Week_02_lab_06_Medal_W
.docx
keyboard_arrow_up
School
Centennial College *
*We aren’t endorsed by this school
Course
123
Subject
Computer Science
Date
Jan 9, 2024
Type
docx
Pages
6
Uploaded by MinisterWater41165
Programming II
Medal: enums, properties, filtering a list
Week02_Lab06
Submission: See course shell for how and when to submit
You will practice using Properties and iterating a list.
The MedalColor Enum
Code the MedalColor enum below:
This enum consist of 3 constants which does not require any special treatment (such as setting any Flags attribute). MedalColor
enum
Constants
Bronze
Silver
Gold
The Medal Class
Code the Medal class below:
This class comprise of five properties, a constructor and a ToString()
method. All the properties are public readonly. Medal
Class
Properties
+
«property setter absent» Name : string
+
«property setter absent» TheEvent : string
+
«property setter absent» Color : MedalColor
+
«property setter absent» Year : int
+
«property setter absent» IsRecord : bool
Methods
+
«constructor» Medal(
name : string
, theEvent : string
, color : string
, year : int
, n.k.p
Winter 2022
Page 1 of 6
Programming II
Medal: enums, properties, filtering a list
Week02_Lab06
isRecord : bool
)
+
ToString() : string
Description of class members
Properties:
All the properties have public getter and the setter is absent making them all readonly properties
Name
– this is a string representing the holder of this object. The getter is public and the setter is absent.
TheEvent
– this is a string representing the event of this object. (Event is a reserved word in C#). The getter is public and the setter is absent.
Color
– this is an enum representing the color of this object The getter is public and the setter is absent.
Year
– this is an integer representing the year of this object. The getter is public and the setter is absent.
IsRecord
– this is a bool indicating if this was a record setting event. The getter is public and the setter
is absent.
Fields:
No fields are defined in this class
Constructor:
public
Medal(
string name, string theEvent, MedalColor color, int year,
bool isRecord)
– This public constructor takes five arguments: a string representing the name, a string representing the event, a string representing the type of medal, an integer representing the year and a bool indicating if a World Record or Olympic Record was set in this event. It assigns the arguments
to the appropriate fields.
Methods:
public override string
ToString()
– This public method overrides the ToString of the object class. It does not take any argument and returns a string representation of the object. You may return a string in the format “
2012 - Boxing(R) Narendra(Gold)
”. If the event is not a record event then the “
(R)
” should not be present in the output. The ToString()
method is the best place to implement this feature.
n.k.p
Winter 2022
Page 2 of 6
Programming II
Medal: enums, properties, filtering a list
Week02_Lab06
Test Harness
Insert the following code statements in the Main()
method of your Program.cs file:
//create a medal object
Medal
m1 = new
Medal
(
"Horace Gwynne
"
, "Boxing"
, MedalColor.
Gold
, 2012, true
);
//print the object
Console
.WriteLine(m1);
//print only the name of the medal holder
Console
.WriteLine(m1.Name);
//create another object
Medal
m2 = new
Medal
(
"Michael Phelps"
, "Swimming"
, MedalColor.
Gold
, 2012, false
);
//print the updated m2
Console
.WriteLine(m2); //create a list to store the medal objects
List
<
Medal
> medals = new
List
<
Medal
>(){ m1, m2};
medals.Add(
new
Medal
(
"Ryan Cochrane
"
, "Swimming"
, MedalColor.
Silver
, 2012, false
));
medals.Add(
new
Medal
(
"Adam van Koeverden
"
, "Canoeing"
, MedalColor.
Silver
, 2012, false
));
medals.Add(
new
Medal
(
"Rosie MacLennan
"
, "Gymnastics"
, MedalColor.
Gold
, 2012, false
));
medals.Add(
new
Medal
(
"Christine Girard
"
, "Weightlifting"
, MedalColor.
Bronze
, 2012, false
));
medals.Add(
new
Medal
(
"Charles Hamelin
"
, "Short Track"
, MedalColor.
Gold
, 2014, true
));
medals.Add(
new
Medal
(
"Alexandre Bilodeau
"
, "Freestyle skiing"
, MedalColor.
Gold
, 2012, true
));
medals.Add(
new
Medal
(
"Jennifer Jones
"
, "Curling"
, MedalColor.
Gold
, 2014, false
));
medals.Add(
new
Medal
(
"Charle Cournoyer
"
, "Short Track"
, MedalColor.
Bronze
, 2014, false
));
medals.Add(
new
Medal
(
"Mark McMorris
"
, "Snowboarding"
, MedalColor.
Bronze
, 2014, false
));
medals.Add(
new
Medal
(
"Sidney Crosby
"
, "Ice Hockey"
, MedalColor.
