WS09-OOP345
.txt
keyboard_arrow_up
School
Seneca College *
*We aren’t endorsed by this school
Course
OOP345
Subject
Computer Science
Date
Feb 20, 2024
Type
txt
Pages
5
Uploaded by BailiffInternetHyena31
Workshop 9: Multi-Threading
You calculate average of the values stored in files, and use multi-threading to read data from the files and to compute averages of the read data.
Learning Outcomes
Upon successful completion of this workshop, you will have demonstrated the abilities to
process partitioned data on two or more threads
write a set of numbers to a file in binary mode
read a set of numbers from a file in binary mode
bind a function to its arguments
Submission Policy
The workshop is divided into two coding parts and one non-coding part:
Part 1: worth 0% of the workshop's total mark, is optional and designed to assist you in completing the second part.
Part 2: worth 100% of the workshop's total mark, is due on Sunday at 23:59:59 of the week of your scheduled lab. Submissions of Part 2 that do not contain the reflection are not considered valid submissions and are ignored.
reflection: non-coding part, to be submitted together with Part 2. The reflection does not have marks associated to it, but can incur a penalty of max 40% of the whole workshop's mark if your professor deems it insufficient (you make your marks from the code, but you can lose some on the reflection).
The workshop should contain only work done by you this term or provided by your professor. Work done in another term (by you or somebody else), or work done by somebody else and not clearly identified/cited is considered plagiarism, in violation of the Academic Integrity Policy.
Every file that you submit must contain (as a comment) at the top your name, your Seneca email, Seneca Student ID and the date when you completed the work.
If the file contains only your work, or work provided to you by your professor, add
the following message as a comment at the top of the file:
I have done all the coding by myself and only copied the code that my professor provided to complete my workshops and assignments.
If the file contains work that is not yours (you found it online or somebody provided it to you), write exactly which parts of the assignment are given to you as help, who gave it to you, or which source you received it from. By doing this you will only lose the mark for the parts you got help for, and the person helping you will be clear of any wrong doing.
Compiling and Testing Your Program
All your code should be compiled using this command on matrix:
/usr/local/gcc/10.2.0/bin/g++ -Wall -pthread -std=c++17 -g -o ws file1.cpp file2.cpp ...
-Wall: compiler will report all warnings
-std=c++17: the code will be compiled using the C++17 standard
-g: the executable file will contain debugging symbols, allowing valgrind to create
better reports
-o ws: the compiled application will be named ws
After compiling and testing your code, run your program as following to check for possible memory leaks (assuming your executable name is ws):
valgrind ws
To check the output, use a program that can compare text files. Search online for
such a program for your platform, or use diff available on matrix.
Part 1 (0%)
The three source files provided for this workshop are
process_data.h -- fully implemented, do not modify
process_data.cpp -- partially implemented,
w9_p1.cpp -- already implemented, do not modify
A data file provided for this workshop is
data_int.dat -- First 4 bytes (int size) in the file contains the total number of data items. The remaining bytes in the file contain the data-item values, where each data-item is of 4 byte (int size).
The computeAvgFactor and computeVarVactor functions, implemented in process_data.cpp, compute the average-factor and variance-factor from the data supplied in the first parameter. These functions are described as comments in the process_data.cpp file, read carefully and understand. These functions are fully implemented, do not change them.
The ProcessData constructor function, in the file process_data.cpp receives a filename as std::string, opens the file in binary mode and reads the total number of data items (first 4 bytes) followed by the data-item values. Detailed description is included as comment in process_data.cpp, read carefully and understand.
Your first task for part 1 of this workshop is to complete the definition of the constructor in class ProcessData to load the data from the binary file whose name is received as a parameter. See above info about the structure of the file. Do not modify existing code in this function, only add what is missing.
