#include #include using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to calculate bonus based on rating // This is the work done in the endOfJob() function // Output cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl; cout << "Employee Salary: $" << employeeSalary << endl; cout << "Employee Rating: " << employeeRating << endl; cout << "Employee Bonus: $" << employeeBonus << endl; return 0; }
#include #include using namespace std; int main() { // Declare and initialize variables. string employeeFirstName; string employeeLastName; double employeeSalary; int employeeRating; double employeeBonus; const double BONUS_1 = .25; const double BONUS_2 = .15; const double BONUS_3 = .10; const double NO_BONUS = 0.00; const int RATING_1 = 1; const int RATING_2 = 2; const int RATING_3 = 3; // This is the work done in the housekeeping() function // Get user input cout << "Enter employee's first name: "; cin >> employeeFirstName; cout << "Enter employee's last name: "; cin >> employeeLastName; cout << "Enter employee's yearly salary: "; cin >> employeeSalary; cout << "Enter employee's performance rating: "; cin >> employeeRating; // This is the work done in the detailLoop() function // Use switch statement to calculate bonus based on rating // This is the work done in the endOfJob() function // Output cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl; cout << "Employee Salary: $" << employeeSalary << endl; cout << "Employee Rating: " << employeeRating << endl; cout << "Employee Bonus: $" << employeeBonus << endl; return 0; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
#include <iostream>
#include <string>
using namespace std;
int main()
{
// Declare and initialize variables.
string employeeFirstName;
string employeeLastName;
double employeeSalary;
int employeeRating;
double employeeBonus;
const double BONUS_1 = .25;
const double BONUS_2 = .15;
const double BONUS_3 = .10;
const double NO_BONUS = 0.00;
const int RATING_1 = 1;
const int RATING_2 = 2;
const int RATING_3 = 3;
// This is the work done in the housekeeping() function
// Get user input
cout << "Enter employee's first name: ";
cin >> employeeFirstName;
cout << "Enter employee's last name: ";
cin >> employeeLastName;
cout << "Enter employee's yearly salary: ";
cin >> employeeSalary;
cout << "Enter employee's performance rating: ";
cin >> employeeRating;
// This is the work done in the detailLoop() function
// Use switch statement to calculate bonus based on rating
// This is the work done in the endOfJob() function
// Output
cout << "Employee Name: " << employeeFirstName << " " << employeeLastName << endl;
cout << "Employee Salary: $" << employeeSalary << endl;
cout << "Employee Rating: " << employeeRating << endl;
cout << "Employee Bonus: $" << employeeBonus << endl;
return 0;
}
![you, and the input statements and
2]
output statements have been
3 #include <iost
written. Read them over carefully
4 #include <stri
</>
5 using namespac
before you proceed to the next
6 int main()
step.
//Declare
2. Design the logic, and write the rest
6.
string emple
of the program using a switch
10
string emplo
statement.
11
double emplo
12
int employee
3. Execute the program by clicking
double emplo
13
the Run button at the bottom of
14
const double
15
const double
the screen entering the following
16
const double
as input:
17
const double
18)
const int RAT
19
const int RAT
ee's name: Jeanne Hanson
20
const int RAT
ee's salary: 70000.00
21
ee's perfornance rating 2
22.
// This is th
23
//Get user i
24.
cout << "Enter
251
cin >> employe
4. Confirm that your output matches
26
cout << "Enter
27
cin >> employe
the following:
28
cout <<"Enter
29
cin employer
ployee Name: Jeanne Hanson
30
cout <<"Enter
ployee Salary: S70000
ployee Rating: 2
31
cin >> employee
32
ployee Bonus: 510500
33
/W This is the
34
/Use switch s
LIMITED TIME
APPLY NOL
Overwatc
Earn exclusive PachiMarchi rewards in
Overwatch!
