Your program will read in a list of high and low integer temperatures and city names from System.in using the Scanner class, then sort the information three different ways: by high temperature, low temperature, and by city name. It is a requirement of the assignment that an ArrayList is used to store the data internally, and the sort method that ArrayList implements for the List interface is used to do the sorting. Here is some sample input/output. The program would first print out a prompt: Enter high/low temperatures and city: The user might then type or paste the following into the terminal or Netbeans' output window: 60 37 Dallas, TX 61 42 Kansas City, KS 75 57 Cleveland, OH 81 70 Fort Lauderdale, FL 79 55 Seattle, WA . Notice that the input ends with a line starting with a period; as in Programming Project 1, the last line could start with any non-digit character. There is no limit on the number of lines of input the program might receive; since your program is using an ArrayList to store the temperature information for each city, it isn't necessary to know in advance how many lines there could be. Then the program would produce the following output for the above input: ***List in original order*** Dallas, TX 37 60 Kansas City, KS 42 61 Cleveland, OH 57 75 Fort Lauderdale, FL 70 81 Seattle, WA 55 79 ***List sorted by low temperature*** Dallas, TX 37 60 Kansas City, KS 42 61 Seattle, WA 55 79 Cleveland, OH 57 75 Fort Lauderdale, FL 70 81 ***List sorted by high temperature*** Dallas, TX 37 60 Kansas City, KS 42 61 Cleveland, OH 57 75 Seattle, WA 55 79 Fort Lauderdale, FL 70 81 ***List sorted by city name*** Cleveland, OH 57 75 Dallas, TX 37 60 Fort Lauderdale, FL 70 81 Kansas City, KS 42 61 Seattle, WA 55 79 Notice that the city names come first on output but last on input, and that the temperatures are all lined up in a column. This will make it easier for your program to read the data, but it will be printed in a manner that is easier for a human to read *** HERE IS THE CODE****************** Please follow the comments and please do exactly according to the comments. // You will need to provide an appropriate header comment. import java.util.ArrayList; import java.util.Scanner; public class ThreeWaySortTemperatures { public static void main(String[] args) { ArrayList cityTempsList = new ArrayList<>(); Scanner inScanner = new Scanner(System.in); System.out.println("Enter high/low temperatures and city:"); while (inScanner.hasNextInt()) { int hiTemp = inScanner.nextInt(); int loTemp = inScanner.nextInt(); String cityName = inScanner.nextLine().trim(); cityTempsList.add(new CityTemperatures(cityName, loTemp, hiTemp)); } // 1. Print the list out in the same order it came in printList(cityTempsList, "***List in original order***"); // 2. Use cityTempsList.sort with the appropriate parameter // to sort the list by low temperature in increasing order // and then print the list // Sort here printList(cityTempsList, "***List sorted by low temperature***"); // 3. Use cityTempsList.sort with the appropriate parameter // to sort the list by high temperature in increasing order // and then print the list // Sort here printList(cityTempsList, "***List sorted by high temperature***"); // 4. Use cityTempsList.sort with the appropriate parameter // to sort the list by city name in increasing order // and then print the list // Sort here printList(cityTempsList, "***List sorted by city name***"); } // Replace this comment with your own comment describing this method. public static void printList(ArrayList list, String title) { System.out.println(title); // Write the code here to print the contents of list so that the // city names, high temperatures and low temperatures line up // in columns. The order of the data on each row should be // city name, then low temperature, then high temperature. // You will need to calculate the length of the longest // city name to line things up properly. // Once you have followed these directions, // DELETE THIS 8 LINE COMMENT. } } CityTemp.java File== // You will need to replace this comment with an appropriate header comment // and you will need to flesh out this bare-bones class declaration // that does very little as currently declared. public class CityTemperatures { public CityTemperatures(String cityName, int loTemp, int hiTemp) {
Your program will read in a list of high and low integer temperatures and city names from System.in using the Scanner class, then sort the information three different ways: by high temperature, low temperature, and by city name. It is a requirement of the assignment that an ArrayList is used to store the data internally, and the sort method that ArrayList implements for the List interface is used to do the sorting.
Here is some sample input/output. The program would first print out a prompt:
Enter high/low temperatures and city:
The user might then type or paste the following into the terminal or Netbeans' output window:
60 37 Dallas, TX 61 42 Kansas City, KS 75 57 Cleveland, OH 81 70 Fort Lauderdale, FL 79 55 Seattle, WA .
Notice that the input ends with a line starting with a period; as in Programming Project 1, the last line could start with any non-digit character. There is no limit on the number of lines of input the program might receive; since your program is using an ArrayList to store the temperature information for each city, it isn't necessary to know in advance how many lines there could be.
Then the program would produce the following output for the above input:
***List in original order***
Dallas, TX 37 60
Kansas City, KS 42 61
Cleveland, OH 57 75
Fort Lauderdale, FL 70 81
Seattle, WA 55 79
***List sorted by low temperature***
Dallas, TX 37 60
Kansas City, KS 42 61
Seattle, WA 55 79
Cleveland, OH 57 75
Fort Lauderdale, FL 70 81
***List sorted by high temperature***
Dallas, TX 37 60
Kansas City, KS 42 61
Cleveland, OH 57 75
Seattle, WA 55 79
Fort Lauderdale, FL 70 81
***List sorted by city name***
Cleveland, OH 57 75
Dallas, TX 37 60
Fort Lauderdale, FL 70 81
Kansas City, KS 42 61
Seattle, WA 55 79
Notice that the city names come first on output but last on input, and that the temperatures are all lined up in a column. This will make it easier for your program to read the data, but it will be printed in a manner that is easier for a human to read
*** HERE IS THE CODE******************
Please follow the comments and please do exactly according to the comments.
// You will need to provide an appropriate header comment.
import java.util.ArrayList;
import java.util.Scanner;
public class ThreeWaySortTemperatures
{
public static void main(String[] args)
{
ArrayList cityTempsList = new ArrayList<>();
Scanner inScanner = new Scanner(System.in);
System.out.println("Enter high/low temperatures and city:");
while (inScanner.hasNextInt())
{
int hiTemp = inScanner.nextInt();
int loTemp = inScanner.nextInt();
String cityName = inScanner.nextLine().trim();
cityTempsList.add(new CityTemperatures(cityName, loTemp, hiTemp));
}
// 1. Print the list out in the same order it came in
printList(cityTempsList, "***List in original order***");
// 2. Use cityTempsList.sort with the appropriate parameter
// to sort the list by low temperature in increasing order
// and then print the list
// Sort here
printList(cityTempsList, "***List sorted by low temperature***");
// 3. Use cityTempsList.sort with the appropriate parameter
// to sort the list by high temperature in increasing order
// and then print the list
// Sort here
printList(cityTempsList, "***List sorted by high temperature***");
// 4. Use cityTempsList.sort with the appropriate parameter
// to sort the list by city name in increasing order
// and then print the list
// Sort here
printList(cityTempsList, "***List sorted by city name***");
}
// Replace this comment with your own comment describing this method.
public static void printList(ArrayList list, String title)
{
System.out.println(title);
// Write the code here to print the contents of list so that the
// city names, high temperatures and low temperatures line up
// in columns. The order of the data on each row should be
// city name, then low temperature, then high temperature.
// You will need to calculate the length of the longest
// city name to line things up properly.
// Once you have followed these directions,
// DELETE THIS 8 LINE COMMENT.
}
}
CityTemp.java File==
// You will need to replace this comment with an appropriate header comment
// and you will need to flesh out this bare-bones class declaration
// that does very little as currently declared.
public class CityTemperatures
{
public CityTemperatures(String cityName, int loTemp, int hiTemp)
{
}
}
Here are the conditions. Thank You


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

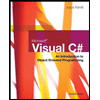
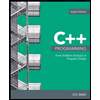
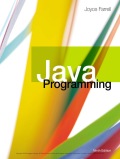
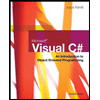
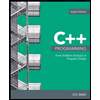
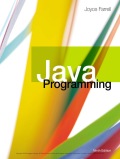