CS 104 Lab 8
.pdf
keyboard_arrow_up
School
Illinois Institute Of Technology *
*We aren’t endorsed by this school
Course
201
Subject
Computer Science
Date
Apr 3, 2024
Type
Pages
5
Uploaded by MinisterAnteaterPerson263
cs104 Exercise Set 8
1.
You compile your code without any syntax errors, and run your program. At
some point, the code does the same thing over and over again and will not stop.
This is called :
a.
A run-on
b.
An infinite loop
c.
A hiccup
d.
A code ride
2.
You need to write a loop that needs to run the same code NUMLOOPS times.
The most appropriate type of loop is :
a.
A while loop
b.
A forever loop
c.
A for loop
d.
A goto loop
3.
You need to write a loop that needs to run the same code some number of times.
You won’t know how many until you run the program. The most appropriate type
of loop is :
a.
A while loop
b.
A forever loop
c.
A for loop
d.
A goto loop
4.
What is the output of the following code:
product = 1, k = 6;
while k < 9
product = product * k;
k = k + 1;
end
out1 = sprintf(‘k is : %d’,k);
out2 = sprintf(‘product is : %d’, product );
disp(out1);
disp(out2);
‘k is: 9’
‘product is: 280’
5.
What is the output of the following code:
k = 6;
for k = 1: 3
out1 = sprintf(‘Hello’ );
disp(out1);
end
out1 = sprintf(‘Done!’ );
disp(out1);
‘Hello’
‘Hello’
‘Hello’
‘Done!’
6.
What is the output of the following code:
k = 6;
for k = 1 : 6
out1 = sprintf(‘Hello’ );
disp(out1);
end
out1 = sprintf(‘Done!’ );
disp(out1);
‘Done!’
7.
What is the output of the following code:
sum = 0; k = 14;
for k = 1:5
sum = sum + k;
end
out1 = sprintf(‘ k is : %d‘,k);
out2 = sprintf(‘sum is : %d’,sum);
disp(out1);
disp(out2);
‘k is: 14‘
‘sum is: 126‘
8.
What is the output of the following code:
sum = 0; k = 14;
for
k = 1:40
if mod(k,10) == 0
sum = sum + k;
end
end
out1 = sprintf(‘ k is : %d‘,k);
out2 = sprintf(‘sum is : %d’,sum);
disp(out1);
disp(out2);
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Text Rectangle. Create a program that draws a rectangle in text mode given the dimensions provided by the user. Attached is a sample of the execution of the program.
Hint: Use a loop and the repetition operator to create the rectangle.
arrow_forward
Nesting Loops
Summary
In this lab, you add nested loops to a Java program provided. The program should print the letter E. The letter E is printed using asterisks, three across and five down. Note that this program uses System.out.print("*"); to print an asterisk without a new line.
Instructions
Write the nested loops to control the number of rows and the number of columns that make up the letter E.
In the loop body, use a nested if statement to decide when to print an asterisk and when to print a space. The output statements have been written, but you must decide when and where to use them.
Execute the program. Observe your output.
Modify the program to change the number of rows from five to seven and the number of columns from three to five. What does the letter E look like now?
arrow_forward
Turtle Drawing with Loops
Objective: To make a drawing using turtle graphics and nested loops
Requirements:
-Your program should ask the user at least two questions, and use the answers to help make the drawing.
-Your program must have at least one pair of nested loops (a loop inside of a loop) to do some of the drawing
-Your drawing cannot be made up only of rotated polygons coming from the center of the screen like we did in class.
-Please write a new program for this assignment - don't use code from any in-class exercises.
-For example, your program could draw a field of flowers, or you could draw a repetitive square pattern like you might see on a rug.
arrow_forward
Write a loop that runs 10 times. Note: You are not required to put anything inside the loop body.
arrow_forward
Write a while loop that will let the user enter a series of integer values and compute the total values and number of values entered. An odd number will stop the loop. Display the number of iterations and the total of the values after the loop terminates. for Loop Write a for loop to display all numbers from 13 - 93 inclusive, ending in 3. Write a for loop to display a string entered by the user backwards. do Loop Write a do .. loop for the random number dice problem from Chap Three. Add a counter variable that is used to count the number of times this code is executed in the loop and stops after 5 times. roll = rand.nextInt(6) + 1; System.out.println(" Roll = " + roll); ctr++;
using java
arrow_forward
The While loop is a type of loop.a. pretestb. posttestc. prequalifiedd. post iterative
arrow_forward
Match the terms to their corresponding descriptions
This loop structure can be used when the number of iterations is known or can be calculated.
Choose...
This loop structure can be used in any situation a loop is needed.
Choose...
This loop can be used whenever we need the user to explicitly indicate when the loop should
"seeding" loop
continue.
"while" loop
This loop can be used when the program expects a stream of values, and one these values
"guarding" loop
signals the end of the loop.
Loop "condition"
"infinite" loop
"sentinel" loop
Next page
"user-confirmation" loop
"for" loop
arrow_forward
Code in Java using Do-while Loop
Write a program that accepts an integer input. If the integer is positive, print the values from the positive integer down to zero. Otherwise, if it is negative, print the values from the negative integer up to zero. Separate each number with a space.
Input
1. One line containing an integer
Output
Enter a number: 5
5 4 3 2 1 0
arrow_forward
Basic while loop expression.
Write a while loop that prints userNum divided by 4 (integer division) until reaching 2 or less. Follow each number by a space. Example output for userNum = 160:40 10 2 Note: These activities may test code with different test values. This activity will perform four tests, with userNum = 160, then with userNum = 8, then with userNum = 0, then with userNum = -1. See "How to Use zyBooks".Also note: If the submitted code has an infinite loop, the system will stop running the code after a few seconds, and report "Program end never reached." The system doesn't print the test case that caused the reported message.
arrow_forward
computer programming
Loop is used to repeat a sequence of actions or statements many times. Simplydescribe the differences between for-loop and while-loop.
arrow_forward
Question#2. The distance a vehicle travels can be calculated as fallout:
Distance = Speed * Time
For example, if a train travels 60 miles-per-hour for three hours, the distance [raveled is 120
miles. Write a program that asks for the speed of a vehicle (in miles-per-hour) and the
number of hours it has traveled. It should use a loop to display the distance a vehicle has
traveled for each hour of a time period specified by the user. For example, if a vehicle is
traveling at 40 mph far a three-hour time period, it should display a report similar to the one
chat follows:
Hour
Distance Traveled
1
90
2
100
3
150
Input Validation: Do not accept a negative number for speed and do not accept any value les
than 1 for time traveled.
arrow_forward
Programming is Java
arrow_forward
A for statement is a loop that goes through a list of things. Therefore, it keeps running as long as there are objects to process. Is this a true or a false statement?
arrow_forward
program4_3.pyWrite a program that uses nested loops to print the output exactly as shown below. NOTE: You must use nested loops.
arrow_forward
Factorials
The factorial of n (written n!) is the product of the integers between 1 and n. Thus 4! = 1*2*3*4 = 24. By definition, 0! = 1.
Factorial is not defined for negative numbers.
Write a program that asks the user for a non-negative integer and computes and prints the factorial of that integer. You'll
need a while loop to do most of the work-this is a lot like computing a sum, but it's a product instead. And you'll need
to think about what should happen if the user enters 0.
1.
2. Now modify your program so that it checks to see if the user entered a negative number. If so, the program should print a
message saying that a nonnegative number is required and ask the user the enter another number. The program should
keep doing this until the user enters a nonnegative number, after which it should compute the factorial of that number.
Hint: you will need another while loop before the loop that computes the factorial. You should not need to change any
of the code that computes the…
arrow_forward
pow(3,4)=?
Select one:
O a. 81
O b. 12
c.
64
O d. 7
arrow_forward
We use For loop instead of a while loop when we know exactly how many times you want to loop through a block of code.
True
False
arrow_forward
6. Distance Traveled
The distance a vehicle travels can be calculated as follows:
distance = speed time
For example, if a train travels 40 miles per hour for 3 hours, the distance traveled is
120 miles.
Write a program that asks the user for the speed of a vehicle (in miles per hour) and how
many hours it has traveled. The program should then use a loop to display the distance the
vehicle has traveled for each hour of that time period. Here is an example of the output:
What is the speed of the vehicle in mph? 40
How many hours has it traveled? 3
Hour Distance Traveled
1
40
2
80
3
120
Input Validation: Do not accept a negative number for speed and do not accept any
value less than 1 for time traveled.
arrow_forward
True or False: The do-while loop is a pretest loop, as opposed to a main loop.
arrow_forward
Create a class name AddAgain for do-while loop that asks the user to enter two The numbers should be added and the sum displayed. The loop should ask the user whether he or she wishes to perform the operation again. If so, the loop should repeat; otherwise it should terminate.
arrow_forward
1. In the first loop, the low number will be the starting point for the loop and thehigh will be the ending point. The loop should display the iterator number andthat number x 10 on the same line, separated by a tab. See example on p. 177.2. The second loop should accumulate all the numbers between the starting pointand ending point. You will need to create and initialize an accumulator such astotal before you start the loop.
arrow_forward
HELP NEEDED ASAP!
Language: JAVA
Write a program in Java that takes an integer value as input from the keyboard and prints a pattern like a right-angle triangle with the line number. For input value 4 the pattern is as follows:
1
22
333
4444
Hint: use nested loops.
arrow_forward
n is an integer variable.Write a while loop that prints integer numbers starting with n and dividing the number to 4 each time, while the new number is greater than 0.(Example: If n is 2077, it will print 2077 519 129 ...)Write only the statements to perform the above explained task, nothing else.Use while loop, don't use for loop.
arrow_forward
A bug collector collects bugs every day for seven days. Design a solution that keeps a running total of the number of bugs collected during the seven days. The loop should ask for the number of bugs collected for each day, and when the loop is finished, the program should display the total number of bugs collected.
arrow_forward
If a loop does not contain within itself a way to terminate, it is called a:
Select one:
a.
infinite loop
b.
do-while loop
c.
while loop
d.
for loop
arrow_forward
True or False: The do-while loop is a pretest loop.
arrow_forward
python
arrow_forward
Convert the following for loop to a while loop:
for (int x = 50; x > 0; x--){
cout << x << " seconds to go.\n";
}
arrow_forward
In C#
arrow_forward
Exercise # 2: Write a while loop that prints all positive numbers that are divisible by 10 and less than the user input value n.
For example, if the user enters 100, the program shall print 10 20 30 40 50 60 70 80 90.
arrow_forward
in python a loop that does not terminate is known as a ?
a. an if-else loop
b. an infinite loop
c. a sentry loop
d. a counting loop
arrow_forward
Task 2 [Low-quality graphics circle]
This task is to help you practice nested for-loops (for-loop inside a for-loop) and if-statements.
Hint: if you want to print a new line, you can print an empty string, e.g., print("").
For this task, we will draw a simple text-based circle.
Recall that a circle is described as follows: x² + y² = r²
Any x, y value, such that x² + y² ≤ r², will be a point inside or on the circle.
With this simple information, we can draw a circle.
Your task should work as follows:
(1) Ask the user to input a radius from [1 to 10].
-> You can assume the input is an integer
-> If the value is not between 1 and 10, keep asking
for input [see example below]
(2) loop y from 10 to 10
loop x from 10 to 10
if the current x,y is inside or on the circle
(see equation above), then
print a '*', otherwise print a '.'
hint: consider using print (..., end="")
Examples of Task 2 (user input in red)
Task 2: Draw circle
----
Input size between 1-10: 11
Input size between 1-10: 0
Input…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
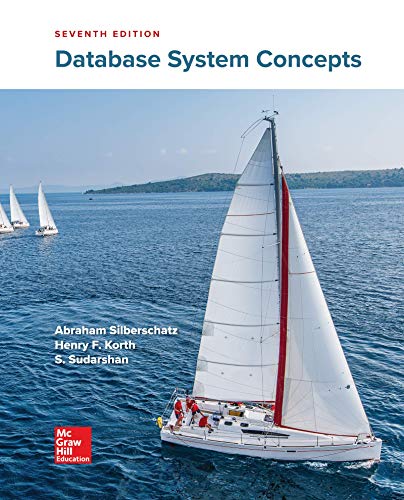
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
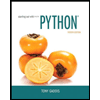
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
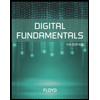
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
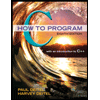
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
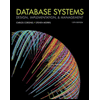
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
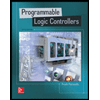
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Text Rectangle. Create a program that draws a rectangle in text mode given the dimensions provided by the user. Attached is a sample of the execution of the program. Hint: Use a loop and the repetition operator to create the rectangle.arrow_forwardNesting Loops Summary In this lab, you add nested loops to a Java program provided. The program should print the letter E. The letter E is printed using asterisks, three across and five down. Note that this program uses System.out.print("*"); to print an asterisk without a new line. Instructions Write the nested loops to control the number of rows and the number of columns that make up the letter E. In the loop body, use a nested if statement to decide when to print an asterisk and when to print a space. The output statements have been written, but you must decide when and where to use them. Execute the program. Observe your output. Modify the program to change the number of rows from five to seven and the number of columns from three to five. What does the letter E look like now?arrow_forwardTurtle Drawing with Loops Objective: To make a drawing using turtle graphics and nested loops Requirements: -Your program should ask the user at least two questions, and use the answers to help make the drawing. -Your program must have at least one pair of nested loops (a loop inside of a loop) to do some of the drawing -Your drawing cannot be made up only of rotated polygons coming from the center of the screen like we did in class. -Please write a new program for this assignment - don't use code from any in-class exercises. -For example, your program could draw a field of flowers, or you could draw a repetitive square pattern like you might see on a rug.arrow_forward
- Write a loop that runs 10 times. Note: You are not required to put anything inside the loop body.arrow_forwardWrite a while loop that will let the user enter a series of integer values and compute the total values and number of values entered. An odd number will stop the loop. Display the number of iterations and the total of the values after the loop terminates. for Loop Write a for loop to display all numbers from 13 - 93 inclusive, ending in 3. Write a for loop to display a string entered by the user backwards. do Loop Write a do .. loop for the random number dice problem from Chap Three. Add a counter variable that is used to count the number of times this code is executed in the loop and stops after 5 times. roll = rand.nextInt(6) + 1; System.out.println(" Roll = " + roll); ctr++; using javaarrow_forwardThe While loop is a type of loop.a. pretestb. posttestc. prequalifiedd. post iterativearrow_forward
- Match the terms to their corresponding descriptions This loop structure can be used when the number of iterations is known or can be calculated. Choose... This loop structure can be used in any situation a loop is needed. Choose... This loop can be used whenever we need the user to explicitly indicate when the loop should "seeding" loop continue. "while" loop This loop can be used when the program expects a stream of values, and one these values "guarding" loop signals the end of the loop. Loop "condition" "infinite" loop "sentinel" loop Next page "user-confirmation" loop "for" looparrow_forwardCode in Java using Do-while Loop Write a program that accepts an integer input. If the integer is positive, print the values from the positive integer down to zero. Otherwise, if it is negative, print the values from the negative integer up to zero. Separate each number with a space. Input 1. One line containing an integer Output Enter a number: 5 5 4 3 2 1 0arrow_forwardBasic while loop expression. Write a while loop that prints userNum divided by 4 (integer division) until reaching 2 or less. Follow each number by a space. Example output for userNum = 160:40 10 2 Note: These activities may test code with different test values. This activity will perform four tests, with userNum = 160, then with userNum = 8, then with userNum = 0, then with userNum = -1. See "How to Use zyBooks".Also note: If the submitted code has an infinite loop, the system will stop running the code after a few seconds, and report "Program end never reached." The system doesn't print the test case that caused the reported message.arrow_forward
- computer programming Loop is used to repeat a sequence of actions or statements many times. Simplydescribe the differences between for-loop and while-loop.arrow_forwardQuestion#2. The distance a vehicle travels can be calculated as fallout: Distance = Speed * Time For example, if a train travels 60 miles-per-hour for three hours, the distance [raveled is 120 miles. Write a program that asks for the speed of a vehicle (in miles-per-hour) and the number of hours it has traveled. It should use a loop to display the distance a vehicle has traveled for each hour of a time period specified by the user. For example, if a vehicle is traveling at 40 mph far a three-hour time period, it should display a report similar to the one chat follows: Hour Distance Traveled 1 90 2 100 3 150 Input Validation: Do not accept a negative number for speed and do not accept any value les than 1 for time traveled.arrow_forwardProgramming is Javaarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
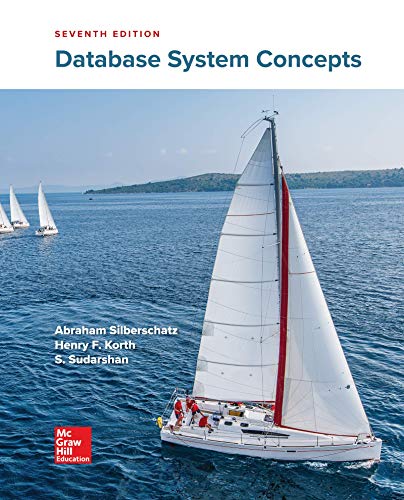
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
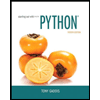
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
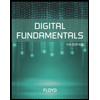
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
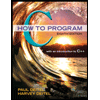
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
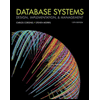
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
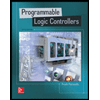
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education