What is “Assign a Value to a Variable”?
“Assigning a value to a variable” means writing a value to the variable. This process involves four entities:
- A data type
- A variable
- The simple assignment operator (=)
- The value that will be copied to the variable
A typical example of assigning a value to a variable is the statement “int a = 4.” Here:
- “int” is the data type
- “a” is the variable
- "=" is the operator
- “4” is the value
Assigning a value is a process that defines the value of a variable or a constant.
A constant is a data item whose value remains constant throughout the execution of the program.
A variable is a data item whose value can vary. The value of a variable changes according to the instructions executed. During the execution of a program, the value of a variable might keep changing.
To see what constants and variables are, let’s start from the bottom up:
A token is the smallest unit of a program that means something to the compiler. Words, punctuation marks and operators are all tokens.
An identifier is a token that has a unique name. In a program, the name is assigned to the token by the programmer. Names can be assigned to variables, constants, functions, arrays, structures, and other constructs.
Seen this way, variables and constants are both examples of identifiers.
What’s Special about an Identifier?
Well, an identifier identifies a particular element in the program—which could be a constant, variable, function, and so forth.
An identifier first needs to be declared. After this, it can be used anywhere in the program to refer to the value associated with it (after initialization).
Identifiers have user-defined names, so there must be some rules for naming identifiers. The rules in the C programming language for naming an identifier or variable are as follows:
- The variable name can only have alphanumeric characters (a-z, A-Z, 0-9) and underscores (_).
- The first character of a variable name must be a letter (a-z, A-Z) or an underscore (_).
- C variable names are case sensitive, so “xyz” and “XYZ” are two different identifiers.
- Special characters (! , . $ @ : ;) are not allowed in the name of variables.
- Keywords cannot be used as C variable. Keywords are special words that have a predefined meaning in C, and which are understood by a C compiler. A programmer can’t change the meaning of a keyword.
There are 32 keywords in C, as in the table below:
auto | double | int | struct | break | else | long | switch |
const | extern | return | union | char | float | short | unsigned |
case | enum | register | typedef | default | goto | size of | void |
continue | for | signed | volatile | do | if | static | while |
Using Variables in Programming
A variable is a container—or space—where a programmer can hold or store data.
The variable name is the name of the memory location where the data associated with the variable is stored.
A data type is an attribute that tells the compiler about the form in which a programmer wants to use the data.
Say “a” is an integer variable. In a 32-bit processor, 4 bytes are allocated to an int variable —as in the figure below:
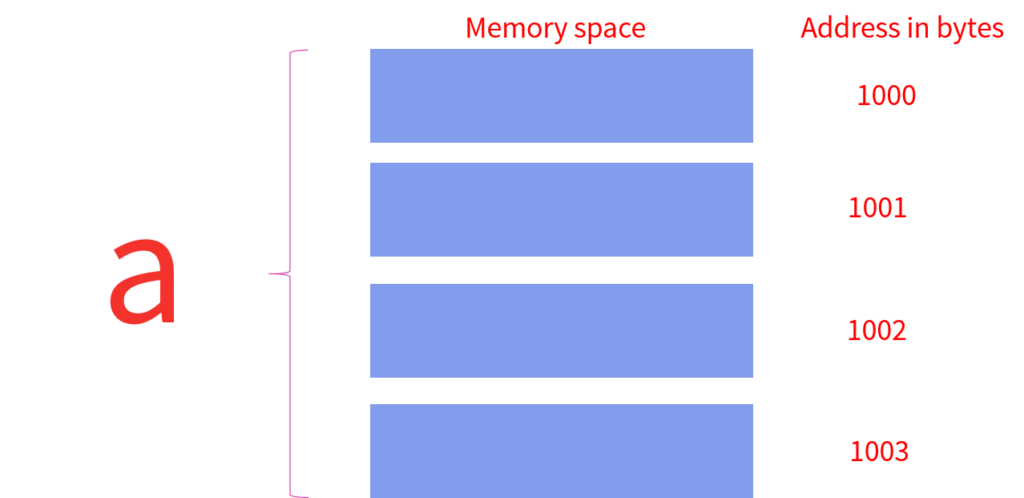
The first byte address allocated to “a” is 1000, and the last byte address allocated is 1003.
Data Types and Their Sizes for Different Compilers
The primary or fundamental data types in common programming languages are the integer type, floating point type, character type and void type.
- The integer data type only stores whole numbers.
- The float data type stores real numbers up to 6 decimal places.
- The char data type only stores characters.
- Void is used to refer to an entity that does not have a value of any type.
This size allocation for these data types varies among compilers. Table 2, below, lists the sizes of different data types in C on different compilers:
Basic data types in C | Size in bytes | ||
16-bit compiler | 32-bit compiler | 64-bit compiler | |
char | 1 | 1 | 1 |
short | 1 | 2 | 2 |
int | 2 | 4 | 4 |
long | 4 | 4 | 8 |
float | 4 | 4 | 4 |
double | 8 | 8 | 8 |
Assigning Values to Variables
Assigning values to variables comprises three processes, as follows:
1. Variable declaration
2. Variable definition
3. Variable initialization
Declaring the variable tells the compiler the variable name, and what type of data the variable will hold. This way, the compiler has information about a variable before using it. Generally, a variable is declared using the extern keyword and outside the main () function. These are called global variable. Here are some examples to initialize global variables:
- extern int a;
- extern float b;
- extern char c;
- extern double d;
Defining the variable indicates to the compiler that it must allocate the required space to the variable so the variable can be used in the program. We can directly define a variable inside the main() function and use it. When we define a variable inside a function, we call it local variable. We can use local variables inside a block or a function where it is declared. The following code example declares local variables a and c.
int main ()
{
int a;
char c;
}
We can even define multiple variables of the same data type in a single line using commas to separate them, as shown in the following statement:
int a, b, c;
Global variables and local variables can be static variable also. The default value of static variables is zero. These variables are initialized only once either inside the function or outside the function. The static variables are alive till the execution of the program.
Variable initialization means initialization of a variable with a value after the variable is declared. Here’s an example:
a = 10;
Here, “a” is the left operand and the variable name, 10 is the right operand and the initial value of "a" and “=” is the assignment operator. The function of the assignment operator is to assign the value of the right operand to the left operand.
We can even define a variable and initialize it (assign it an initial value) in a single statement, as in the following statement where "a" is an integer variable:
int a = 10;
Let’s look at a code segment that sums up what we have learned:
#include <stdio.h>
extern int a; // Variable declaration, global variable
int main ()
{
int a; /* variable definition: */
float b, c; /* local variable, defining multiple variables */
a = 5; /* initialization with assignment statement*/
b = 1.5;
c = 2.5;
/* display the statement */
printf("I need %d balls, %f kg of apples and %f kg of oranges. n", a,b);
return 0;
}
Output: I need 5 balls, 1.5 kg of apples and 2.5 kg of oranges.
Here, %d and %f are format specifiers for the integer and float data types respectively.
Context and Application
Whenever we have to write a line of code, we have to declare variables most of the time. There are very less number of program without a single variable. This topic is significant in the professional exams for both undergraduate and graduate courses, especially for
- Bachelor in Computer Science
- Masters in Computer Science
- Bachelor of Computer Application
- Masters of Computer Application
- Bachelor of Technology in Computer Science and engineering
- Masters of Technology in Computer Science and engineering
Want more help with your computer science homework?
*Response times may vary by subject and question complexity. Median response time is 34 minutes for paid subscribers and may be longer for promotional offers.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.
Search. Solve. Succeed!
Study smarter access to millions of step-by step textbook solutions, our Q&A library, and AI powered Math Solver. Plus, you get 30 questions to ask an expert each month.