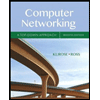
Your task is to implement a function that, given an error tolerance err_tol (a floating-point value between 0 and 1) computes and prints the required input parameters for each
Requirements: Function signature (no return value). Be sure to import the Python modules you created for Parts 1, 2, and 3, e.g., import p1arch.
def pi_allpi(err_tol: float)
Notice that when you run your code repeatedly with the same err_tol input, you get the same values for Archimedes and Wallis (i.e., they are deterministic), but you keep getting different answers for Monte Carlo (non-deterministic). This is because Monte Carlo uses pseudo-random numbers for the darts' positions, and so gets different sets of points with every run.
To make sure you get the same result every time you run, you can initialize the pseudorandom number generator with a fixed seed first, before calling your p3mc.pi_mc function, e.g., random.random(10). This is a common technique used for testing non-deterministic computations.
Part 1
#p1arch
import math
def pi_arch(num_sides: int) -> float:
"""(int) -> float
>>>pi_arch(8)
3.0614674589207183
>>>pi_arch(16)
3.121445152258052
>>>pi_arch(100)
3.141075907812829
"""
inner_angle_b = 360.0 / num_sides
half_angle_a = inner_angle_b/ 2
one_half_side_s = math.sin(math.radians(half_angle_a))
side_s = one_half_side_s * 2
polygon_circumference= num_sides * side_s
pi = polygon_circumference / 2
return pi
Part 2
#p2 Wallis
def pi_wallis(num_pairs: int) ->float
"""(int) -> float
>>>wallis(100)
3.1337874906281575
>>>wallis(1000)
3.1308077460303785
>>>wallis(10000)
3.141514118681855
"""
acc = 1 # initialize accumulator to 1 for multiplication
num = 2 # numerator starts at 2
for a_pair in range(pairs):
left_term = num / (num - 1) # denominator is numerator - 1
right_term = num / (num + 1) # denominator is numerator + 1
acc = acc * left_term * right_term # compute running product
num = num + 2 # prepare for next term
pi = acc * 2
return pi
Part 3
#p3mc (monte carlo)
import random
from math import sqrt
def monte_pi(num_darts: int) -> float:
"""
>>>round(monte_pi(2000_000), 2)
3.14
"""
in_circle=0
for dart in range(num_darts):
x = random.random()
y= random. random()
if sqrt(x**2 + y**2) < 1.0:
in_circle +=1
pi=in_circle / num_darts * 4
return pi

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Please help solve the python problemarrow_forwardWrite a fraction calculator program. Your program should check for the division by 0, have and use the following functions: abs - returns the absolute value of a given integer.min - returns the smallest of two positive integers.gcd - returns the greatest common divisor of two positive integers. the program should run repeatedly until the user wants to quit. please provide an explanation and don't use expert/professional commands. thank you in advance!arrow_forwardDescription of the Problem Write a program to allow the academic staff to enter the coursework marks and final marks for the students. The program reads scores in three categories: test, assignments and final. Each category is weighted: its points are scaled up to a fraction of the 100 percent grade for the course. As the program begins reading each category, it first prompts for the category's weight. The user begins by entering student id and name follows by the scores earned on test. The program asks whether test scores were shifted, interpreting an answer of 1 to mean "yes" and 2 to mean "no." If there is a shift, the program prompts for the shift amount, and the shift is added to the user's test score. Exam scores are capped at a max of 100; for example, if the user got 95 and there was a shift of 10, the score to use would be 100. The midterm's "weighted score" is printed, which is equal to the user's score multiplied by the exam's weight. Next, the program prompts for data about…arrow_forward
- Auto-graded programming assignments may use a Unit test to test small parts of a program. Unlike a Compare output test, which evaluates your program's output for specific input values, a Unit test evaluates individual functions to determines if each function: . is named correctly and has the correct parameters and return type • calculates and returns the correct value (or prints the correct output) name The zyLabs auto-grader runs main.py as a script. In main.py, the line if '__main__': is used to separate the main code from the functions' code so that each function can be unit tested. Enter statements to be run as the main code under if _name__ == '____main__':. Indent the statements so the statements belong to the if block. Refer to the subsection Importing modules and executing scripts under section Module basics for more information about running a program as a script. Note: Do not remove if name program produces the correct output. This example lab uses multiple unit tests to test…arrow_forwardYour first task will be to write a simple movement simulator on a snakes and ladders board. For this questionyou can ignore the snakes and ladders and just simply assume you only have to deal with moving. You willneed to simulate rolling the die - this can be done by using the Python random module and the randint method.Your function play_game will take as input the length of the board (an integer), "play" the game by rolling the diemultiple times until the sum of rolls is larger or equal to the length of the board. (note: this is one of the possibleand simplest end rules). The function should return the total number of rolls required to finish the the particulargame that was played. Obviously this number will vary as it depends on the specific random rolls performedduring the movement simulation.arrow_forwardPlease solve this in python. DO NOT use spicy library. Expected outcome is: 1.2446380979332121 1.251316587879806arrow_forward
- Hello,So for my assignment I had to create a program that would find the sum and then average of a list of numbers. I managed to do that part fine, but I was also meant to use double data type for my variables which I think I may have done right too? But for some reason in my output for my answer Im still getting a whole number, when I should be getting a decimal number. Does anyone see a mistake im making?arrow_forwardUsing Newton Raphson's methodDesign a program in jupyter notebook phyton with programming code for the calculation of hydraulic channels in trapezoidal, quadrangular and triangular shapes.This is to be able to obtain calculations such as depth, area, perimeter, hydraulic radius, water mirror, etc. With his explanation of how the code was made and how the program works.Use the Newton Raphson method and if possible the Secant method or the Bisection methodarrow_forwardWrite a python program with the following functions:1. the function factorial_loop(num) that takes an integer num and returns the value of the factorial of num written as num!, where num! = num * (num-1) * (num-2) * … * 2 * 1. This function must use a loop (iteration) to compute the value of num!.2. the function factorial_recursive(num) that takes an integer num and returns the value of the factorial of num (i.e., num!) as defined above. This function must use a recursive algorithm to compute the value of num!.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
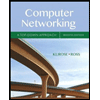
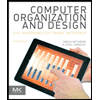
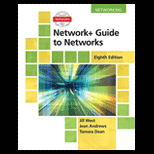
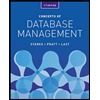
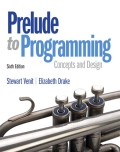
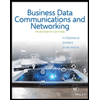