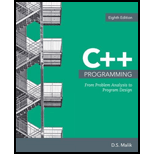
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN: 9781337102087
Author: D. S. Malik
Publisher: Cengage Learning
expand_more
expand_more
format_list_bulleted
Textbook Question
thumb_up100%
Chapter 3, Problem 12SA
What is the output of the following program? (2, 3, 6, 8)
#include <iostream>
#include <cmath>
#include <string>
#include <iomanip>
using namespace std;
int main()
{
double x, y;
string str;
x = 9.0;
y = 3.2;
cout << fixed << showpoint << setprecision (2);
cout << x << "^" << y << " = " << pow(x, y) << endl;
cout << "5.0^2.5 = " << pow(5.0, 2.5) << endl;
cout << "sqrt (48.35) = " << sqrt (48.35) << endl;
cout << "static_cast<int>(sqrt(pow (y, 4))) = "
<< static_cast<int>(sqrt(pow (y, 4))) << endl;
str = "Predefined functions simplify
cout << "Length of str = " << str.length() << endl;
return 0;
}
Expert Solution & Answer

Want to see the full answer?
Check out a sample textbook solution
Students have asked these similar questions
What will be the output of the following program in C++?
#include<iostream>
using namespace std;
int x = 1;
int main()
{
int x = 2;
{
int x = 3;
cout << ::x << endl;
}
return 0;
}
What will be the output of the following program in C++?
#include<iostream>using namespace std;int x = 1;int main(){int x = 2;{int x = 3;cout << ::x << endl;}return 0;}
Question:
Trace and comment each and every line of the C++ program below ;
#include <iostream>
using namespace std;
int binarySearch(int arr[], int left, int right, int num)
{
if (right >= left) {
int mid = left + (right - left) / 2;
if (arr[mid] == num)
return mid;
if (arr[mid] > num)
return binarySearch(arr, left, mid - 1, num);
return binarySearch(arr, mid + 1, right, num);
}
return -1;
}
int main(void)
{
int arr[] = { 2, 3, 4, 8, 10, 40 };
int check = 40;
int n = sizeof(arr) / sizeof(arr[0]);
int result = binarySearch(arr, 0, n - 1, check);
(result == -1)
? cout << "Element is not present in array"
: cout << "Element is present at index " << result;
return 0;
}
Chapter 3 Solutions
C++ Programming: From Problem Analysis to Program Design
Ch. 3 - Mark the following statements as true or false. An...Ch. 3 - Prob. 7SACh. 3 - What does function sqrt do? Which header file must...Ch. 3 - Prob. 9SACh. 3 - Prob. 10SACh. 3 - 11. What is the purpose of the manipulator setw?...Ch. 3 - 12. What is the output of the following program?...Ch. 3 - Prob. 13SACh. 3 - Suppose that name is variable of type string. What...Ch. 3 - 16. Write a C++ statement that uses the...
Ch. 3 - 19. The following program is supposed to read the...Ch. 3 - Prob. 20SACh. 3 - Prob. 21SACh. 3 - 22. Suppose that infile is an ifstream variable...Ch. 3 - 24. Suppose that infile is an 18stream variable...Ch. 3 - 1. Consider the following incomplete C++ program:...Ch. 3 - 2. Consider the following program in which the...Ch. 3 - Write a program that prompts the user to enter the...Ch. 3 - 4. During each summer, John and Jessica grow...Ch. 3 - 5. Three employees in a company are up for a...Ch. 3 - 6. Write a program that accepts as input the mass,...Ch. 3 - Interest on a credit card's unpaid balance is...Ch. 3 - Prob. 8PECh. 3 - Dairy Farm decided to ship milk in containers in...Ch. 3 - 10. Paula and Danny want to plant evergreen trees...
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Consider the following obfuscated and confusing program code below. #include <iostream> using namespace std; int fib(int); int main() { cout << "Enter an index for the Fibonacci number: "; int index; cin >> index; cout << "Fibonacci number at index " << index << " is " << fib(index) << endl; return 0; } int fib(int index) { if (index == 0) return 0; else if (index == 1) return 1; else return fib(index - 1) + fib(index - 2); } Create a C++ Builder Console Project and copy/paste the above code into the .cpp file of the project. Run the project... it should run fine. Now, REFORMAT the code to demonstrate you know Software Engineering principles in making code readable and understandable. FOLLOW the exmples that are given in the textbook and the learning activities. ADD COMMENTS to document what the program/code does. You will be expected to format your code throughout this course using proper indentations and placement of curly braces. STUDY…arrow_forwardIn C programming, if the return type of a function is Void how can you implement assert statements? For example, Void ceasar(int n, char*x); //if char = c and n = 3 output == f how do i use assert statements as this does not work assert (caesar(3,c)==f);arrow_forwardC++ programming 2. What is the output of the following program (when embedded in a complete program)? void Fun( int& x, int y, int& z); int main( ) { int a, b, c; a = 10; b = 20; c = 30; Fun(a, b, c); cout << a << " " << b << " " << c << endl; return 0; } void Fun( int& x, int y, int& z) { cout << x << " " << y << " " << z << endl; x = 1; y = 2; z = 3; }arrow_forward
- The language we are using here is in Racket. Please enter the following code: (cond ((equal? 16 3) (+ 3 8)) ((equal? 16 8) 12) (else (* 6 3))) Write the return value for the above code. If replacing all of the 16's in the above code with 8, what is the return value? What about replacing the 16’s with 3? What does the cond function do?arrow_forwardFix the following c++ code : #include<iostream>using namespace std;void Ashraf(int [][],int,int);int main(){int a[2][3];Ashraf(a,2,3);}void Ashraf (int a[ ] [], int R, int C){for(int r=0; r<R; r++){for(int c=0; c<C; c++){a[r][c]=1;cout<<a[r][c]<<"\t";}cout<<endl;}arrow_forwardplease code this for me in c++ using void, functions, loops, (basic c++ not complicated or highlevel please). I tried to code it myself but there are many mistakes I dont know how to fix so please write a similar one for me but it works. The question is only 1 but it has 2 parts (2 different functions that should be in one code) MY CODE: #include <iostream>using namespace std; int IsPalindrome(int p);void GCD(); int main (){ int choice; cout << "Please enter a choice: "<<endl; cout << "Enter one for IsPalindrome "<<endl; cout << "Enter 2 for GCD "<<endl; cout << "Enter 3 to exit the program "<<endl; cout << "Choice: "<<endl; cin >> choice; if (choice == 1) { int p; cout << "Please enter a 3 digit number: "<<endl; cin >> p; if(p%10==p/100) { cout<<p<<" is a palindrome " <<endl; } else {…arrow_forward
- I need complete explanation of c++ code line by line. Need Explanation of all lines of code what is happening in this code where the data is stored.? Code #include <iostream>#include <iomanip>#include <time.h>#include <fstream>#include <conio.h>#include <string.h>#include <stdlib.h>using namespace std;class Bank {public:};class ATMAccountHolders:public Bank{string accountHolders;string accountHoldersAddress, branch;int accountNumber;double startBalance;double accountBalance; double amount;int count;public:void deposit();void withdraw();void accountExit();ATMAccountHolders(){accountNumber = 7787;accountHolders = " Ammad Naseer";accountHoldersAddress = " House no.112";startBalance = 6000.00;accountBalance = 6000.00;branch = " Islamabad";amount = 20000; }}; void ATMAccountHolders::deposit(){system("cls"); cout<<" ATM ACCOUNT DEPOSIT SYSTEM ";cout<<"\n\nThe Names of the Account Holders are…arrow_forwardWrite a function that counts and returns the number of vowels in the string. (For the purposes of this exercise, we are talking about the standard 5 vowels -- A, E, I, O, U). Write a function that counts and returns the number of consonants in the string. Write a function that converts the string to all lowercase. Write a function that converts the string to all uppercase. Write the following functions. Each of these functions should have a single parameter -- accepting a c-style string as an argument. The function should only do what is specified (note that none of these functions do any output to the screen). Your functions should use const in the prototype wherever it is appropriate: Write the main function that offers a menu and allows the user to test the functions you have implemented. Program C++, must use c- stylearrow_forwardWhat is the output of the following C++ code? #include <iostream> using namespace std; class Base { public: int m; Base(int n=0) : m(n) { cout << "Base" << endl; } }; class Derived: public Base { public: double d; Derived(double de = 0.0) : d(de) { cout << "Derived" << endl; } }; int main() { cout << "Instantiating Base" << endl; Base cBase; cout << "Instantiating Derived" << endl; Derived cDerived; return 0; }arrow_forward
- Write a code for the following using Console.WriteLine.arrow_forwardWhat is the output of the following C++ code? (2, 3) Submit your answer to dropbox. inti=1; int num=2; while(i<5) { num-num*i; i=i+1; } cout<<"i="<<i<<"and num="<<num<<endl;arrow_forwardGive solution in C ++ Language with secreenshoot of source code. Part 01In this task, you need to do the following:• Write a function named displayMessage() that takes user name as input in character array and then shows greetings• Now take the name input in main() and pass the name as an argument to displayMessage() function• Change the displayMessage() method such that it returns the number of characters after displaying the greetings part 02Write a function power that takes two parameters a and b. And it returns the power as ab.arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
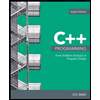
C++ Programming: From Problem Analysis to Program...
Computer Science
ISBN:9781337102087
Author:D. S. Malik
Publisher:Cengage Learning
What Are Data Types?; Author: Jabrils;https://www.youtube.com/watch?v=A37-3lflh8I;License: Standard YouTube License, CC-BY
Data Types; Author: CS50;https://www.youtube.com/watch?v=Fc9htmvVZ9U;License: Standard Youtube License