lab03_tables
.html
keyboard_arrow_up
School
Temple University *
*We aren’t endorsed by this school
Course
1013
Subject
Computer Science
Date
Dec 6, 2023
Type
html
Pages
23
Uploaded by samzahroun
Lab 3: Tables
¶
Welcome to lab 3!
This week, we will focus on manipulating tables. We will import our data sets into tables
and complete the majority of analysis using these tables. Tables are described in
Chapter
6
of the Inferential Thinking text. A related approach in Python programming is to use
what is known as a
pandas dataframe
which we will need to resort to occasionally.
Pandas is a mainstay datascience tool.
First, set up the tests and imports by running the cell below.
In [1]:
import numpy as np
from datascience import * # Brings into Python the datascience Table object
# These lines load the tests.
from gofer.ok import check
In [2]:
# Enter your name as a string
# Example
dogname = "Fido"
# Your name
name = "Sam Zahroun"
1. Introduction
¶
For a collection of things in the world, an array is useful for describing a single attribute
of each thing. For example, among the collection of US States, an array could describe
the land area of each. Tables extend this idea by describing multiple attributes for each
element of a collection.
In most data science applications, we have data about many entities, but we also have
several kinds of data about each entity.
For example, in the cell below we have two arrays. The first one contains the world
population in each year (estimated by the US Census Bureau), and the second contains
the years themselves. These elements are in order, so the year and the world population
for that year have the same index in their corresponding arrays.
In [3]:
population_amounts = Table.read_table("world_population.csv").column("Population")
years = np.arange(1950, 2016,1)
print("Population column:", population_amounts)
print("Years column:", years)
Population column: [2557628654 2594939877 2636772306 2682053389 2730228104 2782098943
2835299673 2891349717 2948137248 3000716593 3043001508 3083966929
3140093217 3209827882 3281201306 3350425793 3420677923 3490333715
3562313822 3637159050 3712697742 3790326948 3866568653 3942096442
4016608813 4089083233 4160185010 4232084578 4304105753 4379013942
4451362735 4534410125 4614566561 4695736743 4774569391 4856462699
4940571232 5027200492 5114557167 5201440110 5288955934 5371585922
5456136278 5538268316 5618682132 5699202985 5779440593 5857972543
5935213248 6012074922 6088571383 6165219247 6242016348 6318590956
6395699509 6473044732 6551263534 6629913759 6709049780 6788214394
6866332358 6944055583 7022349283 7101027895 7178722893 7256490011]
Years column: [1950 1951 1952 1953 1954 1955 1956 1957 1958 1959 1960 1961 1962 1963 1964
1965 1966 1967 1968 1969 1970 1971 1972 1973 1974 1975 1976 1977 1978 1979
1980 1981 1982 1983 1984 1985 1986 1987 1988 1989 1990 1991 1992 1993 1994
1995 1996 1997 1998 1999 2000 2001 2002 2003 2004 2005 2006 2007 2008 2009
2010 2011 2012 2013 2014 2015]
Suppose we want to answer this question:
When did world population cross 6 billion?
You could technically answer this question just from staring at the arrays, but it's a bit
convoluted, since you would have to count the position where the population first
crossed 6 billion, then find the corresponding element in the years array. In cases like
these, it might be easier to put the data into a
Table
, a 2-dimensional type of dataset.
The expression below:
•
creates an empty table using the expression
Table()
,
•
adds two columns to the table by calling
with_columns
with four arguments
(column and data for each),
•
assigns the result to the name
population
, and finally
•
evaluates
population
so that we can see the table.
The strings
"Year"
and
"Population"
are column labels that we have chosen. Ther
names
population_amounts
and
years
were assigned above to two arrays of the same
length. The function
with_columns
(you can find the documentation
here
) takes in
alternating strings (to represent column labels) and arrays (representing the data in
those columns), which are all separated by commas. Tip: Both
population_amounts
and
years
need the same number of data points or an error will be returned on attempting to
construct the table.
