EE418-Assignment#2-Sol
.pdf
keyboard_arrow_up
School
University of Washington *
*We aren’t endorsed by this school
Course
418
Subject
Electrical Engineering
Date
Dec 6, 2023
Type
Pages
18
Uploaded by ChefDove1401
EE 418 - Assignment 2 - Solutions
Total Points: 100
Autumn Quarter, 2023
Prof. Radha Poovendran
Department of Electrical and Computer Engineering
University of Washington, Seattle, WA 98195
November 4, 2023
Note:
•
This homework contains both computation questions (marked as
[Com]
) which are required to do by
hand calculations and programming questions (marked as
[Pro]
) which are required to write Python
/MATLAB codes. Zero points will be awarded if
[Com]
questions are solved via Python/MATLAB
scripts and if
[Pro]
questions are solved by hand calculations.
•
Show the computation steps and/or justify your answers in all the
[Com]
questions. Failure to show
any intermediate computation steps in
[Com]
questions will result zero points.
•
You can use and modify the Python functions provided in the file section of the EE 418 canvas page
when answering the
[Pro]
questions.
•
In addition to problems 1-9,
Graduate students
are required to solve questions 10-13 marked as
[GRAD]
. Undergraduate students may attempt these questions.
However, undergraduate students
will not be awarded any extra points for attempting
[GRAD]
questions. Total number of points for
graduate students will be scaled back to 100 points.
•
You can discuss with others but you need to write your own computation steps, justifications and/or
Python/MATLAB codes.
•
Your answers to this homework must be submitted through canvas as a single zip file
containing the following:
i
)
hand written and scanned or word or pdf answers to all the
computational and discussion questions as single pdf file.
ii
)
Python/MATLB codes for
programming questions as in filename.py or filename.m respectively.
•
Name of your submission zip file should follow the following format. “ #
$
EE418
HW2.zip
”,
where “#” and “
$
” should be replaced with your first name and last name, respectively.
1
1.
[Pro]
(Affine Cipher Decryption) Please answer the following questions.
(a) (10 pts) Please write a Python/MATLAB function for affine cipher decryption.
This function
should take the ciphertext (
Y
) and key value pair (
a, b
) as inputs and output plaintext (
x
).
(b) (5 pts) Use your function developed in part (
a
) to decrypt the provided cipher text file “
sampleA-
CAD.txt
”. Use the key value pair, (
a, b
) = (9
,
−
17).
i
) Write the decrypted text to a file name “#
$
affine
output.txt
”, where “#” and “
$
” should be
replaced with your first name and last name, respectively.
ii
) Print the 30
th
to 39
th
ciphertext characters in the file “
sampleACAD.txt
” and their corre-
sponding plaintext.
Answers:
(a) Sample Python code is provided in Figure 1 below.
Figure 1:
Sample Python function for affine cipher decryption.
(b) Answer is “sture cont”.
2
2.
[Com]
(Extended Euclidean Algorithm, 5 pts
×
2 = 10 pts)
(a) Using the
extended Euclidean algorithm
, compute integers
x
and
y
such that 754
x
+233
y
= 1.
Show all the steps in your calculations.
(b) Find 754
−
1
mod 233 and 233
−
1
mod 754
Answers:
(a) First using Euclidean algorithm,
754
=
2(233) + 288
(1)
233
=
0(288) + 233
(2)
288
=
1(233) + 55
(3)
233
=
4(55) + 13
(4)
55
=
4(13) + 3
(5)
13
=
4(3) + 1
(6)
Next we rewrite equation (6) as follows.
1 = 13
−
4(3)
(7)
Substituting “3” in equation (7) by equation (5) gives:
1
=
13
−
4(55
−
4(13))
1
=
−
4(55) + 17(13)
(8)
Substituting “13” in equation (8) by equation (4) gives:
1
=
−
4(55) + 17(233
−
4(55))
1
=
17(233)
−
72(55)
(9)
Substituting “55” in equation (9) by equation (3) gives:
1
=
17(233)
−
72(288
−
233)
1
=
89(233)
−
72(288)
(10)
Substituting “288” in equation (10) by equation (1) gives:
1
=
89(233)
−
72(754
−
2(233)) +
1
=
−
72(754) + 233(233)
(11)
Hence,
x
=
−
72 and
y
= 233.
(b) Applying (mod233) to the both left and right sides of equation (11) yields,
−
72(754)
mod 233
=
1
mod 233
754
−
1
mod 233
=
−
72
mod 233
754
−
1
mod 233
=
161
mod 233
Applying (mod754) to the both left and right sides of equation (11) yields,
233(233)
mod 754
=
1
mod 754
233
−
1
mod 754
=
233
mod 754
3
3.
[Com]
(Hill and Affine Ciphers, 5 pts
×
2 = 10 pts) This is an example of cascading encryption scheme
with two consecutive Hill cipher encryptions followed by an affine cipher encryption.
Consider the following cryptosystem with a smaller set of 11 English letters, i.e.,
a
through
k
, which
map to 0 through 10, respectively. The cryptosystem consists of hill ciphers with keys
K
1
and
K
2
that
are both 2x2 matrices, and an affine cipher with key
K
3
. Suppose that
K
1
=
1
3
0
1
,
K
2
=
2
1
1
0
,
K
3
= (7
,
2). For example, if the plaintext is
x
= (5
,
8), then the encryption process is: First plaintext
x
is encrypted using Hill cipher with key
K
1
, then the resulting ciphertext is encrypted again using
Hill cipher with key
K
2
, and finally, the ciphertext obtained from 2nd Hill cipher is encrypted using
an Affine cipher with key
K
3
to obtained the ciphertext
y
of plaintext
x
. This process is also shown
in the following equations.
First Hill Cipher: (5
,
8)
·
1
3
0
1
mod 11 = (5
,
1)
(12)
Second Hill Cipher: (5
,
1)
·
2
1
1
0
mod 11 = (0
,
5)
(13)
Affine Cipher: (7
·
0 + 2
,
7
·
5 + 2) mod 11 = (2
,
4)
(14)
In general, the plaintext is
x
= (
x
1
, x
2
), and the ciphertext is
y
= (
y
1
, y
2
). Next, you will combine the
above three ciphers with the given keys into one single cipher.
(a) Please write down encryption rule (i.e, Find a matrix
K
and a scalar
b
such that
xK
+
b
1
1
×
2
=
y
).
Simplify your answer and express the numbers in
Z
11
if possible.
(b) Please write down decryption rule(i.e, Find a matrix
¯
K
and a vector
¯
b
such that
y
¯
K
+
¯
b
=
x
).
Simplify your answer and express the numbers in
Z
11
if possible.
Answers:
(a) We first derive the single-cipher encryption rule:
In the first Hill cipher, we get
(
y
(1)
1
, y
(1)
2
) = (
x
1
, x
2
)
K
1
= (
x
1
, x
2
)
1
3
0
1
= (
x
1
,
3
x
1
+
x
2
) mod 11
In the second Hill cipher, we get
(
y
(2)
1
, y
(2)
2
) = (
y
(1)
1
, y
(1)
2
)
K
2
= (
x
1
,
3
x
1
+
x
2
)
2
1
1
0
= (5
x
1
+
x
2
, x
1
) mod 11
In the Affine cipher, we get
(
y
(3)
1
, y
(3)
2
) = (7
·
y
(2)
1
+ 2
,
7
·
y
(2)
2
+ 2) = (7(5
x
1
+
x
2
) + 2
,
7
x
1
+ 2)
= (35
x
1
+ 7
x
2
+ 2
,
7
x
1
+ 2) mod 11
= (2
x
1
+ 7
x
2
+ 2
,
7
x
1
+ 2) mod 11
Thus, the single-cipher encryption rule is
(
y
1
, y
2
) = (2
x
1
+ 7
x
2
+ 2
,
7
x
1
+ 2) mod 11
or equivalently,
[
y
1
, y
2
] = [
x
1
, x
2
]
2
7
7
0
+ 2[1
,
1] mod 11
4
Therefore,
K
=
2
7
7
0
mod 11 and
b
= 2 mod 11.
(b) The decryption rule is given by: [
x
1
, x
2
] = ([
y
1
, y
2
]
−
[2
,
2])
2
7
7
0
−
1
.
First note that det
K
= 2
×
0
−
7
×
7 =
−
49 and gcd(
−
49
,
11) = 1.
Hence,
K
−
1
mod 11 exists.
Now we have,
2
7
7
0
−
1
mod 11 = (
−
49)
−
1
0
−
7
−
7
2
mod 11
Note that (
−
49)
−
1
mod 11 = 6
−
1
mod 11 = 2 mod 11 since 6
×
2 mod 11 = 12 mod 11 =
1 mod 11.
Hence,
2
7
7
0
−
1
mod 11 = 2
0
−
7
−
7
2
mod 11 =
0
−
14
−
14
4
mod 11 =
0
8
8
4
mod 11
These yield:
[
x
1
, x
2
] = ([
y
1
, y
2
]
−
[2
,
2])
2
7
7
0
−
1
= ([
y
1
, y
2
]
−
[2
,
2])
0
8
8
4
= [
y
1
, y
2
]
0
8
8
4
+ [6
,
9]
Therefore,
¯
K
=
0
8
8
4
and
¯
b
= [6
,
9].
5
4.
[Com]
(Cryptanalysis, 2.5pts
×
4 = 10 pts) We use “X”, “Y”, and “Z” to denote Sender, Receiver, and
Eavesdropper, respectively. “X” is sending a message to “Y” using one of the following cryptosystems.
The plaintext of the message consists of the letter
a
repeated a few hundred times. “Z” knows what
cryptosystem is being used, but not the key, and intercepts only the ciphertext. For systems (a), (b),
(c) and (d), state how “Z” will recognize that the plaintext is one repeated letter and decide whether
or not “Z” can deduce the letter and key. (Note: for system (c), the solution very much depends on
the fact that the repeated letter is
a
, rather than
b, c, . . .
)
(a) Shift cipher
(b) Affine cipher
(c) Hill cipher (with a 2
×
2 matrix)
(d) Vigen`
ere cipher
Answers:
(a)
•
Recall shift cipher encryption is defined as:
y
i
=
x
i
+
k
mod 26
,
(15)
where
x
i
,
y
i
, and
k
denote the
i
th
plaintext, the
i
th
ciphertext and the shift cipher encryp-
tion key, respectively. Let
i
∈ {
1
,
2
, . . . , l
}
, where
l
denotes the length of the plaintext.
Note that from equation (15), when the plaintext is composed of a one repeated letter
(i.e.,
x
1
=
x
2
=
. . .
=
x
l
=
x
), ciphertext will also be composed of one repeated letter
(i.e.,
y
1
=
y
2
=
. . .
=
y
l
=
y
). On the other hand for any two
i, j
∈ {
1
,
2
, . . . , l
}
such
that
i
̸
=
j
, if
x
i
̸
=
x
j
, then
y
i
̸
=
y
j
. Since in this case “
Z
” will observe a ciphertext with
a repeated letter which indicates “
Z
” that the plaintext is composed of an one repeated
letter.
•
Let
y
denote the repeated ciphertext letter observed by “
Z
” and
x
be the repeated
plaintext letter. With this information “
Z
” can construct the following formula.
y
=
x
+
k
mod 26
When “
X
” is encrypting only one plaintext letter this is the only equation that can be
formulated by “
Z
”. However, this equation has two unknowns
x
and
k
. Therefore, “
Z
”
will not be able to deduce the plaintext
x
or shift cipher encryption key
k
.
(b)
•
Recall shift cipher encryption is defined as:
y
i
=
ax
i
+
b
mod 26
,
(16)
where
x
i
,
y
i
, and (
a, b
) denote the
i
th
plaintext, the
i
th
ciphertext and the affine cipher
encryption key, respectively.
Let
i
∈ {
1
,
2
, . . . , l
}
, where
l
denotes the length of the
plaintext. Then assume there can be a case such that
y
i
=
y
j
for any two
x
i
̸
=
x
j
, where
i, j
∈ {
1
,
2
, . . . , l
}
and
i
̸
=
j
. Then from equation (16), we get:
ax
i
+
b
mod 26
=
ax
j
+
b
mod 26
a
−
1
ax
i
+
b
mod 26
=
a
−
1
ax
j
+
b
mod 26
(17)
x
i
+
b
−
b
mod 26
=
x
j
+
b
−
b
mod 26
x
i
mod 26
=
x
j
mod 26
(18)
Note that in equation (17), we are allowed to multiple both sides of the equation with
a
−
1
mod 26, since valid key in affine cipher is always chosen such that gcd(
a,
26) = 1.
Equation (18) suggests that
x
i
=
x
j
, which contradicts our original assumption of
x
i
̸
=
x
j
. Then by contradiction for any two
i, j
∈ {
1
,
2
, . . . , l
}
such that
i
̸
=
j
, if
x
i
̸
=
x
j
,
6
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Question Ach
calculate the decimal equivalent of the hexadecimal number 3AE2 .Show proper steps and calculation.. .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this line .
arrow_forward
Content: Analyze and explain further the step by step answer. Thank you.
arrow_forward
Solve the problem and simplify the output function using Quine–Mc Cluskey Methods. The following requirements must be met in solving the problem.
Requirement:a. Truth Tableb. Timing Diagramc. Quine – Mc Cluskey Method d. Logic Diagram
A private company wants to decide on a certain issue. Each of the four officials has an equal share of voting right. At least two of them must approve the solution in order to implement it. Each of them has a switch which closes to vote YES and open to vote NO.
arrow_forward
Write assembly language program.
arrow_forward
Activity 5:
An automated QA machine selects the product in an industry based on the perfection of color,
weight, and length which are expressed by the variables C, W, and L, respectively. If a feature is
perfect the machine takes input as '1', '0' otherwise. Based on the conditions, machine decides
whether to reject (R) a product or not. Assume R = 1, if at least two of the features are not perfect.
Otherwise, R = 0. Solve the following.
arrow_forward
the extension of python module is
.numpy O
.python O
.py
none
arrow_forward
• SuperNe liı.
A moodle.nct.edu.om
Convert the octal NUMBER 2713. 6464
to hexadecimal number showing the steps
(Note : The problem should be solved in
separate paper with steps and scanned copy
should be submitted along with other answers
Final answer must be entered in the boxes
provided below. For Hexadecimal digits from A to F,
enter their equivalent decimal value in the
corresponding box; do not enter the letter (For eg-
for A, write 10, for B write 11 etc);
Enter only one digit in each box. If there is any
unused box, fill it with 0 -do not leave it blank)
In the written submission, use the letters A to F for
hex digits instead of the values)
Integer part =
Fractional part =
II
arrow_forward
Answer please sir! Only correct answer will be getting thumb's up. Otherwise i will do rrate negativity.
arrow_forward
Using any design approach, plan and design the following problem:
(show the Block Diagram, Truth Table, Simplification Approach Solution, Circuit Diagram)
2. Simple Security System
A simple security system for two doors consists of a card reader and a keypad.
Cand Reader
To Door
To Dor 2
To Alam
Logic
Circuit
D
E
Keypad
A person may open a particular door if he or she has a card containing the corresponding
code and enters an authorized keypad code for that card. The outputs from the card reader
are as follows:
A B
No card inserted
Valid code for door 1 0 1
0 0
Valid code for door 2 1 1
Invalid card code
10
To unlock a door, a person must hold down the proper keys on the keypad and, then, insert
the card in the reader. The authorized keypad codes for door 1 are 101 and 110, and the
authorized keypad codes for door 2 are 101 and 011. If the card has an invalid code or if the
wrong keypad code is entered, the alarm will ring when the card is inserted. If the correct
keypad code is…
arrow_forward
Design a code converter that converts a decimal digit from
BCD to excess-3 code, the input variables are organized as
(A BC D) respectively with A is the MSB, the output
variables are organized as (W X Y Z) respectively with W is
the MSB, put the invalid decimal numbers as don't care.
X= BCD'+B'D+B'C
X= BC'D'+B'D+BC
X= BC'D'+B'D+B'C
X= BC'D'+BD+B'C
arrow_forward
module structural_example (F, A, B, C);
input A, B, C;
output F;
"always comment your code
so you know what you didl"/
* This code define the structure of the circuit "/
* First symbol is always output for not, and, and or /
I/C is inverted and stored in D, output is named
I/B AND with D, store result in E, output is named
I/A OR with E, store result in F
not (D,C):
and (E,B,D):
or (FA,E):
endmodule
The Boolean function FIAB.C)described by the structural model of the circuit in Verilog HDL shown in the figure is
O a. FIABC) -I m(4,6,7)
Ob FIABC)|| M(0,1,2,3)
OC FIAB.C) - TI M0.1.2.3.5,7)
Od. FIABC) - E m(0,1,2,3,5,7)
arrow_forward
Why do we call some ICs universal ICs?
(write your answer in the box below (do not include this in your pdf f
arrow_forward
Signed integers are represented in two's complementary formats. Does the same
arithmetic hardware (or algorithm) work exactly the same between
a. Unsigned addition and signed addition?
b. Unsigned subtraction and signed subtraction?
c. Unsigned multiplication and signed multiplication(if the product obtained
the same length as the operands)?
d. Unsigned division and signed division?
Please give your answer as "true" or "false" for the above 4 questions.
arrow_forward
Hello I am having trouble with a practice problem for my digital circuits class. I would appreciate if you can help me out. Thanks.
arrow_forward
Please answer question 1C, 1D with details on why its true or false. Please make handwriting legible. Thank you
arrow_forward
course Digital Logic Design
Question :
Course Digital Logic Design.
Question :Colors of different nature can be produced by mixing three fundamental colors that are red, green and blue. Consider you are assigned a task to design a digital system that is equipped with three different LEDs and four different color sensors. One LED is red (symbolized with R), one is green (symbolized with G) and one is blue (symbolized with L) while one sensor detects white color (symbolized with W), one detects black color (symbolized with B), one detects cyan color (symbolized with C) and one sensor detects magenta color (symbolized with M).It is known that each color sensor produces logic 1 output if and only if when an object of its corresponding color passes in front of it, otherwise logic 0.Each LED turns on when logic 0 is provided to it and remains turned off otherwise. Three LEDs are to be turned on according to the following conditions.i.If only white color is detected then all three LEDs…
arrow_forward
The observation of a certain measurement is described by the following figure.
'True' value
A
Which of the following statement is correct ?
O a.
low accuracy and high precision
O b. low accuracy and low precision
Oc high accuracy and low precision
O d. high accuracy and high precision
arrow_forward
Could you explain this question in details, please.
This question is about the Embedded System (Electrical & Electronic engineering).
- Explain meaning of Vetronics.
arrow_forward
The numbers from 0-9 and a no characters
is the Basic 1 digit seven segment display
* .can show
False
True
In a (CA) method of 7 segments, the
anodes of all the LED segments are
* "connected to the logic "O
False
True
Some times may run out of pins on your
Arduino board and need to not extend it
* .with shift registers
True
False
arrow_forward
Q1 (a) Describe TWO (2) advantages of DIGITAL over to ANALOG in data storage.
(1 mark)
(b)
As technology enhance, higher space memory becomes necessity for storage purpose.
With the help of appropriate diagram, describe how the digital data is stored using optical
disc (CD-ROM).
(1 mark)
(c)
Convert the decimal number 458 into following numbers by showing all the steps.
i. Octal number
(2 marks)
ii.
Hexadecimal number
(2 marks)
iii.
Binary number
(2 marks)
iv. Gray code
(2 marks)
arrow_forward
1. An arithmetic system operates with 3-digit decimal numbers with sign presented in BCD (12 bits),
negative numbers utilize the 10's complement form, one decimal digit (4 bits) is occupied by sign: 0 for
"+" and 9 for "-".
1a) Show the most positive and the most negative numbers in both decimal and BCD forms.
1b) Present numbers A=+36 and B= -42 in BCD.
1c) Present the 10's complements of A and B in BCD.
1d) Perform the following operations bit-by-bit, show carries, apply BCD correction (add 6) when
necessary (the sum of two decimal digits is greater than 9).
1d1) A+B in BCD.
1d2) A - B = A+(-B) in BCD by addition of A and the 10's complement of B.
1d3) B-A= B + (-A) in BCD by addition of B and the 10's complement of A.
arrow_forward
Convert the OCTAL NUMBER 6313.5177
to decimal number (show the steps in paper , scan and upload along with other answers)
decimal no =
arrow_forward
3 For each of the following Boolean expression, do the followings:
3.1
H = AB + B
a. Draw the electrical schematic diagram using IEC standardized referencing,
symbol and terminal numbering.
b. Describe the operation of the circuit
3.2
L = AB + AC + ABC
a. Draw the electrical schematic diagram using IEC standardized referencing,
symbol and terminal numbering.
a. Describe the operation of the circuit
目
arrow_forward
Design a 5 bits input logic circuit that can be output F to go active in (High) under the following conditions :
-All input are logic 1
-An odd number of inputs are logic 1
-non of input are logic 1
arrow_forward
Please answer in typing format
arrow_forward
A fruit processing company wants to install an apple processing plant in Quetta. Company required the design of a system to pack four apples in a box but the apples vary in sizes. For simplicity an apple is characterized as ‘Big’ or ‘Small’ with logic ‘1’ and logic ‘0’ respectively. In this way there will be four categories of boxes so, a color label should be placed on each box to distinguish different boxes. The boxes should be labeled using following rule,
If all four apples are small, the box should be labeled red
If only two apples are small, the box should be labeled blue
If only one apples is big, the box should be labeled green
If all four apples are big, the box should be labeled yellow
If only three apples are found big, the box should be labeled with both red and yellow.
List the truth table.
Draw the circuit diagram of system using decoder.
arrow_forward
Problem
Assign
Unit 3
Write the Boolean
Equation
Unit 3
Obtain the sum of
products
Description
A
B
C
a
.X
(W+X' + Z') (W' + Y')(W' + X + Z') (W+X') (W+Y+Z)
arrow_forward
Question 1 Digital Electronics and Combinational Logic
la) Analog and Digital Electronics
i. Write either "digital" or "analog" in this to indicate whether the property in that row is
typical of digital electronics or analog electronics. The first row has been completed as an
example.
Property
Difficult, manual circuit design
Continuous valued signals
Tolerant of electrical noise
Circuit state tends to leak
Intolerant of component variations
Digital/Analog
Analog
ii. In older cars the timing of the electrical pulses to the spark plugs was controlled by a
mechanical distributor. This contained a rotating contact that was mechanically linked to the
rotation of the engine. Newer cars use electronic ignition. Electrical sensors detect the position
and speed of the motor and a digital controller sends ignition pulses to the spark plugs.
Briefly describe 2 likely benefits of the digital electronic ignition system over the mechanical
one. An example is given first.
More flexible control: the…
arrow_forward
The subject is: Automatic Control Systems
Please send the answer by typing ONLY. I don't want any handwritten.
Also, don't copy-paste from the net, as plagiarism will be counted.
arrow_forward
write 1600 words about electrical car..
Please solve this as soon as possible
arrow_forward
A system uses two-dimensional parity. Find the parity unit for the following three data units: 00110 11001 00001. Assume even parity. (write down the sequence of 0's and 1's without any blank space). hints: answer is 6 bit
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
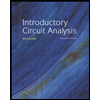
Introductory Circuit Analysis (13th Edition)
Electrical Engineering
ISBN:9780133923605
Author:Robert L. Boylestad
Publisher:PEARSON
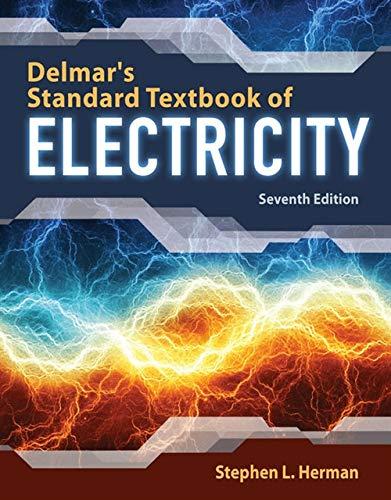
Delmar's Standard Textbook Of Electricity
Electrical Engineering
ISBN:9781337900348
Author:Stephen L. Herman
Publisher:Cengage Learning
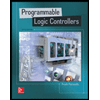
Programmable Logic Controllers
Electrical Engineering
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
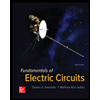
Fundamentals of Electric Circuits
Electrical Engineering
ISBN:9780078028229
Author:Charles K Alexander, Matthew Sadiku
Publisher:McGraw-Hill Education
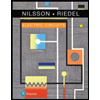
Electric Circuits. (11th Edition)
Electrical Engineering
ISBN:9780134746968
Author:James W. Nilsson, Susan Riedel
Publisher:PEARSON
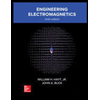
Engineering Electromagnetics
Electrical Engineering
ISBN:9780078028151
Author:Hayt, William H. (william Hart), Jr, BUCK, John A.
Publisher:Mcgraw-hill Education,
Related Questions
- Question Ach calculate the decimal equivalent of the hexadecimal number 3AE2 .Show proper steps and calculation.. .Full explain this question and text typing work only We should answer our question within 2 hours takes more time then we will reduce Rating Dont ignore this line .arrow_forwardContent: Analyze and explain further the step by step answer. Thank you.arrow_forwardSolve the problem and simplify the output function using Quine–Mc Cluskey Methods. The following requirements must be met in solving the problem. Requirement:a. Truth Tableb. Timing Diagramc. Quine – Mc Cluskey Method d. Logic Diagram A private company wants to decide on a certain issue. Each of the four officials has an equal share of voting right. At least two of them must approve the solution in order to implement it. Each of them has a switch which closes to vote YES and open to vote NO.arrow_forward
- Write assembly language program.arrow_forwardActivity 5: An automated QA machine selects the product in an industry based on the perfection of color, weight, and length which are expressed by the variables C, W, and L, respectively. If a feature is perfect the machine takes input as '1', '0' otherwise. Based on the conditions, machine decides whether to reject (R) a product or not. Assume R = 1, if at least two of the features are not perfect. Otherwise, R = 0. Solve the following.arrow_forwardthe extension of python module is .numpy O .python O .py nonearrow_forward
- • SuperNe liı. A moodle.nct.edu.om Convert the octal NUMBER 2713. 6464 to hexadecimal number showing the steps (Note : The problem should be solved in separate paper with steps and scanned copy should be submitted along with other answers Final answer must be entered in the boxes provided below. For Hexadecimal digits from A to F, enter their equivalent decimal value in the corresponding box; do not enter the letter (For eg- for A, write 10, for B write 11 etc); Enter only one digit in each box. If there is any unused box, fill it with 0 -do not leave it blank) In the written submission, use the letters A to F for hex digits instead of the values) Integer part = Fractional part = IIarrow_forwardAnswer please sir! Only correct answer will be getting thumb's up. Otherwise i will do rrate negativity.arrow_forwardUsing any design approach, plan and design the following problem: (show the Block Diagram, Truth Table, Simplification Approach Solution, Circuit Diagram) 2. Simple Security System A simple security system for two doors consists of a card reader and a keypad. Cand Reader To Door To Dor 2 To Alam Logic Circuit D E Keypad A person may open a particular door if he or she has a card containing the corresponding code and enters an authorized keypad code for that card. The outputs from the card reader are as follows: A B No card inserted Valid code for door 1 0 1 0 0 Valid code for door 2 1 1 Invalid card code 10 To unlock a door, a person must hold down the proper keys on the keypad and, then, insert the card in the reader. The authorized keypad codes for door 1 are 101 and 110, and the authorized keypad codes for door 2 are 101 and 011. If the card has an invalid code or if the wrong keypad code is entered, the alarm will ring when the card is inserted. If the correct keypad code is…arrow_forward
- Design a code converter that converts a decimal digit from BCD to excess-3 code, the input variables are organized as (A BC D) respectively with A is the MSB, the output variables are organized as (W X Y Z) respectively with W is the MSB, put the invalid decimal numbers as don't care. X= BCD'+B'D+B'C X= BC'D'+B'D+BC X= BC'D'+B'D+B'C X= BC'D'+BD+B'Carrow_forwardmodule structural_example (F, A, B, C); input A, B, C; output F; "always comment your code so you know what you didl"/ * This code define the structure of the circuit "/ * First symbol is always output for not, and, and or / I/C is inverted and stored in D, output is named I/B AND with D, store result in E, output is named I/A OR with E, store result in F not (D,C): and (E,B,D): or (FA,E): endmodule The Boolean function FIAB.C)described by the structural model of the circuit in Verilog HDL shown in the figure is O a. FIABC) -I m(4,6,7) Ob FIABC)|| M(0,1,2,3) OC FIAB.C) - TI M0.1.2.3.5,7) Od. FIABC) - E m(0,1,2,3,5,7)arrow_forwardWhy do we call some ICs universal ICs? (write your answer in the box below (do not include this in your pdf farrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Introductory Circuit Analysis (13th Edition)Electrical EngineeringISBN:9780133923605Author:Robert L. BoylestadPublisher:PEARSONDelmar's Standard Textbook Of ElectricityElectrical EngineeringISBN:9781337900348Author:Stephen L. HermanPublisher:Cengage LearningProgrammable Logic ControllersElectrical EngineeringISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
- Fundamentals of Electric CircuitsElectrical EngineeringISBN:9780078028229Author:Charles K Alexander, Matthew SadikuPublisher:McGraw-Hill EducationElectric Circuits. (11th Edition)Electrical EngineeringISBN:9780134746968Author:James W. Nilsson, Susan RiedelPublisher:PEARSONEngineering ElectromagneticsElectrical EngineeringISBN:9780078028151Author:Hayt, William H. (william Hart), Jr, BUCK, John A.Publisher:Mcgraw-hill Education,
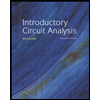
Introductory Circuit Analysis (13th Edition)
Electrical Engineering
ISBN:9780133923605
Author:Robert L. Boylestad
Publisher:PEARSON
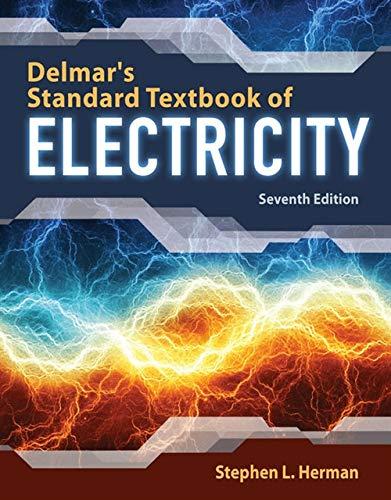
Delmar's Standard Textbook Of Electricity
Electrical Engineering
ISBN:9781337900348
Author:Stephen L. Herman
Publisher:Cengage Learning
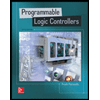
Programmable Logic Controllers
Electrical Engineering
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education
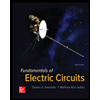
Fundamentals of Electric Circuits
Electrical Engineering
ISBN:9780078028229
Author:Charles K Alexander, Matthew Sadiku
Publisher:McGraw-Hill Education
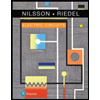
Electric Circuits. (11th Edition)
Electrical Engineering
ISBN:9780134746968
Author:James W. Nilsson, Susan Riedel
Publisher:PEARSON
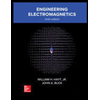
Engineering Electromagnetics
Electrical Engineering
ISBN:9780078028151
Author:Hayt, William H. (william Hart), Jr, BUCK, John A.
Publisher:Mcgraw-hill Education,