exercise_assignment_2
.py
keyboard_arrow_up
School
Mesa Community College *
*We aren’t endorsed by this school
Course
156
Subject
Computer Science
Date
Dec 6, 2023
Type
py
Pages
2
Uploaded by Jo_Bird
# EA 2
# Jodyleeann Kalulu
# CHAPTER 5
# 1. Create a variable named num_1 and assign 14 to it (1 pt.).
num_1 = 14
# 2. Create a variable named num_2 and assign 79 to it (1 pt.).
num_2 = 79
# 3. Add num_1 to num_2 and store it in ans_one, print ans_one (2 pts.).
ans_one = num_1 + num_2
print(ans_one)
# 4. Subtract num_1 from num_2 and store it in ans_two, print ans_two (2 pts.).
ans_two = num_1 - num_2
print(ans_two)
# 5. Multiply num_1 by num_2 and store it in ans_three, print ans_three (2 pts.).
ans_three = num_1 * num_2
print(ans_three)
# 6. Divide num_2 by num_1 and store it in ans_four, print ans_four (2 pts.).
ans_four = num_2 / num_1
print(ans_four)
# 7. Use the integer division operator to divide num_2 by num_1 and store it in
ans_five, print ans_five (2 pts.).
ans_five = num_2 // num_1
print(ans_five)
# 8. Return the remainder of num_2 divided by num_1 and store it in ans_six, print
ans_six (2 pts.).
ans_six = num_2 % num_1
print(ans_six)
# 9. Use the is_integer method to check if num is an integer and print the value
returned by the method (2 pts.).
num = 15.6 # DO NOT DELETE OR MODIFY THIS LINE OF CODE
print(num.is_integer())
# 10. Use the pow function to print out 5 raised to the power of -3 (1 pt.).
print(pow(5, -3))
# 11. Print num_3 so that 3 decimal places are displayed and it is grouped by
thousands (1 pt.).
num_3 = 987654321.123456789 # DO NOT DELETE OR MODIFY THIS LINE OF CODE
print(round(num_3 , 3))
# 12. Write a print statement using an f-string that prints out the following
sentence using the variables defined below.
# In 2017, New Zealand had a population of 4,793,700 and a life expectancy of 71.61
years. The round function
# should not be used (2 pts.).
year = 2017 # DO NOT DELETE OR MODIFY THIS LINE OF CODE
population = 4793700 # DO NOT DELETE OR MODIFY THIS LINE OF CODE
expectancy = 71.6143 # DO NOT DELETE OR MODIFY THIS LINE OF CODE
print(f"In {year}, New Zealand had a population of {population:,} and a life
expectancy of {expectancy:.2f} years.")
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Program in c++ language
arrow_forward
Instructions:
In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and a character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main(), there are two Persons already created: one Male Person and one Female Person.
Your task is to first ask the user for the age of the Male Person and the age of the Female Person.
Then, define and declare a function called createKidPerson() which has the following definition:
Return type - Person
Name - createKidPerson
Parameters
Person father - the father of the kid to be created
Person mother - the mother of the kid to be created
Description - creates a new Person and returns this. The age of this Person will be set to 1 while its gender will be set based on the rules mentioned above.
Finally, create a new Person and call this createKidPerson() in the main and then pass this newly…
arrow_forward
Function #2: largest Parameters: num1 - a floating point number being compared against num2 and num3 num2 - a floating point number being compared against num1 and num3 num3 - a floating point number being compared against num1 and num2 Description: Write a function that takes in three numbers as parameters and prints out the largest of the three. If two or all of the numbers are equal, or even all three, then just print the value once. Test Cases: >>>largest(433.1, 2340.32, 12323.7) 12323.7 >>>largest(12.0, 32.1, 32.1) 32.1 >>>largest(23.44, 23.44, 23.44) 23.44
arrow_forward
Food Functions - How do I do this practice exercise using Swift code?
arrow_forward
Q3-
What is the memory content for the following data section variables?
L1 db OAAh, 0BBH
L2 dw OCCDDH
L3 db 0EEh, OFFH
L1
L2
L3
arrow_forward
1 # SuperMarket.py - This program creates a report that lists weekly hours work
The student file provided for this lab includes the necessary variable declarations and input and
2 # by employees of a supermarket. The report lists total hours for
output statements. You need to implement the code that recognizes when a control break should
3 # each day of one week.
occur. You also need to complete the control break code. Be sure to accumulate the daily totals
4 # Input: Interactive
5 # Output: Report.
for all days in the week.
6.
7 # Declare variables.
Comments in the code tell you where to write your code.
8 HEAD1 = "WEEKLY HOURS WORKED"
9 DAY_FOOTER = "Day Total "
10 SENTINEL = "done"
Instructions
# Named constant for sentinel value
11 hoursWorked = 0
# Current record hours
12 hoursTotal = 0
# Hours total for a day
1. Study the prewritten code to understand what has already been done.
|13 prevDay = ""
|14 notDone = True
# Previous day of week
2. Write the control break code
# loop…
arrow_forward
Assume that grade is a variable whose value is a letter student_grade-- any one of the following letters:
'A', 'B', 'C', 'D', 'F". Assume further that there are the
following int variables, declared and already initialized: acount, bcount, ccount, dcount, fcount.
Write a switch statement that increments the appropriate variable (acount, bcount, ccount, etc.) depending
on the value of grade. So if grade is 'A' then acount is incremented; if grade is'B'
then bcount is incremented, and so on.
arrow_forward
printTodaysDate is a function that accepts no parameters and returns no value.
Write a statement that calls printTodaysDate.
arrow_forward
5act2 Please help me code in python programming.
arrow_forward
Summary
2 HouseSign.py - This program calculates prices for cu
house signs.
In this lab, you complete a prewritten Python program for a carpenter who creates personalized
house signs. The program is supposed to compute the price of any sign a customer orders, based
4
on the following facts:
5 # Declare and initialize variables here.
6
# Charge for this sign.
• The charge for all signs is a minimum of $35.00.
# Number of characters.
8
# Color of characters.
• The first five letters or numbers are included in the minimum charge; there is a $4 charge for
9
# Type of wood.
10
each additional character.
11 # Write assignment and if statements here as appropr
• If the sign is make of oak, add $20.00. No charge is added for pine.
12
13 # Output Charge for this sign.
• Black or white characters are included in the minimum charge; there is an additional $15
14 print("The charge for this sign is $" + str(charge))
charge for gold-leaf lettering.
15
Instructions
1. Make sure the file HouseSign.py…
arrow_forward
add is a function that accepts two int parameters and returns their sum.
Two int variables, euroSales and asiaSales, have already been declared and initialized. Another int variable, eurasiaSales, has already been declared.
Write a statement that calls add to compute the sum of euroSales and asiaSales and store this value in eurasiaSales.
arrow_forward
Usage: mortgagepmt [-s] -r rate [-d downpayment] price
In this assignment, you are asked to do a mortgage payment calculation. All information needed for this will be passed to the program on the command line. There will be no user input during the execution of the program.
You will need a few pieces of information. The price of the home and the amount of the down payment. You will also need to know the interest rate and the term of the mortgage. To figure your mortgage payment, start by converting your annual interest rate to a monthly interest rate by dividing by 12. Next, add 1 to the monthly rate. Third, multiply the number of years in the term of the mortgage by 12 to calculate the number of monthly payments you’ll make. Fourth, raise the result of 1 plus the monthly rate to the negative power of the number of monthly payments you’ll make. Fifth, subtract that result from 1. Sixth, divide the monthly rate by the result. Last, multiply the result by the amount you want to borrow.…
arrow_forward
Code it fast
Screenshot attach for confirmation
arrow_forward
3.
Which among the following shows a valid use of the Direction enumeration as a parameter to the moveCharacter function? Select al that apply.
enum Direction { case north, south, west, east}func moveCharacter(x: Int, y: Int, facing: Direction) {// code here}
moveCharacter(x: 0, y: 0, facing: .southwest)
moveCharacter(x: 0, y: 0, facing: Direction.north)
moveCharacter(x: 0, y: 0, facing: .south)
moveCharacter(x: 0, y: 0, facing: Direction.northeast)
arrow_forward
Write a set of functions that calculate the cost of movie tickets for a family. These functions should use a pass-by-reference variable that accesses the double running_total variable in main().
Using a loop, keep displaying the ticket prices for each age group:
Something like:
Model Hall Movie Ticket Box Office
Under Age 3 (free)
Ages 3-11 ($10.25)
Ages 12-54 ($15.50)
Age 55 or older ($12)
If 4 tickets are purchased (not counting under age 3) then 20% discount
Within the loop:
Keep asking the user to input the moviegoer age and to enter a zero for the age when they are finished inputting all the family members.
Also, within the loop:
Keep a running_total that is output to the screenafter each age has been entered.
This running_total should be accessed through the functions using apass-by-reference variable.
Finally, after the looping is done:
Calculate the total cost, including a 7.5% sales tax, and output it to the screen.
arrow_forward
8.
Which among the following show a valid use of the Color enumeration as a parameter to the setPixel function? Select all that apply. Take note that this is a right minus wrong question.
enum Color { case red, green, blue}func setPixel(x: Int, y: Int, color: Color) {// code here}
Which among the following show a valid use of the Color enumeration as a parameter to the setPixel function? Select all that apply. Take note that this is a right minus wrong question.
enum Color { case red, green, blue}func setPixel(x: Int, y: Int, color: Color) {// code here}
1. setPixel(x: 0, y: 0, color: .blue)
2. setPixel(x: 0, y: 0, color: .orange)
3. setPixel(x: 0, y: 0, color: Color.green)
4. setPixel(x: 0, y: 0, color: Color.yellow)
arrow_forward
assign(self, assignment:Assignment) -> AssignmentResult:
"""
This function is to simulate the process of the student receiving an assignment, then working on the
assignment, then submitting the assignment and finally receiving grade for the assignment. This function will
receive an assignment then a grade should be calculated using the following formula:
grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0)
After the grade is calculated the student's energy should be decreased by percentage difficulty.
Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy
should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0)
Finally the grade calculated should be stored internally with in this class so it can be retrieved later.
Then an Assignment Result object should be created with the student's ID, the assignment…
arrow_forward
SwapPoints
Objective: To write two missing functions that manipulate objects, to complete a given program.
I recommend you start early on this and all programming assignments. They are an important part of your grade, and they use material from the notes and exercises we did in the previous module. (They don't relate to this module's notes or exercise.)
So your job is to write the two functions below, which are missing from the program:
inputNewPlayerLocation: to input (x,y) coordinates from user (using Scanner), then return a Point object with those coordinates.
swapPoints: to swap the coordinates of two Point objects. (Hint: To keep the main function's reference variables relevant, you can't swap Point objects entirely. You need to change the x and y coordinates inside the Point objects.)
arrow_forward
Write a function named validate_variable_name() that asks the user to enter a variable name as a parameter as in the examples below. It then
checks whether the variable name entered is a valid variable name based on the following rules:
• A variable name can only have lowercase letters, digits and underscores.
• A variable name cannot start with a number.
A variable name cannot be any of the following keywords: 'False', 'None', 'True', 'and', 'as', 'assert', 'async', 'await', 'break', 'class', 'continue', 'def', 'del',
'elif', 'else', 'except', 'finally', 'for', 'from', 'global', 'if', 'import', 'in', 'is', 'lambda', 'nonlocal', 'not', 'or', 'pass', 'raise', 'return', 'try', 'while', 'with', 'yield'
If the variable name breaks any of the above rules, then the function should print the message "This variable name is not valid." If it satisfies all the
conditions then the function should print the message "This variable name is valid."
For example:
Test
Input
Result…
arrow_forward
/ create the following functions:
GetInteger - returns an integer DONE
GetDouble - returns a double
CheckInteger (called from GetInteger) - checks the range of an integer
CheckDouble(called from GetDouble) - checks the range of a doubleCalculateSum - overloaded for integers and doubles as parameters DONE
CalculateProduct - overloaded for integers and doubles as parameters DONE
ShowSums - shows the sums of integers and the sum of doubles
ShowProducts - shows the products of integers and the product of doubles.
if you can create a function ShowInstructions that passes the type (integer or double) as a string. ShowInstructionsIntegers and ShowInstructionsDoubles
arrow_forward
Create a function called getHex() as follows:
• This function should return a value of type char and have a
parameter of type int.
• The function should use either an iflelse or a switch to return
the corresponding hex value of the number as a char value.
o If the number is between 0 and 9, add '0' to the
parameter.
o If the number is between 10 and 15, a local char variable
should be set equal to the correct letter ('A', 'B', 'C', 'D',
'E', or 'F').
arrow_forward
1- Write a program that prompts the user to enter 5 integers and performs the following:
i- The program should have a function named add, which would take 5
integers as parameters and print the sum of those numbers.
ii- The program should have a function named product, which would take 5
integers as parameters and print the product of those numbers.
iii- The program should have a function named average, which would take 5
integers as parameters and print the average of those numbers.
iv- The program should have a function named subtract, which would take 5
integers as parameters and print the subtraction of those numbers.
After taking five integers as input from user, your program should as user whether he/she wants to add, subtract, multiply or calculate the average. You could follow a similar pattern:
1 – Press 1 to perform addition.2 – Press 2 to perform multiplication. 3 – Press 3 to perform subtraction.4 – Press 4 to calculate average5 – Press 5 to exit…
arrow_forward
Write a function named getNumber, which uses a reference parameter to accept an integer argument. The function should prompt the user to enter a number in the range of 1 through 100. The input should be validated and stored in the parameter variable.
arrow_forward
This is Final Warning ⚠️ Don't post AI generated answer or plagiarised answer. If I see these things I'll give you multiple downvotes and will report immediately.
arrow_forward
Write a program that:
Defines an enum named Classification. The enum Classification will have 2 data values: undergraduate, graduate.
Defines an enum named Status. The enum Stutus will have 2 data values: parttime, fulltime.
Defines an enum named Residency. The enum Residency will have 2 data values: resident, international.
Defines a student name variable.
Creates a Classification, Status, and Residency, credit hour, cost per credit hour, and tuition variable.
Reads the value of the Classification, Status, and Residency, student name and credit hour variable from the user.
If the student is a fulltime undergraduate resident student, tuition is credit hour times 345.00
If the student is a fulltime undergraduate international student, tuition is credit hour times 685.00
If the student is a fulltime graduate resident student, tuition is credit hour times 545.00
If the student is a fulltime graduate international student , tuition is credit hour times 885.00
If the student is a parttime…
arrow_forward
Write a program that:
Defines an enum named Classification. The enum Classification will have 2 data values: undergraduate, graduate.
Defines an enum named Status. The enum Stutus will have 2 data values: parttime, fulltime.
Defines an enum named Residency. The enum Residency will have 2 data values: resident, international.
Defines a student name variable.
Creates a Classification, Status, and Residency, credit hour, cost per credit hour, and tuition variable.
Reads the value of the Classification, Status, and Residency, student name and credit hour variable from the user.
If the student is a fulltime undergraduate resident student, tuition is credit hour times 345.00
If the student is a fulltime undergraduate international student, tuition is credit hour times 685.00
If the student is a fulltime graduate resident student, tuition is credit hour times 545.00
If the student is a fulltime graduate international student , tuition is credit hour times 885.00
If the student is a parttime…
arrow_forward
Vb
In C++
Create a program that lets you convert the date you want into unix form for example:
if youre date is:
"2011-05-15 18:00:00" is converted to 1330970400Make sure to use "gmtime_s" as gmtime has been DEPRICATED
arrow_forward
When you try to access to a local variable outside the function an error is generatedSelect one:a. Falseb. True
arrow_forward
Create a function named fnTuition that calculates the tuition for a student. This function accepts one parameter, the student ID, and it calls the fnStudentUnits function that you created in task 2. The tuition value for the student calculated according to the following pseudocode:
if (student does not exist) or (student units = 0)
tuition = 0
else if (student units >= 9)
tuition = (full time cost) + (student units) * (per unit cost)
else
tuition = (part time cost) + (student units) * (per unit cost)
Retrieve values of FullTimeCost, PartTimeCost, and PerUnitCost from table Tuition.
If there is no student with the ID passed to the function, the function should return -1.
Code two tests: 1) a student who has < 9 student units, and 2) for a student who has >= 9 student units. For each test, display StudentID and the result returned by the function. Also, run supportive SELECT query or queries that prove the results to be correct.
arrow_forward
Gamex, an online game website launches a game for kids where the kids have to pick up balls from a pool and drag them to the ribbon beneath the pool. The pool contains red, blue and green colored
balls. Tester function of the game checks whether the kid have placed the picked balls according to the following constraints:
The kid should have picked atleast one red, one blue and two green colored balls.
The kid should place the balls in the following order. Red balls followed by green followed by blue balls.
The number of red balls should be equal to blue balls.
iv.
i.
ii.
ii.
The number of green balls should be twice as of red balls.
Design pushdown automata to simulate the function of tester. Justify your answer.
arrow_forward
Question 16
Code a JavaScript function called isLeapYear() that accepts one integer value as its parameter and will return true if this value is a leap year and false if it is not.
Rules: A leap year is divisible by four but not divisible by 100 unless it is divisible by 400.
E.g. 1600, 1912, 1996, 2000, 2020 and 2124 are leap years where 1000 and 3000 are not
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
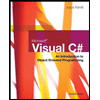
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Related Questions
- Program in c++ languagearrow_forwardInstructions: In the code editor, you are provided with the definition of a struct Person. This struct needs an integer value for its age and a character value for its gender. Furthermore, you are provided with a displayPerson() function which accepts a struct Person as its parameter. In the main(), there are two Persons already created: one Male Person and one Female Person. Your task is to first ask the user for the age of the Male Person and the age of the Female Person. Then, define and declare a function called createKidPerson() which has the following definition: Return type - Person Name - createKidPerson Parameters Person father - the father of the kid to be created Person mother - the mother of the kid to be created Description - creates a new Person and returns this. The age of this Person will be set to 1 while its gender will be set based on the rules mentioned above. Finally, create a new Person and call this createKidPerson() in the main and then pass this newly…arrow_forwardFunction #2: largest Parameters: num1 - a floating point number being compared against num2 and num3 num2 - a floating point number being compared against num1 and num3 num3 - a floating point number being compared against num1 and num2 Description: Write a function that takes in three numbers as parameters and prints out the largest of the three. If two or all of the numbers are equal, or even all three, then just print the value once. Test Cases: >>>largest(433.1, 2340.32, 12323.7) 12323.7 >>>largest(12.0, 32.1, 32.1) 32.1 >>>largest(23.44, 23.44, 23.44) 23.44arrow_forward
- Food Functions - How do I do this practice exercise using Swift code?arrow_forwardQ3- What is the memory content for the following data section variables? L1 db OAAh, 0BBH L2 dw OCCDDH L3 db 0EEh, OFFH L1 L2 L3arrow_forward1 # SuperMarket.py - This program creates a report that lists weekly hours work The student file provided for this lab includes the necessary variable declarations and input and 2 # by employees of a supermarket. The report lists total hours for output statements. You need to implement the code that recognizes when a control break should 3 # each day of one week. occur. You also need to complete the control break code. Be sure to accumulate the daily totals 4 # Input: Interactive 5 # Output: Report. for all days in the week. 6. 7 # Declare variables. Comments in the code tell you where to write your code. 8 HEAD1 = "WEEKLY HOURS WORKED" 9 DAY_FOOTER = "Day Total " 10 SENTINEL = "done" Instructions # Named constant for sentinel value 11 hoursWorked = 0 # Current record hours 12 hoursTotal = 0 # Hours total for a day 1. Study the prewritten code to understand what has already been done. |13 prevDay = "" |14 notDone = True # Previous day of week 2. Write the control break code # loop…arrow_forward
- Assume that grade is a variable whose value is a letter student_grade-- any one of the following letters: 'A', 'B', 'C', 'D', 'F". Assume further that there are the following int variables, declared and already initialized: acount, bcount, ccount, dcount, fcount. Write a switch statement that increments the appropriate variable (acount, bcount, ccount, etc.) depending on the value of grade. So if grade is 'A' then acount is incremented; if grade is'B' then bcount is incremented, and so on.arrow_forwardprintTodaysDate is a function that accepts no parameters and returns no value. Write a statement that calls printTodaysDate.arrow_forward5act2 Please help me code in python programming.arrow_forward
- Summary 2 HouseSign.py - This program calculates prices for cu house signs. In this lab, you complete a prewritten Python program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based 4 on the following facts: 5 # Declare and initialize variables here. 6 # Charge for this sign. • The charge for all signs is a minimum of $35.00. # Number of characters. 8 # Color of characters. • The first five letters or numbers are included in the minimum charge; there is a $4 charge for 9 # Type of wood. 10 each additional character. 11 # Write assignment and if statements here as appropr • If the sign is make of oak, add $20.00. No charge is added for pine. 12 13 # Output Charge for this sign. • Black or white characters are included in the minimum charge; there is an additional $15 14 print("The charge for this sign is $" + str(charge)) charge for gold-leaf lettering. 15 Instructions 1. Make sure the file HouseSign.py…arrow_forwardadd is a function that accepts two int parameters and returns their sum. Two int variables, euroSales and asiaSales, have already been declared and initialized. Another int variable, eurasiaSales, has already been declared. Write a statement that calls add to compute the sum of euroSales and asiaSales and store this value in eurasiaSales.arrow_forwardUsage: mortgagepmt [-s] -r rate [-d downpayment] price In this assignment, you are asked to do a mortgage payment calculation. All information needed for this will be passed to the program on the command line. There will be no user input during the execution of the program. You will need a few pieces of information. The price of the home and the amount of the down payment. You will also need to know the interest rate and the term of the mortgage. To figure your mortgage payment, start by converting your annual interest rate to a monthly interest rate by dividing by 12. Next, add 1 to the monthly rate. Third, multiply the number of years in the term of the mortgage by 12 to calculate the number of monthly payments you’ll make. Fourth, raise the result of 1 plus the monthly rate to the negative power of the number of monthly payments you’ll make. Fifth, subtract that result from 1. Sixth, divide the monthly rate by the result. Last, multiply the result by the amount you want to borrow.…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,
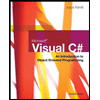
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,