In [4]:
population = Table().with_columns(
"Population", population_amounts,
"Year", years
)
population
Out[4]:
Population
Year
2557628654 1950
2594939877 1951
2636772306 1952
2682053389 1953
2730228104 1954
2782098943 1955
2835299673 1956
2891349717 1957
Population
Year
2948137248 1958
3000716593 1959
... (56 rows omitted)
Now the data are all together in a single table! It's much easier to parse this data--if you
need to know what the population was in 1959, for example, you can tell from a single
glance. We'll revisit this table later.
Question 1
From the example in the cell above, identify the variables or data types for each of the
following: which variable contains the table? which variable contains an array? On the
right of the equals sign provide the correct variable name.
In [5]:
table_var = population
array_var = years
In [6]:
check('tests/q1.py')
Out[6]:
All tests passed!
2. Creating Tables
¶
Question 2
In the cell below, we've created 2 arrays. In these examples, we're going to be looking at
the Enviornmental Protection Index which describes the state of sustainability in each
country. More information can be found:
Yale EPI
. Using the steps above, assign
top_10_epi
to a table that has two columns called "Country" and "Score", which hold
top_10_epi_countries
and
top_10_epi_scores
respectively.
In [7]:
top_10_epi_scores = make_array(82.5, 82.3, 81.5, 81.3, 80., 79.6, 78.9, 78.7, 77.7, 77.2)
top_10_epi_countries = make_array(
'Denmark',
'Luxembourg',
'Switzerland',
'United Kingdom',
'France',
'Austria',
'Finland',
'Sweden',
'Norway',
'Germany'
)
top_10_epi = Table().with_columns(
"Country", top_10_epi_countries,
"Score", top_10_epi_scores
)
# We've put this next line here so your table will get printed out when you
# run this cell.
top_10_epi
Out[7]:
Country
Score
Denmark
82.5
Luxembourg
82.3
Switzerland
81.5
United Kingdom
81.3
France
80
Austria
79.6
Finland
78.9
Sweden
78.7
Norway
77.7
Germany
77.2
In [8]:
check('tests/q2.py')
Out[8]:
All tests passed!
Loading a table from a file
¶
In most cases, we aren't going to go through the trouble of typing in all the data
manually. Instead, we can use our
Table
functions.
Table.read_table
takes one argument, a path to a data file (a string) and returns a
table. There are many formats for data files, but CSV ("comma-separated values") is the
most common.
Question 3
The file
yale_epi.csv
in the current directory contains a table of information about 180
countries with their corresponding Environmental Performance Index (EPI) based on 32
indicators of sustainability. Load it as a table called
epi
using the
Table.read_table
function.
In [9]:
epi = Table.read_table("yale_epi.csv")
epi
Out[9]:
Country
Score
Decade Change
Rank
Afghanistan
25.5
5
178
Angola
29.7
5.3
158
Country
Score
Decade Change
Rank
Albania
49
10.2
62
United Arab Emirates
55.6
11.3
42
Argentina
52.2
5
54
Armenia
52.3
4.5
53
Antigua and Barbuda 48.5
3.3
63
Australia
74.9
5.5
13
Austria
79.6
5.4
6
Azerbaijan
46.5
4
72
... (170 rows omitted)
In [10]:
check('tests/q3.py')
Out[10]:
All tests passed!
Notice the part about "... (170 rows omitted)." This table is big enough that only a few of
its rows are displayed, but the others are still there. 10 are shown, so there are 180
movies total.
Where did
yale_epi.csv
come from? Take a look at
this lab's folder
. You should see a file
called
yale_epi.csv
.
Open up the
yale_epi.csv
file in that folder and look at the format. What do you notice?
The
.csv
filename ending says that this file is in the
CSV (comma-separated value)
format
.
3. Using lists
¶
A
list
is another Python sequence type, similar to an array. It's different than an array
because the values it contains can all have different types. A single list can contain
int
values,
float
values, and strings. Elements in a list can even be other lists! A list is
created by giving a name to the list of values enclosed in square brackets and separated
by commas. For example,
values_with_different_types = ['data', 8, 8.1]
Lists can be useful when working with tables because they can describe the contents of
one row in a table, which often corresponds to a sequence of values with different types.
