CIS1500_Assignment3_Description_updated
.pdf
keyboard_arrow_up
School
University of Guelph *
*We aren’t endorsed by this school
Course
1300
Subject
Computer Science
Date
Apr 3, 2024
Type
Pages
12
Uploaded by MajorThunderIbex35
1) CIS1500 Assignment 3: Student Finance Calculator
a)
Total Marks:
10 marks
b)
Weight:
10%
c)
Due:
Friday, 1st Dec 2023, at 11:59 pm
Welcome to your last CIS1500 assignment! Please ensure you read all the sections and
follow the
instructions exactly
as we will grade your submission with an auto-grader. You must
test your
assignment on the SoCS Linux server
, and it will be similarly graded on the SoCS Linux server for
consistency.
Your program should only use concepts already taught in this course.
You will receive a 0 if your code
includes: global variables
(variables declared outside of functions),
goto, malloc, calloc, regex, or if
your code fails to compile without errors.
Note there are already two global
constants
provided for you
in the .h file.
This pdf goes in conjunction with videos available on courselink.
There is also a file containing “Skeleton Code”; you should use this as your starting point. It contains 3
files:
2)
A .c file for your main called
skeleton.c. You must rename this before submitting.
3)
A .c file for your functions called
A3_Functions.c. Do
not
rename this before submitting.
4)
A .h file for function prototype called
A3_Functions.h. This file is already completed, do not
modify it.
You do not need to submit it.
This document has 15 pages. Read them all and understand the requirements.
REMEMBER: You are to submit
one zip file containing two source code files
for your program.
●
The name of the
zip file
follows the following format:
studentNumberA3.zip
o
Example:
John
Snow’s
student
number
is
1770770
then
the
zip
file
name
is
1770770A3.zip
●
The two files within the zip you are to submit are non-compiled code C files (ends with .c)
containing your code
o
Do not include any other files or folders in your submission; they will be ignored.
o
Do not include this file inside another folder; it will be ignored by the auto-grader.
●
The name of the
C file
containing your main follows the following format:
studentNumberA3.c
o
Example: John Snow’s student number is 1770770 then the C file name is 1770770A3.c
●
The name of the C file containing your functions is A3_Functions.c
●
Incorrect submission will result in a grade of 0.
●
More details are outlined in Section 4: Program Submission and Administration Information.
Start Early. Get Help Early. Test Often.
1. Background
Please refer to the A1 and A2 outline for background, as this assignment is heavily based on A2. You
need to thoroughly understand both assignment outlines. In this assignment we will make 3 major
changes:
●
A menu is displayed asking the user what the wish to do.
●
Finance data is located in files, they can be loaded from files to view, or new files can be
created with the program.
●
We can change the “budget rules”, whereas previously we had hardcoded a 50/30/20 rule,
it can now be set and changed to bette
●
When finance data is loaded, it is kept in a struct that is provided. We are able to store
finance information for multiple people/years so an array of structs is required.
The output of your program should look very similar to that of A2 with three exceptions:
●
We use a menu system for the user to select options.
●
We request a name and year for finance data in addition to the actual finance information.
This program is intended to test your ability to:
●
Use structs, pass them to functions, return from functions, and modify the members.
●
Read and write data from/to text files.
This is an example of the contents of the data file. We have provided multiple sample files for you to test
your code with. When testing we will only use valid input files, with the exact format as those provided.
The course and assignment are not intended as actual finance advice.
2. Program Requirements
Your program will start with a welcome message, then prompt the user to select an option from a menu.
The menu requires the user to enter an integer value associated with the member option.
i)
2.1 Required Functions and Menu
This assignment has 5 options, each requiring 1 or two mandatory functions:
1)
Read finances from file.
This option will allow the user to enter a filename, which will load the data from that associated file. The
filename is passed into the function
FinanceData readFinanceDataFromFile(char filename[]);
which
will create a FinanceData struct, set the members of the struct to the appropriate values, and then return
the struct. Most of the data for the members are stored in the file, but some values (ex. monthly_funds)
may need to be calculated within the function. If a file cannot be loaded the message “Error: File not
found\n” is displayed.