Your second task is to write definition of the operator() function in process_data.cpp. Prototype of this function is in the header file process_data.h. The first parameter of this function receives target-filename to which the data is to be written in binary format. The second parameter is a double reference variable
for holding computed average value. The third parameter is double reference variable for holding computed variance value.
You compute the average value by calling computeAvgFactor with the following parameters: data, total_items as the size of the array, and again total_items as divisor. The fourth argument is going to be a variable in which the computed average value is to be stored.
You compute variance value by calling computeVarFactor with first, second and third
arguments same as before, i.e., in the case of computeAvgFactor. The fourth argument is the average value computed by computeAvgFactor and fifth argument is the variable in which the computed variance is to be stored.
You open the target data file and write the data (total_items, and data) in the format of the input data file data_int.dat.
w9_p1 Module (supplied)
The tester module has been supplied. Do not modify the existing code!
When doing the workshop, you are encouraged to write your own tests, focusing on a single implemented feature at the time, until you get all functionality in place.
Sample Output
When the program is started with the command (the input file is provided):
ws data_int.dat data_int_target.dat
the output should look like the one from the sample_output.txt file.
Test Your Code
To test the execution of your program, use the same data as shown in the output example above.
Upload your source code to your matrix account. Compile and run your code using the
latest version of the g++ compiler (available at /usr/local/gcc/10.2.0/bin/g++) and
make sure that everything works properly.
Then, run the following command from your account (replace profname.proflastname with your professor’s Seneca userid):
~profname.proflastname/submit 345_w9_p1
and follow the instructions.
This part represents a milestone in completing the workshop and is not marked!
Part 2 (100%)
Your second task is to extend your work in part 1 to include multi-threaded logic for calculating average and variance of the data read from the file.
The following enhancements have already been made in the process_data module for handling the multi-threaded computation:
class definition for ProcessData has been enhanced by including member variables for handling the multi-threaded computation.
The constructor definition for ProcessData has been enhanced for handling the muli-
threaded computation.
The destructor has been enhanced
Overloaded bool operator function has also been enhanced
Your job is to enhance the operator() function you created in part 1 for multi-
threaded computation. Specifically, you include:
Computation of average through multi-threading: to compute average, you call the function computeAvgFactor in different threads with different parts of the data items divided by partition-indices.
bind total_items to the function computeAvgFactor as the divisor parameter.
create threads by passing the following arguments to the function: address of the first element of each partition as arr, number of elements in the partition as size, and an element of the resource variable created to hold average-factors as avg.
join the threads.
add all the average-factors into the variable to hold total average; this shold be one of the parameters of the operator() function.
Computation of variance through multi-threading: to compute variance, you call the function computeVarFactor in different threads with different parts of the data items divided by partition-indices. You follow a procedure similar to the one you followed while computing the average, with following exceptions:
use the average value computed in the previous step as the 4th parameter.
bind total_items and the computed average as divisor and avg, respectively, to the function ,before creating the threads.
the last parameter should be an element of the resource variable created to store variance-factors.
you add the variance-factors computed by threads to compute the total variance.
You open the target data file and write the data (total_items, and data) in the format of the input data file data_int.dat.
Your code must be free from memory leak.
When binding an argument to a function, consider the following reference:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
File System: It is highly useful in file system handling where for example
the file allocation table contains a sequential list of locations where the files
is split up and stored on a disk. Remember that overtime it is hard for an
OS to find disk space to cover the entire file so it usually splits these up into
chunks across the physical hard drive and stores a sequential list of links
together as a linked list.
Write an algorithm for the above problem and analyse the efficiency of
the algorithm.
arrow_forward
C++
You must create a header file for each task with implementation as the execute task.
Input file A contains numeric values and the Output File A is sorted.
Algorithm:
a ← read Value from file (A);
while not End Of File (A) {
b ← read Value from file (A);
If a> b {
return false;
}
a ← b;
}
return true;
arrow_forward
createDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles.
2. Reads the profiles from the input file.
3. For each person in the file
1. creates a Profile object with the information from file (see input file format below).
2. insert the newly created profile into the next position in the database array (instance variable).
arrow_forward
In python
arrow_forward
Challenge Activity 1: Word List File Writer and Reader
(a)Write a console and GUI program using *PYTHON* that asks the user how many words they would like to write to a file, and then asks the user to enter that many words, one at a time. The words should be written to a file.
(b) Write another program that reads the words from the file and displays the following data:
The number of words in the file.
The longest word in the file.
The average length of all of the words in the file.
arrow_forward
debug
arrow_forward
Local addresses
The "A1" address system has coordinates that exist over the whole worksheet. If you have a block of data specified somewhere within that worksheet, it can be useful to be able to specify the addresses relative to that block.
This can be done with INDEX(), which takes 3 arguments. The first argument is a rectangular range of data, for example A2:E8. The second and third arguments are numbers specifying an offset down then right from the top left of that data range. Unlike OFFSET(), the numbering starts at 1, so INDEX(A2:E8, 4, 2) refers to cell B5.
Instructions
Use the block of data for North East India + North Myanmar, from A11 to G19 as the reference.
In cell I1, use INDEX() to get the number of Blues in Sikkim.
In cell I2, get the number of White-yellows in Mizoram Hills.
please show the formulas in excel thank you :)
Area
Locality
Skipper
Swallowtail
White-yellow
Blue
Brush-footed
Blues in Sikkim
Indian Subcontinent
Indian Subcontinent
307
94
99…
arrow_forward
INSTRUCTION: C++ Language Create a function that writes to a file named Inventy.txt the information of 8 items. Ask the user for the item's name, the item's quantity in storage, and the item's price and write it to the file. Create a function that reads the file and items' quantity and price to two different arrays. Create a function that computes the subtotal of each item. Implement all functions inside the main program and the computed value should be displayed with the item's name.
arrow_forward
9act1 Please help me answer this in python programming.
arrow_forward
java code
Create a program that allows a user to input customer records (ID number, first name, last name, and balance owed) and save each record to a file.
arrow_forward
python
arrow_forward
File Attributes
5. File attributes
(a) File name: file name is a very important file attribute When a file changes name, it seems to change its
identity (just like when you change your name)
The oldest file naming we may remember is the 8 +3 convention in DOS (in DOS, all file names can NOT be
bigger than 8 characters, special characters like spaces were not allowed etc.). So a file like C:\\Program
Files\\Adobe\\Photoshop can cause problems in DOS, and it has to be represented in different ways using strange
character like~ (tilde).
Elaborate on your understanding of file names or file naming in different operating systems (Windows, Mac,
Linux etc.). For example, some OS like NTFS may allow longer file name, allow space, and even allow non
English like characters such as Chinese file names. Is there a maximum length of file names (can you have a file
name of 1,000 characters long?)
(b) File creation date and modification date. File can be created and modified using different ways of…
arrow_forward
PSC
Oct 13
waynekizzo
7:20
X
2
arrow_forward
POWERSHELL TASK
Try to swap two strings/names in a file and the file name should be a command-line argument.
arrow_forward
C programming language
Criteria graded:
Declare file pointers Open file Read from file Write to file Close file
Instructions:
Write a segment of code that opens a file called “numbers.txt” for reading. This file is known to have 10 numbers in it. Read in each number and print a ‘*’ to the screen if the number is even. If the number is odd, do nothing. Conclude by closing this file.
arrow_forward
Introduction:It is often necessary to reformat data in files. Sometimes it is done for readability, sometimes it is done to fit as input to different programs.
Purpose:The purpose of the task is to provide proficiency in simple text management and file management.The task is the basis for file management.
Reading instructions:You should be done with all the steps up to and including file management.
Implementation:
You will create a standalone program that performs the tasks below. Start by solving task one and complete that task before starting task 2.
