LaraBaltazarPA2
.docx
keyboard_arrow_up
School
Washington State University *
*We aren’t endorsed by this school
Course
325
Subject
Computer Science
Date
Apr 3, 2024
Type
docx
Pages
2
Uploaded by JusticeCrab4670
Lara Baltazar
Programming Assignment 2
Chapter 2, Programming Exercise 14: Compound Interest
Due September 10, 2023 at 6:00pm
Description of the assignment:
Create a program that would allow users to calculate the future value from a loan accruing compounded interest. This would be solved using user input for the principle, interest rate, compounded interest terms per year, and years compounded. The interest rate should be entered as a percentage.
Program design:
Chapter 2, Programming Exercise 14. Compound Interest
Use the following values for the variables to test the program and illustrate input and output results:
p = $1,000.00
r = 5%
n = 12
t = 10 years
Pseudocode:
Get the starting principle, annual interest rate, compounding per year and years compounded from user input.
Convert the interest rate as a percentage into decimal form.
Calculate the future value and return the output.
Python program code:
#Get starting principle
principle = float(input("Enter the starting principle: "))
#Get annual interest rate
interest_rate = float(input("Enter the annual interest rate as a percentage: "))
#Get compounding per year
compounding = float(input("How many times per year is the interest compounded?: "))
#Get years compounded
time = float(input("For how many years will the account earn interest?: "))
#Calculate interest
interest = interest_rate/100
#Calculate future value
future_value = principle*((1+(interest/compounding))**(compounding*time))
print(f"At the end of {time:,.0f} years, you will have ${future_value:,.2f}.")
Program input/output:
Any errors encountered and how they were diagnosed/resolved:
At first, the output was returned as $1,000. This was because of a calculation error in the formula. After solving said error, the program returned the correct amount.
Applied learning with business context/takeaways:
This assignment taught me how to:
1.
Use the f-input command.
2.
Adjust the number of digits being displayed to the right of a decimal point in the output of said f-input command.
3.
Use different types of operations, including exponents as well as complex formulas within Python.
Within a business context, this assignment simulates a situation in which I would need to code a program that can seamlessly calculate compound interest on a savings account or a loan.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
PROGRAMMING LANGUAGE: C# (Visual Studio .NET Framework)
Please include the output picture, code thanks.
arrow_forward
PROGRAM REQUIREMENTS:You are tasked to create a program that will compute for the salary of an employee for a week. The program should be based on the following requirements:1. An employee works for a regular of 40 hours a week. Beyond that is considered an over time. For example, an employee worked for 48 hours in that week, it means he has an 8-hour over time and he is entitled to an overtime pay.2. The overtime rate is 25% of the rate per hour of an employee and the overtime pay is computed as number of overtime hours multiplied by the overtime rate per hour.3. The gross pay of an employee is its salary without deductions. It is calculated by multiplying the rate per hour and the regular number of hours worked added with overtime pay. 4. Each employee is entitled to several benefits such as SSS, Pagibig and Philhealth. These benefits are deducted to the gross pay of an employee.5. The SSS contribution is computed as follows:Gross pay SSS<=5, 000 Php105.005, 001 – 10, 000 5% of…
arrow_forward
Problem Definition: Create and design a program that will accept employee’s information such as employee number, employee name, employee status (T-Tenured / P-Probitionary / C-Contractual), employee position title, basic salary, year end bonus and year hired.
Program Specifications:
Create and design a Menu Option for Employee Information with an ASCII Art.
A Python class/object concept must be apply to perform the following individual method:
Data Entry. Create the Python Methods to accept employee’s information.
Salary Entry. Designed a screen message to accept employee’s salary.
Gross salary = hours worked multiply (160 hrs) by rate per hour
Year End Bonus = Annual Salary * Bonus % (see table below)
Annual Salary = Gross salary multiply by 12 months
Display Information. Designed the employee’s information pay slip.
Consider the following criteria of employees’ salary:
Years of Service
Year Bonus
Employee (rate per hour)
Employee Status
1
10%…
arrow_forward
Process Scheduling: Select all statements below that are true
The waiting time is included in the turnaround time.
In interactive systems, short response times are unimportant.
The time taken in an interactive program from the issuance of a command to the
commencement of a response to that command is known as the response time.
The response time is the time required for a particular process to complete, from
submission time to completion.
