Copy of CITS2401_Lab2
.pdf
keyboard_arrow_up
School
The University of Western Australia *
*We aren’t endorsed by this school
Course
2401
Subject
Computer Science
Date
Apr 3, 2024
Type
Pages
5
Uploaded by EarlManateeMaster1057
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
1/17
Before you edit something, Please select 'File' above, and
click 'Save a copy in Drive'
CITS2401 Lab2
NOTE: Please refer to the FAQ Q2.4
Q2.4: Can I ask questions (via Email or Discord) before the Lab?
We would like to ask Not to do it before the lab.
We always thank you for sharing good questions, but those questions can be answered during
the lab class. The reason why we share those lectures and labs (on Sunday) is for you to skim
through the topic we will cover.
So, please attend the lab ±rst and ask those questions during/after the lab to the teacher. So
your question can be easily answered as the teacher will go through with you.
We do understand you would like to know some content as soon as you can. But we have more
than 350 students in this unit and it will be really di²cult to manage all questions posted on
Discord if students start asking questions before attending actual lab sessions. Thanks for your
understanding!
Lab 2 Checklist
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
2/17
Play with Floor Divide and Modulo (refer Lecture 1, page 17)
0.[Recap] Floor divide and Modulo
We have learned the following seven operators: +
, -
, *
, /
, **
, //
, %
Among those operators, we will go through the following:
//
Floor divide. (Divide and round down)
%
Modulo. (Divide and return the remainder)
Try running the following code. Make sure you understand this!
height = 183
print("You are " + str(height // 100) + "m and " + str(height % 100) + " cm tall.")
1
2
height = 183
print("You are " + str(height // 100) + "m and " + str(height % 100) + " cm tal
You are 1m and 83 cm tall.
Let's try:
Task 1
: Write a program that performs exactly
like the following:
1
2
3
4
5
day = int(input('Enter a number of days: '))
weeks = int(day) // 7
days = int(day) % 7
print('That is {} weeks and {} days!'.format(weeks, days))
Enter a number of days: -5
That is -1 weeks and 2 days!
Task 2:
Guess what would be the output of the following (not executing the program):
1. What is -5 // 7
?
2. What is -5 % 7
?
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
3/17
1. -1
2. 2
Lists, Tuples, Sets, and Dictionary (Lecture 2, page 5 - 51)
1.Python Collections
There are four collection data types in the Python: List, Tuple, Set, and Dictionary.
Those are used to store multiple items in a single variable.
The table can be also found in the Lecture 2, page 34
.
Lists (Lecture 2, page 6 - 32)
1-1.Lists
Lists are created using square brackets [ ]
. Each object is called an element
or item
of the list
and each element/item
can be accessed using an index, starting from 0
. We can store multiple
different data types in a single list.
Lists are mutable
objects. This means that we can change their elements by accessing them
directly in an assignment statement. We can also add, change or remove elements from the list.
Since lists are indexed, we can store multiple items with the same value. The len()
function is
one of the commonly used methods and it can check the number of items in the list.
Part 1
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
4/17
Try out the following piece of code.
colour = ["red", "orange", "yellow", "green", "blue", "pink"]
print(colour[0])
Note:
Don't name your variable list
since it is a built-in function in Python!
1. Change the index to 1 or 2 to print out other elements.
2. Add a new item "purple"
to the list by using the append()
method. Print out your list to
check that it worked correctly.
3. What happens if you try print(colour[-1])
? What about other negative numbers, like -2
or -4?
4. Change "pink"
to "indigo"
so that you get a proper rainbow!
5. What happens if we print the element with index 7?
red
yellow
['red', 'orange', 'yellow', 'green', 'blue', 'pink', 'purple']
pink
['red', 'orange', 'yellow', 'green', 'blue', 'indigo', 'purple']
---------------------------------------------------------------------------
IndexError Traceback (most recent call last)
<ipython-input-14-84d903ef2ad0> in <cell line: 14>
()
12 print
(
colour
)
13 ---> 14 print
(
colour
[
7
])
IndexError
: list index out of range
SEARCH STACK OVERFLOW
1
2
3
4
5
6
7
8
9
10
11
12
13
14
colour = ["red", "orange", "yellow", "green", "blue", "pink"]
print(colour[0])
print(colour[2])
colour.append("purple")
print(colour)
print(colour[-2])
colour[5] = 'indigo'
print(colour)
Part 2
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
5/17
Try out the following piece of code.
word = ["n", "a", "n", "a"]
print(word)
1. Extract the second to fourth items in the word
list by using the print()
method.
