lab 10
.pdf
keyboard_arrow_up
School
San Jose State University *
*We aren’t endorsed by this school
Course
130
Subject
Mechanical Engineering
Date
Feb 20, 2024
Type
Pages
11
Uploaded by PrivateRook16679
Lab
10:
Solving
Nonlinear
Equations
|l
©
Matthew
Leineweber,
PhD
BME
130
-
Numerical
Methods
in
Biomedical
Engineering
San
Jose
State
University
Instructor:Prof.
Abdulmelik
Mohammed
Lab
TA:
Nihar
Prakash,
Ammar
Babiker
By:
Philippe
Moffet,
Lab
Group
#:
03,
Year:
Fall
2023
Table
of
Contents
Introduction
Anonymous
Functions
Activity
1
Activity
2
Newton
Raphson
Method
Activity
3
Activity
4
Using
MATLAB's
fzero
to
Solve
Nonlinear
Equations
Activity
5
Activity
6
Using
fsolve
Activity
7
Post
Lab
References
and
Documentation
Function
Definitions
Newton
Raphson
Function
(to
be
completed
by
student)
Introduction
So
far
we
have
been
exploring
“bracketing
methods”
for
solving
nonlinear
equations,
namely
the
method
of
False
Position
(a.k.a
Interpolation)
and
the
Bisection
method.
These
techniques
are
so
called
because
they
converge
on
a
solution
by
successively
iterating
a
pair
of
boundary
points
that
lie
on
either
side
of
the
root
until
the
range
between
the
boundaries
is
negligibly
small.
Of
these
two
methods,
the
False
Position
technique
will
typically
converge
faster
than
the
Bisection
method
for
functions
with
steep
slopes
near
the
root,
but
may
take
significantly
longer
if
the
slope
is
very
shallow.
We
also
saw
how
we
can use
function
handles
to
pass
functions
as
input
arguments
into
other
functions.
This
capability
becomes
extremely
useful
trying
to
iteratively
solve
equations,
since
it
allows
us
to
write
a
single
solver
function
(e.g.
FalsePosition2)
to
be
used
with
any
expression
to
be
solved.
However,
defining
standalone
function
files
or
sub-functions
can
be
somewhat
cumbersome
when
all
our
function
needs
to
do
is
evaluate
a
single
mathematical
expression.
Luckily,
MATLAB
allows
us
to
define
anonymous
functions
that
can
streamline
this
process
allow
us
to
define
simple
functions
on
the
fly.
In
today’s
lab
we
will
build
off
the
foundation
we
laid
last
week
to
introduce
a
new
technique
for
solving
nonlinear
equations,
and
show
how
we
can use
anonymous
functions
to
simplify
the
solving
process.
This
new
solver,
the
Newton
Raphson
method,
will
often
converge
much
faster
than
our
previous
methods
(i.e.
require
fewer
iterations).
We
will
also
explore
how
we
can
use
some
of
MATLAB'’s
pre-built
functions
to
solve
equations
as
well.
Anonymous
Functions
We've
already
seen
how
writing
functions
and
sub-functions
makes
our
lives
much
easier
by
removing
the
need
to
customize
every
section
of
code
to
tailor
a
specific
problem.
However,
if
we
only
want
our
function
to
perform
one
or
two
steps,
such
as
evaluating
the
result
of
a
mathematical
expression,
writing
an
entire
function
m-file
or
sub-function
can
be
tedious
a
little
cumbersome.
Enter
the
anonymous
function.
Anonymous
functions
can
accept
inputs
and
return
outputs,
like
a
regular
function,
but
they
contain
only
one
or
two
executable
lines
of
code,
and
can
be
defined
at
the
command
line,
in
a
script,
or
within
another
user-defined
function.
These
special
functions
are
defined
with
the
format:
fun
=
@(inputArguments)
expr
Here,
fun
is
the
name
of
the
anonymous
function,
inputArguments
is
the
list
of
input
arguments,
and
expr
is
the
single
line
of
code
(expression)
for
the
function.
In
this
anonymous
function
definition,
the
‘@
symbol
tells
MATLAB
that
fun
will
be
a
function
with
input
variable:
inputArguments.
You
may
recognize
the
use
of
the
‘@
symbol
from
our
discussion
of
function
handles
last
week.
Anonymous
functions
basically
combine
a
function
definition
and
a
function
handle
into
a
single
statement.
For
example,
if
we
were
to
write
an
anonymous
function
that
takes
an
input
xand
returns
its
cube,
x3,
we
would
write:
cube
=
@
(x)
x"3
cube
=
function_handle
with
value:
@(x)x"3
Once
an
anonymous
function
is
defined,
it
can
be
used
to
evaluate
the
function
when
the
input
variable
is
assigned
a
specific
value:
cube(2)
ans
=
8
Which
is
just
the
value
x°
evaluated
at
x
=
3.
As
shown
above,
to
call
(use)
an
anonymous
function,
simply
type
the
name
of
the
anonymous
function,
followed
by
the
value
for
the
input
variable
in
parentheses.
If
you
wish
to
assign
an
array
to
the
input
variable
rather
than
a
single
scalar
value,
you
must
first
define
the
anonymous
function
with
element-by-element
powers
instead:
n
=
I1x3
cube
=
@
(x)
x.”3
cube
=
function
_handle
with
value:
@(x)x.”"3
cube(n)
ans
=
1x3
1
8
27
Activity
1
Define
an
anonymous
function
to
perform
the
following
calculation
f(x)
=
X
+2x+2—
10&3_2’(2
Then
use
the
function
to
evaluate
f(x)
for
x=5
andfor
x=[-1
4
9]
%
Define
the
anonymous
function
x=@(x)x."3+(2*x)+2-
(10*exp(-2*x.72))
fx
=
function_handle
with
value:
@(x)x."3+(2*x)+2-(10*exp(-2*x."2))
Run
the
function
to
perform
the
calculation
on
the
following
inputs
%
Define
the
x-values
to
be
tested
X1l
=
5;
xr
=
[-1
4
9];
Assign
the
result
of
f(x)
to
variables
f1
and
f2,
respectively.
%
Evaluate
fx1
=
f(x1)
and
fx2
=
f(x2)
f1
=
fx(x1)
f1
=
137
2
=
fx(xr)
f2
=
1x3
-2.3534
74.0000
749.0000
So
far
we
have
explored
anonymous
functions
with
only
one
input
variable,
however,
it's
possible
to
have
several
input
variables
(e.g.
x,
y,
z,
etc.).
To
write
an
anonymous
function
for
the
Pythagorean
Theorem,
which
we’ll
call
hypotenuse,
we
would
have
two
input
variables,
a
and
b,
representing
the
lengths
of
the
sides
of
a
right
triangle,
and
the
output
will
be
the
length
of
the
hypotenuse.
hypotenuse
=
@
(a,b)
sqrt(a”2+b”2)
hypotenuse
=
function
handle
with
value:
@(a,b)sgrt(a*2+b”2)
To
use
multiple
input
variables
in
an
anonymous
function,
just
separate
them
by
commas
in
parentheses
after
the
“@”.
Let’s
evaluate
the
function
for
¢
=
3
and
»
=
4:
hypotenuse(3,4)
ans
=
5
What
if
you
would
like
to
evaluate
several
values
in
a
multivariable
function?
The
first
step,
just
like
with
single
variable
functions,
is
to
define
the
anonymous
function
element-by-element:
hypotenuse
=
@
(a,b)
sqrt(a.”2+b.”2)
hypotenuse
=
function_handle
with
value:
@(a,b)sgrt(a.”2+b.”2)
Now,
let’s
find
the
hypotenuse
length
for
two
different
triangles,
one
with
sides
length
5
and
12,
and
the
other
with
sides
length
7
and
24
hypotenuse([5,7],[12,24])
ans
=
1x2
13
25
Where
13
is
the
output
corresponding
to
the
|5,
7]
input,
and
25
is
the
output
corresponding
to
the
[12,24]
input.
You'll
notice
that
square
brackets
were
used
for
each
of
the
two
input
variables,
a
and
b.
It
looks
confusing
that
terms
from
different
triangles
are
grouped
together,
but
in
order
for
MATLAB
to
compute
the
function
as
desired,
this
format
is
necessary.
Note,
that
the
previous
line
of
code
is
equivalent
to
the
following
set
of
commands:
A=
[5,7];
B
[12,24];
hypotenuse(A,B)
ans
=
1x2
13
25
You
can
also
use
pre-defined
variables
in
an
anonymous
function’s
mathematical
expression,
but
note
that
if
you
assign
new
values
to
variables
used
in
your
anonymous
function,
the
original
values
will
still
be
used
unless
you
redefine
your
anonymous
function.
Activity
2
Let’s
illustrate
this
last
point
using
the
code
below.
Before
running
the
code,
write
down
what
you
think
the
values
for
output?
and
output2
should
be.
%
Define
Variables
'a'
and
'b':
a
=
3;
b
=
4;
%
Define
the
anonymous
function
fun
=
@(x,y)
a*x
+
b*y;
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
Identify the lines
arrow_forward
Motiyo
Add explanation
arrow_forward
Plz solve within 30min I vill give definitely upvote and vill give positive feedback thank you
arrow_forward
permanent-magnet (pm) genera x
Bb Blackboard Learn
L STAND-ALONE.mp4 - Google Dri x
O Google Drive: ülwgjuó jc lis u
O ME526-WindEnergy-L25-Shuja.p x
O File | C:/Users/Administrator/Desktop/KFUPM%20Term%232/ME526/ME526-WindEnergy-L25-Shuja.pdf
(D Page view
A Read aloud
T) Add text
V Draw
Y Highlight
O Erase
17
of 26
Wind Farms
Consider the arrangement of three wind turbines in the following schematic in which wind
turbine C is in the wakes of turbines A and B.
Given the following:
- Uo = 12 m/s
A
-XẠC = 500 m
-XBC = 200 m
- z = 60 m
- Zo = 0.3 m
U.
-r, = 20 m
B
- CT = 0.88
Compute the total velocity deficit, udef(C) and the velocity at wind turbine C, namely Vc.
Activate Windows
Go to Settings to activate Windows.
Wind Farms (Example Answer)
5:43 PM
A 4)) ENG
5/3/2022
I!
arrow_forward
Please do not copy other's work and do not use ChatGPT or Gpt4,i will be very very very appreciate!!!
Thanks a lot!!!!!
arrow_forward
https://ethuto.cut.ac.za/webapps x
Content
Take Test: Main assessment link - x
ethuto.cut.ac.za/webapps/assessment/take/launch.jsp?course_assessment_id= 26832 1&course_id3_10885_1&content_id%3 625774 1&step3Dnull
Question Completion Status:
QUESTION 20
A single-stage, single-acting air compressor with runs at speed 600 rpm. It has a polytropic index of
1.26. The induced volume flow rate is 7.2 cubic meters per min and the air must be delivered at a
pressure of 668 kPa. The free air conditions are 101.3 kPa and 18 degrees Celsius. The clearance
volume is 6% of the stroke volume and the stroke/bore ratio is 1.6/1. Calculate the delivery air.
Hint answer usaully in C and no need for °C.
arrow_forward
- Moos X
O CIVL3106_yahiaz_sprir x
Part 1
b Answered: soil mechar x
elearn.squ.edu.om/mod/quiz/attempt.php?attempt3D2055593&cmid=903343
IO YouTube Maps
التعلم القبلي G
IG SYSTEM (ACADEMIC)
raur. p
Time le
From the figure below determine Cc (compression index)
1.9
1.7
1.5
1.3
1.1
0.9
0.7
0.5 +
10
100
1000
Pressure (kPa)
Paragraph
BI
Void ratio
!!
arrow_forward
kamihq.com/web/viewer.html?state%=D%7B"ids"%3A%5B"1vSrSXbH_6clkKyVVKKAtzZb_GOMRwrCG"%5D%...
lasses
Gmail
Copy of mom it for..
Маps
OGOld Telephone Ima.
Preview attachmen...
Kami Uploads ►
Sylvanus Gator - Mechanical Advantage Practice Sheet.pdf
rec
Times New Roman
14px
1.5pt
BIUSA
A Xa x* 三三
To find the Mechanical Advantage of ANY simple machine when given the force, use MA = R/E.
1.
An Effort force of 30N is appliled to a screwdriver to pry the lid off of a can of paint. The
screwdriver applies 90N of force to the lid. What is the MA of the screwdriver?
MA =
arrow_forward
please in detaild
arrow_forward
QUESTION 1
In 2020, the world faces a critical health issue called Covid-19. During this period, the
government of Malaysia have implemented Movement Control Order (MCO) where many
sectors have been closed temporarily. This implementation has caused school to shut down and
all class activities conducted through online. Same goes to private sectors. This has caused
electricity usage increased rapidly.
AM
PM
Image courtesy : https://bit.ly/3eAqi7j)
As an owner of house in Figure 1, identify three renewable resources and elaborate how can
you utilise your house, to harvest energy from environment. In your explanation:
a.
Include the location of installation
b.
Any criteria or reason for the selection of (a)
Drawback or side effect of the chosen methods.
с.
d.
If structure need modification, explain the need of the modification (If any)
arrow_forward
Don't Use Chat GPT Will Upvote And Give Handwritten Solution Please
arrow_forward
MECT361
Mechatronics Components and Instrumentation
Please solve the problem in keupord
arrow_forward
O 17161489
C Get Homework Help With
b My Questions | bartleby
O Mail - Castro Alvarez, Flavic X
Content
A learn-us-east-1-prod-fleet01-xythos.s3.amazonaws.com/5c1270dbb5a74/17161489?response-cache-control=private%2C%20max-age%3D21600&respon. ☆
ME 3022 - HW 19
1. (More practice from Monday) A 90° elbow in a horizontal pipe is used to
direct a flow of oil (SG = 0.87) upward at a rate of 40 kg/s. The cross-
sectional diameter of the elbow is a constant 20 cm. The elbow discharges at
A into a large holding tank where the level of oil is 1.8 m above A. The
50 cm
"weight" of the elbow and the oil in it is 50 kg. Determine (a) the gage
pressure at the inlet of the elbow and (b) the anchoring force needed to hold
the elbow stationary. Take the momentum-flux correction factor to be 1.03
40 kg/s
at both the inlet and the outlet.
Activar Windows
Ve a Configuración para activar Windows.
Esperando blackboard.ohio.edu.
17:07
P Escribe aquí para buscar
a ) ENG
14/10/2020
近
arrow_forward
use LMTD for part a and b
arrow_forward
HW_5_01P.pdf
PDF
File | C:/Users/Esther/Downloads/HW_5_01P.pdf
2 Would you like to set Microsoft Edge as your default browser?
Set as default
To be most productive with Microsoft Edge, finish setting up your
Complete setup
Maybe later
browser.
(D Page view A Read aloud V Draw
7 Highlight
2
of 3
Erase
5. Two cables are tied to the 2.0 kg ball shown below. The ball revolves in a horizontal
circle at constant speed. (Hint: You will need to use some geometry and properties of
triangles and their angles!)
60°
1.0 m
60°
© 2013 Pearson Education, Inc.
(a) For what speed is the tension the same in both cables?
(b) What is the tension?
2.
2:04 PM
O Type here to search
C A
2/9/2021
(8)
arrow_forward
Hello I'm having trouble with this assignment, I don't understand how the plot function works for ordered pairs. I undertand that the points of the line would be the origin(0,0) and (x = cos(theta) . y = sin(theta)).
arrow_forward
pls help me to answer this questions
arrow_forward
Part III Capstone Problem
Interactive Physics - [Lab7Part3.IP]
Eile Edit World View Object Define Measure Script Window Help
Run StoplI Reset
圖|& 品凸?
Time
Length of Spring 22
6.00
dx
Center of Mass of Rectangle 2
5.000
Tension of Rope 3
Jain@
IFI
... N
ot
rot
***lad
Split
4.000
Velocity of Center of Mass of Rectangle 2
Vx Vx
V Vy
MM
Ve
- m/s
m/s
3.00
*** m/s
Vo
..* lad/s
2 00
Center of Mass of Rectangle 1
1.000
tol
rot
*.* rad
EVelocity of Center of Mass of Rectangle 1
Vx Vx
VVy
M
0.000
-m/s
w 30
m/s
w..
MI
Ve
母100
*** m/s
Vo
... rad/s
+
EAcceleration of Center of Mass of Rectangle 1
Ax Ax
A Ay
AUJAI
Ae
--- m/s^2
... m/s^2
-- m/s^2
.-- rad/s^2
3.00
Aø
Mass M1 = 2.25 kg is at the end of a rope that is 2.00 m in length. The initial angle with
respect to the vertical is 60.0° and M1 is initially at rest. Mass M1 is released and strikes M2
= 4.50 kg exactly horizontally. The collision is elastic. After collision, mass M2 is moving on
a frictionless surface, but runs into a rough patch 2.00…
arrow_forward
PHYS 14 midterms - Word
Carl John Rivas
O X
File
Home
Insert
Design
Layout
References
Mailings
Review
View
Help
O Tell me what you want to do
유 Share
X Cut
P Find
Arial
- 10
A A
Aa -
AaBbCcDdE AaBbCcDdE AaBbCc AaBbCcDc A aBI
自 Copy
abc Replace
Paste
A . aly v A
1 Normal
1 No Spaci. Heading 1
Heading 2
V Format Painter
v abe
X,
Title
A Select
Clipboard
Font
Paragraph
Styles
Editing
A= 2x + 3y
B=-2x + y
4. A lightweight drone (1.00 kg) is launched at 800 m high and moves upward at a constant velocity (while
ignoring the effects of gravity only on the drone). The balloon, when measured at a horizontal distance from
you, is about 1600 m away from you. At the moment when the drone moves, you shoot a bullet (weight =180
g) with an initial velocity of 1009 m/s at a fixed angle a, where sin a=3/5 and cos a= 4/5. (g = 9.8 m/s?) (10
pts each)
a. How long does it take for the bullet to collide with the drone?
b. At what height will the bullet hit it?
Page 1 of 2
542 words
170%
PC
A O 4) 6:51 PM
arrow_forward
immediately
arrow_forward
Plz solve within 30 min I vill definitely upvote
arrow_forward
+ → CO
A student.masteryconnect.com/?iv%3D_n5SY3Pv5S17e01Piby
Gr 8 Sci Bench 1 GradeCam Rutherford TN 2021
AHMAD, ASHNA
D0
3 of 35
A student develops a model of an electric motor using two pins, a wire coil,
coil continues to spin with a certain speed.
wire coil
pins
magnet
tape
battery
How can the student increase the speed of the electric motor?
O by using wider pins
O by using thinner pins
O by using less wire in the clil
O by using more wire in the coil
e Type here to search
近
arrow_forward
i need the answer quickly
arrow_forward
Dessert - sealed
Date:
Time:
Made: 28/11/21 18:22
Ready: 28/11/21 20:22
Discard:03/12/21 18:22 Fri
OLOGY BLANCHARDSTOWN - Google Chrome
pluginfile.php/394133/mod_resource/content/1/PME1%20Sample%20Class%20Test%20Dec%202021.pdf
FTECHNOLOGY BLANCHARDSTOWN
2/9 |
250% + | E O
a) Calculate the principal stresses, given that the state of plane stress for an element is:
Oy = 65MPA,
Oy =-55MPA,
Txy =-30MPa
TXY
Y
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
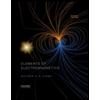
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
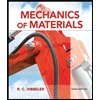
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
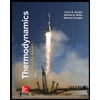
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
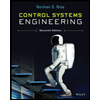
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
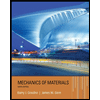
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
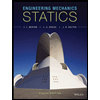
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- permanent-magnet (pm) genera x Bb Blackboard Learn L STAND-ALONE.mp4 - Google Dri x O Google Drive: ülwgjuó jc lis u O ME526-WindEnergy-L25-Shuja.p x O File | C:/Users/Administrator/Desktop/KFUPM%20Term%232/ME526/ME526-WindEnergy-L25-Shuja.pdf (D Page view A Read aloud T) Add text V Draw Y Highlight O Erase 17 of 26 Wind Farms Consider the arrangement of three wind turbines in the following schematic in which wind turbine C is in the wakes of turbines A and B. Given the following: - Uo = 12 m/s A -XẠC = 500 m -XBC = 200 m - z = 60 m - Zo = 0.3 m U. -r, = 20 m B - CT = 0.88 Compute the total velocity deficit, udef(C) and the velocity at wind turbine C, namely Vc. Activate Windows Go to Settings to activate Windows. Wind Farms (Example Answer) 5:43 PM A 4)) ENG 5/3/2022 I!arrow_forwardPlease do not copy other's work and do not use ChatGPT or Gpt4,i will be very very very appreciate!!! Thanks a lot!!!!!arrow_forwardhttps://ethuto.cut.ac.za/webapps x Content Take Test: Main assessment link - x ethuto.cut.ac.za/webapps/assessment/take/launch.jsp?course_assessment_id= 26832 1&course_id3_10885_1&content_id%3 625774 1&step3Dnull Question Completion Status: QUESTION 20 A single-stage, single-acting air compressor with runs at speed 600 rpm. It has a polytropic index of 1.26. The induced volume flow rate is 7.2 cubic meters per min and the air must be delivered at a pressure of 668 kPa. The free air conditions are 101.3 kPa and 18 degrees Celsius. The clearance volume is 6% of the stroke volume and the stroke/bore ratio is 1.6/1. Calculate the delivery air. Hint answer usaully in C and no need for °C.arrow_forward
- - Moos X O CIVL3106_yahiaz_sprir x Part 1 b Answered: soil mechar x elearn.squ.edu.om/mod/quiz/attempt.php?attempt3D2055593&cmid=903343 IO YouTube Maps التعلم القبلي G IG SYSTEM (ACADEMIC) raur. p Time le From the figure below determine Cc (compression index) 1.9 1.7 1.5 1.3 1.1 0.9 0.7 0.5 + 10 100 1000 Pressure (kPa) Paragraph BI Void ratio !!arrow_forwardkamihq.com/web/viewer.html?state%=D%7B"ids"%3A%5B"1vSrSXbH_6clkKyVVKKAtzZb_GOMRwrCG"%5D%... lasses Gmail Copy of mom it for.. Маps OGOld Telephone Ima. Preview attachmen... Kami Uploads ► Sylvanus Gator - Mechanical Advantage Practice Sheet.pdf rec Times New Roman 14px 1.5pt BIUSA A Xa x* 三三 To find the Mechanical Advantage of ANY simple machine when given the force, use MA = R/E. 1. An Effort force of 30N is appliled to a screwdriver to pry the lid off of a can of paint. The screwdriver applies 90N of force to the lid. What is the MA of the screwdriver? MA =arrow_forwardplease in detaildarrow_forward
- QUESTION 1 In 2020, the world faces a critical health issue called Covid-19. During this period, the government of Malaysia have implemented Movement Control Order (MCO) where many sectors have been closed temporarily. This implementation has caused school to shut down and all class activities conducted through online. Same goes to private sectors. This has caused electricity usage increased rapidly. AM PM Image courtesy : https://bit.ly/3eAqi7j) As an owner of house in Figure 1, identify three renewable resources and elaborate how can you utilise your house, to harvest energy from environment. In your explanation: a. Include the location of installation b. Any criteria or reason for the selection of (a) Drawback or side effect of the chosen methods. с. d. If structure need modification, explain the need of the modification (If any)arrow_forwardDon't Use Chat GPT Will Upvote And Give Handwritten Solution Pleasearrow_forwardMECT361 Mechatronics Components and Instrumentation Please solve the problem in keupordarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
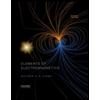
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
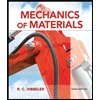
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
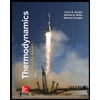
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
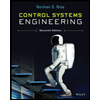
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
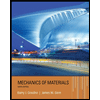
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
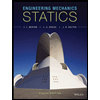
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY