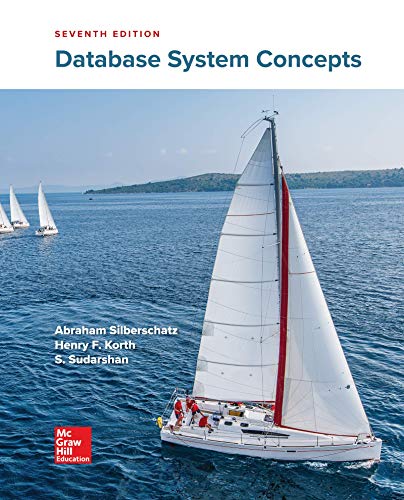
def getDimension():
row = int(input("Enter number of rows (between 4 and 10): "))
while row < 4 or row > 10:
row = int(input("Invalid input! Enter number of rows (between 4 and 10): "))
column = int(input("Enter number of columns (between 4 and 10): "))
while column < 4 or column > 10:
column = int(input("Invalid input! Enter number of columns (between 4 and 10): "))
return row, column
def printBoard(board):
for row in board:
print("|", end="")
for cell in row:
if cell == "":
print(" ", end="")
else:
print(cell, end="")
print("|", end="")
print()
print("-" * (len(board[0]) * 2 + 1))
def dropToken(board, col, token):
row = len(board) - 1
while row >= 0:
if board[row][col] == "":
board[row][col] = token
return row
row -= 1
return -1
def isValidLocation(board, col):
return board[0][col] == ""
def isTie(board):
for row in board:
for cell in row:
if cell == "":
return False
return True
def checkWinner(board, token):
# Check horizontal
for row in board:
for col in range(len(row) - 3):
if row[col] == token and row[col+1] == token and row[col+2] == token and row[col+3] == token:
return True
# Check vertical
for col in range(len(board[0])):
for row in range(len(board) - 3):
if board[row][col] == token and board[row+1][col] == token and board[row+2][col] == token and board[row+3][col] == token:
return True
# Check diagonal positive
for col in range(len(board[0]) - 3):
for row in range(len(board) - 3):
if board[row][col] == token and board[row+1][col+1] == token and board[row+2][col+2] == token and board[row+3][col+3] == token:
return True
# Check diagonal negative
for col in range(len(board[0]) - 3):
for row in range(3, len(board)):
if board[row][col] == token and board[row-1][col+1] == token and board[row-2][col+2] == token and board[row-3][col+3] == token:
return True
return False
# Main game loop
row, column = getDimension()
board = [["" for j in range(column)] for i in range(row)]
game_over = False
current_player = "X"
while not game_over:
printBoard(board)
if current_player == "X":
col = int(input("Player X, enter column to drop your token (0-{}): ".format(column-1)))
while not isValidLocation(board, col):
col = int(input("Column is full or invalid! Player X, enter column to drop your token (0-{}): ".format(column-1)))
row = dropToken(board, col, current_player)
if row == -1:
print("Error: invalid row")
continue
if checkWinner(board, current_player):
printBoard(board)
print("Congratulations! Player {} wins!".format(current_player))
game_over = True
elif isTie(board):
printBoard(board)
print("The game is a tie.")
game_over = True
current_player = "O" # Switch player
else:
col = int(input("Player O, enter column to drop your token (0-{}): ".format(column-1)))
while not isValidLocation(board, col):
col = int(input("Column is full or invalid! Player O, enter column to drop your token (0-{}): ".format(column-1)))
row = dropToken(board, col, current_player)
if row == -1:
print("Error: invalid row")
continue
if checkWinner(board, current_player):
printBoard(board)
print("Congratulations! Player {} wins!".format(current_player))
game_over = True
elif isTie(board):
printBoard(board)
print("The game is a tie.")
game_over = True
current_player = "X" # Switch player
print("Thanks for playing!")