Below is an example of the program output
2)
Enter finances and save to file
If the user selects option 2, they are prompted to enter a filename. Then the function
FinanceData
readFinanceDataFromUser();
is called which asks the name and year for the finances. Then the
questions from A2 are asked to obtain finance information. This information is all loaded into a newly
created struct, and that struct is returned. Remember that some of the struct members will require you to
perform calculations based on the input.
After the struct is created and added to an array of structs for future use, the struct is passed to the
function
void saveFinanceRecordToFile(FinanceData fd, char filename[MAX_STRING_LEN]);
which saves the data the filename recorded previously.
3)
Enter budget rules (ex. 50/30/20).
While previously we always followed
50/30/20 rules - for expenses, wants, and
savings - in A3 the user may choose to
select new ratios. So a user may instead opt
to have ratios 70/30/0 for 70% of funds
going to expenses, 30% for wants, and 0%
for savings.
To do this you will need variables that
store these rations, and use the function
void getBudgetRules(int
*essentials_ratio, int *wants_ratio, int
*savings_ratio);
which takes the ratios as
a reference and modifies them based on the
user input.
*** Hints:
- I recommend starting with option 2. It is
separated into two parts, you can copy a lot
of your A2 code for reading input and
create the struct. After you have tested that
and other features, you can work on saving
the struct to a file later.
- Next I would work on option 4, this
option mostly uses existing code that we
provided, and gives you a chance to verify
the output.
- Thirdly I would work on option 3. Now
that
you
have
the
ability
to
print
the
finance data you can verify that it works.
-
Remember
that
you
can
add
print
statements for testing wherever you need.
This is often the easiest and most effective
way to test that your code is doing what
you think, and helps tremendously with
debugging.
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Course Title : Operating SystemQuestion :
How you will create new user using terminal in Ubuntu? Add screenshots of all steps for new user creation including terminal and Login page in Answer sheet. Every student has to create new user as of his/her arid number for example (18-arid-2929).
Note: screenshots are mandatory.
arrow_forward
add tags for these feature files with --tags option:
When you select scenarios by one tag
When you select scenarios that have one or another tag
When you select scenarios that have 2 tags
When you disable scenarios that have a specific tag
Given Scenarios:
Feature: Login functionalityBackground:Given a web browser is at the BrainBucket login pageScenario: user can't login without entering emailGiven User is not logged inWhen Password is enteredAnd User click Login buttonThen 'Warning: No match for E-Mail Address and/or Password' will be shownScenario: user can recover his passwordGiven User is not logged inWhen User clicks 'Forgotten Password' buttonAnd enters his emailThen Message 'An email with a confirmation link has been sent your email address.' will be dispalyed
arrow_forward
Write a report on Linux on mobile phones. Report must cover the following: Introduction to embedded Linux, How Linux is being used on mobile phones, what phones are using Linux, what modifications have been projects for mobile phones.
Note:
Reports should be at least 5‐7 pages. Your report must include diagrams and cite references.
Failing to cite references will lead to loss of marks
DO NOT COPY DIRECTLY FROM SOURCE BUT WRITE IN YOUR OWN WORDS
arrow_forward
Powershell Commands
Create two groups for two teams in your company (server development) and (software testing). There are 3 members in each team need to create. They are (Jaspreet, Lina and Hanh) for the server development team, and (Nina, David and Rahul) for the software testing team. Each member has to have some role description. Lina temporarily left so her account must be disabled. Rahul left the company to a new job. Lina came back to work so her account must be enabled again. The company decided to change the name of the server development to (software development).
arrow_forward
using a code in Linux As a developer, a local Library approaches you and requests you to develop a library management system for them. Some of the requirements stated by the library are:
The Admin should be able to create accounts for different users of the system
Librarian
User
Do everything that all types of users can do
The Program should be menu driven,
The Librarian should have the following on their menu:
Insert a book (in a book_Records)
View Issued Books
Display all books available on the system
Search for a book (in a book_Records)
Delete a book
A Registered User should be able to
Search for a book,
Issue a book
Display all books available
Unregistered User should be able to :
Display all books in the library
Search for a book
The book_Records file should be auto-updated after Insertion and Deletion
Every book in the system should have the following details :
Book_id
Book_name
Author
Publishing_house
Year_of_publishing
arrow_forward
I need help with the part below and please add comments in the code to help me understand better what I am doing wrong in my own code.
