WrittenReport02
.docx
keyboard_arrow_up
School
University of Utah *
*We aren’t endorsed by this school
Course
3200
Subject
Computer Science
Date
Apr 3, 2024
Type
docx
Pages
20
Uploaded by UltraRoseArmadillo41
CS 3200-001
Spring 2024
Instructor:
Martin Berzins
Duke Nguyen
u1445624
February 13, 2024
Assignment #2 – Written Report
(Due date
: February 11, 2024 by 11:59PM)
What to turn in:
For the assignments, we expect both SOURCE CODE
and a written
REPORT be uploaded as a zip or tarball file to Canvas.
•
Source code for all programs that you write, thoroughly documented.
o
Include a README file describing how to compile and run your code.
•
Your report should be in PDF format and should stand on its own.
o
It should describe the methods used.
o
It should explain your results and contain figures.
o
It should also answer any questions asked above.
o
It should cite any sources used for information, including source code.
o
It should list all of your collaborators.
This homework is due on February 11
th
at 11:59 pm. If you don't understand these directions, please feel free to email the TAs or come see one of the TAs or the instructor during office hours well in advance of the due date.
1
Problem 1:
Question 5.10 of Cleve Moler’s book
(50 points)
I initially sort a set of (x, y) data points in ascending order of their x-values and then to
create a scatter plot of these sorted points. This line sorts the rows of the matrix yy based on the
values in its second column. The matrix yy is assumed to contain data points, with each row
representing a point. The second column likely contains the x-values of these points. After this
line, yy will be rearranged so that its rows are in ascending order of the x-values.
% Sort the data based on x values
sortrows(yy, 2);
sorted_x = yy(:, 2);
sorted_y = yy(:, 1);
% Plot the sorted data
figure;
plot(sorted_x, sorted_y, '*'
);
xlabel(
'x'
);
ylabel(
'y'
);
title(
'Sorted and Plotted Data'
);
grid on
;
2
(a)
(10 points)
As your first experiment, use the code to read this data file and sort it and
plot it . You should see something like the figure of sample output below.
The first line sorts the rows of the matrix yy based on the values in its second column.
The matrix yy is assumed to contain data points, with each row representing a point. The second
column likely contains the x-values of these points. After this line, yy will be rearranged so that
its rows are in ascending order of the x-values. The next two lines extract the second and first
column of the now-sorted matrix yy and stores it in the variable sorted_x and sorted_y
respectively. Those columns represent the x and y-values of the data points, now in sorted order.
Use the plot method, a graph is obtained as below:
Figure 1.1:
A graph plotting the given data after being sorted
3
Using the formula above, the sorted data points are now scaled in the range [-1,1] by
using the below snipper of codes.
% Linear scaling for x and y
x_min = min(sorted_x);
x_max = max(sorted_x);
y_min = min(sorted_y);
y_max = max(sorted_y);
scaled_y = (2 * sorted_y) / (y_max - y_min) - (y_max + y_min) / (y_max - y_min);
scaled_x = (2 * sorted_x) / (x_max - x_min) - (x_max + x_min) / (x_max - x_min);
Hence, we will obtain an updated version of the graph.
Figure 1.2:
A graph plotting the same data set after being sorted and scaled
4
(b)
(10 points)
Use a linear scaling to put both x and y values in the range [-1,1] such as
y
i
=
2
(
y
max
−
y
min
)
y
i
−
(
y
max
+
y
min
)
(
y
max
−
y
min
)
The below code is structured to execute polynomial fitting at varying degrees,
specifically for degrees 5, 10, 15, 20, and 25. It caters to a dataset containing 82 points, with the
data already scaled to fit within the range of -1 to 1. The process involves creating a
Vandermonde matrix for each polynomial degree, which is then used to perform least squares
fitting on the scaled data. This fitting process computes the polynomial coefficients that best
approximate the scaled data in a least squares sense.
For each polynomial degree, it evaluates the fitted polynomial over a collection of 1001
points in range from -1 to 1. This evaluation helps visualize how well the polynomial fits the data
across this range. The results are plotted, showing both the original data points and the
corresponding polynomial fit, thus providing a clear visual representation of the fitting quality. % Degree of the polynomial
m_values = [5, 10, 15, 20, 25];
n = 82; % Number of data points
% Evaluate the polynomial at 1001 points
xplot = linspace(-1, 1, 1001);
% Loop over each degree m
for m = m_values
5
(c)
(20 points)
Write a program that uses the Monomial Vandermode matrix
approximation to produce a least squares approximation to work with this data set. In
this case n
= 82 as there are 82 data points and m is the degree of the polynomial with
m + 1
terms. Use values of m
equal to 5, 10, 15, 20, 25. Plot the values of the
polynomial by evaluating the Vandemonde polynomial at (say) 1001 points and use
plots to show how the different polynomials behave. Contrast the visual appearance of
the polynomial in each of the five cases m
= 5, 10, 15, 20, 25. Use the supplied
regression code on the canvas page as a model if you find that helpful. An easier option is to use the matlab functions polyfit
and polyval
. If you
use these you will not be able to estimate the condition numbers of the matrices.
Instead MATLAB will tell you that the matrix is ill-conditioned.
% Create Vandermonde matrix for fitting
A = ones(n, m+1);
for j = 2:m+1
A(:, j) = scaled_x.^(j-1);
end
% Perform least squares fitting
B = A' * A;
c = A' * scaled_y;
a = B \ c; % Polynomial coefficients
% Create Vandermonde matrix for evaluation
Aplot = ones(length(xplot), m+1);
for j = 2:m+1
Aplot(:, j) = xplot.^(j-1);
end
% Evaluate the polynomial
yFit = Aplot * a;
% Plot the data and the polynomial
figure;
plot(scaled_x, scaled_y, '*'
, xplot, yFit, '-'
);
xlabel(
'x'
);
ylabel(
'y'
);
title([
'Polynomial Fit with m = '
, num2str(m)]);
grid on
;
legend(
'true values'
,
'best fit line'
)
NKK
end
The code block above performs polynomial fitting using the Vandermonde matrix and
least squares method for different polynomial degrees (5, 10, 15, 20, and 25) on a set of 82 data
points that have been scaled to the range [-1, 1]. For each specified degree, the code constructs a Vandermonde matrix based on the scaled
x-values, solves the least squares problem to determine the polynomial coefficients, and then
evaluates these polynomials at 1001 evenly spaced points within [-1, 1]. Each resulting
polynomial is plotted against the scaled data, allowing for visual comparison of the fit quality
across different polynomial degrees. This procedure illustrates how increasing the polynomial degree affects the fit to the data,
highlighting the trade-off between fitting the data closely and potentially overfitting, especially
with higher degrees.
The graphs obtained for each degree of polynomial regression are as follow:
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Assessment Description
Honors Students: Complete this assignment according to the directions provided in the "Honors Addendum," located in Class Resources.
This activity has multiple parts. All parts must be completed prior to documentation submission.
Part 1: Reading and Writing Text Files.
In this activity, you will learn how to read and write text files using non buffered and buffered File I/O classes, then learn how to parse a String into tokens using the String split() method, and finally, how to handle exceptions using various approaches.
Part 2: Reading and Writing JSON Files.
In this activity, you will learn how to read, write, and serialize JSON files to and from Java Objects.
Refer to the instructions within "Activity 4 Guide" to complete the assignment.
APA style is not required, but solid academic writing is expected.
This assignment uses a rubric. Please review the rubric prior to beginning the assignment to become familiar with the expectations for successful…
arrow_forward
Assignment 2 Directions
Please choose Option 1 or Option 2. You do not need to do both.
Option 1:
Please write a short paper based on Module 2 Hardware Components. Anything in the module can be a topic.
Write a summary paper as follows:
• Prepare a Word document with a minimum of 3 pages (not including title and references pages) and a minimum of 3
solid references.
Be sure to use APA Guidelines for references.