A list of lists can be used to describe multiple rows.
Each column in a table is a collection of values with the same type (an array). If you
create a table column from a list, it will automatically be converted to an array. A row, on
the ther hand, mixes types.
Here's a table from Chapter 5. (Run the cell below.)
In [11]:
# Run this cell to recreate the table
flowers = Table().with_columns(
'Number of petals', make_array(8, 34, 5),
'Name', make_array('lotus', 'sunflower', 'rose')
)
flowers
Out[11]:
Number of petals
Name
8
lotus
34
sunflower
5
rose
Question 4
Create a list that describes a new fourth row of this table. The details can be whatever
you want, but the list must contain two values: the number of petals (an
int
value) and
the name of the flower (a string). For example, your flower could be "pondweed"! (A
flower with zero petals)
In [12]:
my_flower = [0, "pondweed"]
my_flower
Out[12]:
[0, 'pondweed']
In [13]:
check('tests/q4.py')
Out[13]:
All tests passed!
Question 5
my_flower
fits right in to the table from chapter 5. Complete the cell below to create a
table of seven flowers that includes your flower as the fourth row followed by
other_flowers
. You can use
with_row
to create a new table with one extra row by
passing a list of values and
with_rows
to create a table with multiple extra rows by
passing a list of lists of values.
In [14]:
# Use the method .with_row(...) to create a new table that includes my_flower
four_flowers = flowers.with_row(my_flower)
# Use the method .with_rows(...) to create a table that
# includes four_flowers followed by other_flowers
other_flowers = [[10, 'lavender'], [3, 'birds of paradise'], [6, 'tulip']]
seven_flowers = four_flowers.with_rows(other_flowers)
seven_flowers
Out[14]:
Number of petals
Name
8
lotus
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
The purpose of this assignment is to give you an opportunity to demonstrate your understanding of the use of lists, dictionaries, importing Python modules, visualizing data analysis, and user experience design. In this exercise, you will use a list to keep track of the results of a dice roll simulation and use dictionaries to help you visualize the results. Using Repl.it or an IDE (e.g. Visual Studio Code) of your choice, create a Python program that allows the user to select the number of dice, and the number of faces per die to roll for a simulation. For example, many board games have the players roll two, six-sided dies, however, some role-playing games may have the players roll more die with more faces.
1. Use a list to keep track of each roll simulation. For example, rolling two, six-sided die twenty times might produce the following Python list of results:
[7, 9, 4, 5, 5, 9, 10, 7, 6, 12, 10, 5, 4, 3, 11, 9, 8, 8, 9, 6]
Update the list and print it after each roll.
2. Using…
arrow_forward
Pandas is a Python library used for working with data sets. It has functions for analyzing, cleaning, exploring, and manipulating data. The name "Pandas" has a reference to both "Panel Data", and "Python Data Analysis" and was created by Wes McKinney in 2008.
Everything in life has both advantages and disadvantages. I discuss 1 advantage and 1 disadvantage of using pandas for Python. Ensure that you attempt to explain your selections.
arrow_forward
7-2 Discussion: Interpreting Multiple Regression Models
Previous Next
In this discussion, you will apply the statistical concepts and techniques covered in this week's reading about multiple regression. You will not be completing work in Jupyter Notebook this week. Instead, you will be interpreting output from your Python scripts for the Module Six discussion. If you did not complete the Module Six discussion, please complete that before working on this assignment.
Last week's discussion involved development of a multiple regression model that used miles per gallon as a response variable. Weight and horsepower were predictor variables. You performed an overall F-test to evaluate the significance of your model. This week, you will evaluate the significance of individual predictors. You will use output of Python script from Module Six to perform individual t-tests for each predictor variable. Specifically, you will look at Step 5 of the Python script to answer…
arrow_forward
In python don't import libraries
arrow_forward
Use Python Code
Your task is : Use pandas to read the csv table . Example : df = pd.read_csv('Covid19Recovered.csv') . Then use the dataframe to make a visualization of the number of recovered patients using matplotlib, seaborn , etc . Just ignore lat and long.