LATEST NEWS](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F4bfbb203-4399-4804-a6b8-bbfebafe4401%2Fc4184380-f956-40bf-95d5-f13144c5b7fb%2Fczws7i7_processed.jpeg&w=3840&q=75)
Transcribed Image Text:you, and the input statements and
2]
output statements have been
3 #include <iost
written. Read them over carefully
4 #include <stri
</>
5 using namespac
before you proceed to the next
6 int main()
step.
//Declare
2. Design the logic, and write the rest
6.
string emple
of the program using a switch
10
string emplo
statement.
11
double emplo
12
int employee
3. Execute the program by clicking
double emplo
13
the Run button at the bottom of
14
const double
15
const double
the screen entering the following
16
const double
as input:
17
const double
18)
const int RAT
19
const int RAT
ee's name: Jeanne Hanson
20
const int RAT
ee's salary: 70000.00
21
ee's perfornance rating 2
22.
// This is th
23
//Get user i
24.
cout << "Enter
251
cin >> employe
4. Confirm that your output matches
26
cout << "Enter
27
cin >> employe
the following:
28
cout <<"Enter
29
cin employer
ployee Name: Jeanne Hanson
30
cout <<"Enter
ployee Salary: S70000
ployee Rating: 2
31
cin >> employee
32
ployee Bonus: 510500
33
/W This is the
34
/Use switch s
LIMITED TIME
APPLY NOL
Overwatc
Earn exclusive PachiMarchi rewards in
Overwatch!
LATEST NEWS
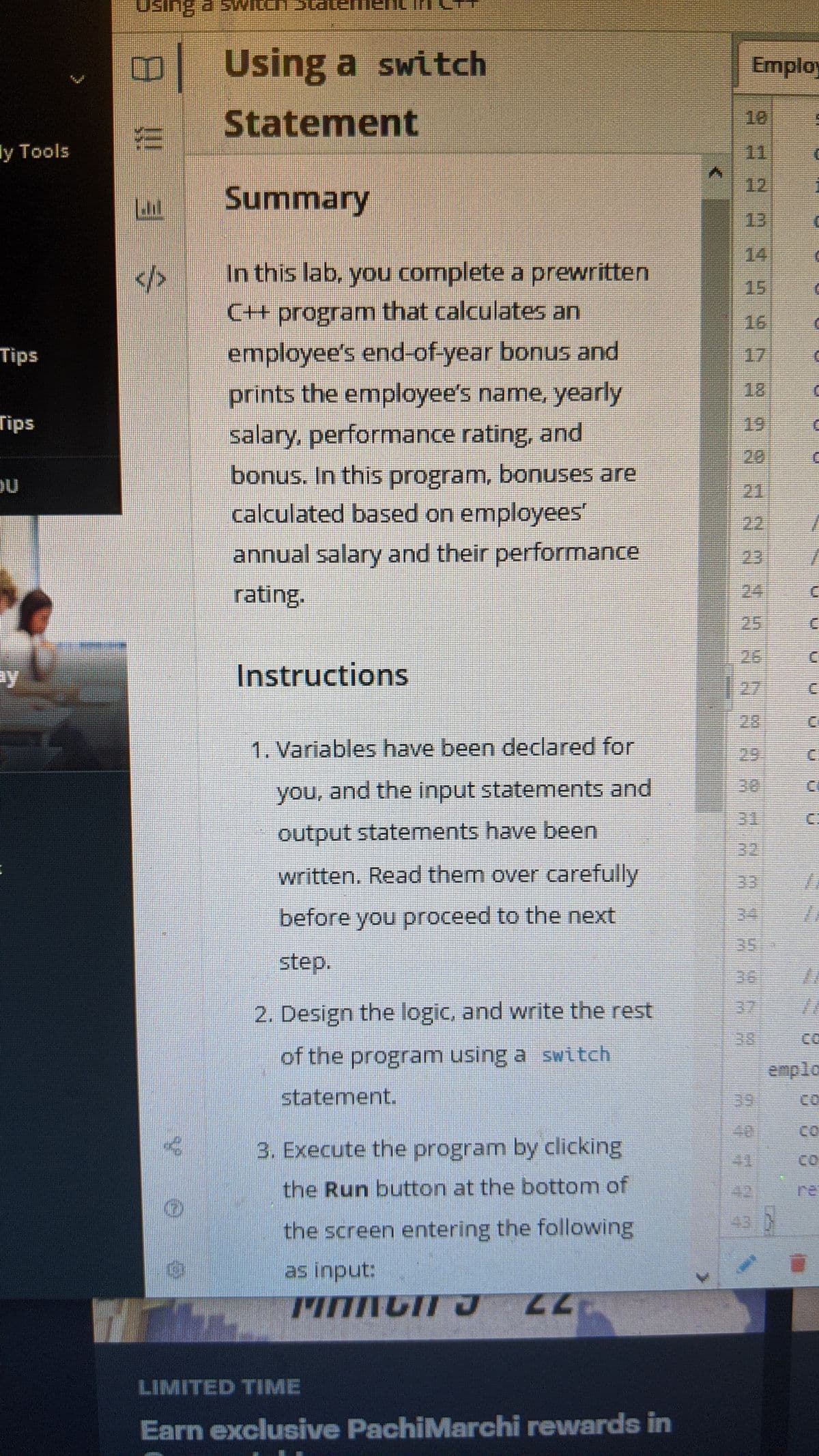
Transcribed Image Text:O Using a switch
Employ
Statement
18
ly Tools
11
12
Summary
13
14
In this lab, you complete a prewritten
</>
15
C+ program that calculates an
16
employee's end-of-year bonus and
prints the employee's name, yearly
