airlines! 360 240 300 200 What is your destination? ([C]hicago, [M]iami, [P]ortland) M What time will you travel? (Enter time between 0-2359) 1345 What type of day are you traveling? (week [E]nd or week [D]ay) D Each ticket will cost: $150.00 Your program is to work as follows: 1. Display a welcome message (e.g., "Welcome to Airlines!") 2. Prompt the user to input his/her destination: Chicago, Miami, or Portland (I recommend you use letters to represent each input) 3. Prompt the user to input what time s/he wishes to travel (in army time, e.g., 800 for 8am or 1530 for 3:30pm) 4. Prompt the user to input what type of day (s) s/he is traveling: Weekday or Weekend (I recommend you use letters to represent each input) 5. Report the price per ticket of the specified type 6. Prompt the user for the number of tickets to be purchased If the number of tickets is fewer than 0, report that the number of tickets ordered is invalid and the order has been cancelled, then exit; otherwise: 7. Compute and display the total amount due (no sales tax this time!) How many tickets do you want? 3 You owe: $450.00 Amount paid? 500.00 You will get in change: $50.00 Your tickets have been ordered! Another example execution Chicago, Weekday, NightFlight > ./Air.out Welcome to Airlines! What is your destination? ([C]hicago, [M]iami, [P]ortland) C What time will you travel? (Enter time between 0-2359) 2230 What type of day are you traveling? (week [E]nd or week [D]ay) D Each ticket will cost: $50.00 How many tickets do you want? 4 You owe: $200.00 8. Prompt the user to enter the amount s/he is paying If the amount paid is less than the amount due, report that the amount paid is too little and the order has been cancelled, then exit; otherwise: 9. Display change and confirm the order has been placed Amount paid? 300.00 You will get $100.00 in change. Your tickets have been ordered! // Add to this partially built code // fill in code where there is a TODO #include using namespace std; change (a float) // TODO: calculate the int main() cout.setf(ios:fixed): cout.setf(ios:showpoint); // show decimals even if not needed cout.precision(2); // two places to the right of the decimal // TODO: enter the missing types below int time; float ticketPrice; destination; //'c'=Chicago, 'P'=Portland, 'M'=Miami typeOfDay; //'D'=weekDay 'E'=weekEnd // TODO: If the user's payment is too little, then print this message: cout << "Welcome to Airlines!" << endl; cout <« "What is your destination? ([C]hicago, [M]iami, [P]ortland) "; cin >> destination; cout << "What time will you travel? (Enter time between 0-2359) "; cin >> time; cout << "That is too little! No tickets ordered."; // TODO: set isDayTime to true if time 5AM or later, but before 7PM cout << "What type of day are you traveling? (week[E]nd or week[D]ay) "; cin >> typeOfDay; // TODO: set isWeekend to true if typeOfDay is 'E', otherwise false // otherwise print these 2 lines: // Depending upon the destination, and whether it is weekend, day/night // set the appropriate price //I recommend using a switch // I am providing much of the Input and Output dialog to simplify this program cout << "Each ticket will cost: Ş" << ticketPrice << endl; cout << "You will get in change: $" << change << endl; int numTickets; cout << "How many tickets do you want? "; cout << "Your tickets have been ordered!"; cin >> num Tickets; // TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it cout << "You owe: $" << totalCost << endl; return 0; // TODO: declare a variable that will hold the user's payment // prompt the user for "Amount paid?" // read in the user's Payment
Chapter2: Using Data
Section: Chapter Questions
Problem 4E: In this chapter, you learned that although a double and a decimal both hold floating-point numbers,...
Related questions
Question
![Your code should work identically to the cases below. Please run each of these to check your code, in the
order given. (You can try more later).
HW 2: Airlines
USE THESE WORDS. DO NOT BE CREATIVE. Creativity is saved for your programming.
Finish the following c++ code by following the instructions
Example execution: Portland, weekend, Nightflight
> . /Air.out
Pricing information:
For Miami travel, flight prices are as follows:
For weekday travel: $150 during the daytime, $100 during the nighttime
For weekend travel: $180 during the daytime, $120 during the nighttime
Chicago flights cost half the price of Miami flights, and Portland flights cost twice as much as Miami flights. For
example, a Portland weekday flight during the nighttime is $200. A Chicago weekend flight during the daytime
is $90.
Welcome to Airlines!
What is your destination? ([C]hicago, [M]iami, [P]ortland) P
What time will you travel? (Enter time between 0-2359) 450
What type of day are you traveling? (week [E]nd or week [D]ay) E
Each ticket will cost: $240.00
How many tickets do you want? 2
You owe: $480.00
Amount paid? 100.00
This is the cost per ticket for each type of travel:
That is too little! No tickets ordered.
WeekendNight
WeekdayDay
WeekdayNight
WeekendDay
180
Miami
150
100
120
Another example execution Miami, Weekday, Dayflight
> ./Air. out
Chicago
Portland
60
75
50
90
Welcome to Airlines!
360
240
300
200
What is your destination? ([C]hicago, [M]iami, [P]ortland) M
What time will you travel? (Enter time between 0-2359) 1345
What type of day are you traveling? (week [E]nd or week [D]ay) D
Each ticket will cost: $150.00
Your program is to work as follows:
1. Display a welcome message (e.g., "Welcome to Airlines!")
2. Prompt the user to input his/her destination: Chicago, Miami, or Portland (I recommend you use letters to
represent each input)
3. Prompt the user to input what time s/he wishes to travel (in army time, e.g., 800 for 8am or 1530 for
3:30pm)
4. Prompt the user to input what type of day (s) s/he is traveling: Weekday or Weekend (I recommend you use
letters to represent each input)
5. Report the price per ticket of the specified type
6. Prompt the user for the number of tickets to be purchased
If the number of tickets is fewer than 0, report that the number of tickets ordered is invalid and the order has
been cancelled, then exit; otherwise:
7. Compute and display the total amount due (no sales tax this time!)
How many tickets do you want? 3
You owe: $450.00
Amount paid? 500.00
You will get in change: $50.00
Your tickets have been ordered!
Another example execution Chicago, Weekday, NightFlight
> ./Air.out
Welcome to Airlines!
What is your destination? ([C]hicago, [M]iami, [P]ortland) C
What time will you travel? (Enter time between 0-2359) 2230
What type of day are you traveling? (week [E]nd or week [D]ay) D
Each ticket will cost: $50.00
How many tickets do you want? 4
You owe: $200.00
8. Prompt the user to enter the amount s/he is paying
If the amount paid is less than the amount due, report that the amount paid is too little and the order has
been cancelled, then exit; otherwise:
9. Display change and confirm the order has been placed
Amount paid? 300.00
You will get $100.00 in change.
Your tickets have been ordered!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6900813d-7c23-46b7-a2db-4b3d463f1913%2F89638a5a-78cd-4381-9bd8-ab887c8f423b%2Fsewti9.jpeg&w=3840&q=75)
Transcribed Image Text:Your code should work identically to the cases below. Please run each of these to check your code, in the
order given. (You can try more later).
HW 2: Airlines
USE THESE WORDS. DO NOT BE CREATIVE. Creativity is saved for your programming.
Finish the following c++ code by following the instructions
Example execution: Portland, weekend, Nightflight
> . /Air.out
Pricing information:
For Miami travel, flight prices are as follows:
For weekday travel: $150 during the daytime, $100 during the nighttime
For weekend travel: $180 during the daytime, $120 during the nighttime
Chicago flights cost half the price of Miami flights, and Portland flights cost twice as much as Miami flights. For
example, a Portland weekday flight during the nighttime is $200. A Chicago weekend flight during the daytime
is $90.
Welcome to Airlines!
What is your destination? ([C]hicago, [M]iami, [P]ortland) P
What time will you travel? (Enter time between 0-2359) 450
What type of day are you traveling? (week [E]nd or week [D]ay) E
Each ticket will cost: $240.00
How many tickets do you want? 2
You owe: $480.00
Amount paid? 100.00
This is the cost per ticket for each type of travel:
That is too little! No tickets ordered.
WeekendNight
WeekdayDay
WeekdayNight
WeekendDay
180
Miami
150
100
120
Another example execution Miami, Weekday, Dayflight
> ./Air. out
Chicago
Portland
60
75
50
90
Welcome to Airlines!
360
240
300
200
What is your destination? ([C]hicago, [M]iami, [P]ortland) M
What time will you travel? (Enter time between 0-2359) 1345
What type of day are you traveling? (week [E]nd or week [D]ay) D
Each ticket will cost: $150.00
Your program is to work as follows:
1. Display a welcome message (e.g., "Welcome to Airlines!")
2. Prompt the user to input his/her destination: Chicago, Miami, or Portland (I recommend you use letters to
represent each input)
3. Prompt the user to input what time s/he wishes to travel (in army time, e.g., 800 for 8am or 1530 for
3:30pm)
4. Prompt the user to input what type of day (s) s/he is traveling: Weekday or Weekend (I recommend you use
letters to represent each input)
5. Report the price per ticket of the specified type
6. Prompt the user for the number of tickets to be purchased
If the number of tickets is fewer than 0, report that the number of tickets ordered is invalid and the order has
been cancelled, then exit; otherwise:
7. Compute and display the total amount due (no sales tax this time!)
How many tickets do you want? 3
You owe: $450.00
Amount paid? 500.00
You will get in change: $50.00
Your tickets have been ordered!