Covid-19 Recovered table (csv):
Province/State
Country/Region
Lat
Long
########
########
########
########
########
########
########
########
########
########
2/1/2020
2/2/2020
2/3/2020
2/4/2020
2/5/2020
2/6/2020
2/7/2020
2/8/2020
2/9/2020
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
3/1/2020
3/2/2020
3/3/2020
3/4/2020
3/5/2020
3/6/2020
3/7/2020
3/8/2020
3/9/2020
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
4/1/2020
4/2/2020
4/3/2020…
arrow_forward
Please answer in Java, thank you.
The purpose of this assignment is to practice your knowledge of how a linked data structure is implemented. This is a more advanced version of a linked list, and will help to give you practice in how to deal with data structures connected through references. This assignment is very important to help you understand the fundamentals of Linked Lists. At the end of this assignment, you should be able to fully understand how any variation of a Linked List is implemented.
Instructions:
For this project you will be implementing a new data structure called LinkedGrid. This is a linked type data structure version of a 2D array. The LinkedGrid can support any combination of dimensions (n x n, n x m, or m x n, where n > m) This data structure has a singly linked system which allows you to traverse the grid in multiple directions in addition to allowing you to retrieve specific rows and columns. The following diagram gives a rough visual of this data…
arrow_forward
A data set has 4 columns--index, CUSTOMER_EMAIL, Net Revenue, and Year.
For each year we need the following information:
New Customer Revenue e.g., new customers not present in previous year only
Lost Customers
New Customers
Total Customers Previous Year
Total Customers Current Year
Please implement it using python.
arrow_forward
PYTHON PROGRAMMING
Files are here: http://www.cse.msu.edu/~cse231/Online/Projects/Project05/
This exercise is about data manipulation.
In this project, we will process agricultural data, namely the adoption of different Genetically Modified (GM) crops in the U.S. The data was collected over the years 2000-2016.
In this project, we are interested in how the adoption of different GM food and non-food crops has been proceeding in different states. We are going to determine the minimum and maximum adoption by state and the years when the minimum and maximum occurred.
Assignment Specifications:
The data files The data files that you use are yearly state-wise percentage plantings for each type of crop:
• alltablesGEcrops.csv: the actual data from the USDA.
• data1.csv: data modified to test this project.
• data2.csv: data modified to test this project, but you do not get to see it
Input: This is real data so it is messy and you have to deal with that. Here are the constraints.…
arrow_forward
we studied arrays as an important data structure in Java. Discuss a situation and give an example for data that can be captured by using one-dimensional array. Explain and provide the code for the situation.Which approach do you prefer and why?
arrow_forward
How will this project be solved in Python? Thanks.
There are example outputs in the picture.
arrow_forward
For this assignment, you will create a glossary (dictionary) of technical terms and definitions. It will be set up as a Python dictionary structure. The file glossary_starter.py is a complete starter framework for the assignment. It includes some initial values for the dictionary. It is long because most of the code has already been written for you.Your task is to complete the five individual functions for adding and deleting terms, looking up terms, listing them, and printing out both the terms and definitions. These functions are all short, just a couple of lines, and use basic dictionary methods and techniques.
""" Program framework for module 9 graded program Creating a dictionary of technical terms and basic definitions key - the word to define value - the definition of the word Note: This isn't a usable application, as new data added is just temporary."""
tech_terms = { "dict": "stores a key/value pair", "list": "stores a value at each index",…
arrow_forward
Convert this Python script to a MATLAB script.
Hint: Use the matlab-python-cheatsheet.
End all assignment statements with a semi-colon to suppress output to screen.
Include the key word end at the end of your function.
You should try developing in MATLAB and not the quiz server.
def make_list(start, step, stop):
Creates a list - first element is start, last element is stop, each element value increases by step.
