WK6
.docx
keyboard_arrow_up
School
American Military University *
*We aren’t endorsed by this school
Course
220
Subject
Computer Science
Date
Feb 20, 2024
Type
docx
Pages
5
Uploaded by ChefSnow3329
ASSIGNMENT:
You are going to enhance the prior assignment by
doing the following.
Use list to create a menu (NOT in the library)
Add a function to the library that will return the results of the four operations in a dictionary object
allInOne(n1, n2)
#Wk6.py
#Student Name: #Course: ENTD220
#Instructor: Dr. Meadors
#Date: 20240210
#Copy Wrong: This is my work
import Wk6_Mylib as Mylib
def main():
try:
lowRange = float(input("Enter your low range:"))
highRange = float(input("Enter your high range:"))
n1 = float(input('Enter your first number:'))
n2 = float(input('Enter your second number:'))
lr = lowRange
hr = highRange
n1, n2 = Mylib.isinrange(lr, hr, n1, n2)
except ValueError:
print("You must enter a number")
else:
calclist = ["1) Add",
"2) Subtract",
"3) Multiply",
"4) Divides",
"5) Scalc",
"6) All in one"]
# printing list for user
for item in calclist:
print(item)
# gathering input for menu selection
menuinput = int(input("Enter your choice: "))
# if then to use menu input and run correct functions
# utilizing many function from library including scalc and dictionary forallinone
# finally an else statement to check for valid input
if menuinput == 1:
print(n1,'+',n2,'=',Mylib.add(n1,n2))
elif menuinput == 2:
print(n1,'-',n2,'=',Mylib.sub(n1,n2))
elif menuinput == 3:
print(n1,'*',n2,'=',Mylib.mult(n1,n2))
elif menuinput == 4:
print(n1,'/',n2,'=',Mylib.div(n1,n2))
elif menuinput == 5:
operator = input("Please choose an opperation [ + - * / ] ")
result_scalc = Mylib.scalc(f"{n1},{n2}", operator)
print(result_scalc)
elif menuinput == 6:
Mylib.AllinOne(n1,n2)
else:
print("Please enter a valid input!")
#Prompt to loop back to beginning.
question = str(input("Do you want to do more calculations? Enter 'yes' or 'no':"))
if question.lower() == "yes":
main()
elif question.lower() == "no":
print("Thank you and have a great day! :-)")
else:
quit()
main()
##################################################
#########################
#Wk6_Mylib.py
#Student Name: #Course: ENTD220
#Instructor: Dr. Meadors
#Date: 20240210
#Copy Wrong: This is my work
def add(n1,n2):
return n1 + n2
def mult(n1,n2):
return n1 * n2
def sub(n1,n2):
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Exercise: empty_dictionary
Description
In this exercise, your function will receive no parameters.
It will create an empty dictionary and return it.
Function Name
empty_dictionary
Parameters
None
Return Value
An empty dictionary.
Examples
empty_dictionary() > 0)
arrow_forward
Homework Assignment-1Topics: Functions, Dictionary, ImportProblem description: Write a python application to simulate an online clothing system. Thepurpose of this assignment is to gain experience in Python dictionary structure, create basicPython functions and import a module.Design solution: The template file contains a list of clothes item sold, each item in the listcontains the item id, name of the item and the prices. The program will generate an items_dictwhere each key is the item id of the cloth and the values will be a list of item name and priceinformation. A customer will be presented with a main menu with 4 different options: displayall items, add an item to cart, checkout and exit the program. A customer can buy an item byentering the ID of the item. This program implements a dictionary called cart, where each key isthe item id and the values are the list of name, price and quantity that user chooses topurchase. The program keeps repeating by displaying the main menu to…
arrow_forward
Data structures
concatenate_dict(dict_list:list)->dict
This function will be given a single parameter known as the Dictionary List. Your job is to combine all the dictionaries found in the dictionary list into a single dictionary and return it. There are two rules for adding values to the dictionary:
1. You must add key-value pairs to the dictionary in the same order they are found in the Dictionary List.
2. If the key already exists, it cannot be overwritten. In other words, if two or more dictionaries have the same key, the key to be added cannot be overwritten by the subsequent dictionaries.