Another example execution Chicago, Weekday, NightFlight
> ./Air.out
Welcome to Airlines!
What is your destination? ([C]hicago, [M]iami, [P]ortland) C
What time will you travel? (Enter time between 0-2359) 2230
What type of day are you traveling? (week [E]nd or week [D]ay) D
Each ticket will cost: $50.00
How many tickets do you want? 4
You owe: $200.00
8. Prompt the user to enter the amount s/he is paying
If the amount paid is less than the amount due, report that the amount paid is too little and the order has
been cancelled, then exit; otherwise:
9. Display change and confirm the order has been placed
Amount paid? 300.00
You will get $100.00 in change.
Your tickets have been ordered!
![// Add to this partially built code
// fill in code where there is a TODO
#include<iostream>
using namespace std;
change (a float)
// TODO: calculate the
int main()
cout.setf(ios:fixed):
cout.setf(ios:showpoint); // show decimals even if not needed
cout.precision(2); // two places to the right of the decimal
// TODO: enter the missing types below
int time;
float ticketPrice;
destination; //'c'=Chicago, 'P'=Portland, 'M'=Miami
typeOfDay; //'D'=weekDay 'E'=weekEnd
// TODO: If the user's payment is too little, then print this message:
cout << "Welcome to Airlines!"
<< endl;
cout <« "What is your destination? ([C]hicago, [M]iami, [P]ortland) ";
cin >> destination;
cout << "What time will you travel? (Enter time between 0-2359) ";
cin >> time;
cout << "That is too little! No tickets ordered.";
// TODO: set isDayTime to true if time 5AM or later, but before
7PM
cout << "What type of day are you traveling? (week[E]nd or week[D]ay) ";
cin >> typeOfDay;
// TODO: set isWeekend to true if typeOfDay is 'E', otherwise false
// otherwise print these 2 lines:
// Depending upon the destination, and whether it is weekend, day/night
// set the appropriate price
//I recommend using a switch
// I am providing much of the Input and Output dialog to simplify this program
cout << "Each ticket will cost: Ş" << ticketPrice << endl;
cout << "You will get in change: $" << change << endl;
int numTickets;
cout << "How many tickets do you want? ";
cout << "Your tickets have been ordered!";
cin >> num Tickets;
// TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it
cout << "You owe: $" << totalCost << endl;
return 0;
// TODO: declare a variable that will hold the user's payment
// prompt the user for "Amount paid?"
// read in the user's Payment](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6900813d-7c23-46b7-a2db-4b3d463f1913%2F89638a5a-78cd-4381-9bd8-ab887c8f423b%2F85hlane.jpeg&w=3840&q=75)
Transcribed Image Text:// Add to this partially built code
// fill in code where there is a TODO
#include<iostream>
using namespace std;
change (a float)
// TODO: calculate the
int main()
cout.setf(ios:fixed):
cout.setf(ios:showpoint); // show decimals even if not needed
cout.precision(2); // two places to the right of the decimal
// TODO: enter the missing types below
int time;
float ticketPrice;
destination; //'c'=Chicago, 'P'=Portland, 'M'=Miami
typeOfDay; //'D'=weekDay 'E'=weekEnd
// TODO: If the user's payment is too little, then print this message:
cout << "Welcome to Airlines!"
<< endl;
cout <« "What is your destination? ([C]hicago, [M]iami, [P]ortland) ";
cin >> destination;
cout << "What time will you travel? (Enter time between 0-2359) ";
cin >> time;
cout << "That is too little! No tickets ordered.";
// TODO: set isDayTime to true if time 5AM or later, but before
7PM
cout << "What type of day are you traveling? (week[E]nd or week[D]ay) ";
cin >> typeOfDay;
// TODO: set isWeekend to true if typeOfDay is 'E', otherwise false
// otherwise print these 2 lines:
// Depending upon the destination, and whether it is weekend, day/night
// set the appropriate price
//I recommend using a switch
// I am providing much of the Input and Output dialog to simplify this program
cout << "Each ticket will cost: Ş" << ticketPrice << endl;
cout << "You will get in change: $" << change << endl;
int numTickets;
cout << "How many tickets do you want? ";
cout << "Your tickets have been ordered!";
cin >> num Tickets;
// TODO: calculate the totalCost based on the number of tickets and ticketPrice, then print it
cout << "You owe: $" << totalCost << endl;
return 0;
// TODO: declare a variable that will hold the user's payment
// prompt the user for "Amount paid?"
// read in the user's Payment
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
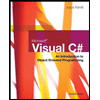
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
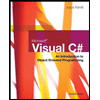
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,