===================================================================================
Question:
can you change the else statement into the picture attached


Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- #include #include #include int main() { int i, j; printf("Columns | JIn"); for (i = 1; i< 4; ++i) { printf("Outer %6d\n", i); for (j = 0; j< i; ++j) { printf(" Inner%10d\n", j); } } /* heading of outer for loop */ /* heading of inner loop */ %3D return (0); } Create a new code modifying it to use "while loop" instead of the "for loop"arrow_forwardComputer Science JAVA #7 - program that reads the file named randomPeople.txt sort all the names alphabetically by last name write all the unique names to a file named namesList.txt , there should be no repeatsarrow_forwardAssume an array is defined as int[] nums = {7, 15, 23, 5};. Which of the following would place the values in the array in descending numeric order? a. Array.Reverse(nums); b. Array.Reverse(nums); Array.Sort(nums); c. Array.Sort(nums); d. Array.Sort(nums); Array.Reverse(nums);arrow_forward
- def rectangle_overlap(rect1_bl_x,rect1_bl_y, rect1_tr_x,rect1_tr_y, rect2_bl_x,rect2_bl_y, rect2_tr_x,rect2_tr_y): """ (int,int,int,int,int,int,int,int) -> str Function determines whether two rectangles overlap. When rectangles overlap, the function checks for the following scenarios 1. The two rectangles share the same coordinates 2. The first rectangle is contained within the second 3. The second rectangle is contained within the first 4. The rectangles have overlapping area, but neither is completely contained within the other Function inputs represent x and y coordinates of bottom left and top right corners of rectangles (see lab document) The function return one of the following strings corresponding to the scenario "no overlap" "identical coordinates" "rectangle 1 is contained within rectangle 2" "rectangle…arrow_forwardProblem Description - JAVA PROGRAMMING Use a Two-dimensional (3x3) array to solve the following problem: Write an application that inputs nine numbers, each of which is between 1 and 10, inclusive. Display the array after the user inputs each value. Rotate/flip the array by changing places. Make the rows columns and vice versa. You have to move the elements to their new locations. Remember to validate the input and display an error message if the user inputs invalid data. Documentation and the screenshot(s) of the results. Example: 1 2 3 4 5 6 7 8 9 the result will be : 1 4 7 2 5 8 3 6 9arrow_forwardclass Main { // this function will return the number elements in the given range public static int getCountInRange(int[] array, int lower, int upper) { int count = 0; // to count the numbers // this loop will count the numbers in the range for (int i = 0; i < array.length; i++) { // if element is in the range if (array[i] >= lower && array[i] <= upper) count++; } return count; } public static void main(String[] args) { // array int array[] = {1,2,3,4,5,6,7,8,9,0}; // ower and upper range int lower = 1, upper = 9; // throwing an exception…arrow_forward
- } function problem13() { var outputObj = document.getElementById("output"); var inputNumber parseInt(document.getElementById("numberInput").value); outputObj.innerHTML = "number: "number: " + inputNumber; // Add line breaks for space outputObj.innerHTML += ""; // Generate the sequence based on the input number for (var i = 1; i "; // Program ended message outputObj.innerHTML += "Program ended";arrow_forwardvoid changeAll(int x[]) { x[0] = x[0] + 5; return; The first element in an array would be increased by 5 The array would be the same because it was passed by value x would be increased by 5 All the elements in the passed array would be incremented by 5arrow_forwarddef ppv(tp, fp): # TODO 1 return def TEST_ppv(): ppv_score = ppv(tp=100, fp=3) todo_check([ (np.isclose(ppv_score,0.9708, rtol=.01),"ppv_score is incorrect") ]) TEST_ppv() garbage_collect(['TEST_ppv'])arrow_forward
- Recipe Program - Java ONLYI am looking to create a program that is a recipe holder. It needs to include at a minimum: At least 1 loop An Array or ArrayList At least 3 Java classes Use of methods The program would allow the user to (as part of a menu selection): Add a recipe Include ingredient list Instructions List all recipes that are in the program (array) Display a single recipe Search Recipes (option to view recipe selected) For example, searching for peanut butter cookie would bring up the recipe or multiple if the same name. The user could then select one of them to view the recipe. Search Recipes with a single ingredient (option to view recipe selected) For example: search for coconut and it would bring up coconut cream pie and German chocolate cake. The user could then select one of them to view the recipe. Close application Also need the program in a UML diagram.arrow_forwardstrobogrammatic number is a number that looks the same when rotated 180 degrees (looked at upside down). Find all strobogrammatic numbers that are of length = n. For example, Given n = 2, return ["11","69","88","96"]. def gen_strobogrammatic(n): Given n, generate all strobogrammatic numbers of length n. :type n: int :rtype: List[str] return helper(n, n) def helper(n, length): if n == 0: return [™"] if n == 1: return ["1", "0", "8"] middles = helper(n-2, length) result = [] for middle in middles: if n != length: result.append("0" + middle + "0") result.append("8" + middle + "8") result.append("1" + middle + "1") result.append("9" + middle + "6") result.append("6" + middle + "9") return result def strobogrammatic_in_range(low, high): :type low: str :type high: str :rtype: int res = count = 0 low_len = len(low) high_len = len(high) for i in range(low_len, high_len + 1): res.extend(helper2(i, i)) for perm in res: if len(perm) == low_len and int(perm) int(high): continue count += 1 return…arrow_forwardPointers: P3: Given the following string, after you call func2, if you printed out the array, what would be printed out? string a = {"c","a","n", func2(a); "y"}; void func2(string *arr) { arr[3) - "t"; arr[2]="vi"; %3Darrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
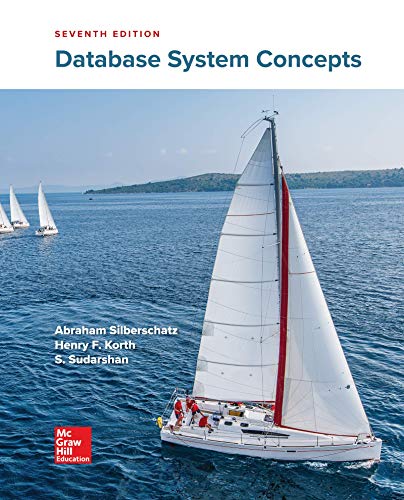
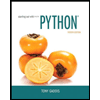
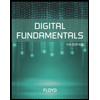
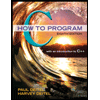
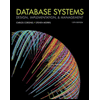
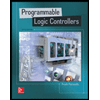