Throughput is the number of processes executed per time unit.
The concept of virtual (run)time in the Completely Fair Scheduler is used to
ensure fairness in the allocation of processor capacity.
arrow_forward
Programming language C#
arrow_forward
Assignment 1/
Reset to Starter Code
2. Problem 2: Product ID Numbers Saved
Problem Description
In this second problem, you will build on and extend the program created while solving the first problem. You will be expected to isolate both the product id number and company number from the barcode number of the item to be purchased by the customer.
Input
You will receive (from the user) the following as input (in order):
• The first and last name of the customer (e.g., Eric Poitras)
• The item to be purchased, identified through a barcode number (e.g., 1909238092)
• The cost of the item to be purchased by the customer (e.g., 8.50)
• The cash amount given by the customer (e.g., 10.00)
Processing
1. Extract the product id and company id numbers
2. Print the data stored in each variable in the corresponding format
Output
1. Customer (Last, First): {LAST} {FIRST}
2. Item Number (Product, Company): {PRODUCT} {COMPANY}
3. Item Cost: (COST}
4. Cast Amount: {CASH}
Examples
Input
Output
Eric…
arrow_forward
Data Structure and Algorithms Laboratory question C++ language please solve asap
Pls send me solution fast within 20 min and i will rate instantly for sure
Solution must be in typed form
arrow_forward
c++ task
arrow_forward
The code is complete with output
arrow_forward
The language that must be used is C#
arrow_forward
Case Study – Depreciation Calculator
Design and develop a solution with functions (modules) and control structures for a program
that, given an original value, a depreciation percentage and a number of years, calculates the
depreciated value after the number of years entered. Show the depreciation and current value
at the end of each year.
Original Value : $ 8000
Depreciation percentage (%)
: 20
Number of year : 3
DEPRECIATION TABLE
Beginning Value
8000.00
6400.00
Depreciation
1600.00
Year
Current Value
1
6400.00
1280.00
5120.00
5120.00
1024.00
4096.00
The program should allow the user to repeat this process as many times as the user likes. Also,
to make your program more robust and avoid problems at run time, do as much status/error
checking as you can in your program.
Q2.
Construct a structure chart with data flow and execution of conditional and loops to
represent the hierarchical structure of modules. Note that the design of the hierarchical
structure of modules should be…
arrow_forward
1. Create a Salary Computation using python programming
2. Create 3 Modules
3. The first module is GrossSalary.py will handle the function for computing the gross salary.
4. The second module is SalaryDeductions.py will handle the function for computing the deductions
5. The last module NetSalary.py will be responsible for computing the net salary.
6. The user will input the following (Name, Hour, Loan, Health Insurance).
7. Tax(12% of the gross salary) and Rate(500/hr) is fixed
arrow_forward
C# simple beginner program!
Create a comment header containing information: Course & section, semester, author, assignment number, the name of the game, abstract. A section declaring your variables and comment the section. Use easy-to-read variable names and follow the naming convention. Comment variables that could be hard to understand
Your basic IF game provides the following to the player:
Display the course information: Course & section, semester, author, assignment#, INSTRUCTIONS
Ask for the player’s name
Ask for three or more choices/decisions
Display the choices selected, or the win/lose description at the end
arrow_forward
Please explain the steps of the coding attached.
arrow_forward
Design a program that will perform critical calculations for a logistics firm. Thelogistics firm uses two different types of Vehicles; Truck and Ship.Purpose of the program is to display whether or not a transportation vehicle (Truck orShip) will be able to perform the transportation task.In order to evaluate, your program will check the distance of the route to be taken, the weight ofthe cargo laden and the total amount of initial fuel the transportation vehicle is initially loaded.If the route, fuel and cargo parameters lead to the conclusion that the vehicle is able to do the tripwith no problems, inform the user that the transportation phase does not require any refuels and itcan be done in one trip. Otherwise, if the program detects that the vehicle is unable to perform thetransportation with the amount of fuel it is loaded in one trip or none at all, inform the user thatthe vehicle cannot perform the transportation and requires a refuel.Information about the consumption rate of…
arrow_forward
Basic Computer Programming ActivityLanguage: CShow the code and how it works thanks
arrow_forward
Quick Answer please
arrow_forward
Code using java programming language.
arrow_forward
Draw flow chart of this program
arrow_forward
PYTHON
Q9: Assignment statements, such as x = 3, define variables in programs. To execute
one in an environment diagram, record the variable name and the value:
Evaluate the expression on the right side of the = sign
Write the variable name and the expression's value in the current frame.