Gold
, 2014, false)
);
medals.Add(
new
Medal
(
"Brad Jacobs
"
, "Curling"
, MedalColor.
Gold
, 2014, false)
);
n.k.p
Winter 2022
Page 3 of 6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Task Class Requirements
The task object shall have a required unique task ID String that cannot be longer than 10 characters. The task ID shall not be null and shall not be updatable.
The task object shall have a required name String field that cannot be longer than 20 characters. The name field shall not be null.
The task object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null.
Verify with Juint testing as well
arrow_forward
Task Class Requirements
The task object shall have a required unique task ID String that cannot be longer than 10 characters. The task ID shall not be null and shall not be updatable.
The task object shall have a required name String field that cannot be longer than 20 characters. The name field shall not be null.
The task object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null.
Task Class with JUnit test case with it!
Please do not copy and paste from others with TaskService. It's not helpful and does not relate to what I am doing. Thank you.
Java language
arrow_forward
Create a product_class.php script that includes a Product class with
parameters: product name, product id, and price
methods:
print_info(): this method will print all the information related to the product
set set_price(): this method takes a "price" argument and updates the corresponding parameter in the object
get get_price(): this method returns the value (i.e. price) of the product
Create a using_product_class.php script that creates 2 objects of the class Product and shows how to use its methods (i.e. print_info(), set_price(), and get_price())
arrow_forward
Q1 Draw uml class diagram
The SUV Rental Provider App has two types of system users namely Client and Admin. The client, who can be Member or Non-Member can search for SUV models and daily rental. Each SUV model consists of SUV model details and daily rental. Thus, the Client can choose to view the SUV model details such as description and images and daily rental based on the types of the selected model. Only Member and Admin are required to log in to access the system. Member gains access to additional services. The additional services for members are: manage a reservation, check membership details, change password, manage credit card and banking information, and log out. Each Member is entitled to reserve up to 10 SUVs. When a member makes a reservation, he/she has to specify the selected SUV model and the number of rental days. The member also can edit and delete a reservation. Based on the reservation, the app generates the rental quotation. The rental quotation includes both the…
arrow_forward
c++ language
Create Class Student▪ Data Members: Name , ID and GPA.▪ Make the data members visibility to be private.▪ Use setter and getter method for each attribute.▪ In the main, set values for the student details using setter function, then retrieve the value using the getter function.▪ Print what you retrieve
arrow_forward
The attributes field of objects is a place where data may be saved. The class is solely responsible for its own properties.
arrow_forward
In the attributes field of an object, you can store data. The class's traits are unique to the class.
arrow_forward
Data may be stored in the attributes field of objects. The class's characteristics are the class's own.
arrow_forward
3.
Which data attribute can you use to indicate that a property in a model class must not be an empty value?
1. Required
2. Range
3. NonNullable
4. NotNull
arrow_forward
hi
i need solution for this
Each account should have a constant, unique id. Id numbers should start from 1000 and increment by 5. The ACCOUNT_ID attribute should be initialised when the account object is created. The id should be generated internally within the class, it should not be passed in as an argument
arrow_forward
Registration
Review
Productid: Int
Customer name: String
Username: String
Password: String
Customerld: Int
CustomerName: String
ID Number: Int
CreateReview();
Email: String
EditReview(Int Reviewld);
RegisterCustomer():
Customer
CustomerName: String
Customerld: Int
PhoneNO: Int
EditCustomer();
Search();
Stock
Оrder
Bookld: Int
OrderID: Int
CustomerID: Int
Quantity: Int
CustomerName: String
BookName: String
AddStock();
ModifyStock(Int Bookld);
SelectStockltem(IntBookld);
Payment: Int
CreateOrder();
EditOrder(Int ordelD);
Books
BookID: Int
BookPrice: Int
BookType: String
AddProduct();
ModifyProduct();
SelectProduct(Int ProductID);
kindly draw ERD for the following class diagram
with proper notation (cardinality)
implement on any tool don't use hand written
please
arrow_forward
Jump to level 1
The ClassSchedule table has the following columns:
StartDate - DATE
EndDate - DATE
Start Time - TIME
End Time - TIME
Complete the SELECT statement to return the hour from the start time and the
hour from the end time.
SELECT
Type your code here */
FROM ClassSchedule;
arrow_forward
Contact Class Requirements
The contact object shall have a required unique contact ID string that cannot be longer than 10 characters. The contact ID shall not be null and shall not be updatable.
The contact object shall have a required firstName String field that cannot be longer than 10 characters. The firstName field shall not be null.
The contact object shall have a required lastName String field that cannot be longer than 10 characters. The lastName field shall not be null.
The contact object shall have a required phone String field that must be exactly 10 digits. The phone field shall not be null.
The contact object shall have a required address field that must be no longer than 30 characters. The address field shall not be null.