Your program should read the files mentioned from the "current working directory".All files are in text format with "\ n" at the end of the line. If you wish, you can use pipes and redirects to solve the file reading and printing instead of std :: ifstream and std :: ofstream.Comment on it in the code along with examples of how the program is used.
The file names.txt contains names and social security numbers in the following form. The…
arrow_forward
Python
arrow_forward
Assessment Description
Honors Students: Complete this assignment according to the directions provided in the "Honors Addendum," located in Class Resources.
This activity has multiple parts. All parts must be completed prior to documentation submission.
Part 1: Reading and Writing Text Files.
In this activity, you will learn how to read and write text files using non buffered and buffered File I/O classes, then learn how to parse a String into tokens using the String split() method, and finally, how to handle exceptions using various approaches.
Part 2: Reading and Writing JSON Files.
In this activity, you will learn how to read, write, and serialize JSON files to and from Java Objects.
Refer to the instructions within "Activity 4 Guide" to complete the assignment.
APA style is not required, but solid academic writing is expected.
This assignment uses a rubric. Please review the rubric prior to beginning the assignment to become familiar with the expectations for successful…
arrow_forward
exampleOne
This program reads data from a physical file, create a reference to the file, perform a computation, display the result, close the file.
package chario;
import java.util.Scanner;
import java.io.*;
//public class Chario {
// public static void main(String[] args) throws IOException
{
File file1 = new File("fileOne.txt"); //Create a reference to the physical file
/*
Use Notepad to create a text file named fileOne.txt
which must be in the same directory that src is in
To find src, open your project in NetBeans and hover its name, which is in
the top left just below the Projects menu; this will display the path to your project
My project path is, yours will be different
I:\\Ajava\161\WPPractice\IO\charstream\chario
My fileOne.txt is located in chario
*/
Scanner getit = new Scanner( file1 );
// connect a Scanner to the file int num, square;
num = getit.nextInt();
square = num * num ;
System.out.println("The square of " + num + " is " + square);
getit.close(); //Close the stream…
arrow_forward
in C# Code in VS studio:
Create a program that has 2 options for a user to select.
The first option accepts information from the user and then save that data to a file on disk.
The second option loads all data stored on that file.
arrow_forward
C#
Write, compile, and test a console application named FileComparison that compares two files. First, use a text editor such as Notepad to save your favorite movie quote. Next, copy the file contents, and paste them into a word-processing program such as Word. Then, write the file-comparison application that displays the sizes of the two files as well as the ratio of their sizes to each other. To discover a file's size, you can create a System.IO.FileInfo object using statements such as the following, where FILE_NAME is a string that contains the name of the file, and size has been declared as an integer:
FileInfo fileInfo = new FileInfo(FILE_NAME) ;Size = fileInfo.Length;
arrow_forward
Disc Scheduling FCFS, SCAN or C-SCAN
Write a program that will calculate the total head movement for either one of this algorithms (FCFS, SCAN or C-SCAN) for all test cases
Your input file starts with the case number, number of tracks, starting track, and the sequence of track numbers it will be accessing//input fileCase 1, 199, 53, 98, 183, 37, 122, 14, 124, 65, 67Case 2, 199, 53, 98, 183, 37, 122, 14, 124, 65, 68Case 3, 199, 53, 98, 183, 37, 122, 14, 124, 65, 69Case 4, 199, 53, 98, 183, 37, 122, 14, 124, 65, 70
Remember the track starts at 0
A sample output file will look like the following. FCFS algorithmCase 1, 640Case 2, 641Case 3, 642Case 4, 643
arrow_forward
C# please
Write a program that will create file with at least 10 lines. Each line of your file should have information delimited by some delimiter (I.e., commas, spaces, slashes, symbols, etc.). After your file has been created with the proper amount of data, you should read your file line by line and display without the delimiters in the same program after closing your writer. Additionally, the information stored into your file should be data that is gathered from a separate class.
Your program should save your file in a specific directory. To promote flexibility, your program should check to see if the chosen directory exists prior creating it, and if it does not exist, your program should create it for you. Additionally, you should allow the user to enter the names of the directory and file instead of hard-coding it into your program.
arrow_forward
Question 21 of 25
When writing to a file, numbers must be explicitly converted to strings.
O True
O False
Question 22 of 25
The 'is' and ==' operations do different things.
OTrue
O False
Question 23 of 25
In the class definition: class MyClass(x.y):
Ox and y are initial values of MyClass instance attributes
Ox and y are base classes that MyClass will inherit from
Question 24 of 25
Python can execute multiple instructions simultaneously by using threads.
OTrue
O False
Question 25 of 25
If x=y appears in a function ((y is not declared global) that is the order of
namespaces that Python searches to find y?
O built-ins, globals, locals
O built-ins, globals, locals, enclosing
O locals, enclosing, globals, built-ins
O locals, globals, built-ins, enclosing
arrow_forward
* Question Completion Status:
A Moving to another question will save this response.
Question 1
Run-time binding is useful for saving on memory because :
It is static linking
Only load modules when they're needed.
Ob.
Don't allow several processes to share one copy of a module.
modules cannot be loaded
d.
A Moving to another question will save this response.
O
DELL
arrow_forward
C++
arrow_forward
JAVA PPROGRAM
Write a program that prompts the user to enter a file name, then opens the file in text mode and reads names. The file contains one name on each line. The program then compares each name with the name that is at the end of the file in a symmetrical position. For example if the file contains 10 names, the name #1 is compared with name #10, name #2 is compared with name #9, and so on. If you find matches you should print the name and the line numbers where the match was found.
While entering the file name, the program should allow the user to type quit to exit the program.
If the file with a given name does not exist, then display a message and allow the user to re-enter the file name.
The file may contain up to 100 names.
You can use an array or ArrayList object of your choosing, however you can only have one array or ArrayList.
Input validation:
a) If the file does not exist, then you should display a message "File 'somefile.txt' is not found." and allow the…
arrow_forward
python function that creates and saves data in a file. the saved data represents exam grades. in the function , you will create n random numbers in the range 1-100, where n is the number of students. The function can be called as follows: createFile(filename, n)
main function, in which the user inputs the file name and the number of students, then the main calls function createfile. the main should preform validation for n (should be > 0), and the filename(should end with .txt)
arrow_forward
FILE HANDLING
please attach sourcecode/c language program
arrow_forward
Please choose the correct answer without exsplaining.. Thank you
arrow_forward
MICIOSoft WOrd
37617da64 Major Assignments
Review
View
ngs
三,三、 |情
AaBbCcD AaBbCcDc AaBbC AaBbCc AABI AgBbCcl
I Normal
11 No Spaci. Heading 1
Heading 2
Title
Subtitle
Styles
Paragraph
This C++ programs on BANKING SYSTEM has account class with data
members like account number, name.deposit, withdraw amount and type of
account. Customer data is stored in a binary file. A customer can deposite and
withdraw amount in his account. User can create, modify and delete account. In
this banking system project, Do not used graphics to keep program simple.
MAIN MENU
01. NEU ACCOUNT
82. DEPOS II AMOUNT
BANK
83. VITHDRAU AMOUNT
84. BALANCE ENQUIRY
MANAGEMENT
05. ALL ACCOUNT HOLDER LIST
86. CLOSE AN ACCOUNT
SYSTEM
87. MODIFY AN ACCOUNT
88. EXIT
Select Your Opt ion (1-8> -
-NEV ACCOUNT ENIRY FORM---
ACCOUNT TRANSCATI ON FORM---
Enter The account Nunber : 186
Enter The account No.: 106
Enter The Nane of The account Holder : SHREYA
Enter Type of The account (C/S) I S
---ACCOUNT STATUS---
Enter Initial…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
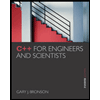
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
Related Questions
- File System: It is highly useful in file system handling where for example the file allocation table contains a sequential list of locations where the files is split up and stored on a disk. Remember that overtime it is hard for an OS to find disk space to cover the entire file so it usually splits these up into chunks across the physical hard drive and stores a sequential list of links together as a linked list. Write an algorithm for the above problem and analyse the efficiency of the algorithm.arrow_forwardC++ You must create a header file for each task with implementation as the execute task. Input file A contains numeric values and the Output File A is sorted. Algorithm: a ← read Value from file (A); while not End Of File (A) { b ← read Value from file (A); If a> b { return false; } a ← b; } return true;arrow_forwardcreateDatabaseOfProfiles(String filename) This method creates and populates the database array with the profiles from the input file (profile.txt) filename parameter. Each profile includes a persons' name and two DNA sequences. 1. Reads the number of profiles from the input file AND create the database array to hold that number profiles. 2. Reads the profiles from the input file. 3. For each person in the file 1. creates a Profile object with the information from file (see input file format below). 2. insert the newly created profile into the next position in the database array (instance variable).arrow_forward
- In pythonarrow_forwardChallenge Activity 1: Word List File Writer and Reader (a)Write a console and GUI program using *PYTHON* that asks the user how many words they would like to write to a file, and then asks the user to enter that many words, one at a time. The words should be written to a file. (b) Write another program that reads the words from the file and displays the following data: The number of words in the file. The longest word in the file. The average length of all of the words in the file.arrow_forwarddebugarrow_forward
- Local addresses The "A1" address system has coordinates that exist over the whole worksheet. If you have a block of data specified somewhere within that worksheet, it can be useful to be able to specify the addresses relative to that block. This can be done with INDEX(), which takes 3 arguments. The first argument is a rectangular range of data, for example A2:E8. The second and third arguments are numbers specifying an offset down then right from the top left of that data range. Unlike OFFSET(), the numbering starts at 1, so INDEX(A2:E8, 4, 2) refers to cell B5. Instructions Use the block of data for North East India + North Myanmar, from A11 to G19 as the reference. In cell I1, use INDEX() to get the number of Blues in Sikkim. In cell I2, get the number of White-yellows in Mizoram Hills. please show the formulas in excel thank you :) Area Locality Skipper Swallowtail White-yellow Blue Brush-footed Blues in Sikkim Indian Subcontinent Indian Subcontinent 307 94 99…arrow_forwardINSTRUCTION: C++ Language Create a function that writes to a file named Inventy.txt the information of 8 items. Ask the user for the item's name, the item's quantity in storage, and the item's price and write it to the file. Create a function that reads the file and items' quantity and price to two different arrays. Create a function that computes the subtotal of each item. Implement all functions inside the main program and the computed value should be displayed with the item's name.arrow_forward9act1 Please help me answer this in python programming.arrow_forward
- java code Create a program that allows a user to input customer records (ID number, first name, last name, and balance owed) and save each record to a file.arrow_forwardpythonarrow_forwardFile Attributes 5. File attributes (a) File name: file name is a very important file attribute When a file changes name, it seems to change its identity (just like when you change your name) The oldest file naming we may remember is the 8 +3 convention in DOS (in DOS, all file names can NOT be bigger than 8 characters, special characters like spaces were not allowed etc.). So a file like C:\\Program Files\\Adobe\\Photoshop can cause problems in DOS, and it has to be represented in different ways using strange character like~ (tilde). Elaborate on your understanding of file names or file naming in different operating systems (Windows, Mac, Linux etc.). For example, some OS like NTFS may allow longer file name, allow space, and even allow non English like characters such as Chinese file names. Is there a maximum length of file names (can you have a file name of 1,000 characters long?) (b) File creation date and modification date. File can be created and modified using different ways of…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- C++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology Ptr
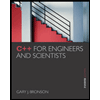
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr