hw7_correct
.py
keyboard_arrow_up
School
Arizona State University *
*We aren’t endorsed by this school
Course
591PYTHON
Subject
Electrical Engineering
Date
Jan 9, 2024
Type
py
Pages
8
Uploaded by UltraBoulderHare16
###################################################################################
#
# Additional project for EEE591 #
# #
# The code for the first part has not been changed #
# from the previous submission. However, in my previous submission, I used combined#
# accuracies, here I am using Test accuracies as mentioned in the problem statement#
###################################################################################
#
import matplotlib.pyplot as plt
from sklearn import datasets
import numpy as np # needed for arrays
from sklearn.model_selection import train_test_split # splits database
from sklearn.preprocessing import StandardScaler # standardize data
# the algorithm
from sklearn.metrics import accuracy_score # grade the results
import pandas as pd
from pandas import DataFrame,read_csv
import seaborn as sns
from sklearn.linear_model import Perceptron # Perceptron Algorithm
from sklearn.svm import SVC # SVM Algorithm
from sklearn.linear_model import LogisticRegression # Logistic Regression Algorithm
from sklearn.ensemble import RandomForestClassifier # Random Forest Algorithm
from sklearn.neighbors import KNeighborsClassifier # KNN Algorithm
from sklearn.tree import DecisionTreeClassifier # Decision Tree Algorithm
from sklearn.tree import export_graphviz
from sklearn.model_selection import train_test_split # split the data
###################################################################################
#######
# The first part (below) of the code reads the data and #
# converts it into aalatable form #
# Note : throughout the code, ignore comments which begin with the header Debug Comments #
###################################################################################
#######
heartdata = pd.read_csv ("heart1.csv") # read the data into a dataframe
X = heartdata [['age','sex','cpt', 'rbp','sc','fbs','rer','mhr','eia','opst','dests','nmvcf','thal'
]] #extracting only the features from the dataframe
Y = heartdata [['a1p2'
]] #extracting the classifcation
X_array = X.to_numpy() #converting the dataframes to arrays for easy manipulation
Y_array = Y.to_numpy()
X_train, X_test, Y_train, Y_test= \
train_test_split(X_array,Y_array,test_size=0.3,random_state=0) #splitting data in train and test
# converting Y_train and Y_test from an array of arrays to a singular array
Y_test_normal = []
for i in Y_test :
Y_test_normal.append(i[0])
Y_test = Y_test_normal
Y_train_normal = []
for i in Y_train :
Y_train_normal.append(i[0])
Y_train = Y_train_normal
###############################################
# Converting above data into standard scalars #
###############################################
sc = StandardScaler() # create the standard scalar
sc.fit(X_train) # compute the required transformation
X_train_std = sc.transform(X_train) # apply to the training data
X_test_std = sc.transform(X_test) # and SAME transformation of test data
###################################################################################
##########
# The next part of the code runs the extracted data through various machine learning models #
# and prints out the "test accuracy", previously I used combined, but now test #
###################################################################################
##########
#########################################################
# #
# Running the above extracted data through a Perceptron #
# #
#########################################################
ppn = Perceptron(max_iter=100, tol=1e-3, eta0=0.001, # Increasing max_iter to 100 increased accuracy for me
fit_intercept=True, random_state=0, verbose=True)
ppn.fit(X_train_std, Y_train) # do the training
print ("\n")
print ("Results for perceptron are : \n")
y_pred_ppn = ppn.predict(X_test_std) # applying on test data
X_combined_std = np.vstack((X_train_std, X_test_std)) # vstack puts first array above the second in a vertical stack
y_combined = np.hstack((Y_train, Y_test)) # hstack puts first array to left of the second in a horizontal stack
# The below part shows how the combination of test and train data did, but we are not going to use it here for eee591 project
# but calculating just for reference
y_combined_pred = ppn.predict(X_combined_std)
###################################################
# printing test accuracy of perceptron #
###################################################
print('Misclassified test samples of perceptron : %d' % \
(Y_test != y_pred_ppn).sum())
print ("Test accuracy of perceptron is : %.2f" % accuracy_score(Y_test, y_pred_ppn),"\n")
####################################################
# #
# Running data through SVM #
# #
####################################################
for c_val in [0.9]: # I found c_val = 0.9 yields
highest value
svm = SVC(kernel='linear', C=c_val, random_state=0)
svm.fit(X_train_std, Y_train) # do the training
y_pred_svm = svm.predict(X_test_std) # work on the test data
# The below part shows how the combination of test and train data did, but we are not going to use it here for eee591 project
# but calculating just for reference
X_combined_std = np.vstack((X_train_std, X_test_std))
y_combined = np.hstack((Y_train, Y_test))
###################################################
# printing test accuracy of SVM #
###################################################
print('\nMisclassified test samples of SVM : %d' % \
(Y_test != y_pred_svm).sum())
print ("Test accuracy of SVM is : %.2f" % accuracy_score(Y_test, y_pred_svm),"\n")
####################################################################
# #
# Running the data through Logistic Regression #
# #
####################################################################
for c_val in [1]: # c_val = 1 yielded highes combined accuracy for me
lr = LogisticRegression(C=c_val, solver='liblinear', \
multi_class='ovr', random_state=0)
lr.fit(X_train_std, Y_train) # apply the algorithm to training data
# combine the train and test data
X_combined_std = np.vstack((X_train_std, X_test_std)) # vstack puts first array
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Write short note on the below mentioned questions, Each question carries one mark.
Students has to answer the questions typewritten in the word (.docx) format.
Figures / Diagrams, equations and solving of problems should be written by hand. Take a picture of
it and attach it inside the word file.
a) High Voltages are preferred for Transmission of Electricity. Why?
b) Why Generation Voltage Levels are low in the range of 11 kV/ 33 kV etc?
C) Why ACSR Conductors are preferred over Copper Conductors?
d) Overhead Transmission Lines are preferred for Transmission of Power for Long Distances, why?
e) Alternating Current is preferred over Direct Current in Power System, Why?
f) Write the Installed Generation Capacity of Sultanate of Oman with current energy share of Conventional and
Non-Conventional Energy Sources.
g) Why the Capacitance effect is neglected in Short Transmission Line.
h) Distinguish between Lumped or Distributed elements in a Transmission Line.
arrow_forward
NUMBER SYSTEM ADDITION
10111 BASE 2 + FEED BASE 16 + 321 BASE 8 = ____________ BASE 8
1020 BASE 10 + 101111 BASE 2 + 212 BASE 8 = ___________BASE 16
F12 BASE 16 + FAAA BASE 16 + CDA BASE 16 = ____________BASE 8
1011 BASE 2 + 1011 BASE 2 + 1101 BASE 2 + A BASE 16=_______BASE 10
444 BASE 8 + 222 BASE 8 + 101 BASE 8 + F BASE 16 = ________BASE 16
101101 BASE 2 + 1110101 BASE 2 + 11101 BASE 2 + 0101 BASE 2= _______BASE 2
arrow_forward
Q3 put "T" in front of true sentence &"F" in front of the false sentence .
A. In differently compound D.C. generator both shunt and series fields are exsit
their effects are additive.
B. Ram Air Turbine(RAT) one sorces secondary power distribution.
C. Lead acid batteries are recharged by constant volts.
arrow_forward
I need a correct answer not a wrong answer please do it
Question: Write the necessity of power supply and briefly describe different types of SMPS and UPS
arrow_forward
Explain what is Grid system?
arrow_forward
Imnlement the simplified expression for the following figure using NOR gates only:
C NOR Y
OX NOR Y
OX NOR Y)
No correct answer
OX NOR Y)
arrow_forward
Please answer fast and short in 15 mint
arrow_forward
Sir, I need more details. Thanks
arrow_forward
With schematic diagram, explain the Wind Power Plant.
arrow_forward
Calculate element and welding currents with mesh analysis.
arrow_forward
SUBJECT: INDUSTRIAL ELECTRONICS
TOPIC: Power Supply Using LM 317 & LM337
NOTE: ANSWER THE FOLLOWING QUESTIONS AND EXPLAIN AND EXPOUND YOUR
ANSWER! MAKE SURE THE ANSWER IS CORRECT TO RATE YOU HIGHLY FOR THE
ANSWER. ANSWER THE TABLE AND OTHER QUESTIONS. THANK YOU! answer with
correct, PLEASE FOLLOW INSTRUCTIONS
ANSWER IT IN CORRECT & Explain THANKS
Make a (3) titles about this project:
A.
B.
C.
Make abstract about this project:
ABSTRACT - [ is a self-contained, short, and powerful statement that describes a larger work.
Abstracts cannot contain equations, figures, or tables.]
Make an introduction about this project that contains the (1) Background of the Project
(2) Objective of the Project, (3) Scope and Limitation of the Project
INTRODUCTION
arrow_forward
id 15186 18course id- 18678 18content id 220760 18question rum t
Apps
M Gmail
YouTube
Maps
Remaining Time: 53 minutes, 27 seconds
Question Completion Status:
A Moving to another question will save this response.
Question 6
In a capacitive load, the current angle:
Lag the voltage by 90.
In phase with the voltage angle.
Between 90 and -90.
Leads the voltage by 90.
A Moving to another question will save this response.
docx. . . . اختبار الاقتصاد النه
docx. . اختبار الاقتصاد النه
A ll slaislI Lisl.docx
aill slail lil.docx
aill sle
ENG 4) A
ETVIE
69
R
T
Y
U
O
OP
S
F
G
K
1S
16
arrow_forward
The system having ±0.5% of F.S.D if the full scale deflection is 50 units, then accuracy is _____
a. ±0.25
b. ±1
c. ±0
d. ±0.5
arrow_forward
What is the total number of systems?
arrow_forward
Please answer with detail and how it is done. Photo is attached with the questions.
arrow_forward
Write the state equadion for the_netwoyK-
Shawabelow.
Uit)
"loope
343
(0op2)多R2
arrow_forward
Please answer ASAP for like this please Asap for like this please...
Please
arrow_forward
B3
arrow_forward
21
Q2/ The one
Per-unit impedaunce digram
line digram 3-Phave pouser System. Draw the
G: 50 MVA, 13.2 KU , Xg = 0.2 Pu
Tig 30MVA , 13.2/220KU, XT,=0-1s P4
Te 30MVA, 22./11 kU, X+Z=0.15pu
T3:30MVA , 13.2/132 kv, XT,=0.15P4
Tu:30MUA 132/11 KV, XTzo.15 Pu
M: 70 MVA, lo.45kv, Xm =0.2 p4
Load, 53 MVA , lo.45kv, o.7 P.R (Lag)
line1: 22o ku, Xline I=4oA
%3D
line2: 132 kv, Xlinez = 6or
Te
220
132
36
Ty
load
38
Select a Common base of 90MUA ,13.2kv
on the generator Side
arrow_forward
1. It describe the relationship of current voltage and resistance.
2. There are the available options for the pilot, In case of a single generator failure in large multiengine aircraft. Choose all the correct answers.
3. This system converts 115AC to 28VDC in most large body aircraft.
arrow_forward
explain outdoor substation
arrow_forward
Vdc0
U=12 V
Circuit
#1
Ried
V LED
www
Ried_series
0.05
Vdc0
U = 12 V
0,04
Circuit
0.03
0.02
0.01
RED LED
GREEN LE
Set current to 10 mA
LED voltages
Given the circuit #1 presented above, answer the following
questions:
BLUE_LEC
Red
0.5
1) Determine the value of the V₁ (voltage forward of the LED)
for the LEDS RED, VDRED GREEN, VREEN and BLUE, VOBLUES
when the current through the LEDs is 10mA,
2) Is the LED in Circuit #1, connected in the way that will be
lighting up or not - Justify your answer
Green
3) Calculate the value of the resistance Rled in Circuit #1, to
ensure that a RED LED will have a current of 10 mA
1.5 2 25
Voltage across LED (V)
4) Calculate the value of the resistance Rled in Circuit #1, to
ensure that a GREEN LED will have a current of 10 mA
Blue
5) Calculate the value of the resistance Rled in Circuit #1, to
ensure that a Blue LED will have a current of 10 mA
6) Given three LEDs, connected in series as shown in the Circui
# 2 (below), calculate the value of the…
arrow_forward
Answer please
arrow_forward
question 4 and 5 from picture.
4) Disadvantages of SMART GRIDs.
5) Recent Government policies in Canada in regard to Smart Grids.
I have create power presentation file so please write according to presentation and give me some pictures regarding smart grid will help a lot. reference for pictures required . thank you so much
arrow_forward
For Figure 1 switch circuit:
a. derive the switching algebra expression that corresponds one to one with the switch circuit.
b. derive an equivalent switch circuit with a structure consisting of a parallel connection of
groups of switches connected in series. (Use 9 switches.)
c. derive an equivalent switch circuit with a structure consisting of a series connection of groups
of switches connected in parallel. (Use 6 switches.)
B'
Figure 1. Switch Circuit
arrow_forward
Redraw the following pictorial diagram as a simple block diagram.
arrow_forward
What is the unit E?
arrow_forward
list the material required for overhead service connection
arrow_forward
q3/
arrow_forward
Good day, sir/ma'am. Here is the final question which I require assistance with. Thank you very much.
Question:
A power transmission line is made of copper 1 .80cm in Find the resistance of one mile of this line.
arrow_forward
What is shop drawings, why we use them?
I
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
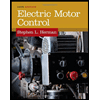
Related Questions
- Write short note on the below mentioned questions, Each question carries one mark. Students has to answer the questions typewritten in the word (.docx) format. Figures / Diagrams, equations and solving of problems should be written by hand. Take a picture of it and attach it inside the word file. a) High Voltages are preferred for Transmission of Electricity. Why? b) Why Generation Voltage Levels are low in the range of 11 kV/ 33 kV etc? C) Why ACSR Conductors are preferred over Copper Conductors? d) Overhead Transmission Lines are preferred for Transmission of Power for Long Distances, why? e) Alternating Current is preferred over Direct Current in Power System, Why? f) Write the Installed Generation Capacity of Sultanate of Oman with current energy share of Conventional and Non-Conventional Energy Sources. g) Why the Capacitance effect is neglected in Short Transmission Line. h) Distinguish between Lumped or Distributed elements in a Transmission Line.arrow_forwardNUMBER SYSTEM ADDITION 10111 BASE 2 + FEED BASE 16 + 321 BASE 8 = ____________ BASE 8 1020 BASE 10 + 101111 BASE 2 + 212 BASE 8 = ___________BASE 16 F12 BASE 16 + FAAA BASE 16 + CDA BASE 16 = ____________BASE 8 1011 BASE 2 + 1011 BASE 2 + 1101 BASE 2 + A BASE 16=_______BASE 10 444 BASE 8 + 222 BASE 8 + 101 BASE 8 + F BASE 16 = ________BASE 16 101101 BASE 2 + 1110101 BASE 2 + 11101 BASE 2 + 0101 BASE 2= _______BASE 2arrow_forwardQ3 put "T" in front of true sentence &"F" in front of the false sentence . A. In differently compound D.C. generator both shunt and series fields are exsit their effects are additive. B. Ram Air Turbine(RAT) one sorces secondary power distribution. C. Lead acid batteries are recharged by constant volts.arrow_forward
- I need a correct answer not a wrong answer please do it Question: Write the necessity of power supply and briefly describe different types of SMPS and UPSarrow_forwardExplain what is Grid system?arrow_forwardImnlement the simplified expression for the following figure using NOR gates only: C NOR Y OX NOR Y OX NOR Y) No correct answer OX NOR Y)arrow_forward
- Calculate element and welding currents with mesh analysis.arrow_forwardSUBJECT: INDUSTRIAL ELECTRONICS TOPIC: Power Supply Using LM 317 & LM337 NOTE: ANSWER THE FOLLOWING QUESTIONS AND EXPLAIN AND EXPOUND YOUR ANSWER! MAKE SURE THE ANSWER IS CORRECT TO RATE YOU HIGHLY FOR THE ANSWER. ANSWER THE TABLE AND OTHER QUESTIONS. THANK YOU! answer with correct, PLEASE FOLLOW INSTRUCTIONS ANSWER IT IN CORRECT & Explain THANKS Make a (3) titles about this project: A. B. C. Make abstract about this project: ABSTRACT - [ is a self-contained, short, and powerful statement that describes a larger work. Abstracts cannot contain equations, figures, or tables.] Make an introduction about this project that contains the (1) Background of the Project (2) Objective of the Project, (3) Scope and Limitation of the Project INTRODUCTIONarrow_forwardid 15186 18course id- 18678 18content id 220760 18question rum t Apps M Gmail YouTube Maps Remaining Time: 53 minutes, 27 seconds Question Completion Status: A Moving to another question will save this response. Question 6 In a capacitive load, the current angle: Lag the voltage by 90. In phase with the voltage angle. Between 90 and -90. Leads the voltage by 90. A Moving to another question will save this response. docx. . . . اختبار الاقتصاد النه docx. . اختبار الاقتصاد النه A ll slaislI Lisl.docx aill slail lil.docx aill sle ENG 4) A ETVIE 69 R T Y U O OP S F G K 1S 16arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
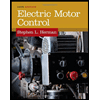