Example:
Dictionary List: [{'Z': 6, 'k': 10, 'w': 3, 'I': 8, 'Y': 5}, {'Y': 1, 'Z': 4}, {'X': 2, 'L': 5}]
Expected: {'Z': 6, 'k': 10, 'w': 3, 'I': 8, 'Y': 5, 'X': 2, 'L': 5}
Dictionary List: [{'z': 0}, {'z': 7}]
Expected: {'z': 0}
Dictionary List: [{'b': 7}, {'b': 10, 'A': 8, 'Z': 2, 'V': 1}]
Expected: {'b': 7, 'A': 8, 'Z': 2, 'V': 1}
arrow_forward
Phyton:
Program 6:
Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it.
You should have the following functions:
· add_from_file() – prompts the user for a file and add the data from it.
· add_by_hand() – prompts the user to enter data until user type a negative number.
· print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions.
Handling errors such as whether the input file exist is extra credit.
To print a 10-column table, you can use the code below:
for i in range(len(data)):
print(f"{data[i]:3}", end="")
if (i+1)%10==0:
print()
print()
Sample run:
Choose an action
1) add data from a file
2) add data by hand
3) print stats
4)…
arrow_forward
Question Completion Status:
3
P
QUESTION 6
Implement the following:
1) Create a dictionary from the following data (the key is the name and the value is the age):
Ann 53
Bill 24
Calvin 36
David 25
2) Use a loop to print all ages on the same line and separated by a space.
3) In the loop above, calculate the average age with a precision of two decimal places.
4) Print the average. Do not just print(34.5).
Just make sure to precisely match the output format below.
Write your code in the ANSWER area provided below (must include comments if using code is not covered in the course).
Example Output
53 24 36 25
Average: 34.50
For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac).
B
IUS
Paragraph
Arial
V
Programs
10pt
Click Save and Submit to save and submit. Click Save All Answers to save all answers.
!!!
描く
A
arrow_forward
import are as are# A dictionary for the simplified dragon text game# The dictionary links a room to other rooms.rooms = {'Great Hall': {'South': 'Bedroom'},'Bedroom': {'North': 'Great Hall', 'East': 'Cellar'},'Cellar': {'West': 'Bedroom'}}location = 'Great Hall' # set starting room# Based on the start room (a key), what is the corresponding dictionary value?print("\n", rooms[location])# Available directionsprint(rooms[location].keys())print(*rooms[location].keys())# Available roomsprint(rooms[location].values())print(*rooms[location].values())direction = ['South', 'North', 'East', 'West']# game loopwhile direction != "exit":print('\nYou are in the',location)possible_moves = rooms[location].keys()print('possible moves', *possible_moves)direction = input("Which direction should I go?").strip().lower()print('You chose to go:', direction)I am supposed to make a program to be able to move from room to room in python, and exit, how do I finish this is as far as I have completed
arrow_forward
Python coding language
Create a static dictionary with a number of users and with the following values:
First name
Last name
Email address
Password
Ask the user for:
5. Email address 6. Password
Loop (for()) through the dictionary and if (if()) the user is found print the following:
7. Hello, first name last name you have successfully logged in 8. Notify the user if the password and email address are wrong 9. Additional challenge: if you want the program to keep asking for a username and password when the combination is wrong, you will need a while() loop. 10. Save the file as assignment03yourlastname.py
arrow_forward
Data structures
dict_from_string(dict_str:str)->dict
This function will be given a single parameter, a string representing a dictionary. Your job is to convert the string into an actual dictionary and return the dictionary. Make sure all key-value pairs in the string exist in the newly created dictionary. The string will contain only numbers or single letters as key values pairs. Make sure all letters are kept as strings and all numbers are converted to integers in the newly created dictionary.
Example:
String Input: '{9: 'V', 'G': 0, 'M': 9, 'u': 3, 2: 'o', 8: 'u', 'q': 9, 'D': 1}'
Expected: {9: 'V', 'G': 0, 'M': 9, 'u': 3, 2: 'o', 8: 'u', 'q': 9, 'D': 1}
String Input: '{10: 'D', 1: 'Z', 5: 'a'}'
Expected: {10: 'D', 1: 'Z', 5: 'a'}
String Input: '{'M': 2, 'V': 0, 3: 'x', 6: 'J', 5: 'J', 7: 'T', 8: 'P', 4: 'q', 1: 'h'}'
Expected: {'M': 2, 'V': 0, 3: 'x', 6: 'J', 5: 'J', 7: 'T', 8: 'P', 4: 'q', 1: 'h'}
String Input: '{3: 'D', 10: 'T', 7: 'm', 'u': 9, 't': 5, 6: 'Z', 'H': 10, 'B':…
arrow_forward
Overview
This is a review assignment where you will use multiple tools you have picked up so far throughout the course. If you're confused about how to do something make sure you look back
at earlier assignments.
In this program you will start by declaring an empty dictionary named population_dict. Then you will use a while-loop to prompt a user for a series of cities and their populations,
storing the input in population_dict). After this you will prompt the user to decide what is the maximum population for a city to be considers a small down. Finally, you will open a file
called populationdata.txt where you will write the results.
Expected Output
Example of standard out (the console):
What is next city? (enter q to quit) Long Beach
What is the population of Long Beach? 362000
What is next city? (enter q to quit) Los Angeles
What is the population of Los Angeles? 382000000
What is next city? (enter q to quit) San Diego
What is the population of San Diego? 136000000
What is next city?…
arrow_forward
Program Three: Lists and Dictionaries
Pizza3.py You need to copy Pizza2.py and make
the following changes:
2.
1. Ask how many pizzas are being purchased.
Use a loop to ask for the size and options of
each pizza. These will be put into a list or a
dictionary.
3.
Your Outputs() should read from the list or
dictionary and format the output as shown
below.
↓
Pizza3
Welcome to Andy's Pizza3 Menu Program
How many pizzas would you like to order? 2
You can choose the pizza size followed by a list of optional toppings
#1 What size pizza would you like to order? S/M/L are the options:m
What toppings would you like to add to customize your pizza?
Enter a Y or N (Yes or No) to the following options:
Would you like cheese? Y/Ny
Choose from the following cheese options:
Please c
Would you like protein? Y/Ny
Choose from the following protein options:
Please enter C/c for Chicken, T/t for Tofu, S/s for Shrimp:c
enter C/c for Cheddar, P/p for Pepper Jack, S/s for Swiss:p
Would you like olives? Y/Ny…
arrow_forward
Program One: Basics
Pizza1.py You do NOT need to use functions or a restart
feature. You DO need comments!
• This program calculates the total cost of buying a Pizza,
and creates an invoice with prices for all the ingredients
and the final total.
• Your program should ask the user to input the size of the
pizza and all the add-ons the user wants.
• Include validation and basic exception handling. Accept
the appropriate letter response in upper or lower case.
• The program must calculate the total price of the pizza
based on the size and the add-ons. You will have all the
add-on prices in your code since the user does not get to
choose what to pay.
• Display the output as shown, controlling for the number
of decimal places, $ sign and alignment.
• Run more than once to test all the pizza options. I will be
testing your program!
Pizza2.py You need to copy Pizza1.py and make the
following changes:
1. Using the MIPO model, rewrite your program so
that a Main() function controls the program…
arrow_forward
Capital QuizWrite a program that creates a dictionary containing the U.S. states as keys, and their capitals as values. (Use the Internet to get a list of the states and their capitals.) The programshould then randomly quiz the user by displaying the name of a state and asking the userto enter that state’s capital. The program should keep a count of the number of correct andincorrect responses. (As an alternative to the U.S. states, the program can use the names ofcountries and their capitals.)
in phyton
arrow_forward
If the following is a pointer based list, give the correct commands that change the list from BEFORE to AFTER.
BEFORE
Plane
Bike
Pickup
Caddy
Truck
Motorbike
Van
Ship
AFTER
Plane
Bike
Caddy
Truck
Motorbike
Van
Scooter
Ship
Select one:
A.insert (5, "Scooter"); delete ("Pickup")
B.insert (6, "Scooter"); delete ("Pickup")
C.delete ("Scooter"); insert(2, "Pickup")
D.delete ("Pickup"); insert (6, "Scooter")
arrow_forward
A ____ supports manipulation of items at any point within a linear collection.
Question 5 options:
dictionary
list
stack
queue
arrow_forward
A Dictionary object’s Remove method returns an integer indicating the number of elements that were removed.
Question 48 options:
True
False
arrow_forward
Program Specification
For this assignment you will write a program to help people record the events of their day by supplying prompts and then saving their responses along with the question and the date to a file.
Functional Requirements
This program must contain the following features:
Write a new entry - Show the user a random prompt (from a list that you create), and save their response, the prompt, and the date as an Entry.
Display the journal - Iterate through all entries in the journal and display them to the screen.
Save the journal to a file - Prompt the user for a filename and then save the current journal (the complete list of entries) to that file location.
Load the journal from a file - Prompt the user for a filename and then load the journal (a complete list of entries) from that file. This should replace any entries currently stored the journal.
Provide a menu that allows the user choose these options
Your list of prompts must contain at least five different prompts.…
arrow_forward
Please code in python
there's a dictionary original_dict = {'jack': 9, 'michael': 48, 'guido': 57, 'john': 33} . Using the generator of dictionaries create a new dictionary, all the entries need to be less than 40 and divide by 3 without the remainder.) (need to use if, multiple conditions)
arrow_forward
Code the following directions in python:
1.Write a statement that creates a dictionary containing the following key-value pairs:
'a' : 1
'b' : 2
'c' : 3
2.Write a statement that creates an empty dictionary.
3.Assume the variable dct references a dictionary. Write an if statement that determines whether the key 'James' exists in the dictionary. If so, display the value that is associated with that key. If the key is not in the dictionary, display a message indicating so.
arrow_forward
Assume the following list exists:
names = ['Chris', 'Katie', 'Joanne', 'Kurt']
Write a statement that uses a dictionary comprehension to create a dictionary named X in which each element contains the length of a name from the list as the key, and the corresponding name's initial character as its value.
arrow_forward
def city_dict(adict): """ Question 6 -Given a dictionary that maps a person to a list of countries they want to travel to, return a dictionary with the value being the list sorted by the last letter in each countries name. If two countries have the same last letter, sort by the first letter.
-For an extra challenge, try doing this in one line (Optional).
Args: adict (dict) Returns: dict
>>> city_dict({"Pablo": ["Belgium", "Canada", "Germany"], "Athena": ["Italy", "France", "Egypt"], "Liv": ["Japan", "Bolivia", "Greece"]})
{'Pablo': ['Canada', 'Belgium', 'Germany'], 'Athena': ['France', 'Egypt', 'Italy'], 'Liv': ['Bolivia', 'Greece', 'Japan']}
>>> city_dict({"Jacob": ["Bahamas", "Brazil", "Chile"], "Lexi": ["Colombia", "Finland", "Panama"], "Emily": ["Ireland", "Russia", "Kenya", "Jordan"]})
{'Jacob': ['Chile', 'Brazil', 'Bahamas'], 'Lexi': ['Colombia', 'Panama', 'Finland'], 'Emily': ['Kenya',…
arrow_forward
Each numeric position in a list is called a(n) ____.
Question 6 options:
index
pointer
reference
iterator
arrow_forward
Overview
This program will have you cut and past a dictionary called food_dict from the specifications, the programmatically go through the list to find people who have
not yet listed their favorite foods. It will then ask for their foods, update the dictionary, and finally print it at the end.
Note: I will change the dictionary during grading
Expected Output
Example 1
What is Janelle's favorite food? Steak
What is Thomas's favorite food?
What is Yolanda's favorite food? Soup
Here are the favorite foods:
Jim's favorite food is Tacos
Bob's favorite food is Burgers
Janelle's favorite food is Steak
Lisa's favorite food is Pizza
Thomas's favorite food is Pho
Yolanda's favorite food is Soup
Finn's favorite food is Bread
Specifications
• You should submit a single file called M4A5.py
• It should follow the submission standards outlined here: Submission Standards
• Your program must loop through the dictionary to find empty values.
• You must copy this dictionary definition to the top of your…
arrow_forward
Word IndexWrite a python program that reads the contents of a text file. The program should create a dictionary inwhich the key-value pairs are described as follows:Key. The keys are the individual words found in the file.576Values. Each value is a list that contains the line numbers in the file where the word (thekey) is found.For example, suppose the word “robot” is found in lines 7, 18, 94, and 138. The dictionarywould contain an element in which the key was the string “robot”, and the value was a listcontaining the numbers 7, 18, 94, and 138.Once the dictionary is built, the program should create another text file, known as a word index,listing the contents of the dictionary. The word index file should contain an alphabetical listingof the words that are stored as keys in the dictionary, along with the line numbers where thewords appear in the original file. Figure 9-1 shows an example of an original text file(Kennedy.txt) and its index file (index.txt
arrow_forward
Code the following directions in python.
1.Assume the variable dct references a dictionary. Write an if statement that determines whether the key 'Jim' exists in the dictionary. If so, delete 'Jim' and its associated value.
2.Write code to create a set with the following integers as members: 10, 20, 30, and 40.
3.Assume each of the variables set1 and set2 references a set. Write code that creates another set containing all the elements of set1 and set2, and assigns the resulting set to the variable set3.
arrow_forward
This is question 5 on the same chapter 9
arrow_forward
flip_matrix(mat:list)->list
You will be given a single parameter a 2D list (A list with lists within it) this will look like a 2D matrix when printed out, see examples below. Your job is to flip the matrix on its horizontal axis. In other words, flip the matrix horizontally so that the bottom is at top and the top is at the bottom. Return the flipped matrix.
To print the matrix to the console:
print('\n'.join([''.join(['{:4}'.format(item) for item in row]) for row in mat]))
Example:
Matrix:
W R I T X
H D R L G
L K F M V
G I S T C
W N M N F
Expected:
W N M N F
G I S T C
L K F M V
H D R L G
W R I T X
Matrix:
L C
S P
Expected:
S P
L C
Matrix:
A D J
A Q H
J C I
Expected:
J C I
A Q H
A D J
arrow_forward
C++
Write a program that will allow a user to enter students into a database.
The student information should include full name, student ID, GPA and status.
The program should allow delete any student based on student ID. You may not use a vecto or an array only the list function.
arrow_forward
This assignment requires you to create a dictionary by reading the text file.
Text file name: LightningP
Text file contents:
Stamkos3538Maroon2933Point5055Kucherov5565Killorn3340
The dictionary should have player names for the keys. The value for each key must be a two-element list holding the player's goals and assists, respectively. Start with an empty dictionary. Then, use a loop to cycle through the text file and add key-value pairs to the dictionary. Close the text file and process the dictionary to print the stats and determine the top scorer as before. Printing the stats for each player is the most challenging part of this program. The required output should be the same well-formatted table as below:
PLAYER COLUMN: 10 characters wide and left-aligned.
GOALS COLUMN: 6 characters wide and centered.
ASSISTS COLUMN: 8 characters wide and centered.
TOTAL COLUMN: 6 characters wide and centered.
Example output:
arrow_forward
def delete_item(...): """ param: info_list - a list from which to remove an item param: idx (str) - a string that is expected to contain an integer index of an item in the in_list param: start_idx (int) - an expected starting value for idx (default is 0); gets subtracted from idx for 0-based indexing
The function first checks if info_list is empty. The function then calls is_valid_index() to verify that the provided index idx is a valid positive index that can access an element from info_list. On success, the function saves the item from info_list and returns it after it is deleted from info_list.
returns: If info_list is empty, return 0. If is_valid_index() returns False, return -1. Otherwise, on success, the function returns the element that was just removed from info_list.
Helper functions: - is_valid_index() """
arrow_forward
Create an application to keep track of a list of students and their grades in a data structures course using a binary search tree.• Create a class called student that contains 2 data members: name and grade and then create a binary search tree of objects of this class. Make sure you validate the grade (A through F, and ? for in-progress grades).• Create a menu that has the following options:o Add a student -- add the student if he/she is not already in the classo Remove a student -- display “Not Found” error message if the student is not in the class.o Update the grade -- display “Not Found” error message if the student is not in the class.o Display class roster
The program should use the binary tree definition.
Please list all main, cpp, and hpp files. There must be a Student class, with Student.hpp and Student.cpp files. Do not use structures.
arrow_forward
Dictionaries:
Given the following dictionary definitions:
Foo = {1:’B’, 2:’D’, 3:’C’, 4:’A’}
Bar = {A:’3’,’B’:1,’C’:2,’D’:4}
Give the resulting value for each of the following expressions:
Foo[Bar[‘A’]]
Foo[Bar[‘B’]]
Bar[Foo[1]]
Bar[Foo[2]]
Use a for loop to display the key/value pairs in the above dictionary, one pair to a line.
arrow_forward
paython programing question
Write a program that creates a list of N elements (Hint: use append() function), swaps the first and the last element and prints the list on the screen. N should be a dynamic number entered by the user.
Sample output:
How many numbers would you like to enter?: 5
Enter a number: 34
Enter a number: 67
Enter a number: 23
Enter a number: 90
Enter a number: 12
The list is: [12, 67,23, 90, 34]
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
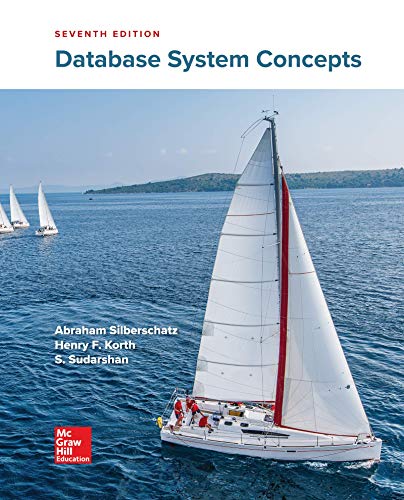
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
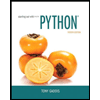
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
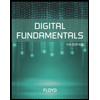
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
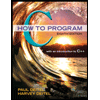
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
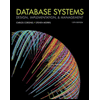
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
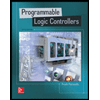
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Exercise: empty_dictionary Description In this exercise, your function will receive no parameters. It will create an empty dictionary and return it. Function Name empty_dictionary Parameters None Return Value An empty dictionary. Examples empty_dictionary() > 0)arrow_forwardHomework Assignment-1Topics: Functions, Dictionary, ImportProblem description: Write a python application to simulate an online clothing system. Thepurpose of this assignment is to gain experience in Python dictionary structure, create basicPython functions and import a module.Design solution: The template file contains a list of clothes item sold, each item in the listcontains the item id, name of the item and the prices. The program will generate an items_dictwhere each key is the item id of the cloth and the values will be a list of item name and priceinformation. A customer will be presented with a main menu with 4 different options: displayall items, add an item to cart, checkout and exit the program. A customer can buy an item byentering the ID of the item. This program implements a dictionary called cart, where each key isthe item id and the values are the list of name, price and quantity that user chooses topurchase. The program keeps repeating by displaying the main menu to…arrow_forwardData structures concatenate_dict(dict_list:list)->dict This function will be given a single parameter known as the Dictionary List. Your job is to combine all the dictionaries found in the dictionary list into a single dictionary and return it. There are two rules for adding values to the dictionary: 1. You must add key-value pairs to the dictionary in the same order they are found in the Dictionary List. 2. If the key already exists, it cannot be overwritten. In other words, if two or more dictionaries have the same key, the key to be added cannot be overwritten by the subsequent dictionaries. Example: Dictionary List: [{'Z': 6, 'k': 10, 'w': 3, 'I': 8, 'Y': 5}, {'Y': 1, 'Z': 4}, {'X': 2, 'L': 5}] Expected: {'Z': 6, 'k': 10, 'w': 3, 'I': 8, 'Y': 5, 'X': 2, 'L': 5} Dictionary List: [{'z': 0}, {'z': 7}] Expected: {'z': 0} Dictionary List: [{'b': 7}, {'b': 10, 'A': 8, 'Z': 2, 'V': 1}] Expected: {'b': 7, 'A': 8, 'Z': 2, 'V': 1}arrow_forward