• Make sure to save the file in the following format: FirstName_LastName_Paper.doc(x)
• Upload the completed file as an attachment to this assignment.
.
Option 2:
1. Create an algorithm to add two integer numbers.
2. Implement the program in a high level language like C or Java. It does not need to run for me, but the code should
be included in a text document called FirstnameLastnameHLA2.txt in your assignment submission.
3. Implement the program in MIPSzy Assembly language. Use the high level code as comments to the right of the
Assembly code as the textbook does.
4. If you…
arrow_forward
help fins the corect asnwer please
arrow_forward
Python
arrow_forward
Create a simple program for a quiz
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
Create a folder named LastName_FirstName (ex. Dahan yoon) in your local
Create a new project named LabExer5B. Set the project location to your own
The program shall:
contain an array of 10 multiple choice questions with three (3) choices each and
require the user to choose among A, B, or C;
Note: Cases are ignored. Lowercase letters are acceptable (a, b, c).
Create a try-catch structure to handle three (3) exceptions. These are when the user inputs the following:
an invalid letter (not A, B, or C)
a number or any special character
blank (no answer)
Prompt the user that he can answer again if any of the three (3) exceptions is
Display the score.
arrow_forward
File
Edit
View
Sign
Window
Help
ECS401U_210571675.pdf
ECS401U_210571... x
16 / 29
125%
罗ア白 ,班
a) Compare and contrast in your own words the way reading from a file using a
File Reader is done in Java with keyboard input using a scanner illustrating your
answer using the following example code fragments.
Note you are not being asked to explain line by line what the code does but use it
to help explain the points made in your answer.
public static void keyboardInput ()
}
new Scanner(System.in);
Scanner scanner
String s;
System.out.println("Please input the data");
s = scanner.nextLine();
System.out.println(s );
return;
{
public static void fileRead () throws IOException
BufferedReader inputStream
new BufferedReader (new FileReader("data.csv"));
inputStream.readLine();
String s =
System.out.println(s);
inputStream.close();
return;
{
arrow_forward
Python
Required information
id fname lname company address city ST zip
103
Art
Venere
8 W Cerritos Ave #54
Bridgeport
NJ
8014
104
Lenna
Paprocki
Feltz Printing
639 Main St
Anchorage
AK
99501
arrow_forward
Java:
OverviewIn this task, you will read data from a text file, process the loaded data, and then save the processed data to a new text file. The purpose is to teach you to read from and save to text files.TaskStatistics department in Germany has made forecasts of what Germany's population will look like by 2050, distributed by age and gender. The text file (population2050.txt) contains statistics on the estimated population for the year 2050. The figure below shows the first 16 lines of the file.
First comes a line that shows which age group the information applies to. This is followed by a row with information on the number of men in that age group, followed by a row with the number of women in that age group. In total, the file contains information for the age groups 0 to 105 and the information about each age group extends over three lines as shown. The rows with information about the number of men and women are formatted using one or more spaces.
Your task is to write a java…
arrow_forward
create a script that will parse data from Rotten Tomatoes, a movie reviews website. The work you have to do is identical to what we covered in lectures 4 and 5, albeit for a different website. Please read and follow the instructions below very carefully.
Step 1
Your script should begin by defining two variables (after importing libraries, etc)
movie a string variable indicating the movie for which reviews will be parsed
pageNum the number of review pages to parse
For example, to parse the first 3 pages of the Gangs of New York reviews, set movie ‘’gangs_of_new_york” and pageNum = 3. Your code should go to the movie’s All Critics reviews page of rotten tomatoes, and parse the first three pages of reviews. Pagination on rotten tomatoes happens by clicking on the “Next” button.
Step 2
For each review contained in each of the pages you requested, parse the following information
The critic This should be 'NA' if the review doesn't have a critic’s name.
The rating. The rating…
arrow_forward
..
arrow_forward
Attached Files:
File Rooms.txt (427 B)
Imagine we were creating a Textbased Adventure Program where a user can navigate through a series of rooms and pick up items, use them, put them down and get points. This assignment has us just reading the rooms of a game from a file into our program and displaying the rooms, not building the entire game.