Tips
17
18
Tips
19
salary, performance rating, and
20
bonus. In this program, bonuses are
calculated based on employees'
21
22
annual salary and their performance
rating.
23
24
25
26
ay
Instructions
27
28
1. Variables have been declared for
29.
C:
30
you, and the input statements and
31
output statements have been
32
written, Read them over carefully
33
before you proceed to the next
34
35
step.
36
32
2. Design the logic, and write the rest
38
CO
of the program using a switch
emplo
statement.
39
CO
40
CO
3. Execute the program by clicking
41
CO
the Run button at the bottom of
42
re
43
the screen entering the following
as input:
LIMITED TIME
Earn exclusive PachiMarchi rewards in
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
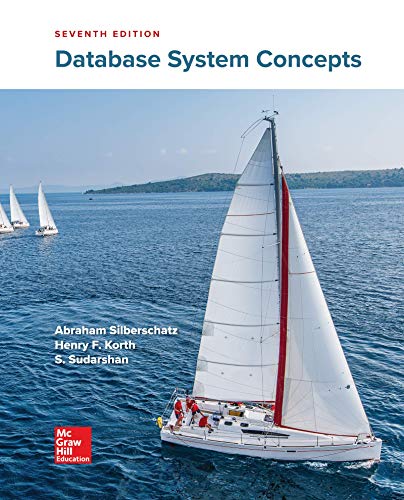
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
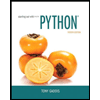
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
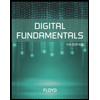
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
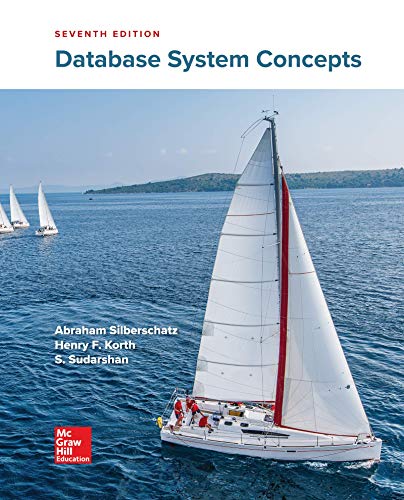
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
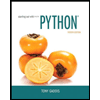
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
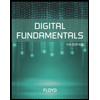
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
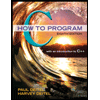
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
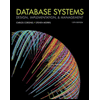
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
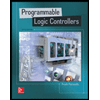
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education