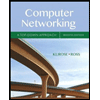
1. Copy the previous program to a new file.
#include <iostream>
using namespace std;
class Vehicle
{
private:
string brand;
public:
Vehicle()
{
this->brand = "TBD";
}
Vehicle(string brand)
{
this->brand = brand;
}
void output()
{
cout << "Brand: " << this->brand << endl;
}
string getBrand() const { return brand; }
void setBrand(const string &brand_) { brand = brand_; }
};
int main()
{
Vehicle a, b("BMW");
a.output();
b.output();
a.setBrand("Tesla");
cout << a.getBrand() << endl;
}
that's my previous code
2. Create a class named Car derived from Vehicle.
a. Private data member: weight — dynamic member int
b. Implement default and two argument constructors
c. Redefine output function
d. Mutator and accessor function for weight
3. Create a class named Boat derived from Vehicle.
a. Private data member: hullLength — dynamic member int
b. Implement default and two argument constructors
c. Redefine output function
d. Mutator and accessor function for hullLength
Use following main() to test your function.
int main(){
Car a,b("Honda",2000);
a.output(); // Brand:TBD Weight: 0
b.output(); // Brand:Honda Weight: 2000
b.setBrand("Tesla");
b.setWeight(3000);
b.output(); // Brand:Tesla Weight: 3000
Boat c,d("Baja",100);
c.output(); // Brand:TBD hullLength: 0
d.output(); // Brand:Baja hullLength: 100
d.setBrand("Bertram");
d.sethullLength(60);
d.output(); // Brand:Bertram hullLength: 60
}
Output from main:
Brand:TBD Weight: 0
Brand:Honda Weight: 2000
Brand:Tesla Weight: 3000
Brand:TBD hullLength: 0
Brand:Baja hullLength: 100
Brand:Bertram hullLength: 60

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- #include <iostream> #include <string> using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to…arrow_forwardC++arrow_forward#ifndef INT_SET_H#define INT_SET_H #include <iostream> class IntSet{public: static const int DEFAULT_CAPACITY = 1; IntSet(int initial_capacity = DEFAULT_CAPACITY); IntSet(const IntSet& src); ~IntSet(); IntSet& operator=(const IntSet& rhs); int size() const; bool isEmpty() const; bool contains(int anInt) const; bool isSubsetOf(const IntSet& otherIntSet) const; void DumpData(std::ostream& out) const; IntSet unionWith(const IntSet& otherIntSet) const; IntSet intersect(const IntSet& otherIntSet) const; IntSet subtract(const IntSet& otherIntSet) const; void reset(); bool add(int anInt); bool remove(int anInt); private: int* data; int capacity; int used; void resize(int new_capacity);}; bool operator==(const IntSet& is1, const IntSet& is2); #endifarrow_forward
- 10. When using a std::vector, what values do we expect to get from the size and empty member functions after calling the clear member function? Complete the table below with the expected values. member function value after donations.clear() donations.size() donations.empty()arrow_forward#include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode* leftPtr; int data; struct treeNode* rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode* TreeNodePtr; void insertNode(TreeNodePtr* treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr* treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if…arrow_forward#include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode *leftPtr; int data; struct treeNode *rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode *TreeNodePtr; void insertNode(TreeNodePtr *treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr *treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if (*treePtr != NULL) { (*treePtr)->data = value;…arrow_forward
- #include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forward#include <iostream> #include <iomanip> #include <string> #include <vector> using namespace std; class Movie { private: string title = ""; int year = 0; public: void set_title(string title_param); string get_title() const; // "const" safeguards class variable changes within function string get_title_upper() const; void set_year(int year_param); int get_year() const; }; // NOTICE: Class declaration ends with semicolon! void Movie::set_title(string title_param) { title = title_param; } string Movie::get_title() const { return title; } string Movie::get_title_upper() const { string title_upper; for (char c : title) { title_upper.push_back(toupper(c)); } return title_upper; } void Movie::set_year(int year_param) { year = year_param; } int Movie::get_year() const { return year; } int main() { cout << "The Movie List program\n\n"…arrow_forwardstruct employee{int ID;char name[30];int age;float salary;}; (A) Using the given structure, Help me with a C program that asks for ten employees’ name, ID, age and salary from the user. Then, it writes the data in a file named out.txt (B) For the same structure, read the contents of the file out.txt and print the name of the highest salaried employee and youngest employee names name in the outputscreen.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
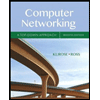
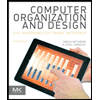
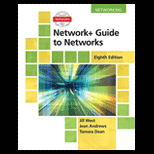
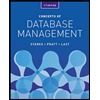
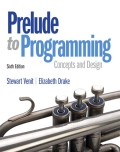
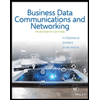