Parameters:
start - int - first element in list
step - int - value to increment each element by
stop - int - last element in list
Returns:
list of ints
return list(range(start, stop + 1, step))
For example:
Test
Result
disp(all(make_list(1, 2, 11)== [1 3 5 7 9 11]))
1
disp(all(make_list(10, -2, -10)
== [10 8 6 4 2 0 -2 -4 -6 -8 -10])) 1
arrow_forward
correct and detailed answer will be upvoted else downvoted. Output screenshot also required.
understudies went to the primary gathering of the Berland SU programming course (n is even). All understudies will be partitioned into two gatherings. Each gathering will go to precisely one example every week during one of the five working days (Monday, Tuesday, Wednesday, Thursday and Friday), and the days picked for the gatherings should be unique. Besides, the two gatherings ought to contain similar number of understudies.
Every understudy has filled an overview where they told which days of the week are advantageous for them to go to an illustration, and which are not.
Your assignment is to decide whether it is feasible to pick two unique week days to plan the examples for the gathering (the main gathering will go to the illustration on the primary picked day, the subsequent gathering will go to the example on the second picked day), and gap the understudies into two gatherings, so…
arrow_forward
Assume you are in charge of compiling the information from books. Either more books
should be placed on the shelf, or the books already on one side of the shelf should be
removed. Choose and explain the best data structure for this situation. Give a flexible
answer on how to add books to the shelf. What happens when a data structure has to
be updated with new information but has insufficient space?
arrow_forward
Using Pandas library in python - Calculate student grades project
Pandas is a data analysis library built in Python. Pandas can be used in a Python script, a Jupyter Notebook, or even as part of a web application. In this pandas project, you’re going to create a Python script that loads grade data of 5 to 10 students (a .csv file) and calculates their letter grades in the course. The CSV file contains 5 column students' names, score in-class participation (5% of final grade), score in assignments (20% of final grade), score in discussions (20% of final grade), score in the mid term (20% of final grade), score in final (25% of final grade). Create the .csv file as part of the project submission
Program Output
This will happen when the program runs
Enter the CSV file
Student 1 named Brian Tomas has a letter grade of B+
Student 2 named Tom Blank has a letter grade of C
Student 3 named Margo True has a letter grade of A
Student 4 named David Atkin has a letter grade of B+
Student 5 named…
arrow_forward
PYTHON PROGRAMMING.
USE PROVIDED STARTING CODE TO COMPLETE THIS TASK.
Create a glossary (dictionary) of technical terms and definitions. It will be set up as a Python dictionary structure. The file glossary_starter.py is a complete starter framework for the assignment. It includes some initial values for the dictionary. It is long because most of the code has already been written for you.
Your task is to complete the five individual functions for adding and deleting terms, looking up terms, listing them, and printing out both the terms and definitions. These functions are all short, just a couple of lines, and use basic dictionary methods and techniques.
PROVIDED CODE:
""" Program framework for module 9 graded program Creating a dictionary of technical terms and basic definitions key - the word to define value - the definition of the word Note: This isn't a usable application, as new data added is just temporary."""
tech_terms = { "dict": "stores a key/value pair",…
arrow_forward
I have a data set with 100 rows and 1 column. I want to insert another column and populate it with '1' in each row. I want this new column to precede the existing column. How do I do this in python?
arrow_forward
What's wrong with this cell?
arrow_forward
If you had the option of having a million-element list, which implementation of the size and isEmpty functions would you use? (Consider the issue with the University of Michigan's alumni database.) If you had the option of making a million little lists, how would you choose? Think about the challenge of tracking dependents for a million income tax filings.)
arrow_forward
Create a BST implementation that uses three arrays to represent the BST: a key array, a left and right array indices array, and a right array indices array. The constructor's maximum size should be preallocated to the arrays. Compare your program's effectiveness to that of a typical implementation.
arrow_forward
Best Practices to Follow:
It’s worth breaking up each functional task in create_blackjack_game() into separate functions. For example, a function to check the score of a player’s hand, to check whether a player has won, and so on. This entire implementation can be done neatly in about eight functions.
Create a Deck of Cards
Complete the create_standard_deck() function, which creates a new deck. The deck itself must be a data structure list. The cards within the deck must be represented as tuples of the form (suit, number), where the suit is a string that must be either ‘hearts’, ‘clubs’, ‘spades’, or ‘diamonds’, and the number is an integer that must be one of the following: 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, or 14. Note that the number used to identify the card has the following association: Jack = 11, Queen = 12, King = 13, and Ace = 14. In terms of value, the picture cards (Jack, Queen, and King) all have a value of 10, and the Ace card has a value of 11. The casino only wants…
arrow_forward
Use Python Code
Your task is : Number of reported COVID-19 deaths by country. (Use pandas to read the csv table. Example : df = pd.read_csv('Covid19Deaths.csv') then do the code using the dataframe)
COVID-19 Deaths table (csv):
Province/State
Country/Region
Lat
Long
########
########
########
########
########
########
########
########
########
########
2/1/2020
2/2/2020
2/3/2020
2/4/2020
2/5/2020
2/6/2020
2/7/2020
2/8/2020
2/9/2020
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
3/1/2020
3/2/2020
3/3/2020
3/4/2020
3/5/2020
3/6/2020
3/7/2020
3/8/2020
3/9/2020
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
########
4/1/2020
4/2/2020
4/3/2020
4/4/2020
4/5/2020
4/6/2020
4/7/2020
Afghanistan
33
65
0
0
0
0
0
0
0
0…
arrow_forward
in relation to python: 'job' and 'sf' table is not sorted(no order). would the arrays you generate in the 'jobs' column be the same if we had sorted them alphabetically instead before generating them? Explain and reference how .group method works and how sorting 'jobs' would impact this.
Image attached is the 'job' column
arrow_forward
Focus on dictionary methods, use of functions, and good programming style For this assignment, you will create a glossary (dictionary) of technical terms and definitions. It will be set up as a Python dictionary structure. The file glossary_starter.py is a complete starter framework for the assignment. It includes some initial values for the dictionary. It is long because most of the code has already been written for you. Your task is to complete the five individual functions for adding and deleting terms, looking up terms, listing them, and printing out both the terms and definitions. These functions are all short, just a couple of lines, and use basic dictionary methods and techniques. Here is some sample output(Attached)
And here is the glossary_starter.py:
""" Program framework for module 9 graded program Creating a dictionary of technical terms and basic definitions key - the word to define value - the definition of the word Note: This isn't a usable…
arrow_forward
try using other data structures to iterate over and see what happens. Does the way for treats those data structures make sense?
arrow_forward
Please written by computer source
Assignment 4 In this assignment you will be using the dataset released by The Department of Transportation. This dataset lists flights that occurred in 2015, along with other information such as delays, flight time etc.
In this assignment, you will be showing good practices to manipulate data using Python's most popular libraries to accomplish the following:
cleaning data with pandas make specific changes with numpy handling date-related values with datetime Note: please consider the flights departing from BOS, JFK, SFO and LAX.
Each question is equally weighted for the total grade.
import os
import pandas as pd
import pandas.api.types as ptypes
import numpy as np
import datetime as dt
airlines_df= pd.read_csv('assets\airlines.csv')
airports_df = pd.read_csv('assets\airports.csv')
flights_df_raw = pd.read_csv('assets\flights.csv', low_memory = False)
Question 1: Data Preprocessing
For this question, perform the following:
remove rows with…
arrow_forward
The following 4 line data source will be input to a wordcount mapreduce implementation having 3 mappers and 2 reducers;FIRST NAME LAST NAME COMP NAMEPHONE PHONE EMAIL WEBJOHN BUTT BENTON JOHNHTTP WWW BENTON COMThe data will be split into individual lines with each line processed by one of the mappers.Using the 1st line from the data source, show the output produced by the mapper that processes that line
arrow_forward
In what situations is it preferable to work with a vector rather than a table?