I have already created my database and the connection and some of the code but am stuck on these parts."Home" and "Create Account" pages
The index.php file will ask the user to log in to the system with a login form
If they're already logged in, the home page will NOT show the login form. It will show a "welcome to our store" type of message instead even if they click on the "home" button in the navigation.
The create-account.php page has them fill out a form for creating an account.
Use real-time JavaScript validation (and regex) to:
Verify that the password and "verify password" match as they are typing.
Verify that the password contains at least one number and is at least 8 characters long
Be sure to display user feedback messages on the screen as they type, e.g.:
Password must contain a number
Password must be 8 characters long
Password and…
arrow_forward
Discuss in You are working on a class assignment in GeorgiaView. You have created a folder on your PC for the class and a subfolder for this assignment. It has a 'start file' that you downloaded and saved to that subfolder. You have completed your work on this file and uploaded it to GeorgiaView (you have not saved it since the original download).
What did you upload? Explain your reasoning. Your answer should include references to the various types of computer storage.
arrow_forward
Objective:
At the end of the exercise, the students should be able to:
Create an adjacency list in Java.
■
Software Requirements:
NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
1. Create a folder named LastName_FirstName in your local drive. (ex. Reyes_Mark)
2. Using NetBeans, create a Java project named AdjacencyList. Set the project location to your own
folder.
3. Create an adjacency list based on the given graph.
B
A
E
D
F
G
arrow_forward
i want you to draw a er model dont give me this draws all tutors answred by it :(i want new one only photos
arrow_forward
Why I am getting this error in stio() ?
I am using Visual Studion 2019
arrow_forward
choose 3 different Linux flavors - also called "distros" (distributions) - and research them. Write a summary paragraph about each one, for a total of 3 paragraphs. Include a references section at the end of your assignment with active links to all the sources you use.
Notes:
Don't use Ubuntu.
arrow_forward
Medical Unlimited Medical Unlimited (the brainchild of a group of young philanthropic medical professionals) is interested in setting up a mobile medical unit that provides free medical services for students. The mobile medical unit will require a patient record and scheduling application that will be used by the medical staff (doctors/nurses) and receptionists working there. The receptionists will use the system to capture new patient information when firsttime patients visit the doctor. Receptionists will also be responsible for scheduling all appointments. Nurses on the other hand will use the s ystem to keep track of the results of each visit including diagnosis, medications, prescription and treatment. Multiple medications may be prescribed during each visit. The nurses can also access the information to print out a history of patient visits. Th e doctors will primarily use the system to view patient history. The doctors may enter some patient treatment information and…
arrow_forward
Medical Unlimited Medical Unlimited (the brainchild of a group of young philanthropic medical professionals) is interested in setting up a mobile medical unit that provides free medical services for students. The mobile medical unit will require a patient record and scheduling application that will be used by the medical staff (doctors/nurses) and receptionists working there. The receptionists will use the system to capture new patient information when firsttime patients visit the doctor. Receptionists will also be responsible for scheduling all appointments. Nurses on the other hand will use the s ystem to keep track of the results of each visit including diagnosis, medications, prescription and treatment. Multiple medications may be prescribed during each visit. The nurses can also access the information to print out a history of patient visits. Th e doctors will primarily use the system to view patient history. The doctors may enter some patient treatment information and…
arrow_forward
A-List
Objective:
At the end of the exercise, the students should be able to:
▪ Create an adjacency list in Java.
Software Requirements:
▪ NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
1. Create a folder named LastName_FirstName in your local drive. (ex. Reyes_Mark)
2. Using NetBeans, create a Java project named AdjacencyList. Set the project location to your own
folder.
3. Create an adjacency list based on the given graph.
B
A
с
E
D
F
G
arrow_forward
Create a simple program for a quiz bee.
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
1. Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local drive.
2. Create a new project named LabExer5B. Set the project location to your own folder.
3. The program shall:
contain an array of 10 multiple choice questions with three (3) choices each and
require the user to choose among A, B, or C;
Note: Cases are ignored. Lowercase letters are acceptable (a, b, c).
4. Create a try-catch structure to handle three (3) exceptions. These are when the user inputs the
following:
• an invalid letter (not A, B, or C)
• a number or any special character
• blank (no answer)
5. Prompt the user that he can answer again if any of the three (3) exceptions is thrown.
6. Display the score.
arrow_forward
Clean and Build will remove intermediate files generated by a previous build and will
build every file in your project:
not specified by the makefile
only if it has changed since the last build
regardless of whether or not it has changed since the last build
Page 4 of 9
arrow_forward
Login.feature file has following scenarios:
@negative
Scenario: User can't login without entering password
Given user is not logged in
When user enters email
And user clicks Login button
Then warning is shown No match for E-Mail Address and/or Password
@negative @skip
Scenario: User can't login without entering email
Given user is not logged in
When user enters password
And user clicks Login button
Then warning is shown No match for E-Mail Address and/or Password
Do the following:
Combine those 2 Scenarios in 1 Scenario Outline
Implement step for a new Scenario Outline
arrow_forward
Laboratory Exercise Guessing Game 2
Objective:
At the end of the exercise, the students should be able to:
Create a simple game that exhibits file input and
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local
Create a new project named LabExer6A. Set the project location to your own
Create a simple guessing game (similar to Hangman or Hangaroo). In this game, the user guesses a letter and then attempts to guess the
Create a Notepad file named txt which will store any number of words each written per line.
The Java program shall:
randomly select a word from the list saved in txt;
display a letter in which some of the letters are replaced by ?; for example, ED??A??ON (for EDUCATION);
place the letter in the correct spot (or spots) in the word each time the user guesses a letter correctly;
inform the user if the guessed letter is not in the word; and…
arrow_forward
Case study: Our client is planning to provide us with their data (integers). They expect us to produce a report with their totals. We observed their latest data and found some possible value pairs to test with: 2 and 5, 4 and 4, 1000 and 2000, 100 and 101. TASK 1. Variables. Review variables, Implement the following code in Eclipse, and ensure it runs without errors. 1. Create a Task1.java file. 2. Take a screenshot of your console output.
arrow_forward
Power ShellProject 1: Power ScriptingINTRODUCTIONYou are a network administrator who is responsible for 100 users and 20 servers for a medium size business. As an administrator who has multiple tasks to get done throughout the day, you need to develop a Power Shell script to help you manage routine tasks that you have to take care of everyday. To simplify your administration create a script with the following objectivesOBJECTIVES Script that will create multiple folders using a do loop and variableCREATE MULTIPLE FOLDER SCRIPT GUIDELINESSETTING UP THE VARIABLES: Open PowerShell ISE Create a variable $intfolders that has a value of 10 Create a variable $intPad that has a value of 0 Create a variable $i that has a value of 1 Create a variable for the new folder o New-Variable –Name strPrefix –Value “testfolder” –Option constantCREATING THE LOOP STATEMENT Do { Set If condition o if ($i -lt 10) Set Command when Condition is Trueo {New-Item –path C: -name $s
arrow_forward
Android Studio displays your project files in the Android view with a set of folders. One of these folders is the manifest folder which contains the project’s AndroidManifest.xml file. Discuss the AndroidManifest.xml file in terms of
Why does the project need this file?
What are the tasks performed by this file? Please clear and correct answer.
arrow_forward
The language I am using is Java
The source code for Shortest Job First Algorithm is already given.