Contact Service Requirements
The contact service shall be able to add contacts with a unique ID.
The contact service shall be able to delete contacts per contact ID.
The contact service shall be able to update contact fields per contact ID. The…
arrow_forward
Data may be stored in the attributes field of objects. The class's characteristics are the class
's own.?
arrow_forward
CRC stands fora. Class, Return value, Compositionb. Class, Responsibilities, Collaborationsc. Class, Responsibilities, Compositiond. Compare, Return, Continue
arrow_forward
Car Class Project
The car classwill havethe following attributes:
•year: an integer that holds the car's model year
•model: a string that holds the make of the car
•make: a string that holds the model of the car
•speed: an integer that holds the car's current speed
Your class should contain thefollowing:
A docstring that briefly describes the class and lists the attributes. The docstring will serve as the documentation for your class.
•A constructor (__init__ method) that takes the car's year model and make as optional arguments. The constructor will set the value of the speed attribute to 0.•An __str__ method that returns the car's year model and makein a string.
•To test your class, create acar object and use the print function to verify that the constructor and __str__ methodsare working correctly.
•An accessor method which returns the value stored in the speed instance variable. Call this method getSpeed().
•A modifier method called accelerate() which adds 5 to the speed variable…
arrow_forward
arrow_back
Starting Out With Visual C# (5th Edition)
5th Edition
Chapter 11, Problem 1PP
arrow_back_ios
PREVIOUS
NEXT
arrow_forward_ios
Question
share_out_linedSHARE SOLUTION
Chapter 11, Problem 1PP
Program Plan Intro
Employee and ProductionWorker Classes
Program plan:
Design the form:
Place a three text boxes control on the form, and change its name and properties to get the employee name, number, and hourly pay rate from the user.
Place a four label boxes control on the form, and change its name and properties.
Place a two radio buttons control on the form, and change its name and properties.
Place a one group box control on the form, and change its name and properties.
Place a command button on the form, and change its name and properties to retrieve the object properties and then display the values into label box.
In code window, write the code:
Program.cs:
Include the required libraries.
Define the namespace “Program11_1”.
Define a class “Program”.
Define a constructor for the…
arrow_forward
Student – with no parameters, initialize all the data members to 0 or null.
Data Members
studentNo – int
fname – String
lname – String
course – String
Method Members
setStudentNo – to set the student number of the student
setFname – to set the firstname of the student
setLname – to set the lastname of the student
setCourse – to set the course of the student
getStudentNo – to get the student number of the student
getFname – to get the firstname of the student
getLname – to get the lastname of the student
getCourse – to get the course of the student
arrow_forward
Food delivery application: This system allows the customers to
search and order the food etc. online. The administrator can also
use the system for administrative purpose like add and delete
dishes etc.
Create your own case study (problem statement) for the
above application and
1. Draw the use case diagram
2. Class diagram with at least 4 classes and explain all the
relationships
3. Draw a DFD-0 for this application
arrow_forward
Lab conditions:
This lab exercise to be completed by the end of the class. No late submission will be accepted
Work as group of two students.
Submit Word document file on D2L
Make sure your following naming format as listed below:
Last name, First Name:
Last name, First Name:
Questions:
1. Research, discuss Explain the purpose of different personal computer (PC) hardware components.
Make sure to address all the aspect of the topic.
Partial list of opcodes:
2. Desktop Computer DIY. Suppose you decide to build a desktop by yourself and your budget is
around $1000 (without OS). Discuss with your team members and list all the parts and tools you have
to purchase with price. List the technical Details and explain what your desktop will be used for, such
as listen to music, word document, 3D design, software development, watch movie and so on.
3. Download and run CPU-Z. Paste your screenshots (technical details) below.
4. Challenge Question
Consider the hypothetical machine:
Instruction…
arrow_forward
Class Design
Within the backend server, we’ll have multiple classes to organize our code.
All the class descriptions are listed below.
FoodWastageRecord
NOTE: You need to design this class.
It represents each food wastage entry recorded by the user through the form on the webpage (frontend).
If you notice the form on the webpage, you’ll see that each FoodWastageRecord will have the following as the data members aka member variables.
Date (as string)
Meal (as string)
Food name (as string)
Quantity in ounces (as double)
Wastage reason (as string)
Disposal mechanism (as string)
Cost (as double)
Each member variable comes with its accessor/mutator functions.
FoodWastageReport
NOTE: You need to design this class.
It represents the report generated on the basis of the records entered by the user. This class will be constructed with all the records entered by the user as a parameter. It will then apply the logic to go over all the records and compute the following:
Names of most…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
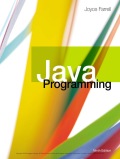
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
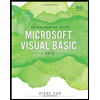
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning
Related Questions
- Task Class Requirements The task object shall have a required unique task ID String that cannot be longer than 10 characters. The task ID shall not be null and shall not be updatable. The task object shall have a required name String field that cannot be longer than 20 characters. The name field shall not be null. The task object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null. Verify with Juint testing as wellarrow_forwardTask Class Requirements The task object shall have a required unique task ID String that cannot be longer than 10 characters. The task ID shall not be null and shall not be updatable. The task object shall have a required name String field that cannot be longer than 20 characters. The name field shall not be null. The task object shall have a required description String field that cannot be longer than 50 characters. The description field shall not be null. Task Class with JUnit test case with it! Please do not copy and paste from others with TaskService. It's not helpful and does not relate to what I am doing. Thank you. Java languagearrow_forwardCreate a product_class.php script that includes a Product class with parameters: product name, product id, and price methods: print_info(): this method will print all the information related to the product set set_price(): this method takes a "price" argument and updates the corresponding parameter in the object get get_price(): this method returns the value (i.e. price) of the product Create a using_product_class.php script that creates 2 objects of the class Product and shows how to use its methods (i.e. print_info(), set_price(), and get_price())arrow_forward
- Q1 Draw uml class diagram The SUV Rental Provider App has two types of system users namely Client and Admin. The client, who can be Member or Non-Member can search for SUV models and daily rental. Each SUV model consists of SUV model details and daily rental. Thus, the Client can choose to view the SUV model details such as description and images and daily rental based on the types of the selected model. Only Member and Admin are required to log in to access the system. Member gains access to additional services. The additional services for members are: manage a reservation, check membership details, change password, manage credit card and banking information, and log out. Each Member is entitled to reserve up to 10 SUVs. When a member makes a reservation, he/she has to specify the selected SUV model and the number of rental days. The member also can edit and delete a reservation. Based on the reservation, the app generates the rental quotation. The rental quotation includes both the…arrow_forwardc++ language Create Class Student▪ Data Members: Name , ID and GPA.▪ Make the data members visibility to be private.▪ Use setter and getter method for each attribute.▪ In the main, set values for the student details using setter function, then retrieve the value using the getter function.▪ Print what you retrievearrow_forwardThe attributes field of objects is a place where data may be saved. The class is solely responsible for its own properties.arrow_forward
- In the attributes field of an object, you can store data. The class's traits are unique to the class.arrow_forwardData may be stored in the attributes field of objects. The class's characteristics are the class's own.arrow_forward3. Which data attribute can you use to indicate that a property in a model class must not be an empty value? 1. Required 2. Range 3. NonNullable 4. NotNullarrow_forward
- hi i need solution for this Each account should have a constant, unique id. Id numbers should start from 1000 and increment by 5. The ACCOUNT_ID attribute should be initialised when the account object is created. The id should be generated internally within the class, it should not be passed in as an argumentarrow_forwardRegistration Review Productid: Int Customer name: String Username: String Password: String Customerld: Int CustomerName: String ID Number: Int CreateReview(); Email: String EditReview(Int Reviewld); RegisterCustomer(): Customer CustomerName: String Customerld: Int PhoneNO: Int EditCustomer(); Search(); Stock Оrder Bookld: Int OrderID: Int CustomerID: Int Quantity: Int CustomerName: String BookName: String AddStock(); ModifyStock(Int Bookld); SelectStockltem(IntBookld); Payment: Int CreateOrder(); EditOrder(Int ordelD); Books BookID: Int BookPrice: Int BookType: String AddProduct(); ModifyProduct(); SelectProduct(Int ProductID); kindly draw ERD for the following class diagram with proper notation (cardinality) implement on any tool don't use hand written pleasearrow_forwardJump to level 1 The ClassSchedule table has the following columns: StartDate - DATE EndDate - DATE Start Time - TIME End Time - TIME Complete the SELECT statement to return the hour from the start time and the hour from the end time. SELECT Type your code here */ FROM ClassSchedule;arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- EBK JAVA PROGRAMMINGComputer ScienceISBN:9781337671385Author:FARRELLPublisher:CENGAGE LEARNING - CONSIGNMENTProgramming Logic & Design ComprehensiveComputer ScienceISBN:9781337669405Author:FARRELLPublisher:CengageProgramming with Microsoft Visual Basic 2017Computer ScienceISBN:9781337102124Author:Diane ZakPublisher:Cengage Learning
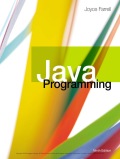
EBK JAVA PROGRAMMING
Computer Science
ISBN:9781337671385
Author:FARRELL
Publisher:CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:9781337669405
Author:FARRELL
Publisher:Cengage
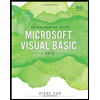
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:9781337102124
Author:Diane Zak
Publisher:Cengage Learning