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
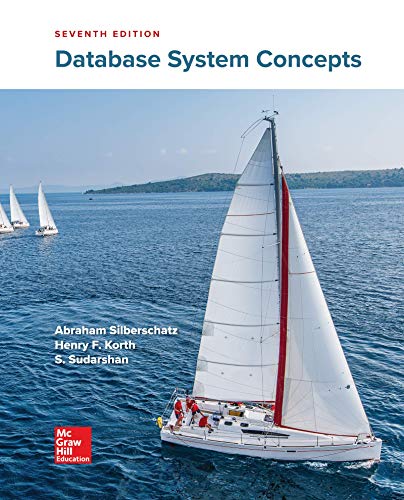
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
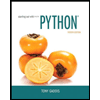
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
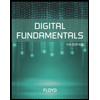
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
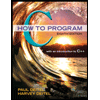
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
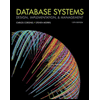
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
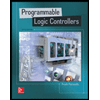
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- The purpose of this assignment is to give you an opportunity to demonstrate your understanding of the use of lists, dictionaries, importing Python modules, visualizing data analysis, and user experience design. In this exercise, you will use a list to keep track of the results of a dice roll simulation and use dictionaries to help you visualize the results. Using Repl.it or an IDE (e.g. Visual Studio Code) of your choice, create a Python program that allows the user to select the number of dice, and the number of faces per die to roll for a simulation. For example, many board games have the players roll two, six-sided dies, however, some role-playing games may have the players roll more die with more faces. 1. Use a list to keep track of each roll simulation. For example, rolling two, six-sided die twenty times might produce the following Python list of results: [7, 9, 4, 5, 5, 9, 10, 7, 6, 12, 10, 5, 4, 3, 11, 9, 8, 8, 9, 6] Update the list and print it after each roll. 2. Using…arrow_forwardPandas is a Python library used for working with data sets. It has functions for analyzing, cleaning, exploring, and manipulating data. The name "Pandas" has a reference to both "Panel Data", and "Python Data Analysis" and was created by Wes McKinney in 2008. Everything in life has both advantages and disadvantages. I discuss 1 advantage and 1 disadvantage of using pandas for Python. Ensure that you attempt to explain your selections.arrow_forward7-2 Discussion: Interpreting Multiple Regression Models Previous Next In this discussion, you will apply the statistical concepts and techniques covered in this week's reading about multiple regression. You will not be completing work in Jupyter Notebook this week. Instead, you will be interpreting output from your Python scripts for the Module Six discussion. If you did not complete the Module Six discussion, please complete that before working on this assignment. Last week's discussion involved development of a multiple regression model that used miles per gallon as a response variable. Weight and horsepower were predictor variables. You performed an overall F-test to evaluate the significance of your model. This week, you will evaluate the significance of individual predictors. You will use output of Python script from Module Six to perform individual t-tests for each predictor variable. Specifically, you will look at Step 5 of the Python script to answer…arrow_forward
- In python don't import librariesarrow_forwardUse Python Code Your task is : Use pandas to read the csv table . Example : df = pd.read_csv('Covid19Recovered.csv') . Then use the dataframe to make a visualization of the number of recovered patients using matplotlib, seaborn , etc . Just ignore lat and long. Covid-19 Recovered table (csv): Province/State Country/Region Lat Long ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## 2/1/2020 2/2/2020 2/3/2020 2/4/2020 2/5/2020 2/6/2020 2/7/2020 2/8/2020 2/9/2020 ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## 3/1/2020 3/2/2020 3/3/2020 3/4/2020 3/5/2020 3/6/2020 3/7/2020 3/8/2020 3/9/2020 ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## ######## 4/1/2020 4/2/2020 4/3/2020…arrow_forwardPlease answer in Java, thank you. The purpose of this assignment is to practice your knowledge of how a linked data structure is implemented. This is a more advanced version of a linked list, and will help to give you practice in how to deal with data structures connected through references. This assignment is very important to help you understand the fundamentals of Linked Lists. At the end of this assignment, you should be able to fully understand how any variation of a Linked List is implemented. Instructions: For this project you will be implementing a new data structure called LinkedGrid. This is a linked type data structure version of a 2D array. The LinkedGrid can support any combination of dimensions (n x n, n x m, or m x n, where n > m) This data structure has a singly linked system which allows you to traverse the grid in multiple directions in addition to allowing you to retrieve specific rows and columns. The following diagram gives a rough visual of this data…arrow_forward
- A data set has 4 columns--index, CUSTOMER_EMAIL, Net Revenue, and Year. For each year we need the following information: New Customer Revenue e.g., new customers not present in previous year only Lost Customers New Customers Total Customers Previous Year Total Customers Current Year Please implement it using python.arrow_forwardPYTHON PROGRAMMING Files are here: http://www.cse.msu.edu/~cse231/Online/Projects/Project05/ This exercise is about data manipulation. In this project, we will process agricultural data, namely the adoption of different Genetically Modified (GM) crops in the U.S. The data was collected over the years 2000-2016. In this project, we are interested in how the adoption of different GM food and non-food crops has been proceeding in different states. We are going to determine the minimum and maximum adoption by state and the years when the minimum and maximum occurred. Assignment Specifications: The data files The data files that you use are yearly state-wise percentage plantings for each type of crop: • alltablesGEcrops.csv: the actual data from the USDA. • data1.csv: data modified to test this project. • data2.csv: data modified to test this project, but you do not get to see it Input: This is real data so it is messy and you have to deal with that. Here are the constraints.…arrow_forwardwe studied arrays as an important data structure in Java. Discuss a situation and give an example for data that can be captured by using one-dimensional array. Explain and provide the code for the situation.Which approach do you prefer and why?arrow_forward
- How will this project be solved in Python? Thanks. There are example outputs in the picture.arrow_forwardFor this assignment, you will create a glossary (dictionary) of technical terms and definitions. It will be set up as a Python dictionary structure. The file glossary_starter.py is a complete starter framework for the assignment. It includes some initial values for the dictionary. It is long because most of the code has already been written for you.Your task is to complete the five individual functions for adding and deleting terms, looking up terms, listing them, and printing out both the terms and definitions. These functions are all short, just a couple of lines, and use basic dictionary methods and techniques. """ Program framework for module 9 graded program Creating a dictionary of technical terms and basic definitions key - the word to define value - the definition of the word Note: This isn't a usable application, as new data added is just temporary.""" tech_terms = { "dict": "stores a key/value pair", "list": "stores a value at each index",…arrow_forwardConvert this Python script to a MATLAB script. Hint: Use the matlab-python-cheatsheet. End all assignment statements with a semi-colon to suppress output to screen. Include the key word end at the end of your function. You should try developing in MATLAB and not the quiz server. def make_list(start, step, stop): Creates a list - first element is start, last element is stop, each element value increases by step. Parameters: start - int - first element in list step - int - value to increment each element by stop - int - last element in list Returns: list of ints return list(range(start, stop + 1, step)) For example: Test Result disp(all(make_list(1, 2, 11)== [1 3 5 7 9 11])) 1 disp(all(make_list(10, -2, -10) == [10 8 6 4 2 0 -2 -4 -6 -8 -10])) 1arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
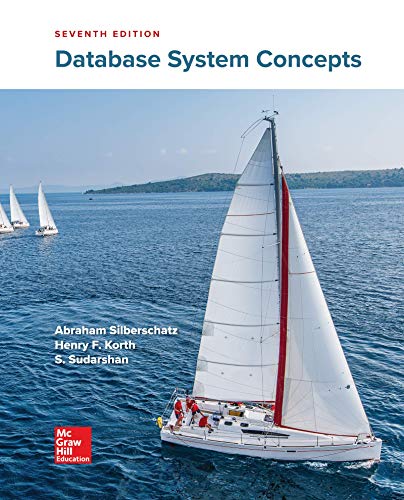
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
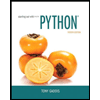
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
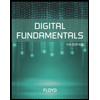
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
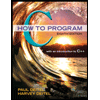
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
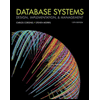
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
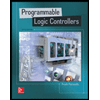
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education