2. Insert "b"
and "a"
at the beginning of the list so you can make it banana.
1
2
3
4
5
6
7
8
9
word = ["n", "a", "n", "a"]
print(word)
print(word[1: ])
word.insert(0, 'b')
word.insert(1, 'a')
print(word)
['n', 'a', 'n', 'a']
['a', 'n', 'a']
['b', 'a', 'n', 'a', 'n', 'a']
Tuples (Lecture 2, page 33 - 39)
1-2.Tuples
Tuples are written with round brackets ( )
. Tuple items are ordered, unchangeable, and allow
duplicate values. Tuple items are indexed, the ±rst item has index [0]
, the second item has
index [1]
etc.
Tuples are unchangeable, meaning that we cannot change, add or remove items after the tuple
has been created. Since tuples are indexed, they can have items with the same value. You can
check the number of items by using len()
.
Part 1
Try out the following piece of code.
myfamily = ("mother", "father", "sister", "brother", "sister")
print(myfamily)
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
6/17
1. Use type()
to check the type of the object myfamily
.
2. Access tuple items sister
by using index numbers.
3. Check whether we can add an item me
by using the append()
method.
4. Check whether we can remove the item brother
by using the pop()
method.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
myfamily = ("mother", "father", "sister", "brother", "sister")
print(myfamily)
print(type(myfamily))
print(myfamily[4])
x = list(myfamily)
x.append('me')
print(x)
x.pop(3)
y = tuple(x)
print(y)
('mother', 'father', 'sister', 'brother', 'sister')
<class 'tuple'>
sister
['mother', 'father', 'sister', 'brother', 'sister', 'me']
('mother', 'father', 'sister', 'sister', 'me')
Sets (Lecture 2, page 40 - 44)
1-3.Sets
Sets are written with curly brackets { }
. Set items are unordered, unchangeable (but mutable),
and do NOT allow duplicate values. Unordered means that the items in a set do not have a
de±ned order. Set items can appear in a different order every time you use them, and cannot be
referred to by index or key.
Sets are unchangeable but mutable, meaning that we cannot change the items after the set has
been created. Once a set is created, you cannot change its items, but you can add new items or
remove items.
Part 1
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
7/17
Try out the following piece of code.
fruit = {"apple", "pineapple", "grape", "apple"}
1. Use print()
to print all items in the set. Can you see all four items? or three items only?
Why?
2. Use type()
and check the type of the object fruit
. Is that what you expected?
3. Add a new item "grapefruit"
using the add()
method.
4. Remove all items from the set fruit
.
5. Again, use type()
and check the type of the object fruit
. Is that what you expected?
³. Create another object empty = {}
and check the type of the object by using type()
. Is
that what you expected?
1
2
3
4
5
6
7
8
9
10
11
12
fruit = {"apple", "pineapple", "grape", "apple"}
print(fruit)
print(type(fruit))
fruit.add('grapefruit')
fruit.clear()
print(type(fruit))
empty = {}
print(type(empty))
{'grape', 'apple', 'pineapple'}
<class 'set'>
<class 'set'>
<class 'dict'>
Dictionary (Lecture 2, page 45 - 50)
1-4.Dictionaries
Dictionaries are also written with curly brackets { }
. Where lists or tuples use numbered indexes
such as list[0]
to access their elements, dictionaries use keys to access their elements.
Dictionaries are used to store data values in key:value pairs. A dictionary is a collection which is
ordered* (
As of Python version 3.7, dictionaries are ordered. In Python 3.6 and earlier, dictionaries
are unordered.
), changeable and does not allow duplicates. In a dictionary, keys are unique so a
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
8/17
given key can only appear once. Dictionaries are changeable, meaning that we can change, add
or remove items after the dictionary has been created.
Part 1
Try out the following piece of code.
laptop = {
"brand": "dell",
"model": "alienware",
"year": 2010
}
1. Print the brand
value of the dictionary.
2. Add new information (
key: value
) by using a boolean
data type as value
and home
as key
:
This laptop is at home now, so "home": True
.
3. Modify the value of the key year
to 2019
.
*Additional Methods for extracting keys or values: keys()
and values()
Try and see how the output comes out.
1
2
3
4
5
6
7
8
9
10
11
laptop = {
"brand": "dell",
"model": "alienware",
"year": 2010
}
print(laptop['brand'])
laptop['home'] = True
print(laptop)
laptop['year'] = 2019
print(laptop)
dell
{'brand': 'dell', 'model': 'alienware', 'year': 2010, 'home': True}
{'brand': 'dell', 'model': 'alienware', 'year': 2019, 'home': True}
Part 2
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
9/17
Write a program that stores information about a user into a dictionary
, and performs exactly
as
follows: *Note
: The yellow-highlighted texts are things that the user types into the terminal.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
name = input('What is the user\'s name? ')
age = input('What is the user\'s age? ')
country = input('What is the user\s country of birth? ')
known = input('What is the user known for? ')
print('The user\'s data is: ')
user_data = {
'name': name,
'age': age,
'country': country,
'known_for': known
}
print(user_data)
What is the user's name? Zain
What is the user's age? 18
What is the user\s country of birth? Pakistan
What is the user known for? skills
The user's data is: {'name': 'Zain', 'age': '18', 'country': 'Pakistan', 'known_for': 'skills'}
2.Control Flow
Control Flow
Programs written so far execute sequentially
(e.g. execute Line 1 then Line 2 so on…)
We can control the ´ow of program by using:
Selection
statements: if, elif, else statements (this week)
Iteration
statements: while, for statements (lab 3)
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
10/17
Flowchart
shapes to represent intention.
Parallelogram – input/output to the program
Rectangle – assignment or computations
Diamond – decision
Arrows – direction of execution
if Statement (Lecture 2, page 54 - 56)
2-1.Control Flow: if
Control ´ow is an important topic in programming. It allows us to execute speci±c branches
depending on the current state of the program. Up until now, all of our programs executed
sequentially. In this week, we will learn how we can control the ´ow of the program by using
selection statements.
Control ´ow: if
statement
IF statement starts with keyword if
Colon symbol : denotes start of if
block
Body of the if
statement is indented with 4 spaces or a tab
Python Conditions and If statements
Python supports the usual logical conditions from mathematics. This will be used in several
ways, most commonly in selection and iteration.
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
11/17
if
takes in a single boolean. If it is True, its branch will execute.
Try the following code:
if 10 > 5: # Note the colon : symbol which denotes the start of a new block.
print("10 is indeed greater than 5.") # Code inside the block must be indented with 4
Note: Python does not accept ±les that mix spaces and tabs as indentation. You may receive
an IndentationError.
If you run into such an issue, look for a setting to make tabs and spaces
visible. Good editors will resolve all tabs to 4 spaces or vice versa, so you shouldn't run into
issues.
1
2
if 10 > 5: # Note the colon : symbol which denotes the start of a new block.
print("10 is indeed greater than 5.") # Code inside the block must be inden
10 is indeed greater than 5.
Part 1: Checking power level
(by using if statement only)
Write a program that asks the user for their power level. Make the program output a different
response depending on whether the user's power level is over 9000 or not (by using two if
statements)
*Note:
The yellow-highlighted texts are things that the user types into the terminal.
02/11/2023, 21:40
Copy of CITS2401_Lab2.ipynb - Colaboratory
https://colab.research.google.com/drive/1JzUhaMQ4sxQ_7AHRAJafh30eCZjRe-8Q#scrollTo=NykcBGJ4vc08&printMode=true
12/17
1
2
3
4
5
6
7
power = int(input('Vegeta, what does the scouter say about his power level? '))
if power > 9000:
print('IT\'S OVER NINE THOUSAND!!!')
if power < 9000:
print('it\'s not over 9000...')
Vegeta, what does the scouter say about his power level? 800
it's not over 9000...
if, else Statement (Lecture 2, page 57 - 61)
2-2.Control Flow: if-else
An if
statement is used to do some action(s) if the condition is true.
IF statement starts with keyword if
Colon symbol : denotes start of if
block
Body of the if
statement is indented with 4 spaces or a tab
The else
keyword catches anything which isn't caught by the preceding conditions.
else statement to do something else if the condition is false
No condition after the else statement
Note:
It is useful to draw ´owcharts to represent the control ´ow in our programs. Below is the
´owchart for a piece of code that includes an if statement
Part 1: If ... Else with a ²ow chart
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Download the text file titled Tweets-short.txt from the following URL and save it in your Jupyter directory folder: https://drive.google.com/open?id=1NJvqi-mgdnFTqbbtpsfRKUjtOZQSLKPKThe file includes 148 tweets(one per each line) containing the keyword election. Each tweet/line is either an original tweet or a retweet. A Retweet is identified by the term RT at the beginning of the line.Write a program that opens the text file and reads it line by line, then only if the tweet in a line is an original tweet (i.e., NOT a Retweet) AND contains the keyword "Biden" writes that on an output txt file. Name the output file [your_last_name].txt.
arrow_forward
FLEX hw
Please add commentaries inside the code
Create a flex file which recognizes if a given string is an email address.
arrow_forward
Using C# language please in Visual Studio 2022 in Windows Form App.
As for the folder-- for convenience sake-- create some folders and insert a couple of names in there, not 200.
arrow_forward
sam Verbena Farm Stand
COMPLETING A FLYER
GETTING STARTED
Open the file NP_WD16_la_FirstLastName_1.docx, available for download
from the SAM website.
Save the file as NP_WD16_la_FirstLastName_2.docx by changing the "1" |
to a "2".
If you do not see the .docx file extension in the Save As dialog box, do
not type it. The program will add the file extension for you automatically.
With the file NP_WD16_1a_FirstLastName_2.docx still open, ensure that
your first and last name is displayed in the footer.
O If the footer does not display your name, delete the file and download a
new copy from the SAM website.
PROJECT STEPS
1. You are helping to publicize a farm stand that sells organic herbs by creating a
flyer for the business. Begin by changing only the top and bottom margins of
the document to 0.5".
Apply the Fill - Green, Accent 1, Shadow text effect to the heading "Verbena
Farm Stand" to make the title of the flyer stand out.
2.
3.
Format the paragraph beginning "Verbena Farm grows…
arrow_forward
Create the file review.txt using “vi”Add text to “review tcmp$221.txt” as shown below 1. My name is 3. I am a junior 2. My major is CTRe arrange the lines according to line no’s using nanoWhat is the default permission of file “review tcmp$221.txt”Change the permission of the above file to 755
arrow_forward
Transcribed Image Text
/* you have a sceneraio for recruting app */ In which you have two types of Record type on Position Object one is for Technical and one is non technical Record type. you have to write a SOQL query to fetch All the reocrd type of the ID of Technical Record Type. Please Run the SOQL Query on the Anonymous Window. also please attach the screenshot of the output. Apex, Salesforce
arrow_forward
It's possible to open and edit four files called asiasales2009.txt if you create four file objects named asia, eu, africa or latin and use them to open and edit the files.
arrow_forward
only a,b,c
this is the pdf of the seq. you can convert to seq.txt format
file:///C:/Users/abdul/Downloads/SodaPDF-converted-seq%20(1).pdf
this is the codon and aminoacid list. you can convert to codon-aa.txt format
file:///C:/Users/abdul/Downloads/SodaPDF-converted-codon-aa-list.pdf
arrow_forward
FLEX hw
Please add commentaries inside the code
Create a flex file which counts how many words start with a capital letter.
arrow_forward
Save : You'll use this command to create a copy of a document while
keeping the original. When you use Save As, you'll need to choose a
different name and/or location for the copied version.
O True
O False
Word includes different options for customizing a table, including adding
rows or columns and changing the table style.
O True
False
If there is text you want to move from one area of the document to
another. you can copy and paste or drag and drop the text.
O True
False
arrow_forward
Thonny C\Users\iqueous fixxbuzz.py @ 12:5
File Edit View Run Tools Help
▸ 33. I STOP
beginl.py
1
2
3
4
5
8
fixxbuzz.py* X trials.py
def fizz():
print (number, "Fizz")
def buzz ():
print (number, "Buzz")
def FizzBuzz():
print (number, "FizzBuzz")
def nothing():
print (number)
for number in range(101):
if number % 3==0 and number % 5==0:
FizzBuzz()
elif number % 5==0:
buzz ()
elif number % 3==0:
fizz ()
nothing ()
else:
Type here to search
O
arrow_forward
close all;clear all;clc;current_script = mfilename('fullpath');script_directory = fileparts(current_script);file_name0 = 'data_00.csv';file_name1 = 'data_01.csv';file_name2 = 'data_02.csv';file_name3 = 'data_03.csv';data0 = csvread([script_directory '\' file_name0]);data1 = csvread([script_directory '\' file_name1]);data2 = csvread([script_directory '\' file_name2]);data3 = csvread([script_directory '\' file_name3]);avg_data = (data1 + data2 + data3) / 3;figure;% plot (data0(:,1), data0(:,2), 'b-', 'LineWidth', 2, 'DisplayName', 'Parabolic Curve');hold on;% plot(avg_data(:, 1), avg_data(:, 2), 'k-', 'Linewidth', 1, 'DisplayName', 'Average Data Points');plot(smooth_data(:, 1), smooth_data(:, 2), 'k-', 'Linewidth', 1, 'DisplayName', 'Smoothed Data');scatter(data1(:,1), data1(:,2), 5, 'r', 'filled', 'DisplayName', 'Sample Data Points 1');scatter(data2(:,1), data2(:,2), 5, 'g', 'filled', 'DisplayName', 'Sample Data Points 2');scatter(data3(:,1), data3(:,2), 5, 'y', 'filled', 'DisplayName',…
arrow_forward
close all; clear all; clc; current_script = mfilename('fullpath'); script_directory = fileparts(current_script);file_name0 = 'data_00.csv'; file_name1 = 'data_01.csv'; file_name2 = 'data_02.csv'; file_name3 = 'data_03.csv'; data0 = csvread([script_directory '\' file_name0]); data1 = csvread([script_directory '\' file_name1]); data2 = csvread([script_directory '\' file_name2]); data3 = csvread([script_directory '\' file_name3]); avg_data = (data1 + data2 + data3) / 3; figure; % plot (data0(:,1), data0(:,2), 'b-', 'LineWidth', 2, 'DisplayName', 'Parabolic Curve');hold on; % plot(avg_data(:, 1), avg_data(:, 2), 'k-', 'Linewidth', 1, 'DisplayName', 'Average Data Points'); plot(smooth_data(:, 1), smooth_data(:, 2), 'k-', 'Linewidth', 1, 'DisplayName', 'Smoothed Data'); scatter(data1(:,1), data1(:,2), 5, 'r', 'filled', 'DisplayName', 'Sample Data Points 1'); scatter(data2(:,1), data2(:,2), 5, 'g', 'filled', 'DisplayName', 'Sample Data Points 2'); scatter(data3(:,1), data3(:,2), 5, 'y',…
arrow_forward
Murach's javascript and Jquery 4th edition (ch9 animation)
I need help with this assignment, please. thanks
Modify a carousel to use animationIn this exercise, you’ll modify a carousel application so that when an image in the carousel is clicked, an enlarged image is displayed using animation.1. Open the application in this folder:carouselThen, run the application and notice that an enlarged image of the first book in the carousel is displayed.2. Review the HTML for the application, and notice that it contains an img element with an id of “image” following the heading. Also notice that the href attributes of the <a>elements in the carousel are set to the URL of the enlarged image to be displayed when the associated carousel image is clicked.3. Code an event handler for the click event of the <a> elements in the list. This event handler should start by getting the URL for the image to be displayed. Then, it should assign this URL to the enlarged image.4. Add animation to…
arrow_forward
M7Pro_Files
Upload your accounts python file here
What to do:
U are to create a report for users that will list their student id number, last name, and first name. Also, you will list their username and email address
The input file is linked below
studentInfo.txt
(opens in new window)
Note: You are to add YOUR first and last name in the first row ( instead fo Mr. Cameron's), the ID SHOULDN'T be your real ID, just a imaginary number there
The output file that your program will generate should look like the one linked below:
loginInfo.txt
(opens in a new window)
Given a text file , studentInfo.txt , linked above (or U can use one you created) that contains only last name( field size = 10) and first name(field size is 10) and student id numbers (field size is 7) you will create a report that will generate the usernames as well as student email accounts as shown in the loginInfo.txt linked above.
Notice that the login name consists of the first 7 characters of the last name, the…
arrow_forward
In this assignment, you have to write down a file comparison application. You can use this chapters codes to
create your file. In your first file, you have to add at least 10 accounts such as (please assume the name of
the file as orginal_account_file.txt)
ID
Name
Surname
Balance
Tel
еMail
1)
Tolga
Medeni
-6500
0555555555 tolgamedeni@ybu.edu.tr
In your second file you have to change Balance, Tel and eMail data of at least five records. In your code,
such as(please assume the file name as changed_account_file.txt):
ID
Name
Surname
Balance
Tel
eMail
1)
Tolga
Medeni
0999999999 tolgamedeni@gmail.com
"you have to display the changes in each record, and display as separate records to show changed and
unchanged records.
"Your code also have to create two separate files to save changed records and un-changed records.
Such as:
File_Unchanged_Records.txt
File_Changed_Records.txt
You have to upload only C. files, the given files have to create these four files (orginal_account_file.txt,…
arrow_forward
Extra 6-1 Develop the Temperature Converter
In this exercise, you’ll use radio buttons to determine whether the conversion is from Fahrenheit to Celsius or vice versa. You’ll also modify the DOM so the labels change when a radio button is clicked, and the page displays an error message when the user enters invalid data.
1. Open the application in this folder:
exercises_extrach06convert_temps
2. Note that the JavaScript file has some starting JavaScript code, including the $() function, three helper functions, three event handler functions, and a DOMContentLoaded event handler that attaches the three event handlers.
3. Review how the toCelsius() and toFarhenheit() event handler functions call the toggleDisplay() helper function and pass it strings to display. Also note that the toggleDisplay() helper function and the convertTemp() event handler function are incomplete.
4. Code the toggleDisplay() function so it changes the text in the labels for the text boxes to the values in the…
arrow_forward
Question Completion Status:
a) Mowngto anotner question wal save this response.
Question 7
$$
\left(\begin{array}{cccc}
-5 & 3 & 1 & 10 \\
5 & 5 & 4 & 6 \\
4 & -2 & 0 & -5
\end{array}\right)
$$
TTI Aruil
$\mathrm{- o phe -a -}$
mouter
SP.VS.049|
arrow_forward
please answer letter B on a jupyter notebook
using this csv file: https://drive.google.com/file/d/1-OknkD0AYhDzWy6sGx0A9cXZ4JxvkrNo/view?usp=sharing
arrow_forward
Da3 KAMJNBR8iYXU09d3c4RbEx_dB9OlGiw/viewform
. For which values of average does the code segment execute
0 69 F
70 79 C
80 -89 B
06
100 A
System.out.print("Enter an integer from 0 to 100: ");
int average = input.nextInt();
%3D
if(average < 100)
System.out.printin("Your grade is A");
else if(average < 90)
System.out.printin("Your grade is B");
else if(average < 80)
System.out.printin("Your grade is C");
else if(average <70)
System.out.printin("Your grade is F");
For all value in the proper [0... 100] range.
Only for values in the [0 .. 69] range.
Only for values in the [70... 100] range.
Only for values in the [90...
100] range.
only for values in the [90... 99] range.
arrow_forward
Search Algorithms
Dataset: We will use cars dataset as part of this project. The dataset is available as part of the assignment (cars.csv and a pdf that describe each of the fields for each record).
TASK 1
The idea of this project is to create a text-based application with the following options:
List all cars. This option will read from the file and when the user selects this option, the program will list all the cars in the file.
Find via Linear search. This option will allow the user to type a model of the car (ID field in the dataset) and it should print out the information about the car searched, the amount of time it took to find the car using linear search. For this option, create a class called SearchMethods and implement the method :
public static <T extends Comparable<T>> int linearSearch(T[] data, int min, int max, T target)
Find via Binary search. This option will allow the user to type a model of the car (ID field in the dataset) and it…
arrow_forward
Powerpoint VBA code that does this:
If the "Add List of Slide Titles" Checkbox is
checked, a list of all of the slides Titles should be
added to the new slide. (This is on Shapes(2) on
the new slide.) ***The answer should be VBA
code***
arrow_forward
Attached File: a2provided:
grades = [[71, 14, 41, 87, 49, 93],[66, 73, 54, 55, 94, 79],[20, 51, 77, 20, 86, 15],[79, 63, 72, 57, 63, 73],[30, 66, 78, 65, 41, 45],[11, 83, 51, 47, 68, 63],[99, 43, 85, 86, 24, 75],[67, 102, 11, 84, 64, 109],[16, 24, 101, 78, 55, 89],[100, 26, 37, 95, 106, 100],[55, 77, 30, 34, 28, 15],[87, 67, 13, 71, 67, 83],[95, 65, 94, 56, 15, 92],[102, 23, 36, 39, 60, 39],[90, 59, 96, 105, 83, 16],[101, 40, 17, 12, 44, 36],[39, 63, 96, 12, 65, 82],[95, 20, 105, 34, 69, 95],[95, 93, 75, 18, 105, 102],[35, 15, 82, 106, 73, 30],[80, 52, 67, 49, 11, 88],[86, 17, 44, 75, 78, 49],[22, 60, 74, 110, 92, 37],[13, 14, 15, 82, 75, 57],[71, 106, 15, 77, 30, 98],[43, 80, 76, 85, 102, 53],[26, 98, 60, 80, 104, 79],[12, 28, 40, 106, 88, 84],[82, 75, 101, 29, 51, 75],[20, 31, 84, 35, 19, 85],[84, 63, 56, 101, 83, 102],[25, 106, 36, 24, 61, 80],[86, 75, 89, 47, 28, 27],[38, 48, 22, 72, 53, 83],[70, 34, 46, 86, 32, 58],[83, 59, 38, 16, 94, 104],[62, 110, 13, 12, 13, 83],[90, 59, 55,…
arrow_forward
import numpy as np
import pandas as pd
URL= 'https://drive.google.com/uc?export=download&id=1iCti5uxMveZIu7ENCjAcGszFFCa3fqnn'
df=pd.read_csv(URL)
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
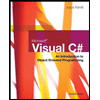
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
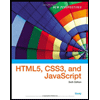
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L
Related Questions
- Download the text file titled Tweets-short.txt from the following URL and save it in your Jupyter directory folder: https://drive.google.com/open?id=1NJvqi-mgdnFTqbbtpsfRKUjtOZQSLKPKThe file includes 148 tweets(one per each line) containing the keyword election. Each tweet/line is either an original tweet or a retweet. A Retweet is identified by the term RT at the beginning of the line.Write a program that opens the text file and reads it line by line, then only if the tweet in a line is an original tweet (i.e., NOT a Retweet) AND contains the keyword "Biden" writes that on an output txt file. Name the output file [your_last_name].txt.arrow_forwardFLEX hw Please add commentaries inside the code Create a flex file which recognizes if a given string is an email address.arrow_forwardUsing C# language please in Visual Studio 2022 in Windows Form App. As for the folder-- for convenience sake-- create some folders and insert a couple of names in there, not 200.arrow_forward
- sam Verbena Farm Stand COMPLETING A FLYER GETTING STARTED Open the file NP_WD16_la_FirstLastName_1.docx, available for download from the SAM website. Save the file as NP_WD16_la_FirstLastName_2.docx by changing the "1" | to a "2". If you do not see the .docx file extension in the Save As dialog box, do not type it. The program will add the file extension for you automatically. With the file NP_WD16_1a_FirstLastName_2.docx still open, ensure that your first and last name is displayed in the footer. O If the footer does not display your name, delete the file and download a new copy from the SAM website. PROJECT STEPS 1. You are helping to publicize a farm stand that sells organic herbs by creating a flyer for the business. Begin by changing only the top and bottom margins of the document to 0.5". Apply the Fill - Green, Accent 1, Shadow text effect to the heading "Verbena Farm Stand" to make the title of the flyer stand out. 2. 3. Format the paragraph beginning "Verbena Farm grows…arrow_forwardCreate the file review.txt using “vi”Add text to “review tcmp$221.txt” as shown below 1. My name is 3. I am a junior 2. My major is CTRe arrange the lines according to line no’s using nanoWhat is the default permission of file “review tcmp$221.txt”Change the permission of the above file to 755arrow_forwardTranscribed Image Text /* you have a sceneraio for recruting app */ In which you have two types of Record type on Position Object one is for Technical and one is non technical Record type. you have to write a SOQL query to fetch All the reocrd type of the ID of Technical Record Type. Please Run the SOQL Query on the Anonymous Window. also please attach the screenshot of the output. Apex, Salesforcearrow_forward
- It's possible to open and edit four files called asiasales2009.txt if you create four file objects named asia, eu, africa or latin and use them to open and edit the files.arrow_forwardonly a,b,c this is the pdf of the seq. you can convert to seq.txt format file:///C:/Users/abdul/Downloads/SodaPDF-converted-seq%20(1).pdf this is the codon and aminoacid list. you can convert to codon-aa.txt format file:///C:/Users/abdul/Downloads/SodaPDF-converted-codon-aa-list.pdfarrow_forwardFLEX hw Please add commentaries inside the code Create a flex file which counts how many words start with a capital letter.arrow_forward
- Save : You'll use this command to create a copy of a document while keeping the original. When you use Save As, you'll need to choose a different name and/or location for the copied version. O True O False Word includes different options for customizing a table, including adding rows or columns and changing the table style. O True False If there is text you want to move from one area of the document to another. you can copy and paste or drag and drop the text. O True Falsearrow_forwardThonny C\Users\iqueous fixxbuzz.py @ 12:5 File Edit View Run Tools Help ▸ 33. I STOP beginl.py 1 2 3 4 5 8 fixxbuzz.py* X trials.py def fizz(): print (number, "Fizz") def buzz (): print (number, "Buzz") def FizzBuzz(): print (number, "FizzBuzz") def nothing(): print (number) for number in range(101): if number % 3==0 and number % 5==0: FizzBuzz() elif number % 5==0: buzz () elif number % 3==0: fizz () nothing () else: Type here to search Oarrow_forwardclose all;clear all;clc;current_script = mfilename('fullpath');script_directory = fileparts(current_script);file_name0 = 'data_00.csv';file_name1 = 'data_01.csv';file_name2 = 'data_02.csv';file_name3 = 'data_03.csv';data0 = csvread([script_directory '\' file_name0]);data1 = csvread([script_directory '\' file_name1]);data2 = csvread([script_directory '\' file_name2]);data3 = csvread([script_directory '\' file_name3]);avg_data = (data1 + data2 + data3) / 3;figure;% plot (data0(:,1), data0(:,2), 'b-', 'LineWidth', 2, 'DisplayName', 'Parabolic Curve');hold on;% plot(avg_data(:, 1), avg_data(:, 2), 'k-', 'Linewidth', 1, 'DisplayName', 'Average Data Points');plot(smooth_data(:, 1), smooth_data(:, 2), 'k-', 'Linewidth', 1, 'DisplayName', 'Smoothed Data');scatter(data1(:,1), data1(:,2), 5, 'r', 'filled', 'DisplayName', 'Sample Data Points 1');scatter(data2(:,1), data2(:,2), 5, 'g', 'filled', 'DisplayName', 'Sample Data Points 2');scatter(data3(:,1), data3(:,2), 5, 'y', 'filled', 'DisplayName',…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Microsoft Visual C#Computer ScienceISBN:9781337102100Author:Joyce, Farrell.Publisher:Cengage Learning,Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageNew Perspectives on HTML5, CSS3, and JavaScriptComputer ScienceISBN:9781305503922Author:Patrick M. CareyPublisher:Cengage Learning
- COMPREHENSIVE MICROSOFT OFFICE 365 EXCEComputer ScienceISBN:9780357392676Author:FREUND, StevenPublisher:CENGAGE L
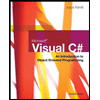
Microsoft Visual C#
Computer Science
ISBN:9781337102100
Author:Joyce, Farrell.
Publisher:Cengage Learning,
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
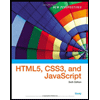
New Perspectives on HTML5, CSS3, and JavaScript
Computer Science
ISBN:9781305503922
Author:Patrick M. Carey
Publisher:Cengage Learning
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:9780357392676
Author:FREUND, Steven
Publisher:CENGAGE L