assign(self, assignment:Assignment) -> AssignmentResult: """ This function is to simulate the process of the student receiving an assignment, then working on the assignment, then submitting the assignment and finally receiving grade for the assignment. This function will receive an assignment then a grade should be calculated using the following formula: grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0) After the grade is calculated the student's energy should be decreased by percentage difficulty. Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0) Finally the grade calculated should be stored internally with in this class so it can be retrieved later. Then an Assignment Result object should be created with the student's ID, the assignment received as a parameter, and the grade calculated. This newly created Assignment Result object should be returned. :return: The an AssignmentResult outlining this process """ sleep(self, hours:float): """ This function restore the student's energy as a rate of 10% per hour. So if they sleep for 8 hours there energy will be restored by 80%. If they have 50% (.5) energy and sleep for 8 hours the will wake up with 90% energy = (.5 * (1+.8)). The max energy a s student may have is 100% (1) :param hours: The number of hours a student will sleep for. Example: .2 is equal to 12 minutes or 20% of an hour. :return: None """ get_energy(self): """ Returns the current energy of the student. A number between 0 and 1 :return: The energy of the student. """
assign(self, assignment:Assignment) -> AssignmentResult: """ This function is to simulate the process of the student receiving an assignment, then working on the assignment, then submitting the assignment and finally receiving grade for the assignment. This function will receive an assignment then a grade should be calculated using the following formula: grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0) After the grade is calculated the student's energy should be decreased by percentage difficulty. Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0) Finally the grade calculated should be stored internally with in this class so it can be retrieved later. Then an Assignment Result object should be created with the student's ID, the assignment received as a parameter, and the grade calculated. This newly created Assignment Result object should be returned. :return: The an AssignmentResult outlining this process """ sleep(self, hours:float): """ This function restore the student's energy as a rate of 10% per hour. So if they sleep for 8 hours there energy will be restored by 80%. If they have 50% (.5) energy and sleep for 8 hours the will wake up with 90% energy = (.5 * (1+.8)). The max energy a s student may have is 100% (1) :param hours: The number of hours a student will sleep for. Example: .2 is equal to 12 minutes or 20% of an hour. :return: None """ get_energy(self): """ Returns the current energy of the student. A number between 0 and 1 :return: The energy of the student. """
Chapter9: Using Classes And Objects
Section: Chapter Questions
Problem 9E
Related questions
Question
assign(self, assignment:Assignment) -> AssignmentResult:
"""
This function is to simulate the process of the student receiving an assignment, then working on the
assignment, then submitting the assignment and finally receiving grade for the assignment. This function will
receive an assignment then a grade should be calculated using the following formula:
grade = 1 - (Student's current energy X Assignment difficulty level). The min grade a student may receive is 0% (0)
After the grade is calculated the student's energy should be decreased by percentage difficulty.
Example if the student has 80% (.8) energy and the assignment is a difficultly level .2 there final energy
should be 64% (.64) = .8 - (.8 * .2). The min energy a student may have is 0% (0)
Finally the grade calculated should be stored internally with in this class so it can be retrieved later.
Then an Assignment Result object should be created with the student's ID, the assignment
received as a parameter, and the grade calculated. This newly created Assignment Result object
should be returned.
:return: The an AssignmentResult outlining this process
"""
sleep(self, hours:float):
"""
This function restore the student's energy as a rate of 10% per hour. So if they sleep for 8 hours there energy
will be restored by 80%. If they have 50% (.5) energy and sleep for 8 hours the will wake up with 90% energy
= (.5 * (1+.8)). The max energy a s student may have is 100% (1)
:param hours: The number of hours a student will sleep for. Example: .2 is equal to 12 minutes or 20% of an hour.
:return: None
"""
get_energy(self):
"""
Returns the current energy of the student. A number between 0 and 1
:return: The energy of the student.
"""
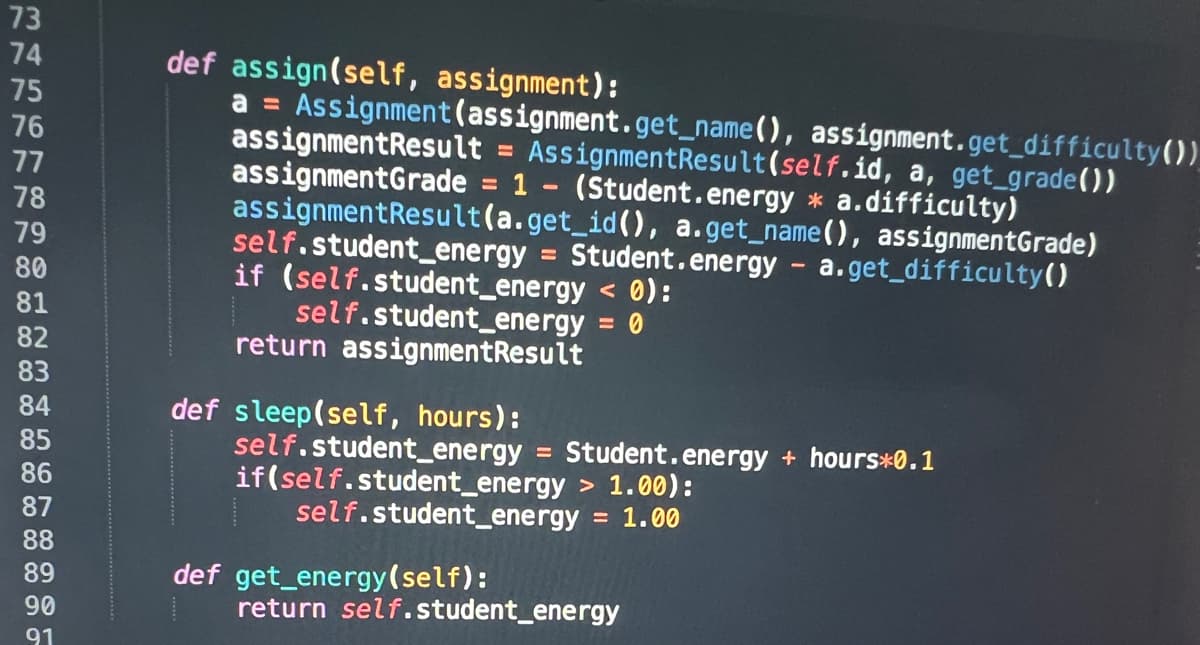
Transcribed Image Text:17455177
73
76
78
79
80
81
82
83
84
85
86
87
88
89
90
91
def assign(self, assignment):
a = Assignment (assignment.get_name(), assignment.get_difficulty())
assignmentResult = AssignmentResult(self.id, a, get_grade())
assignmentGrade = 1 - (Student.energy * a. difficulty)
assignmentResult(a.get_id (), a.get_name(), assignmentGrade)
self.student_energy Student.energy - a.get_difficulty()
< 0):
= 0
if (self.student_energy
self.student_energy
return assignmentResult
def sleep(self, hours):
self.student_energy Student.energy + hours*0.1
if(self.student_energy > 1.00):
self.student_energy = 1.00
def get_energy (self):
return self.student_energy
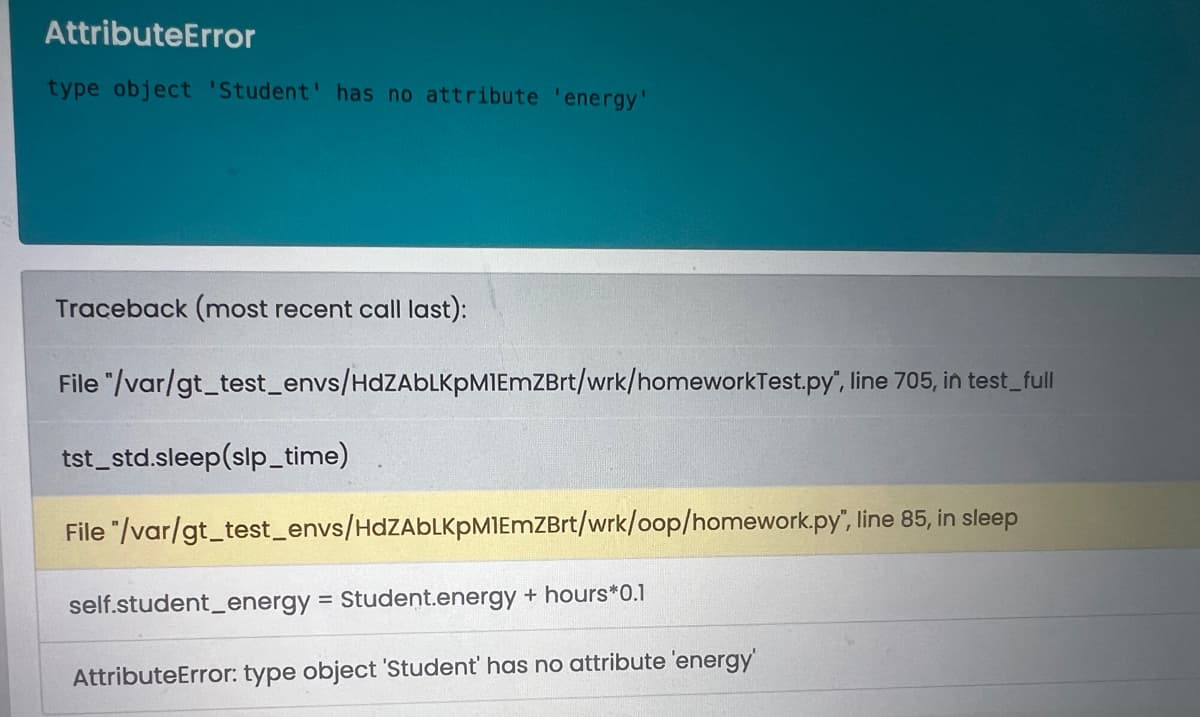
Transcribed Image Text:AttributeError
type object 'Student' has no attribute 'energy'
Traceback (most recent call last):
File "/var/gt_test_envs/HdZAbLKpM1EmZBrt/wrk/homeworkTest.py", line 705, in test_full
tst_std.sleep(slp_time)
File "/var/gt_test_envs/HdZAbLKPM1EmZBrt/wrk/oop/homework.py", line 85, in sleep
self.student_energy = Student.energy + hours*0.1
AttributeError: type object 'Student' has no attribute 'energy'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
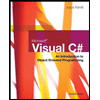
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
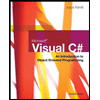
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,