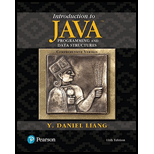
(Algebra: solve quadratic equations) The two roots of a quadratic equation ax2 + bx + c = 0 can be obtained using the following formula:
b2 − 4ac is called the discriminant of the quadratic equation. If it is positive, the equation has two real roots. If it is zero, the equation has one root. If it is negative, the equation has no real roots.
Write a
Note you can use Math. pow(x, 0.5) to compute

Algebra: solve quadratic equation
Program Plan:
- Import the required packages.
- Create a class Exercise
- Define the main method.
- Define the scanner input and prompt user to enter the value of a,b,c.
- Get the input.
- Calculate the discriminant value.
- Condition to validate the discriminant value.
- After validation the value gets of the root gets calculated based on the condition.
- Display the result of roots.
The below program is used to solve the quadratic equation:
Explanation of Solution
Program:
//import the required packages
import java.util.Scanner;
//define the class exercise
public class Exercise
{
public static void main(String[] args)
{
//scanner input
Scanner input = new Scanner(System.in);
/*prompt user to enter the value of the a,b and c*/
System.out.print("Enter a, b, c: ");
//get value of a
double a = input.nextDouble();
//get value of b
double b = input.nextDouble();
//get value of c
double c = input.nextDouble();
//equation to calculate the discriminant
double discriminant = b * b - 4 * a * c;
//condition to validate the discriminant value
if (discriminant < 0)
{
//display result
System.out.println("The equation has no real roots");
}
//condition to validate the discriminant value
else if (discriminant == 0)
{
//equation to calculate the root value
double r1 = -b / (2 * a);
//display result
System.out.println("The equation has one root " + r1);
}
//condition to validate the discriminant value
else
{
// (discriminant > 0)
//equation to calculate the root value
double r1 = (-b + Math.pow(discriminant, 0.5)) / (2 * a);
//equation to calculate the root value
double r2 = (-b - Math.pow(discriminant, 0.5)) / (2 * a);
//display result
System.out.println("The equation has two roots " + r1 + " and " + r2);
}
}
}
Enter a, b, c: 1
2.0
1
The equation has one root -1.0
Additional Output 1:
Enter a, b, c: 1.0
3
1
The equation has two roots -0.3819660112501051 and -2.618033988749895
Additional Output 2:
Enter a, b, c: 1
2
3
The equation has no real roots
Want to see more full solutions like this?
Chapter 3 Solutions
Introduction to Java Programming and Data Structures, Comprehensive Version (11th Edition)
Additional Engineering Textbook Solutions
Starting out with Visual C# (4th Edition)
Web Development and Design Foundations with HTML5 (8th Edition)
Software Engineering (10th Edition)
Starting Out with Java: From Control Structures through Objects (6th Edition)
Modern Database Management (12th Edition)
Java How to Program, Early Objects (11th Edition) (Deitel: How to Program)
- Fill in the blanks to rewrite the following statement with variables: “Given any positive real number, there is a positive real number that is smaller.” (a) Given any positive real number r, there is _____________ s such that s is ___________ . (b) For any _________ , __________ such that s < rarrow_forwardSuppose the value of b is false and the value of x is 0. What is the value of each of the following expressions?arrow_forward
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrMicrosoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,C++ Programming: From Problem Analysis to Program...Computer ScienceISBN:9781337102087Author:D. S. MalikPublisher:Cengage Learning
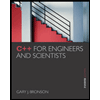
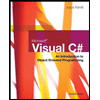
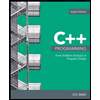