Please help me to create a GUI for my code for CPU Scheduling: Shortest Job First. I have created the design for the GUI using windows builder in Java Eclipse.
I can't fully upload the code for my GUI because of the word limit in Bartleby. Therefore, you can do your own design of the GUI with the code in it. I need a functional and working code for the GUI where I can input the desired number I want for the burst time and arrival time. I hope you can help me with this. Thank you so much.
package test;
import java.util.*;
import javax.swing.JOptionPane; public class SJF { public static void main(String args[]) { Scanner sc = new Scanner(System.in); int x= Integer.parseInt(JOptionPane.showInputDialog("Enter number of process")); int processID[] = new int[x]; //To display the process ID int arTime[] = new int[x]; // arTime means arrival…
arrow_forward
question 61/62
arrow_forward
Downloads/
ht 8 (File X
English
localhost:8888/notebooks/Downloads/CSE110%20Lab%20Assignment%208%20(File%201_0%20%26%20Exception).ipynb
Cjupyter CSE110 Lab Assignment 8 (File I_O & Exception) Last Checkpoint: 6 hours ago (unsaved changes)
Logout
File
Edit
View
Insert
Cell
Kernel
Widgets
Help
Trusted
Python 3 O
• Run
с
Code
Task 10
Write a python program that finds the corresponding energy values from a list of frequency values which are taken as input from the user, using the formula,
E = h * f, where h is a constant of value: 1050
Exceptions to handle:
• Make sure all elements in the lists are numbers
Example 1:
Input:
[2,3]
Output:
[2100, 3150]
====
Example 2:
Input:
[2,boss]
Output:
Wrong input type
In [ ]: #to do
OneDrive
Screenshot saved
Task 11
The screenshot was added to your
OneDrive.
Imagine Salman Khan is going to the sets of a new movie. He decides to cycle everyday on his way to the sets. For that he uses a distan
finds the distance based on the present day's date, which is…
arrow_forward
Downloads/
ht 8 (File X
English
localhost:8888/notebooks/Downloads/CSE110%20Lab%20Assignment%208%20(File%201_0%20%26%20Exception).ipynb
Cjupyter CSE110 Lab Assignment 8 (File I_O & Exception) Last Checkpoint: 6 hours ago (unsaved changes)
Logout
File
Edit
View
Insert
Cell
Kernel
Widgets
Help
Trusted
Python 3 O
• Run
Code
Task 9
Write a python program that will take a string input from the user. Your task is to count the number of vowels and consonants in the string.
Using try, if the number of vowels is greater than or equal to the number of consonants, then raise a runtime error. Print 'Number of vowels greater/equal to
consonants. Please paraphrase.'.
Otherwise, print 'The sentence will work.'.
Note: a,e,i,o,u are called vowels and all the other 21 english alphabets are consonants.
=====:
Example 1:
Input:
'his age is 10'
Output:
Number of vowels greater/equal to consonants. Please paraphrase.
Example 2:
Input:
'he is 10 years old'
Output:
The sentence will work.
In [ ]: #to do
Task…
arrow_forward
Task Performance User Log-in
Objective:
At the end of the activity, the students should be able to:
Create a program that handles exception and allows writing to and reading from a text
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local
Create a new project named TaskPerf6. Set the project location to your own
Write a program that will:
Ask the user to select between two (2) options:
Register: User shall input his desired username and password. These shall be saved in a text file named txt. Only alphanumeric characters are allowed.
Login: User is asked to input a username and password. Validate using the text file created from the Register option. If both the username and password are correct, display the message “Successfully logged in”, else, display “Incorrect username or password”.
arrow_forward
Software Requirements:
Latest version of NetBeans IDE
Java Development Kit (JDK) 8
Procedure:
1. Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local drive.
2. Create a new project named LabExer9. Set the project location to your own folder.
3. Draw a cartoon character using lines and shapes. Apply colors. Feel free to use the following
methods:
drawline ()
• drawRect ()
• fillRect()
drawRoundRect()
fillRoundRect()
drawoval ()
fill0val()
• drawarc()
fillArc()
drawPolygon()
fillPolygon()
copyArea()
setPaint()
fill()
setStroke()
draw()
arrow_forward
Downloads/
ht 8 (File X
English
localhost:8888/notebooks/Downloads/CSE110%20Lab%20Assignment%208%20(File%201_0%20%26%20Exception).ipynb
C jupyter CSE110 Lab Assignment 8 (File I_O & Exception) Last Checkpoint: 6 hours ago (unsaved changes)
Logout
File
Edit
View
Insert
Cell
Kernel
Widgets
Help
Trusted
Python 3 O
+
• Run
с
Code
Task 11
Imagine Salman Khan is going to the sets of a new movie. He decides to cycle everyday on his way to the sets. For that he uses a distance calculator which
finds the distance based on the present day's date, which is asked as an input from the user. You should first take the day and then the month as your inputs.
The inputs should be stored in variables named day and month .The distance calculator uses the following formula to calculate a number which dictates the
number of kilometers he must cycle on his way to work.
Distance = lif day<10, 5+(day*2)/month
|else, 3+(day/month)
Exceptions to handle:
-Check for 0 division error exception in the formula
-Type…
arrow_forward
please create a document to use Crontab in linux. like scheduling a task or anything. please explain
arrow_forward
Objective:
At the end of the exercise, the students should be able to:
Create a simple game that exhibits file input and output.
Software Requirements:
• Latest version of NetBeans IDE
• Java Development Kit (JDK) 8
Procedure:
1. Create a folder named LastName_FirstName (ex. Reyes_Mark) in your local drive.
2. Create a new project named LabExer6A. Set the project location to your own folder.
3. Create a simple guessing game (similar to Hangman or Hangaroo). In this game, the user guesses
a letter and then attempts to guess the word.
4. Create a Notepad file named words.txt which will store any number of words each written per line.
5. The Java program shall:
• randomly select a word from the list saved in words.txt;
display a letter in which some of the letters are replaced by ?; for example, ED??A??ON
(for EDUCATION);
place the letter in the correct spot (or spots) in the word each time the user guesses a letter
correctly;
inform the user if the guessed letter is not in the word; and…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
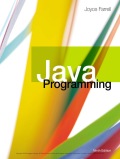
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
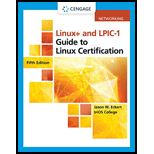
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L
Related Questions
- Course Title : Operating SystemQuestion : How you will create new user using terminal in Ubuntu? Add screenshots of all steps for new user creation including terminal and Login page in Answer sheet. Every student has to create new user as of his/her arid number for example (18-arid-2929). Note: screenshots are mandatory.arrow_forwardadd tags for these feature files with --tags option: When you select scenarios by one tag When you select scenarios that have one or another tag When you select scenarios that have 2 tags When you disable scenarios that have a specific tag Given Scenarios: Feature: Login functionalityBackground:Given a web browser is at the BrainBucket login pageScenario: user can't login without entering emailGiven User is not logged inWhen Password is enteredAnd User click Login buttonThen 'Warning: No match for E-Mail Address and/or Password' will be shownScenario: user can recover his passwordGiven User is not logged inWhen User clicks 'Forgotten Password' buttonAnd enters his emailThen Message 'An email with a confirmation link has been sent your email address.' will be dispalyedarrow_forwardWrite a report on Linux on mobile phones. Report must cover the following: Introduction to embedded Linux, How Linux is being used on mobile phones, what phones are using Linux, what modifications have been projects for mobile phones. Note: Reports should be at least 5‐7 pages. Your report must include diagrams and cite references. Failing to cite references will lead to loss of marks DO NOT COPY DIRECTLY FROM SOURCE BUT WRITE IN YOUR OWN WORDSarrow_forward
- Powershell Commands Create two groups for two teams in your company (server development) and (software testing). There are 3 members in each team need to create. They are (Jaspreet, Lina and Hanh) for the server development team, and (Nina, David and Rahul) for the software testing team. Each member has to have some role description. Lina temporarily left so her account must be disabled. Rahul left the company to a new job. Lina came back to work so her account must be enabled again. The company decided to change the name of the server development to (software development).arrow_forwardusing a code in Linux As a developer, a local Library approaches you and requests you to develop a library management system for them. Some of the requirements stated by the library are: The Admin should be able to create accounts for different users of the system Librarian User Do everything that all types of users can do The Program should be menu driven, The Librarian should have the following on their menu: Insert a book (in a book_Records) View Issued Books Display all books available on the system Search for a book (in a book_Records) Delete a book A Registered User should be able to Search for a book, Issue a book Display all books available Unregistered User should be able to : Display all books in the library Search for a book The book_Records file should be auto-updated after Insertion and Deletion Every book in the system should have the following details : Book_id Book_name Author Publishing_house Year_of_publishingarrow_forwardI need help with the part below and please add comments in the code to help me understand better what I am doing wrong in my own code. I have already created my database and the connection and some of the code but am stuck on these parts."Home" and "Create Account" pages The index.php file will ask the user to log in to the system with a login form If they're already logged in, the home page will NOT show the login form. It will show a "welcome to our store" type of message instead even if they click on the "home" button in the navigation. The create-account.php page has them fill out a form for creating an account. Use real-time JavaScript validation (and regex) to: Verify that the password and "verify password" match as they are typing. Verify that the password contains at least one number and is at least 8 characters long Be sure to display user feedback messages on the screen as they type, e.g.: Password must contain a number Password must be 8 characters long Password and…arrow_forward
- Discuss in You are working on a class assignment in GeorgiaView. You have created a folder on your PC for the class and a subfolder for this assignment. It has a 'start file' that you downloaded and saved to that subfolder. You have completed your work on this file and uploaded it to GeorgiaView (you have not saved it since the original download). What did you upload? Explain your reasoning. Your answer should include references to the various types of computer storage.arrow_forwardObjective: At the end of the exercise, the students should be able to: Create an adjacency list in Java. ■ Software Requirements: NetBeans IDE Java Development Kit (JDK) 8 Procedure: 1. Create a folder named LastName_FirstName in your local drive. (ex. Reyes_Mark) 2. Using NetBeans, create a Java project named AdjacencyList. Set the project location to your own folder. 3. Create an adjacency list based on the given graph. B A E D F Garrow_forwardi want you to draw a er model dont give me this draws all tutors answred by it :(i want new one only photosarrow_forward
- Why I am getting this error in stio() ? I am using Visual Studion 2019arrow_forwardchoose 3 different Linux flavors - also called "distros" (distributions) - and research them. Write a summary paragraph about each one, for a total of 3 paragraphs. Include a references section at the end of your assignment with active links to all the sources you use. Notes: Don't use Ubuntu.arrow_forwardMedical Unlimited Medical Unlimited (the brainchild of a group of young philanthropic medical professionals) is interested in setting up a mobile medical unit that provides free medical services for students. The mobile medical unit will require a patient record and scheduling application that will be used by the medical staff (doctors/nurses) and receptionists working there. The receptionists will use the system to capture new patient information when firsttime patients visit the doctor. Receptionists will also be responsible for scheduling all appointments. Nurses on the other hand will use the s ystem to keep track of the results of each visit including diagnosis, medications, prescription and treatment. Multiple medications may be prescribed during each visit. The nurses can also access the information to print out a history of patient visits. Th e doctors will primarily use the system to view patient history. The doctors may enter some patient treatment information and…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTLINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.Computer ScienceISBN:9781337569798Author:ECKERTPublisher:CENGAGE L
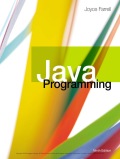
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
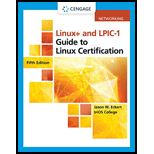
LINUX+ AND LPIC-1 GDE.TO LINUX CERTIF.
Computer Science
ISBN:9781337569798
Author:ECKERT
Publisher:CENGAGE L