Use these rules to draw a simple diagram for the assignment statements below.
x = 10 % 4
y = x
x **= 2
arrow_forward
Python
arrow_forward
QUESTION:
Malaysia has introduced the household group based on the income classification which is B40, M40
and T20 as indicated in Figure 1. As a programmer, you are required to create a program that can
classify the household group and calculate household income tax.
Median Income
Income Range
Household Group
(RM)
(RM)
В1
1,929
Less than 2,500
B2
2,786
2,500 - 3,169
В40
B3
3,556
3,170 - 3,969
B4
4,387
3,970 - 4,849
M1
5,336
4,850 - 5,879
M2
6,471
5,880 - 7,099
M40
M3
7,828
7,110 - 8,699
M4
9,695
8,700 - 10,959
T1
12,586
10,960 - 15,039
T20
T2
19,781
15,039 or more
*Source: Household Income and Basic Amenities Survey Report 2019, Department of Statistics Malaysia
Figure 1: Household group based on the income range.
Based on the household income range given above, the following tax applies to all Malaysians
according to their respective household group as shown in Table 1.
Table 1: Applicable tax based on the household group.
Household Group Tax Applied (%)
B1
0%
B2
0%
B3
1.8%
В4
2.0%…
arrow_forward
Q # 1.
Implement a Result Compilation System for CP Course. Program will take
Marks of 3 Quizzes, 2 assignments, one midterm and one final term from the
user as input. Marks of each quiz will be out of 15, marks of each assignment
will be out of 40. Marks of Midterm will be out of 60 and Final term out of
80. Program will compute total marks in the subject according to the
following grading scheme and display on the screen.
Category
Weightage
1
Quizzes
25%
Assignments
15%
3
Mid-term
20%
4
Final-term
40%
Total Marks
100
2.
arrow_forward
Question 1
Money Exchange wants to develop a program to display how much Malaysian Ringgit (RM) would be required to buy British Pound (Pound), US Dollar (USD), Australian Dollar (AUD) and Saudi Riyal (Riyal). The exchange rates are as in the Table 1 below.
The staff can choose the listed foreign currency. The numbers 1, 2, 3 and 4 would be used to represent Pound, USD, AUD, Riyal respectively. They will key in the currency amount and the program will display how much Malaysian Ringgit (RM) would be required to buy the chosen foreign currency. Use constant to fix the exchange rates.
Table 1: Exchange Rate
Foreign Currency Units [=1 Malaysian ringgit]
Rate
1 Pound Sterling (Pound)
5.81
1 US Dollar (USD)
4.12
1 Australian Dollar (AUD)
3.23
1 Saudi Riyal (RIYAL)
1.10
Use the following functions :
double get_input( ) //to receive input for the selected foreign currency
double foreign_currency( ) //to determine the RM required for the selected foreign…
arrow_forward
python
arrow_forward
Computer Science
: Create a simple loan calculator system. You are
free to use any IDE you like. Here are the
requirements: • The user should be prompted to
enter the following fields: • Loan amount •
Interest rate • Number of years • The program
should output: • The monthly payment amount
• The total amount paid back • The compound
interest • The user should have the option to
display a table of all payments with the
following headers: • Payment no. • Amount •
Interest • Principal • Remaining Balance
arrow_forward
Output for Sample Input 1
3+
Copy
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
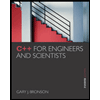
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
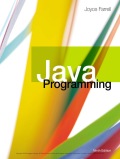
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Related Questions
- PROGRAMMING LANGUAGE: C# (Visual Studio .NET Framework) Please include the output picture, code thanks.arrow_forwardPROGRAM REQUIREMENTS:You are tasked to create a program that will compute for the salary of an employee for a week. The program should be based on the following requirements:1. An employee works for a regular of 40 hours a week. Beyond that is considered an over time. For example, an employee worked for 48 hours in that week, it means he has an 8-hour over time and he is entitled to an overtime pay.2. The overtime rate is 25% of the rate per hour of an employee and the overtime pay is computed as number of overtime hours multiplied by the overtime rate per hour.3. The gross pay of an employee is its salary without deductions. It is calculated by multiplying the rate per hour and the regular number of hours worked added with overtime pay. 4. Each employee is entitled to several benefits such as SSS, Pagibig and Philhealth. These benefits are deducted to the gross pay of an employee.5. The SSS contribution is computed as follows:Gross pay SSS<=5, 000 Php105.005, 001 – 10, 000 5% of…arrow_forwardProblem Definition: Create and design a program that will accept employee’s information such as employee number, employee name, employee status (T-Tenured / P-Probitionary / C-Contractual), employee position title, basic salary, year end bonus and year hired. Program Specifications: Create and design a Menu Option for Employee Information with an ASCII Art. A Python class/object concept must be apply to perform the following individual method: Data Entry. Create the Python Methods to accept employee’s information. Salary Entry. Designed a screen message to accept employee’s salary. Gross salary = hours worked multiply (160 hrs) by rate per hour Year End Bonus = Annual Salary * Bonus % (see table below) Annual Salary = Gross salary multiply by 12 months Display Information. Designed the employee’s information pay slip. Consider the following criteria of employees’ salary: Years of Service Year Bonus Employee (rate per hour) Employee Status 1 10%…arrow_forward
- Process Scheduling: Select all statements below that are true The waiting time is included in the turnaround time. In interactive systems, short response times are unimportant. The time taken in an interactive program from the issuance of a command to the commencement of a response to that command is known as the response time. The response time is the time required for a particular process to complete, from submission time to completion. Throughput is the number of processes executed per time unit. The concept of virtual (run)time in the Completely Fair Scheduler is used to ensure fairness in the allocation of processor capacity.arrow_forwardProgramming language C#arrow_forwardAssignment 1/ Reset to Starter Code 2. Problem 2: Product ID Numbers Saved Problem Description In this second problem, you will build on and extend the program created while solving the first problem. You will be expected to isolate both the product id number and company number from the barcode number of the item to be purchased by the customer. Input You will receive (from the user) the following as input (in order): • The first and last name of the customer (e.g., Eric Poitras) • The item to be purchased, identified through a barcode number (e.g., 1909238092) • The cost of the item to be purchased by the customer (e.g., 8.50) • The cash amount given by the customer (e.g., 10.00) Processing 1. Extract the product id and company id numbers 2. Print the data stored in each variable in the corresponding format Output 1. Customer (Last, First): {LAST} {FIRST} 2. Item Number (Product, Company): {PRODUCT} {COMPANY} 3. Item Cost: (COST} 4. Cast Amount: {CASH} Examples Input Output Eric…arrow_forward
- The language that must be used is C#arrow_forwardCase Study – Depreciation Calculator Design and develop a solution with functions (modules) and control structures for a program that, given an original value, a depreciation percentage and a number of years, calculates the depreciated value after the number of years entered. Show the depreciation and current value at the end of each year. Original Value : $ 8000 Depreciation percentage (%) : 20 Number of year : 3 DEPRECIATION TABLE Beginning Value 8000.00 6400.00 Depreciation 1600.00 Year Current Value 1 6400.00 1280.00 5120.00 5120.00 1024.00 4096.00 The program should allow the user to repeat this process as many times as the user likes. Also, to make your program more robust and avoid problems at run time, do as much status/error checking as you can in your program. Q2. Construct a structure chart with data flow and execution of conditional and loops to represent the hierarchical structure of modules. Note that the design of the hierarchical structure of modules should be…arrow_forward1. Create a Salary Computation using python programming 2. Create 3 Modules 3. The first module is GrossSalary.py will handle the function for computing the gross salary. 4. The second module is SalaryDeductions.py will handle the function for computing the deductions 5. The last module NetSalary.py will be responsible for computing the net salary. 6. The user will input the following (Name, Hour, Loan, Health Insurance). 7. Tax(12% of the gross salary) and Rate(500/hr) is fixedarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Programming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageC++ for Engineers and ScientistsComputer ScienceISBN:9781133187844Author:Bronson, Gary J.Publisher:Course Technology PtrEBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
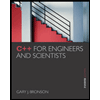
C++ for Engineers and Scientists
Computer Science
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Course Technology Ptr
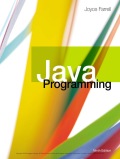
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT