Lab 3 - Processing and Analyzing Data (1)
.pdf
keyboard_arrow_up
School
Oregon State University, Corvallis *
*We aren’t endorsed by this school
Course
351
Subject
Mechanical Engineering
Date
Dec 6, 2023
Type
Pages
10
Uploaded by SuperRose6727
Page 1 of 10
Lab 3 - Processing and Analyzing Data
ME 351 - Introduction to Instrumentation and Measurement Systems
Lab Days: 2
What we’ll learn:
■
How to do frequency analysis
○
MATLAB FFT package
■
How to filter data
○
Moving average filter (software low-pass filter)
○
MATLAB low-pass and high-pass
Signoffs
Email all signoff videos to:
lab_3_s.8rz5dzg2xv47xnkb@u.box.com
Part 1: Finding Trends in Data
Before, we’ve extracted raw data from sensors. But oftentimes, we’re more interested in
trends than each data point. For instance, data may be regularly repeating, and we may
want to know how frequently it repeats. We can try to do so by determining how many
times it peaks during a set time.
Tasks
1.
Open your MATLAB plotting script for your photocell data from Lab 2.
2.
Write a program using the
findpeaks()
function built into MATLAB
(
mathworks.com/help/signal/ref/findpeaks.html
) that counts the number of
times your data “peaks”, or has a high po
int.
a.
Note:
You
do not need
to
use the additional options (e.g
“MinPeakDistance”). The most basic version of
findpeaks()
is fine.
3.
Plot the position of the peaks your program identified (using the
scatter()
function) along with your raw data (using the
plot()
function).
4.
Find and record the average frequency of the strobe light used in the last lab
according to your program.
a.
Using the
[pks, locs]
results from
findpeaks()
helps with this.
Page 2 of 10
Discussion Question 1
Does the most basic version of
findpeaks()
tend to overestimate or underestimate the
frequency of the signal (the trend in the data)? Why do you think this is?
Tasks, Continued
Another way to find trends is to analyze data to try to approximate it as waves and see
if they fit well - this is called frequency analysis, and we can do it in MATLAB using fast
Fourier transforms, or
fft()
(
mathworks.com/help/matlab/ref/fft.html
).
5.
Go to the MATLAB help page for the fast Fourier transform function.
6.
Using the “Noisy Signal” example, analyze
your own photocell data
with
fft()
.
a.
Keep in mind that the example generates data for analysis while we
already have data to analyze. Make sure you are running the analysis on
your own data!
b.
The initial parameters must also be changed to fit our data. You can
determine the real sampling frequency (Fs) by finding the average
sampling period (difference between timestamps) and dividing 1 by it.
Remember
to convert the period from milliseconds to seconds first.
i.
Note:
Sampling frequency (Fs) is not a time difference. It is a
frequency with units of Hz. If your FFT plot looks strange, the first
place you should check to debug is your value for Fs.
7.
Plot the single-sided spectrum analysis (P1) with respect to frequency in Hz.
8.
Change the x-axis limits of your plot if necessary to ensure the frequency value
associated with the peak is obvious to those looking at your graph.
9.
Find and record the frequency of the light according to the FFT analysis.
10.
Combine your peak-finding graph and your FFT graph as subplots in the same
MATLAB figure (
mathworks.com/help/matlab/ref/subplot.html
).
11.
Show your figure to a teacher for sign-off 1.
12.
Alternatively, save your figure and submit it via email for sign-off 1.
a.
DO NOT
take a screenshot of the figure. Save the plot programmatically
with
print
or
saveas
, or, in the figure window, use [File] →
[Save as].
b.
Save the file as an .jpg, .jpeg., .png, or .pdf file and title it
“
Firstname_Lastname-L3-
S3”. The file must be smaller than 25 MB.
c.
Email the file to the
box
account above. You should receive a confirmation
email that the file was uploaded successfully.
Page 3 of 10
13.
Make a copy of your MATLAB code for your lab report.
Discussion Question 2
Include the plot of your most basic version of
findpeaks()
and your
fft()
plot
generated in the above tasks. What frequencies did you find for peak-finding and for
FFT? How do your frequencies compare between peak-finding and FFT? If they are
different, which one do you think is more accurate and why? You may find it helpful to
do some simple visual analysis of your data. Keep in mind the formatting instructions
on the template for figures.
Discussion Question 3
In your FFT plot, you might see that there is a large peak at 0Hz. What does this peak
represent? Hint: If a wave has a frequency of 0, does it oscillate or is it constant?
Part 2: Filtering A Noisy Sensor
Filtering is particularly helpful for noisy sensors. One very common noisy sensor is an
inertial measurement unit (IMU) - a sensor that can measure some aspects of the
motion it’s experiencing.
Since our kits don’t come with an IMU, we have provided noisy data from an IMU on
Canvas. We’re using the MPU
-6050, an often-used IMU with an accelerometer and
gyroscope in it. Because of its popularity, there are several guides and libraries for its
use. If you need to get your own sensors for a project in the future, be sure to search for
guides and libraries before you buy! Planning ahead like this can save you quite a lot of
headache.
Tasks
1.
Go to this Adafruit MPU-6050 guide to learn about the sensor used to collect this
data:
learn.adafruit.com/mpu6050-6-dof-accelerometer-and-gyro/overview
2.
Watch this video to learn how an accelerometer works:
youtu.be/KZVgKu6v808
Discussion Question 4
How does a 1-axis accelerometer work? How might an accelerometer measure in 3
axes? How many axes does the MPU-6050 measure in?
Tasks, Continued
Noise can confuse our analyses, since they have to separate the real data (signal) from
the random errors in the data (noise). The more random error, the noisier the data is. We
can alleviate the effects of noise by filtering the data before we analyze it. One of the
Page 4 of 10
easiest ways to do so is to add a real-time moving average filter. Real-time filters are
also useful for when your system acts on incoming data and is sensitive to noise. If you
want your system to behave appropriately, you will often have to implement real-time
filtering to avoid acting on noise, rather than the intended signal.
An Arduino example for a real-time moving average filter can be found
by going to your Arduino IDE and opening [File] → [Examples] →
[03.Analog] → [Smoothing]. You can
use this to smooth noisy data from
any sensor you can read data from (keep in mind for final project).
Since you do not have access to an IMU for the Arduino, we will not be using a
real-time
moving average filter. Instead, you will clean the data in post-processing by using pre-
recorded data and running a moving average filter on it in MATLAB. The recorded data
is from flipping the IMU around the Z-axis in time with a metronome set to 90 BPM
(
https://youtu.be/I7mFvUl9HjA
).
Tasks
1.
Download the pre-
recorded IMU data “imu_flipping_data.csv” from Canvas.
a.
The first column is time in milliseconds and the second column is the
acceleration read by the z-axis accelerometer.
2.
Download th
e starter code “moving_avg_filter_starter_code.m
”
from Canvas.
3.
Read carefully through the provided code to understand how a moving average
filter works.
4.
To compare the effects of window size on our data, filter the raw data with
window sizes 10, 50, and 100.
5.
Plot your filtered signal at each window size on a single subplot (raw, 10, 50,
100). Run an FFT analysis on each set of data (4 in total), and plot each individual
FFT analysis as a subplot under the combined plot of raw and filtered data. (The
result should be five vertically stacked subplots.)
a.
Note: If your FFT plots don’t look right, make sure you convert from
milliseconds to seconds and that your
Fs
value is appropriate.
6.
Show your figure to a teacher for sign-off 2.
7.
Alternatively, save your figure and submit it via email for sign-off 2.
a.
DO NOT
take a screenshot of the figure. Save the plot programmatically
with
print
or
saveas
, or, in the figure window, use [File] →
[Save as].
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Questions
solve 2nd question
arrow_forward
:4G
10:48
Shashi
Just now
Engineering MechanCTE A1 - Lopy - Copy Canspat ibylity Mode- Word (Product Activation Iasiled)
INSTRT
DESIGN
FAGE LAVOUT
RETERENCES
MAILINGS
REVIEW
VIEW
Times New Ri-|18
KA Aa E-E- E. EE TAaBbCc[ AaBbCc[ AaBbC AaBbCc AaB AuBbCcD AaBbCcC AaBbCcl
BIU X, x* A- - A
1 Normai TNo Spac Houding Heading 2
Titte
Subtine
Subtle Em
Enptiat le
Paah
Set
Fas = 0.776 KN (C).
Question 2: Determine the force in members BC, CF, and FE. State if the
members are in tension or compression.
4 ft
B
4 ft
4 ft
-4 ft-
600 lb
600 lb
800 lb
3E ENGLISH (UNTED STATES)
Nidhi is presenting
arrow_forward
Investigate, and select appropriate sensors for the specified system.
arrow_forward
PLEASE ANSWER NUMBER 14.MECH 221-KINEMATICS: PLEASE GIVE DETAILED ANSWER AND CORRECT ANSWERS. I WILL REPORT TO BARTLEBY THOSE TUTORS WHO WILL GIVE INCORRECT ANSWERS.
arrow_forward
I need help solving this problem.
arrow_forward
9:19 M
Yo, 4G+ ul l
LTE1
1c576dc540f8a78ffa178324d524@thread.tacv2
ch
have started. For now, meeting transcripts are only available to select Teams users. Privacy policy
Di
Unit 13 - Microsoft PowerPoint
ANIMATIONS
Sign in
TRANSITIONS
SLIDE SHOW
REVIEW
VIEW
Nitro Pro 9
E tE. IlN Text Direction
CO) Align Text
Convert to SmartArt
O\\D00-
A Find
Z Shape Outline Replace
A Select
32 A A E-E
a Shape Fill-
BIUS alx AV- Aa
4 n Arrange Quick
Styles - O Shape Effects
Font
Paragraph
Drawing
Editing
ΤΥΡE Β
Example: 1 A rigid bar AB, 9 m long, is
supported by two vertical rods at its end and
in a horizontal position under a load P as
shown in figure. Find the position of the load
P so that the bar AB remains horizontal.
5 m
A = 445 mm 2
A = 1000 mm 2
9 m
E = 2 x 10 5
E = 1 x 10 5
3m
A
B
01:01:20
to add notes
NOTES
COMMENTS
9 ::
7 --
70% X
Activate Windows
20 Settings to activate
202002090096 AKHIL...
202002090069 pravi.
202101040208 Manis..
No
1=
1/
86
arrow_forward
Subject: Mechanical Measurements
Do not copy other online answers
arrow_forward
REC
M Gmail
x4 BIT203 - Google x
Gmail: Free. Pria x
Dashboard
BIT203-Study W x
docs.google.com/document/d/1unVPvr_GhUFhrQuNdGICLqFle1ZB51_BICKagvoXoSU/edite
BIT203-Study WorkShop * D e
a Share
File Edit View insert Format Tools Add-ons Help Lastedit was made minutes ngoby Seri ECT
150 -
Nomal tet
Times Ne
12 • BIU
Discuss the tools and technologies for collaboration and social business that are available and
how they provide value to an organization.
I
arrow_forward
You are watching a live concert. You can also find the concert streaming live on Spotify. About how far must you stand from the stage in order for
the live concert and the live stream to be perfectly in sync?
HINT: Assume the radio signal (Spotify) has to travel all the way around the Earth.
circumference of the Earth (average): 40,041,000 m
Speed of sound: 345 m/s
Speed of light: 300,000,000 m/s
arrow_forward
X A file cord is used to - Google G
X Take Test: ENGR1001 Quiz - En H
x Meet - phg-kzjs-vog
LA
x Workshop Quiz Sec 30,50- s20M
x Courses
b.cas.edu.om/webapps/assessment/take/take.jsp?course_assessment_id=_9826_1&course_id=_18688_1&content_id= 197521 1&question_num_5.X3D0&toggle_state-qSho..
Remaining Time: 06 minutes, 35 seconds.
Less than half of the time remair
Q Question Completion Status:
The reading of Vernier Caliper shown in the Figure is
10
20
30
40
60
80
0 1
3
4
6 7
8
10
.02 mm
29.54 mm
Ob 27.5 mm
0.295 mm
Oc.
Windows b 28.25 mm
ull lis St
ENG 4
70
- LO
50
arrow_forward
و واجب تصصيم.pdf
x 21- ale - la ay de aLis
yae li leslal aie paai B
x Machine Design - Eng. Peter - Se
C:/Users/MI/Desktop/i20%als-pdf
LCD Custom Charac. O
Share | Home Page O
B الفصول الدراسية تحويل JPG إلى .- PDF
YouTube
Gmail M
100%
3 / 1
واجب تصميم.pdf
4.5 The principal stresses induced at a point in
a machine component made of steel 50C4
(Sy = 460 N/mm2) are as follows:
Oj = 200N/mm²
Calculate the factor of safety by (i) the
maximum shear stress theory, and (ii) the
distortion energy theory.
0, = 150 N/mm? 03 =0
%3D
[(1) 2.3 (ii) 2.55]
09:35
AR
arrow_forward
Don't use chatgpt will upvote
arrow_forward
Part III Capstone Problem
Interactive Physics - [Lab7Part3.IP]
Eile Edit World View Object Define Measure Script Window Help
Run StoplI Reset
圖|& 品凸?
Time
Length of Spring 22
6.00
dx
Center of Mass of Rectangle 2
5.000
Tension of Rope 3
Jain@
IFI
... N
ot
rot
***lad
Split
4.000
Velocity of Center of Mass of Rectangle 2
Vx Vx
V Vy
MM
Ve
- m/s
m/s
3.00
*** m/s
Vo
..* lad/s
2 00
Center of Mass of Rectangle 1
1.000
tol
rot
*.* rad
EVelocity of Center of Mass of Rectangle 1
Vx Vx
VVy
M
0.000
-m/s
w 30
m/s
w..
MI
Ve
母100
*** m/s
Vo
... rad/s
+
EAcceleration of Center of Mass of Rectangle 1
Ax Ax
A Ay
AUJAI
Ae
--- m/s^2
... m/s^2
-- m/s^2
.-- rad/s^2
3.00
Aø
Mass M1 = 2.25 kg is at the end of a rope that is 2.00 m in length. The initial angle with
respect to the vertical is 60.0° and M1 is initially at rest. Mass M1 is released and strikes M2
= 4.50 kg exactly horizontally. The collision is elastic. After collision, mass M2 is moving on
a frictionless surface, but runs into a rough patch 2.00…
arrow_forward
image 116
arrow_forward
Conventional float actuated device is used as a level measurement sensor, which consist of pulley and a pointer which move over a graduated scale. Let say you are asked to develop a controller-based float actuated system having a sensor for level measurement or may be more than one sensor is required for accurate level measurement, which sensor will you use and why? Draw the whole schematic showing the position of the sensor or sensors. Will pulley be part of your new digital system, if yes justify your answer, if no, then how you will develop a system without using pulley.
arrow_forward
Please do one through 3
arrow_forward
During ansys simulation,
Designers always get deferent result
when they change mesh size.
True
False
arrow_forward
answer with explanations
arrow_forward
AutoSave On
X
Aptos (Body)
U
quiz 2. Saved
hayley forster
Home Insert Draw Design Layout References Mailings Review View Zotero Help
11
BI Uab x₂
ab x x A
A
Editing
B
饅
te
Paragraph Styles Editing
Dictate
Sensitivity
Editor
Add-ins
A ▾
V
D
A
Aav A A
oard
ly
Font
ly
Styles
Voice
Sensitivity
Editor
Add-ins
12 13 14 15 16 17 18 19 10 11 12 13 14
LE
15 -
b
b
h
h
Determine the moment of inertia of the composite area about the x axis. Set a = 400 mmmm, b =
160 mmmm, h = 110 mmmm, r = 90 mmmm.
Express your answer with the appropriate units
of 1
62 words
▷ English (Australia) Text Predictions: On
FOCUS
Le
+ 117%
ПPLCT
HPLC?
arrow_forward
Hartley Electronics, Inc., in Nashville, producesshort runs of custom airwave scanners for the defense industry.The owner, Janet Hartley, has asked you to reduce inventory byintroducing a kanban system. After several hours of analysis, youdevelop the following data for scanner connectors used in onework cell. How many kanbans do you need for this connector?Daily demand 1,000 connectorsLead time 2 daysSafety stock 12 dayKanban size 500 connectors
arrow_forward
57% O O
P 1:21
ZAIN IQ I.
Lecture (5).pptx - Microsoft PowerPoint
Animations
Slide Show
Review
View
IIA Text Direction-
|Align Text
Convert to Smartart {}* Arrange Quick
Shape Fill-
Shape Outline Repla
A Find
32, AA =,=,| 一前
u s
AL1ゆ4G-
US abe AV
Aa- A- EE
Styles
Shape Effects
A Select
Font
Paragraph
Drawing
Editin
Engineering Mechanics
Statics (Equilibrium of a Particle)
Ayad A. M.
Q/ Use the conditions of equilibrium to solve the following problem
and find the magnitude of the resultant force acting on the screw eye
and its direction measured clockwise from the x axis.
F- 250 N
F- 150 N
30
105
9 68% O
底 )
arrow_forward
Chrome
File
Edit
View
History
Bookmarks
People
Tab
Window
Help
McGraw-Hill Campus - ALEKS Science - CHM1045 GEN CHEM 1 BLENDED 669113
A bconline.broward.edu/d21/le/content/466883/fullscreen/12868783/View
McGraw-Hill Campus - ALEKS Science
O GASES
Interconverting pressure and force
A chemistry graduate student is designing a pressure vessel for an experiment. The vessel will contain gases at pressures up to 470.0 MPa. The student's
design calls for an observation port on the side of the vessel (see diagram below). The bolts that hold the cover of this port onto the vessel can safely withstand
a force of 2.80 MN.
pressure vessel
bolts
side
View
port
Calculate the maximum safe diameter w of the port. Round your answer to the nearest 0.1 cm.
O cm
Explanation
Check
O2021 McGraw-Hill Education. All Rights Reserved. Terms of Use
FEB
arrow_forward
۱:۳ ۳
من
Asiacell l.
joined the meeting sula äibt Jgsso
Lecture (5).pptx - Microsoft PowerPoint
Animations
Slide Show
Review
View
IIA Text Direction -
Shape Fill-
A Fi
32, AA =,三,|评一前。
AZLOG
Align Text-
Convert to SmartArt { }*
Shape Outline
Re
Arrange Quick
Styles
Drawing
Aa
A
Shape Effects-
A Se
Font
Paragraph
Ed
Engineering Mechanics
Statics (Equilibrium of a Particle)
Ayad A. M.
Q/ Use the conditions of equilibrium to solve the following problem
and find the magnitude of the resultant force acting on the screw eye
and its direction measured clockwise from the x axis.
F- 250 N
F- 150 N
30
105
68%
یوسف عامر
1:26p iclul 21.02.22
arrow_forward
MECT361
Mechatronics Components and Instrumentation
PLEASE GIVE ME THE short answer and wite it by keyword
thanks
arrow_forward
kamihq.com/web/viewer.html?state%=D%7B"ids"%3A%5B"1vSrSXbH_6clkKyVVKKAtzZb_GOMRwrCG"%5D%...
lasses
Gmail
Copy of mom it for..
Маps
OGOld Telephone Ima.
Preview attachmen...
Kami Uploads ►
Sylvanus Gator - Mechanical Advantage Practice Sheet.pdf
rec
Times New Roman
14px
1.5pt
BIUSA
A Xa x* 三三
To find the Mechanical Advantage of ANY simple machine when given the force, use MA = R/E.
1.
An Effort force of 30N is appliled to a screwdriver to pry the lid off of a can of paint. The
screwdriver applies 90N of force to the lid. What is the MA of the screwdriver?
MA =
arrow_forward
о (14) Аянами Рей-YouTube
O (14) [FREE FOR PROFIT] Playboi C x
b Success Confirmation of Questio X
О Мессенджер
O MENG250-S2022-HW_03.pdf
O File | C:/Users/Lenovo/Downloads/MENG250-S2022-HW_03.pdf
ES O
MENG250-S2022-HW_03.pdf
2 / 3
150%
Problem 1
Calculate the reactions of the truss shown and use:
(a) the Method of Joints to determine the axial force in each member.
(b) The Method of Sections to verify the values of the axial forces in members EF, BC
and BF.
Indicate also whether each axial force is tensile (T) or compressive (C).
E
F
120KN
3.0 m
A
D
В
100KN
100KN
4.0 m
4.0 m
4.0 m
Activate Windows
Go to Settings to activate Windows.
1:18 PM
A ENG
4/14/2022
21
>
...
...
+
II
arrow_forward
о (14) Аянами Рей-YouTube
O (14) [FREE FOR PROFIT] Playboi C x
b Success Confirmation of Questio X
О Мессенджер
O MENG250-S2022-HW_03.pdf
O File | C:/Users/Lenovo/Downloads/MENG250-S2022-HW_03.pdf
ES O
MENG250-S2022-HW_03.pdf
2 / 3
150%
+ |
Problem 2
Determine the coordinates of the centroid of the following composite areas.
100 mm
140 mm
2 in
8 in
3 in
50 mm
140 mm
-4 in
-6 in
200 mm
10 in
(a)
(b)
Activate Windows
Go to Settings to activate Windows.
PbMZzXCghHY.jpg
Show all
1:19 PM
A ENG
4/14/2022
21
>
...
...
+
II
arrow_forward
О (14) Аянами Рей- YouTube
O (14) [FREE FOR PROFIT] Playboi C x
b Success Confirmation of Questio X
О Мессенджер
O MENG250-S2022-HW_03.pdf
O File | C:/Users/Lenovo/Downloads/MENG250-S2022-HW_03.pdf
ES O
MENG250-S2022-HW_03.pdf
3 / 3
125%
+ |
Problem 3
Considering that the following structures are in equilibrium, draw their free-body
diagram and determine the reactions at the supports.
40KN/m
А
В
1.5 m
5.0 m
a)
w = 3(1-x²/25) [KN/m]
В
A
5 m
b)
Activate Windows
Go to Settings to activate Windows.
1:20 PM
A ENG
4/14/2022
21
>
...
...
II
arrow_forward
DOM
1500 N
A327197870 X
SA LPU Live
C MCQ (Mult x Creative Steal X
Battlefield haX
97870 133937_2022_G2CA3MEC107.pdf
6 News
[OFFICIAL]Filmora.
Hillm
Clipmaker - Online...
INTROANIME- Pan... Adventure Archives...
Free Download PC..
|E Readin-
%001
Q 回
ictioniesS.
OSIV
tension in the cables.
DULL
N 09
Q3: As shown in figure, find
that at what distance body A
will attain a velocity of 3 m/s
4.
B.
when
starts
from
rest.
N 000
3.
Assume
coefficient
frietion as 0.2 for block and
of
surface and pulleys
are
frictionless and weightless.
2.
20:07
14-01-2022
NI
arrow_forward
AutoSave
STATICS - Protected View• Saved to this PC -
O Search (Alt+Q)
Off
ERIKA JOY DAILEG
EJ
File
Home
Insert
Draw
Design
Layout
References
Mailings
Review
View
Help
Acrobat
O Comments
E Share
PROTECTED VIEW Be careful-files from the Internet can contain viruses. Unless you need to edit, it's safer to stay in Protected View.
Enable Editing
Situation 9 - A 6-m long ladder weighing 600 N is shown in the Figure. It is required to determine
the horizontal for P that must be exerted at point C to prevent the ladder from sliding. The
coefficient of friction between the ladder and the surface at A and B is 0.20.
25. Determine the reaction at A.
26. Determine the reaction at B.
27. Determine the required force P.
4.5 m
1.5 m
H=0.2
30°
Page 5 of 5
671 words
D. Focus
100%
C
ЕPIC
GAMES
ENG
7:24 pm
w
US
16/02/2022
IZ
arrow_forward
Please help with b
arrow_forward
please in detaild
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
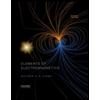
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
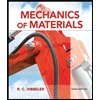
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
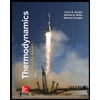
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
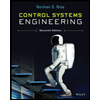
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
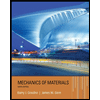
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
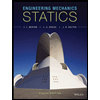
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- solve 2nd questionarrow_forward:4G 10:48 Shashi Just now Engineering MechanCTE A1 - Lopy - Copy Canspat ibylity Mode- Word (Product Activation Iasiled) INSTRT DESIGN FAGE LAVOUT RETERENCES MAILINGS REVIEW VIEW Times New Ri-|18 KA Aa E-E- E. EE TAaBbCc[ AaBbCc[ AaBbC AaBbCc AaB AuBbCcD AaBbCcC AaBbCcl BIU X, x* A- - A 1 Normai TNo Spac Houding Heading 2 Titte Subtine Subtle Em Enptiat le Paah Set Fas = 0.776 KN (C). Question 2: Determine the force in members BC, CF, and FE. State if the members are in tension or compression. 4 ft B 4 ft 4 ft -4 ft- 600 lb 600 lb 800 lb 3E ENGLISH (UNTED STATES) Nidhi is presentingarrow_forwardInvestigate, and select appropriate sensors for the specified system.arrow_forward
- PLEASE ANSWER NUMBER 14.MECH 221-KINEMATICS: PLEASE GIVE DETAILED ANSWER AND CORRECT ANSWERS. I WILL REPORT TO BARTLEBY THOSE TUTORS WHO WILL GIVE INCORRECT ANSWERS.arrow_forwardI need help solving this problem.arrow_forward9:19 M Yo, 4G+ ul l LTE1 1c576dc540f8a78ffa178324d524@thread.tacv2 ch have started. For now, meeting transcripts are only available to select Teams users. Privacy policy Di Unit 13 - Microsoft PowerPoint ANIMATIONS Sign in TRANSITIONS SLIDE SHOW REVIEW VIEW Nitro Pro 9 E tE. IlN Text Direction CO) Align Text Convert to SmartArt O\\D00- A Find Z Shape Outline Replace A Select 32 A A E-E a Shape Fill- BIUS alx AV- Aa 4 n Arrange Quick Styles - O Shape Effects Font Paragraph Drawing Editing ΤΥΡE Β Example: 1 A rigid bar AB, 9 m long, is supported by two vertical rods at its end and in a horizontal position under a load P as shown in figure. Find the position of the load P so that the bar AB remains horizontal. 5 m A = 445 mm 2 A = 1000 mm 2 9 m E = 2 x 10 5 E = 1 x 10 5 3m A B 01:01:20 to add notes NOTES COMMENTS 9 :: 7 -- 70% X Activate Windows 20 Settings to activate 202002090096 AKHIL... 202002090069 pravi. 202101040208 Manis.. No 1= 1/ 86arrow_forward
- Subject: Mechanical Measurements Do not copy other online answersarrow_forwardREC M Gmail x4 BIT203 - Google x Gmail: Free. Pria x Dashboard BIT203-Study W x docs.google.com/document/d/1unVPvr_GhUFhrQuNdGICLqFle1ZB51_BICKagvoXoSU/edite BIT203-Study WorkShop * D e a Share File Edit View insert Format Tools Add-ons Help Lastedit was made minutes ngoby Seri ECT 150 - Nomal tet Times Ne 12 • BIU Discuss the tools and technologies for collaboration and social business that are available and how they provide value to an organization. Iarrow_forwardYou are watching a live concert. You can also find the concert streaming live on Spotify. About how far must you stand from the stage in order for the live concert and the live stream to be perfectly in sync? HINT: Assume the radio signal (Spotify) has to travel all the way around the Earth. circumference of the Earth (average): 40,041,000 m Speed of sound: 345 m/s Speed of light: 300,000,000 m/sarrow_forward
- X A file cord is used to - Google G X Take Test: ENGR1001 Quiz - En H x Meet - phg-kzjs-vog LA x Workshop Quiz Sec 30,50- s20M x Courses b.cas.edu.om/webapps/assessment/take/take.jsp?course_assessment_id=_9826_1&course_id=_18688_1&content_id= 197521 1&question_num_5.X3D0&toggle_state-qSho.. Remaining Time: 06 minutes, 35 seconds. Less than half of the time remair Q Question Completion Status: The reading of Vernier Caliper shown in the Figure is 10 20 30 40 60 80 0 1 3 4 6 7 8 10 .02 mm 29.54 mm Ob 27.5 mm 0.295 mm Oc. Windows b 28.25 mm ull lis St ENG 4 70 - LO 50arrow_forwardو واجب تصصيم.pdf x 21- ale - la ay de aLis yae li leslal aie paai B x Machine Design - Eng. Peter - Se C:/Users/MI/Desktop/i20%als-pdf LCD Custom Charac. O Share | Home Page O B الفصول الدراسية تحويل JPG إلى .- PDF YouTube Gmail M 100% 3 / 1 واجب تصميم.pdf 4.5 The principal stresses induced at a point in a machine component made of steel 50C4 (Sy = 460 N/mm2) are as follows: Oj = 200N/mm² Calculate the factor of safety by (i) the maximum shear stress theory, and (ii) the distortion energy theory. 0, = 150 N/mm? 03 =0 %3D [(1) 2.3 (ii) 2.55] 09:35 ARarrow_forwardDon't use chatgpt will upvotearrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
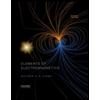
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
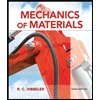
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
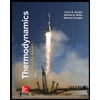
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
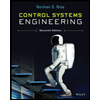
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
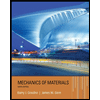
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
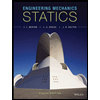
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY