lab2_listbag
.py
keyboard_arrow_up
School
Carleton University *
*We aren’t endorsed by this school
Course
2100
Subject
Computer Science
Date
Feb 20, 2024
Type
py
Pages
4
Uploaded by BarristerElk2701
# SYSC 2100 Winter 2024 Lab 2
# An implementation of ADT Bag that uses an instance of Python's built-in
# list type as the underlying data structure.
import random
__author__ = 'Belal Alobieda'
__student_number__ = '101151327'
class ListBag:
def __init__(self, iterable=[]) -> None:
"""Initialize this ListBag.
If no iterable is provided, the new ListBag is empty.
Otherwise, initialize the ListBag by adding the values
provided by the iterable.
>>> bag = ListBag()
>>> bag
ListBag([])
>>> bag = ListBag([1, 4, 3, 6, 3])
>>> bag
ListBag([1, 4, 3, 6, 3])
"""
self._elems = []
for item in iterable:
self._elems.append(item)
# or, self.add(item)
def __str__(self) -> str:
"""Return a string representation of this ListBag.
>>> bag = ListBag()
>>> str(bag)
'{}'
>>> bag = ListBag([1, 4, 3, 6, 3])
>>> str(bag)
'{1, 4, 3, 6, 3}'
Note: Depending on how __str__ is implemented, the order of the
elements in the returned string may be different.
"""
# Use the string representation of the bag's underlying list,
# with the enclosing '[]' replaced by '{}'.
s = str(self._elems)
return '{' + s[1:len(s) - 1] + '}'
def __repr__(self) -> str:
"""Return the canonical string representation of this ListBag.
>>> bag = ListBag()
>>> repr(bag)
'ListBag([])'
>>> bag = ListBag([3, 1, 2, 3, 4])
>>> repr(bag)
'ListBag([3, 1, 2, 3, 4])'
Note: Depending on how __repr__ is implemented, the order of the
list elements in the returned string may be in a different.
"""
# For a ListBag object, obj, the expression eval(repr(obj))
# returns a new ListBag that is equal to obj.
return "{0}({1})".format(self.__class__.__name__, self._elems)
# or, return self.__class__.__name__ + '(' + str(self._elems) + ')'
def __len__(self) -> int:
"""Return the number of items in this ListBag.
>>> bag = ListBag()
>>> len(bag)
0
>>> bag = ListBag([1, 4, 3, 6])
>>> len(bag)
4
"""
return len(self._elems)
def __iter__(self) -> 'list_iterator':
"""Return an iterator for this ListBag.
>>> bag = ListBag([3, 6, 3])
>>> for x in bag:
... print(x)
...
3
6
3
"""
return iter(self._elems)
def __contains__(self, item: any) -> bool:
"""Return True if item is in this ListBag; otherwise False.
>>> bag = ListBag()
>>> 2 in bag
False
>>> bag = ListBag([1, 4, 3, 6])
>>> 4 in bag
True
>>> 7 in bag
False
"""
return item in self._elems
def add(self, item: any) -> None:
"""Add item to this ListBag.
>>> bag = ListBag([1, 4, 3, 6])
>>> bag.add(3)
>>> len(bag)
5
>>> str(bag)
'{1, 4, 3, 6, 3}'
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
data structures in java
arrow_forward
Exercise, maxCylinderVolume
F# system function such as min or methods in the list module such as List.map are not allowed
Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0.
Examples:
> maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304
arrow_forward
In C++ Write the DLList member function void DLList <T>:: RemoveAllDuplicatesOf (T& x) function, which delete all the occurrences of x (but one) in a sorted DLL.
Example: If the current list is [5, 7, 7, 7, 10, 15, 25, 25]then calling:RemoveAllDuplicatesOf(7); will change the current list to [5, 7, 10, 15, 25, 25}
arrow_forward
Write a Python Code for the given constructor and conditions:
Given Constructor: def __init__ (self, a)
Pre-condition: Array cannot be empty.
Post-condition: This is the default constructor of MyList class. This constructor creates a list from an array.
arrow_forward
In C++ Plz
LAB: Grocery shopping list (linked list: inserting at the end of a list)
Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node.
Ex. if the input is:
4 Kale Lettuce Carrots Peanuts
where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list.
The output is:
Kale Lettuce Carrots Peanuts
ItemNode.h Default Code:
#include <iostream>#include <string>using namespace std;
class ItemNode {private: string item; ItemNode* nextNodeRef;
public: // Constructor ItemNode() { item = ""; nextNodeRef = NULL; }
// Constructor ItemNode(string itemInit) { this->item = itemInit; this->nextNodeRef = NULL; }
// ConstructorItemNode(string itemInit, ItemNode *nextLoc) {this->item = itemInit;this->nextNodeRef = nextLoc;}
// Insert node after this…
arrow_forward
In C++ Write the DLList member function bool DLLidt<T>::insert_before(int index, T& x) that is going to insert a node with the value of x before the node at position index in the list.
Example:
If the current list is [5, 6, 7, 8]
insert_before(2,0);
will change the current list to [5,6,0,7,8]
**You need to use get_at function, and use it only one time to optimize the call of your code.
** Don’t forget to fix the next, and prev pointers for each node.
** for inserting before 0 index, you may use add_to_head function.
arrow_forward
Programming in C:
Challenge 1: Create and Print a Doubly Linked List
In class, I briefly mentioned how to create a doubly linked list (a linked list with two directions: forward and backwards). This task challenges you to create a doubly linked list of 5 nodes. If your nodes were called n1, n2, n3, n4 and n5 (which they shouldn’t, please give them original names), your list should be linked as follows:
We discussed what "start" and "end" should be.
Then, also in main, you will have to print the list forwards by taking advantage of the arrows pointing to the right. You will also have to print it backwards taking advantage of the arrows pointing to the left. Again, everything must be done in main. Hint: look at the way in which I printed the singly linked list in class.
In other words, if your nodes had the values: n1=1, n2=2, n3=3, n4=4 and n5=5; your program should print:1 3 5 2 4 (forwards) and 4 2 5 3 1 (backwards). Please give different values to your nodes, they should not have…
arrow_forward
C++ ProgrammingActivity: Deque Linked List
Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow.
#include "deque.h"
#include "linkedlist.h"
#include <iostream>
using namespace std;
class DLLDeque : public Deque {
DoublyLinkedList* list;
public:
DLLDeque() {
list = new DoublyLinkedList();
}
void addFirst(int e) {
list->addAt(e,1);
}
void addLast(int e) {
list->addAt(e,size()+1);
}
int removeFirst() {
return list->removeAt(1);
}
int removeLast() {
return list->removeAt(size());
}
int size(){
return list->size();
}
bool isEmpty() {
return list->isEmpty();
}
// OPTIONAL: a helper method to help you debug
void print() {…
arrow_forward
data structures in java
arrow_forward
Complete the code:-
class MyList:
# constructor
def __init__(self, a):
# converting array to list
newList = a.tolist()
# printing the list
print("List is:", newList).
arrow_forward
for c++ please thank you
please type the code so i can copy and paste easily thanks.
4, List search
Modify the linked listv class you created in the previous programming challange to include a member function named search that returns the position of a specific value, x, in the lined list. the first node in the list is at position 0, the second node is at position 1, and so on. if x is not found on the list, the search should return -1 test the new member function using an approprate driver program.
here is my previous programming challange.
#include <iostream>
using namespace std;
struct node
{
int data;
node *next;
};
class list
{
private:
node *head,*tail;
public:
list()
{
head = NULL;
tail = NULL;
}
~list()
{
}
void append()
{
int value;
cout<<"Enter the value to append: ";
cin>>value;
node *temp=new node;
node *s;
temp->data = value;
temp->next = NULL;
s = head;
if(head==NULL)
head=temp;
else
{
while (s->next != NULL)
s = s->next;
s->next =…
arrow_forward
Read this: Complete the code in Visual Studio using C++ Programming Language with 1 file or clear answer!!
- Give me the answer in Visual Studio so I can copy***
- Separate the files such as .cpp, .h, or .main if any!
Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts
Develop the following functionality:
Develop a linked list node struct/class
You can use it as a subclass like in the book (Class contained inside a class)
You can use it as its own separate class
Your choice
Maintain a private pointer to a node class pointer (head)
Constructor
Initialize head pointer to null
Destructor
Make sure to properly delete every node in your linked list
push_front(value)
Insert the value at the front of the linked list
pop_front()
Remove the node at the front of the linked list
If empty, this is a no operation
operator <<
Display the contents of the linked list just like you would print a character…
arrow_forward
code
arrow_forward
data structures-java language
quickly pls
arrow_forward
1. Create a doubly-linked list of string and add the following
methods
Add at the head
b. Add at the tail
Remove from head
4. Remove from tail
arrow_forward
# define stemmer functionstemmer = SnowballStemmer('english')
# tokenise datatokeniser = TreebankWordTokenizer()tokens = tokeniser.tokenize(data)
# define lemmatiserlemmatizer = WordNetLemmatizer()
# bag of wordsdef bag_of_words_count(words, word_dict={}): """ this function takes in a list of words and returns a dictionary with each word as a key, and the value represents the number of times that word appeared""" for word in words: if word in word_dict.keys(): word_dict[word] += 1 else: word_dict[word] = 1 return word_dict
# remove stopwordstokens_less_stopwords = [word for word in tokens if word not in stopwords.words('english')]
# create bag of wordsbag_of_words = bag_of_words_count(tokens_less_stopwords)
Use the stemmer and lemmatizer functions (defined in the cells above) from the relevant library to find the stem and lemma of the nth word in the token list.
Function Specifications:
Should take a list as input and…
arrow_forward
struct insert_at_back_of_dll {
// Function takes a constant Book as a parameter, inserts that book at the
// back of a doubly linked list, and returns nothing.
void operator()(const Book& book) {
/ // TO-DO (2) ||||
// Write the lines of code to insert "book" at the back of "my_dll".
//
// // END-TO-DO (2) |||
}
std::list& my_dll;
};
arrow_forward
Programming language: Java
Topic: linked list
arrow_forward
help
arrow_forward
Please complete the ADT SortedList by finishing all the constructors and methods in the class template SortedList.h (C++)
SortedList.h:
#ifndef _SORTED_LIST
#define _SORTED_LIST
#include"Node.h"
template<class ItemType>
class SortedList
{
private:
Node<ItemType> *head;
int itemCount;//Current count of list items
Node<ItemType> *getNodeBefore(const ItemType &anEntry)const;
Node<ItemType> *getNodeAt(int position) const; //Return a pointer that hold the address of the node at 'position'
public:
SortedList(); //Default constructor
SortedList(const LinkedSortedList<ItemType> &aList); //Copy constructor
bool isEmpty() const; //Check if the list empty (no item stored in the array)
int getLength() const; //Returns the number of items in the list
bool remove(int position); //Remove an entry at given position from the list
void clear(); //remove all the items from the list
ItemType getEntry(int position) const; //Retrieves an item on the list at the…
arrow_forward
### Q5: Reduce No Change Python
def reduce_no_change(fn, lst, base):
"""Same as Q4. However, preserve the lst in this problem.
Object can be any python type which the input
Not Allowed To Import Libraries
Args:
fn (function): Combination function which takes in two arguments and return
an value with the same type as the second argument
lst (List): A list of any type
base (Object): A value of custom type which fn can handle.
Returns:
Object: A value after applying fn on lst.
>>> reducer = lambda x, y: x + y
>>> lst = [1, 2, 3]
>>> a = reduce_lst(reducer, lst, 0)
>>> a # a = reducer(reducer(reducer(base, lst[0]), lst[1]), lst[2])
6
>>> lst
>>> [1, 2, 3] # we preserve the list
"""
### Modify your code here
### Modify your code here
arrow_forward
Question 2: Linked List Implementation
You are going to create and implement a new Linked List class. The Java Class name is
"StringLinkedList". The Linked List class only stores 'string' data type. You should not change the
name of your Java Class. Your program has to implement the following methods for the
StringLinked List Class:
1. push(String e) - adds a string to the beginning of the list (discussed in class)
2. printList() prints the linked list starting from the head (discussed in class)
3. deleteAfter(String e) - deletes the string present after the given string input 'e'.
4. updateToLower() - changes the stored string values in the list to lowercase.
5. concatStr(int p1, int p2) - Retrieves the two strings at given node positions p1 and p2. The
retrieved strings are joined as a single string. This new string is then pushed to the list
using push() method.
arrow_forward
Part 5. Operator Overload:
add
Next, you will add the ability to use the addition operator (+) in conjunction with Simpy objects and floats.
You will implement
add
such that the left-hand side operand of an addition expression can be either a simpy object or a float value
using a Union type. The
add_method should return a new Simpy object and should not mutate the object the method is called on.
When the right-hand side of an addition expression is also a simpy object, you should assert that both objects' values attributes have equal
lengths. Then, you should produce a new simpy object where each item in its values attribute corresponds to the items of the original
Simpy objects at the same index added together. For example:
a = Simpy([1.0, 1.0, 1.0])
b = Simpy([2.0, 3.0, 4.0])
c = a + b
print(c)
# Output: Simpy([3.0, 4.0, 5.0])
When the right-hand side of an addition expression is a float value, you should produce a new simpy object where each item corresponds to
the item at the same…
arrow_forward
This is hard help please its from my study guide in C++ LEVEL 2
arrow_forward
Data Structures and algorithms:
Topic: Doubly and circular Linked Lists in java:
Please solve this on urgent basis:
Attach output's picture and explain every statement in commments:
Create a doubly Linked list, So far you have come across with numbers that are prime and you have a great knowledge about them using that knowledge help Alice solve the problem of finding all the numbers that are prime and collectively return the sum of those prime numbers stored in doubly linked list.
You can use more than 1 function to solve this problem.
For example
Linked List contains Data: 8,12,3,17,11,
Output: 31
Explanation: 3+17+11 = 31
arrow_forward
Assignment1: Analyse the case study given in chapter 3 Linked listAnswer the following questions from Library.java1. Write the different class names with class keyword
chapter 3 Linked list :
https://drive.google.com/file/d/1NRN_3Fx3smkjwv1jgG8hzaiGfnNfeeDb/view?usp=sharing
arrow_forward
Using C++ Programming :::: Simply Linked List
arrow_forward
Java (LinkedList) - Grocery Shopping List
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
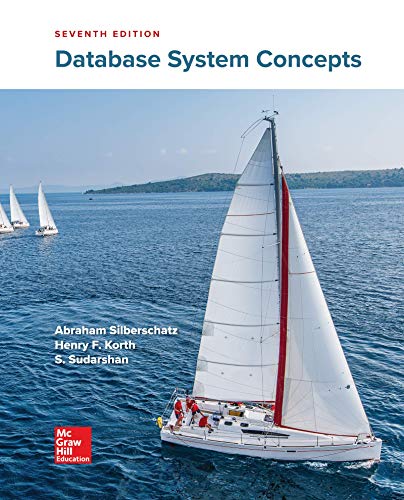
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
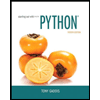
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
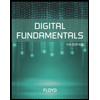
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
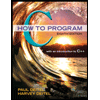
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
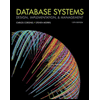
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
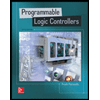
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- data structures in javaarrow_forwardExercise, maxCylinderVolume F# system function such as min or methods in the list module such as List.map are not allowed Write a function maxCylinderVolume that takes a list of floating-point tuples that represent dimensions of a cylinder and returns the volume of the cylinder that has the largest volume. Each tuple has two floating point values that are both greater than zero. The first value is the radius r and the second value is the height h. The volume of the cylinder is computed using ??2h. The value π is represented in F# with System.Math.PI. If the list is empty, return 0.0. Examples: > maxCylinderVolume [(2.1, 3.4); (4.7, 2.8); (0.9, 6.1); (3.2, 5.4)];;val it : float = 194.3137888> maxCylinderVolume [(0.33, 0.66)];;val it : float = 0.2257988304arrow_forwardIn C++ Write the DLList member function void DLList <T>:: RemoveAllDuplicatesOf (T& x) function, which delete all the occurrences of x (but one) in a sorted DLL. Example: If the current list is [5, 7, 7, 7, 10, 15, 25, 25]then calling:RemoveAllDuplicatesOf(7); will change the current list to [5, 7, 10, 15, 25, 25}arrow_forward
- Write a Python Code for the given constructor and conditions: Given Constructor: def __init__ (self, a) Pre-condition: Array cannot be empty. Post-condition: This is the default constructor of MyList class. This constructor creates a list from an array.arrow_forwardIn C++ Plz LAB: Grocery shopping list (linked list: inserting at the end of a list) Given main(), define an InsertAtEnd() member function in the ItemNode class that adds an element to the end of a linked list. DO NOT print the dummy head node. Ex. if the input is: 4 Kale Lettuce Carrots Peanuts where 4 is the number of items to be inserted; Kale, Lettuce, Carrots, Peanuts are the names of the items to be added at the end of the list. The output is: Kale Lettuce Carrots Peanuts ItemNode.h Default Code: #include <iostream>#include <string>using namespace std; class ItemNode {private: string item; ItemNode* nextNodeRef; public: // Constructor ItemNode() { item = ""; nextNodeRef = NULL; } // Constructor ItemNode(string itemInit) { this->item = itemInit; this->nextNodeRef = NULL; } // ConstructorItemNode(string itemInit, ItemNode *nextLoc) {this->item = itemInit;this->nextNodeRef = nextLoc;} // Insert node after this…arrow_forwardIn C++ Write the DLList member function bool DLLidt<T>::insert_before(int index, T& x) that is going to insert a node with the value of x before the node at position index in the list. Example: If the current list is [5, 6, 7, 8] insert_before(2,0); will change the current list to [5,6,0,7,8] **You need to use get_at function, and use it only one time to optimize the call of your code. ** Don’t forget to fix the next, and prev pointers for each node. ** for inserting before 0 index, you may use add_to_head function.arrow_forward
- Programming in C: Challenge 1: Create and Print a Doubly Linked List In class, I briefly mentioned how to create a doubly linked list (a linked list with two directions: forward and backwards). This task challenges you to create a doubly linked list of 5 nodes. If your nodes were called n1, n2, n3, n4 and n5 (which they shouldn’t, please give them original names), your list should be linked as follows: We discussed what "start" and "end" should be. Then, also in main, you will have to print the list forwards by taking advantage of the arrows pointing to the right. You will also have to print it backwards taking advantage of the arrows pointing to the left. Again, everything must be done in main. Hint: look at the way in which I printed the singly linked list in class. In other words, if your nodes had the values: n1=1, n2=2, n3=3, n4=4 and n5=5; your program should print:1 3 5 2 4 (forwards) and 4 2 5 3 1 (backwards). Please give different values to your nodes, they should not have…arrow_forwardC++ ProgrammingActivity: Deque Linked List Explain the flow of the code not necessarily every line, as long as you explain what the important parts of the code do. The code is already correct, just explain the flow. #include "deque.h" #include "linkedlist.h" #include <iostream> using namespace std; class DLLDeque : public Deque { DoublyLinkedList* list; public: DLLDeque() { list = new DoublyLinkedList(); } void addFirst(int e) { list->addAt(e,1); } void addLast(int e) { list->addAt(e,size()+1); } int removeFirst() { return list->removeAt(1); } int removeLast() { return list->removeAt(size()); } int size(){ return list->size(); } bool isEmpty() { return list->isEmpty(); } // OPTIONAL: a helper method to help you debug void print() {…arrow_forwarddata structures in javaarrow_forward
- Complete the code:- class MyList: # constructor def __init__(self, a): # converting array to list newList = a.tolist() # printing the list print("List is:", newList).arrow_forwardfor c++ please thank you please type the code so i can copy and paste easily thanks. 4, List search Modify the linked listv class you created in the previous programming challange to include a member function named search that returns the position of a specific value, x, in the lined list. the first node in the list is at position 0, the second node is at position 1, and so on. if x is not found on the list, the search should return -1 test the new member function using an approprate driver program. here is my previous programming challange. #include <iostream> using namespace std; struct node { int data; node *next; }; class list { private: node *head,*tail; public: list() { head = NULL; tail = NULL; } ~list() { } void append() { int value; cout<<"Enter the value to append: "; cin>>value; node *temp=new node; node *s; temp->data = value; temp->next = NULL; s = head; if(head==NULL) head=temp; else { while (s->next != NULL) s = s->next; s->next =…arrow_forwardRead this: Complete the code in Visual Studio using C++ Programming Language with 1 file or clear answer!! - Give me the answer in Visual Studio so I can copy*** - Separate the files such as .cpp, .h, or .main if any! Develop a C++ "doubly" linked list class of your own that can hold a series of signed shorts Develop the following functionality: Develop a linked list node struct/class You can use it as a subclass like in the book (Class contained inside a class) You can use it as its own separate class Your choice Maintain a private pointer to a node class pointer (head) Constructor Initialize head pointer to null Destructor Make sure to properly delete every node in your linked list push_front(value) Insert the value at the front of the linked list pop_front() Remove the node at the front of the linked list If empty, this is a no operation operator << Display the contents of the linked list just like you would print a character…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
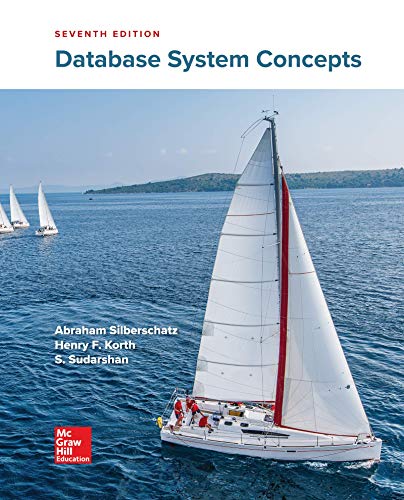
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
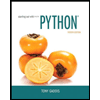
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
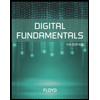
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
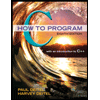
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
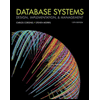
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
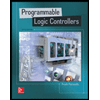
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education