- Phyton: Program 6: Write a program that allows the user to add data from files or by hand. The user may add more data whenever they want. The print also allows the user to display the data and to print the statistics. The data is store in a list. It’s easier if you make the list global so that other functions can access it. You should have the following functions: · add_from_file() – prompts the user for a file and add the data from it. · add_by_hand() – prompts the user to enter data until user type a negative number. · print_stats() – print the stats (min, max, mean, sum). You may use the built-in functions. Handling errors such as whether the input file exist is extra credit. To print a 10-column table, you can use the code below: for i in range(len(data)): print(f"{data[i]:3}", end="") if (i+1)%10==0: print() print() Sample run: Choose an action 1) add data from a file 2) add data by hand 3) print stats 4)…arrow_forwardQuestion Completion Status: 3 P QUESTION 6 Implement the following: 1) Create a dictionary from the following data (the key is the name and the value is the age): Ann 53 Bill 24 Calvin 36 David 25 2) Use a loop to print all ages on the same line and separated by a space. 3) In the loop above, calculate the average age with a precision of two decimal places. 4) Print the average. Do not just print(34.5). Just make sure to precisely match the output format below. Write your code in the ANSWER area provided below (must include comments if using code is not covered in the course). Example Output 53 24 36 25 Average: 34.50 For the toolbar, press ALT+F10 (PC) or ALT+FN+F10 (Mac). B IUS Paragraph Arial V Programs 10pt Click Save and Submit to save and submit. Click Save All Answers to save all answers. !!! 描く Aarrow_forwardimport are as are# A dictionary for the simplified dragon text game# The dictionary links a room to other rooms.rooms = {'Great Hall': {'South': 'Bedroom'},'Bedroom': {'North': 'Great Hall', 'East': 'Cellar'},'Cellar': {'West': 'Bedroom'}}location = 'Great Hall' # set starting room# Based on the start room (a key), what is the corresponding dictionary value?print("\n", rooms[location])# Available directionsprint(rooms[location].keys())print(*rooms[location].keys())# Available roomsprint(rooms[location].values())print(*rooms[location].values())direction = ['South', 'North', 'East', 'West']# game loopwhile direction != "exit":print('\nYou are in the',location)possible_moves = rooms[location].keys()print('possible moves', *possible_moves)direction = input("Which direction should I go?").strip().lower()print('You chose to go:', direction)I am supposed to make a program to be able to move from room to room in python, and exit, how do I finish this is as far as I have completedarrow_forward
- Python coding language Create a static dictionary with a number of users and with the following values: First name Last name Email address Password Ask the user for: 5. Email address 6. Password Loop (for()) through the dictionary and if (if()) the user is found print the following: 7. Hello, first name last name you have successfully logged in 8. Notify the user if the password and email address are wrong 9. Additional challenge: if you want the program to keep asking for a username and password when the combination is wrong, you will need a while() loop. 10. Save the file as assignment03yourlastname.pyarrow_forwardData structures dict_from_string(dict_str:str)->dict This function will be given a single parameter, a string representing a dictionary. Your job is to convert the string into an actual dictionary and return the dictionary. Make sure all key-value pairs in the string exist in the newly created dictionary. The string will contain only numbers or single letters as key values pairs. Make sure all letters are kept as strings and all numbers are converted to integers in the newly created dictionary. Example: String Input: '{9: 'V', 'G': 0, 'M': 9, 'u': 3, 2: 'o', 8: 'u', 'q': 9, 'D': 1}' Expected: {9: 'V', 'G': 0, 'M': 9, 'u': 3, 2: 'o', 8: 'u', 'q': 9, 'D': 1} String Input: '{10: 'D', 1: 'Z', 5: 'a'}' Expected: {10: 'D', 1: 'Z', 5: 'a'} String Input: '{'M': 2, 'V': 0, 3: 'x', 6: 'J', 5: 'J', 7: 'T', 8: 'P', 4: 'q', 1: 'h'}' Expected: {'M': 2, 'V': 0, 3: 'x', 6: 'J', 5: 'J', 7: 'T', 8: 'P', 4: 'q', 1: 'h'} String Input: '{3: 'D', 10: 'T', 7: 'm', 'u': 9, 't': 5, 6: 'Z', 'H': 10, 'B':…arrow_forwardOverview This is a review assignment where you will use multiple tools you have picked up so far throughout the course. If you're confused about how to do something make sure you look back at earlier assignments. In this program you will start by declaring an empty dictionary named population_dict. Then you will use a while-loop to prompt a user for a series of cities and their populations, storing the input in population_dict). After this you will prompt the user to decide what is the maximum population for a city to be considers a small down. Finally, you will open a file called populationdata.txt where you will write the results. Expected Output Example of standard out (the console): What is next city? (enter q to quit) Long Beach What is the population of Long Beach? 362000 What is next city? (enter q to quit) Los Angeles What is the population of Los Angeles? 382000000 What is next city? (enter q to quit) San Diego What is the population of San Diego? 136000000 What is next city?…arrow_forward
- Program Three: Lists and Dictionaries Pizza3.py You need to copy Pizza2.py and make the following changes: 2. 1. Ask how many pizzas are being purchased. Use a loop to ask for the size and options of each pizza. These will be put into a list or a dictionary. 3. Your Outputs() should read from the list or dictionary and format the output as shown below. ↓ Pizza3 Welcome to Andy's Pizza3 Menu Program How many pizzas would you like to order? 2 You can choose the pizza size followed by a list of optional toppings #1 What size pizza would you like to order? S/M/L are the options:m What toppings would you like to add to customize your pizza? Enter a Y or N (Yes or No) to the following options: Would you like cheese? Y/Ny Choose from the following cheese options: Please c Would you like protein? Y/Ny Choose from the following protein options: Please enter C/c for Chicken, T/t for Tofu, S/s for Shrimp:c enter C/c for Cheddar, P/p for Pepper Jack, S/s for Swiss:p Would you like olives? Y/Ny…arrow_forwardProgram One: Basics Pizza1.py You do NOT need to use functions or a restart feature. You DO need comments! • This program calculates the total cost of buying a Pizza, and creates an invoice with prices for all the ingredients and the final total. • Your program should ask the user to input the size of the pizza and all the add-ons the user wants. • Include validation and basic exception handling. Accept the appropriate letter response in upper or lower case. • The program must calculate the total price of the pizza based on the size and the add-ons. You will have all the add-on prices in your code since the user does not get to choose what to pay. • Display the output as shown, controlling for the number of decimal places, $ sign and alignment. • Run more than once to test all the pizza options. I will be testing your program! Pizza2.py You need to copy Pizza1.py and make the following changes: 1. Using the MIPO model, rewrite your program so that a Main() function controls the program…arrow_forwardCapital QuizWrite a program that creates a dictionary containing the U.S. states as keys, and their capitals as values. (Use the Internet to get a list of the states and their capitals.) The programshould then randomly quiz the user by displaying the name of a state and asking the userto enter that state’s capital. The program should keep a count of the number of correct andincorrect responses. (As an alternative to the U.S. states, the program can use the names ofcountries and their capitals.) in phytonarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
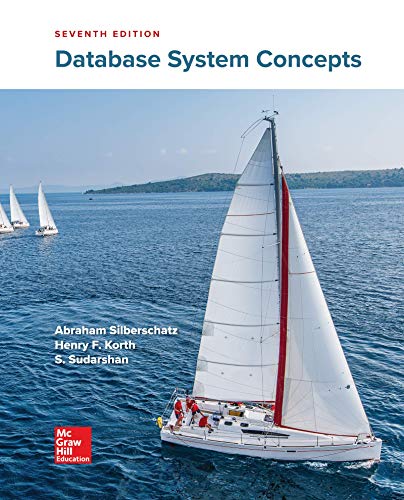
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
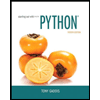
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
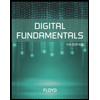
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
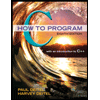
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
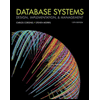
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
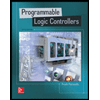
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education