Create a new Java Project. Name it TestReadRoomFile or something like that.
Add a Room class to the project according to the following UML.
Room
-roomNumber: int
the room number
-roomName: String
the name of the room
-description: String
the description of the room
+Room(roomNumber: int, roomName:String, description:String)
Constructor. Assign the parameters to the instance variables.
+getRoomName():String
returns the name of the room
+getRoomNumber(): int
returns the room number
+getDescription(): String
returns the description
+toString():String
returns a string with all the room information.
Create a ReadRoomFile class similar…
arrow_forward
Lab Tasks – complete these
Use the information provided here, in the slideshow and in chapter 12 of our text to do the following exercise.
Step 1: On the desktop or wherever you will be saving your java program, use notepador other basic text editor (NOT WORD) to create a .txt file. In this file, type out a list of 10 integers (one on each line or with a space between each). Save. Do this manually. Not with Java.
Step 2. Now write a program with the following:
1) In the program creating a File object that connects to the .txt file that you just created.
2) Add code that will check if the file exists and if it doesn’t, it will create it. (Yes, we know it definitely exists but do this part anyway.)
3) Set up a Scanner so that you can read the values from the file and print them to the screen using a loop. You will probably need to use the .hasNext() method. Also, you may want to set up a counter to keep track of how many values there are for future use. (Note that the .length()…
arrow_forward
how to correct this exercise.
COP2002: Programming Logic & Design with PythonProject 4Project: Download and save the file named proj_4_to_fix_wishes.py. The program has five errors including a syntax error, runtime errors, and logic errors. Find and fix all the errors. You must also document the errors you found and how you fixed them. Since you can only upload a single file into Canvas, put the explanation of the errors at the top of the program, as comments. The output of your fixed program should look as shown:Sample Console Display:
Notes• You are not required to validate if the user enters a character or a string. The only validation is the one in the program: the user must enter values equal to or greater than 0.• You do not need to validate the user's response to the question, "Enter an item? y/n". If the user enters anything other than 'y' or 'n' the program can simply end.• Save the corrected file with the filename xxx_wishes_fixed.py (where xxx = your initials) or as…
arrow_forward
C++ Visual Studio 2019
Instructions
Complete #1 & #2. Please submit just one file for the classes and main to test the classes. Just create the classes before main and submit only one cpp file. Do not create separate header files. Please note: the deleteNode needs to initialize the *nodePtr and the *previousNode. The code to do this can be copied from here: ListNode *nodePtr, *previousNode = nullptr;
arrow_forward
It should be designed as a Desktop Application (with GUI) developed with Java programming language.Note that you are not designing a Web Application or a Mobile Application.Create a project named “FavoriteBooks” which keeps a list of the books that a user has read and liked.This list is stored in a text document (.txt) file and for each book it keeps the following data separated by acomma (,):Book id, Title, Category, First Author Name and Surname, Award Winner, Rating, Year, Number of Pages, andShort Description. Book id: The data type of “Book id” is integer and will be incremented automatically by 1 whenever anew book is added to the list. Title: The data type of “Title” is String. Category: The data type of “Category” is String. It shows the category of a Book such as Arts, Biography,History, Science Fiction, Cookbook, Literature, etc.. First Author Name and Surname: The data type of “Author Name and Surname” is String. Award Winner: The data type of “Award” is String. If the…
arrow_forward
shell scripting
arrow_forward
Required information
id fname lname company address city ST zip
103
Art
Venere
8 W Cerritos Ave #54
Bridgeport
NJ
8014
104
Lenna
Paprocki
Feltz Printing
639 Main St
Anchorage
AK
99501
arrow_forward
The names and student numbers of students are save in a text file called stnumbers.txt. Example of the content of the text file:
Peterson
20570856
Johnson
12345678
Suku
87654321
Westley
12345678
Venter
87654321
Mokoena
79012400
Makubela
29813360
Botha
30489059
Bradley
30350069
Manana
30530679
Shabalala
28863496
Smith
87873909
Nilsson
30989698
Makwela
30256607
Govender
30048117
Ntumba
30598303
Ramsamy
29952239
Skosana
29982995
Jameson
30228484
Xulu
29092248
Wasserman
27469352
Bester
28615425
Babane
27154033
Maboya
29897890
Mahlangu
30031338
Majavu
30165970
Myene
30954177
Motaung
30907276
Ramaroka
30804507
Radebe
30007674
Sekake
30017416
Zwane
30038227
Shuro
30238072
Viljoen
28881389
Sithole
45688555
Write a function called displayData() to receive the array and number of elements as parameters and display the names and student numbers of the students with a heading and neatly spaced.
Write a function, isValid(), which receives a number as parameter and determines whether the number…
arrow_forward
JavaScript Loops
Create an HTML
document called
assignment2.html
that implements a webpage that
dynamically creates (using JavaScript code) a 15X15 HTML table and displays in each
cell the (row, column) information for that cell. Allow the user to select the number of
rows and columns to redraw a new table.
Follow these guidelines to implement your assignment:
1.
Create a JavaScript file called assignment2.js and write the code for a function
that draws the table.
2.
Invoke the function when the visitor clicks on the assignment2.html page.
3.
Load the assignment2.js file at the end of the body in assignment2.html.
4.
(
10% of the grade
) Accept user input for the number of rows and columns in the
table.
5.
(
10% of the grade
) Use good web design practices to enhance visually your
html page. Add a title, picture, colors, copyright line, etc.
arrow_forward
Using C# (Sharp) with Visual studio:
Make a class of Book with 5 properties - ISBN, author, title, publisher, copyright date
In the main method, create 5 instances of the Book class with different data for each
Create a LIST and add each of the 5 objects to the LIST
Use a foreach loop to display the ISBN, author, title, publisher, and copyright date in the LIST
Create a DICTIONARY and add each of the 5 objects (key should be ISBN)
Display author, title, publisher, copyright date for each book
Create a SEARCH feature: ask the user to type in an ISBN. If the entry matches an item in your dictionary, display the author, title, publisher, and copyright date. If there are no matches, display "ISBN not found". Hint: use ContainsKey
Create a REMOVE feature: ask the user to type in an ISBN to remove a book from the list. If the entry matches an item in your dictionary, remove the item and re-display your list. If there are no matches, display "ISBN not found".
arrow_forward
Attached Files:
File Items.txt (119 B)
Imagine we were creating a Textbased Adventure program and our Rooms in the assignment above could hold Items. We are not writing the complete game, but lets write a program that will build the Item.txt file.
Create a new project. Add a class called Item that stores information about an item that can be placed in a room. An item has a name, a description and the room number in which the item is initially is stored. LAMP, a brightly shining lamp, 3 is an example of data that an Item might contain. Using the UML model below, create the private data members and methods for constructing an item.
Create an ItemFileWriter class with a static method to write the items from the given ArrayList to a text file.. The items text file will hold the item name, item description and the initial room that the item is to be loaded into, on seperate lines followed by a blank line. An example of the file that is to be constructed is attached.
Construct a…
arrow_forward
A Comma Separated Value (CSV) text file called movies.txt is located in the directory of your Eclipse Project.( If your Eclipse Project name is Assignment2, the text file is located under Assignment2, NOT src folder)
It contains data for one movie on each line.Each Movie contains the following data items:Title(String), Year(int-4 digits), Runtime(double).
Example:
Gemini Man,2019,1.97
Mortal Engines,2018,2.01
Write Java program(s) to do the following:1) Read the movies.txt Text file and write the data as a Serialized file called movies.ser to the local directory(same as movies.txt).2) Read the movies.ser serialized file and output the data on to the Console in the format shown below.
NOTE: DO NOT just write out the data from movies.txt using System.out.println(). This will receive no credit. You MUST read the data from the movies.ser file and output the data.We will check the movies.ser file for grading.
The spacing for the output is: Title(20), Year (8) and Runtime (10.2)Title and…
arrow_forward
Lab Assignment 2: Playfair Cipher
Overview: You are required to use the Playfair cipher to encrypt the message: "Butch." You will
do the encryption by hand. The key for encryption is “Govern." You will need to show your work
for the encryption process. Your submission should be a typed text file
arrow_forward
Using C# language please in Visual Studio 2022 in Windows Form App.
As for the folder-- for convenience sake-- create some folders and insert a couple of names in there, not 200.
arrow_forward
Please i want DRAWif you can not draw it in picture
leave it to someone else
i need only photo
arrow_forward
#this is a python programtopic: operation overloading, Encapsulation
please find the attached image
arrow_forward
Program a python program that demonstrates reading and comparing
Create a python program that demonstrates reading and comparing multiple files, processes the input files based on the business rules, and produces an output file.
Needed Files
1. The timeclock.txt Download timeclock.txtfile contains the hours worked data for every employee as well as the shift they worked. The layout for the file is as follows: EMPLOYEE_NUMBER, HOURS_WORKED, SHIFTTimeclock.txt
22476, 45, 3
24987, 30, 148283, 50, 285437, 25, 3
2. The personnel.txt Download personnel.txtfile contains the names of all employees and their hourly pay rates. The layout for the file is as follows: EMPLOYEE_NUMBER, EMPLOYEE_NAME, PAY_RATEPersonnel.txt
11235, Bob Smith, 10.50
22476, John Roberts, 12.3524987, Mary Johnson, 15.9032743, Brad Carson, 11.2548283, Alice Martin, 14.2085437, Aaron James,15.15
Note: Both the timeclock.txt and payroll.txt files are pre-sorted by EMPLOYEE_NUMBER in ascending order.
Business…
arrow_forward
Rooms.txt (427 B)
Imagine we were creating a Textbased Adventure Program where a user can navigate through a series of rooms and pick up items, use them, put them down and get points. This assignment has us just reading the rooms of a game from a file into our program and displaying the rooms, not building the entire game.
Create a new Java Project. Name it TestReadRoomFile or something like that.
Add a Room class to the project according to the following UML.
Room
-roomNumber: int
the room number
-roomName: String
the name of the room
-description: String
the description of the room
+Room(roomNumber: int, roomName:String, description:String)
Constructor. Assign the parameters to the instance variables.
+getRoomName():String
returns the name of the room
+getRoomNumber(): int
returns the room number
+getDescription(): String
returns the description
+toString():String
returns a string with all the room information.
Create a ReadRoomFile class similar to the OOP example…
arrow_forward
8
File
Help
Tell me what you want to do
Home Insert Design Layout References Mailings Review View
iGET GENUINE OFFICE Your license isn't genuine, and you may be a victim of software counterfeiting. Avoid interruption and keep your files safe with genuine Office today.
Page 1 of 1
80 words
Accessibility: Investigate
CS309
CS309 ASSIGNMENT 9 - Word
A process description for "Pay Commission" is provided below:
For each COMMISSION EARNED
If EXTRA BONUS equals Y
|
-If PAYMENT TOTAL is greater than $80,000
Add 3% to COMMISSION PERCENT
Output SPECIAL LETTER
Output AWARD LIST
Else Add 2% to COMMISSION PERCENT
Output AWARD LIST
Else
If PAYMENT TOTAL is greater than $80,000
Add 1% to COMMISSION PERCENT
Output SPECIAL LETTER
Calculate COMMISSION COMMISSION PERCENT times PAYMENT TOTAL
Required:
Construct a Decision Table for the above process description.
delinki asylum
Get genuine Office
Learn more
F
+
+
X Q
X
100%
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
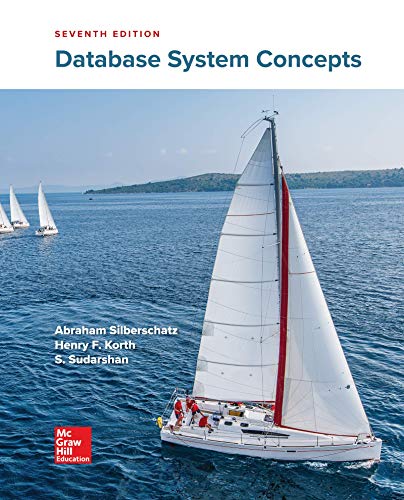
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
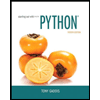
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
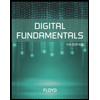
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
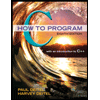
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
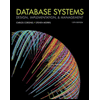
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
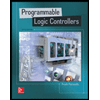
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
Related Questions
- Assessment Description Honors Students: Complete this assignment according to the directions provided in the "Honors Addendum," located in Class Resources. This activity has multiple parts. All parts must be completed prior to documentation submission. Part 1: Reading and Writing Text Files. In this activity, you will learn how to read and write text files using non buffered and buffered File I/O classes, then learn how to parse a String into tokens using the String split() method, and finally, how to handle exceptions using various approaches. Part 2: Reading and Writing JSON Files. In this activity, you will learn how to read, write, and serialize JSON files to and from Java Objects. Refer to the instructions within "Activity 4 Guide" to complete the assignment. APA style is not required, but solid academic writing is expected. This assignment uses a rubric. Please review the rubric prior to beginning the assignment to become familiar with the expectations for successful…arrow_forwardAssignment 2 Directions Please choose Option 1 or Option 2. You do not need to do both. Option 1: Please write a short paper based on Module 2 Hardware Components. Anything in the module can be a topic. Write a summary paper as follows: • Prepare a Word document with a minimum of 3 pages (not including title and references pages) and a minimum of 3 solid references. Be sure to use APA Guidelines for references. • Make sure to save the file in the following format: FirstName_LastName_Paper.doc(x) • Upload the completed file as an attachment to this assignment. . Option 2: 1. Create an algorithm to add two integer numbers. 2. Implement the program in a high level language like C or Java. It does not need to run for me, but the code should be included in a text document called FirstnameLastnameHLA2.txt in your assignment submission. 3. Implement the program in MIPSzy Assembly language. Use the high level code as comments to the right of the Assembly code as the textbook does. 4. If you…arrow_forwardhelp fins the corect asnwer pleasearrow_forward
- Pythonarrow_forwardCreate a simple program for a quiz Software Requirements: Latest version of NetBeans IDE Java Development Kit (JDK) 8 Procedure: Create a folder named LastName_FirstName (ex. Dahan yoon) in your local Create a new project named LabExer5B. Set the project location to your own The program shall: contain an array of 10 multiple choice questions with three (3) choices each and require the user to choose among A, B, or C; Note: Cases are ignored. Lowercase letters are acceptable (a, b, c). Create a try-catch structure to handle three (3) exceptions. These are when the user inputs the following: an invalid letter (not A, B, or C) a number or any special character blank (no answer) Prompt the user that he can answer again if any of the three (3) exceptions is Display the score.arrow_forwardFile Edit View Sign Window Help ECS401U_210571675.pdf ECS401U_210571... x 16 / 29 125% 罗ア白 ,班 a) Compare and contrast in your own words the way reading from a file using a File Reader is done in Java with keyboard input using a scanner illustrating your answer using the following example code fragments. Note you are not being asked to explain line by line what the code does but use it to help explain the points made in your answer. public static void keyboardInput () } new Scanner(System.in); Scanner scanner String s; System.out.println("Please input the data"); s = scanner.nextLine(); System.out.println(s ); return; { public static void fileRead () throws IOException BufferedReader inputStream new BufferedReader (new FileReader("data.csv")); inputStream.readLine(); String s = System.out.println(s); inputStream.close(); return; {arrow_forward
- Python Required information id fname lname company address city ST zip 103 Art Venere 8 W Cerritos Ave #54 Bridgeport NJ 8014 104 Lenna Paprocki Feltz Printing 639 Main St Anchorage AK 99501arrow_forwardJava: OverviewIn this task, you will read data from a text file, process the loaded data, and then save the processed data to a new text file. The purpose is to teach you to read from and save to text files.TaskStatistics department in Germany has made forecasts of what Germany's population will look like by 2050, distributed by age and gender. The text file (population2050.txt) contains statistics on the estimated population for the year 2050. The figure below shows the first 16 lines of the file. First comes a line that shows which age group the information applies to. This is followed by a row with information on the number of men in that age group, followed by a row with the number of women in that age group. In total, the file contains information for the age groups 0 to 105 and the information about each age group extends over three lines as shown. The rows with information about the number of men and women are formatted using one or more spaces. Your task is to write a java…arrow_forwardcreate a script that will parse data from Rotten Tomatoes, a movie reviews website. The work you have to do is identical to what we covered in lectures 4 and 5, albeit for a different website. Please read and follow the instructions below very carefully. Step 1 Your script should begin by defining two variables (after importing libraries, etc) movie a string variable indicating the movie for which reviews will be parsed pageNum the number of review pages to parse For example, to parse the first 3 pages of the Gangs of New York reviews, set movie ‘’gangs_of_new_york” and pageNum = 3. Your code should go to the movie’s All Critics reviews page of rotten tomatoes, and parse the first three pages of reviews. Pagination on rotten tomatoes happens by clicking on the “Next” button. Step 2 For each review contained in each of the pages you requested, parse the following information The critic This should be 'NA' if the review doesn't have a critic’s name. The rating. The rating…arrow_forward
- ..arrow_forwardAttached Files: File Rooms.txt (427 B) Imagine we were creating a Textbased Adventure Program where a user can navigate through a series of rooms and pick up items, use them, put them down and get points. This assignment has us just reading the rooms of a game from a file into our program and displaying the rooms, not building the entire game. Create a new Java Project. Name it TestReadRoomFile or something like that. Add a Room class to the project according to the following UML. Room -roomNumber: int the room number -roomName: String the name of the room -description: String the description of the room +Room(roomNumber: int, roomName:String, description:String) Constructor. Assign the parameters to the instance variables. +getRoomName():String returns the name of the room +getRoomNumber(): int returns the room number +getDescription(): String returns the description +toString():String returns a string with all the room information. Create a ReadRoomFile class similar…arrow_forwardLab Tasks – complete these Use the information provided here, in the slideshow and in chapter 12 of our text to do the following exercise. Step 1: On the desktop or wherever you will be saving your java program, use notepador other basic text editor (NOT WORD) to create a .txt file. In this file, type out a list of 10 integers (one on each line or with a space between each). Save. Do this manually. Not with Java. Step 2. Now write a program with the following: 1) In the program creating a File object that connects to the .txt file that you just created. 2) Add code that will check if the file exists and if it doesn’t, it will create it. (Yes, we know it definitely exists but do this part anyway.) 3) Set up a Scanner so that you can read the values from the file and print them to the screen using a loop. You will probably need to use the .hasNext() method. Also, you may want to set up a counter to keep track of how many values there are for future use. (Note that the .length()…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
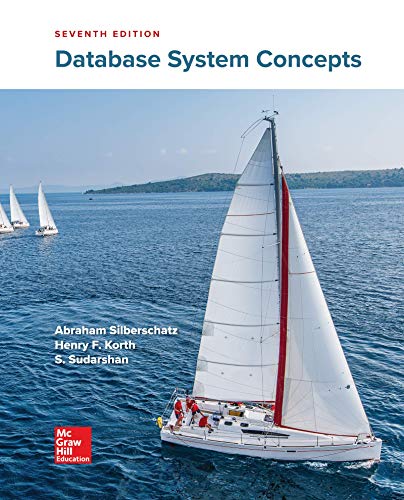
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
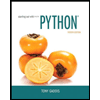
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
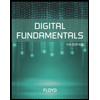
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
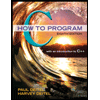
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
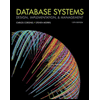
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
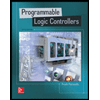
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education