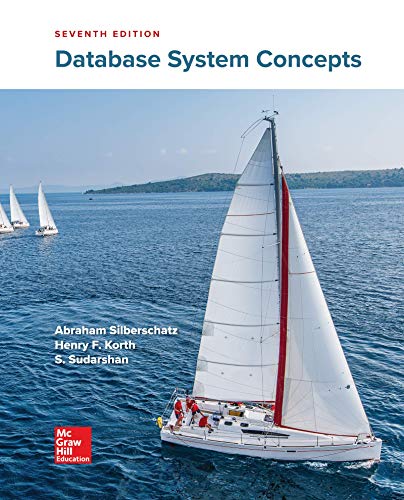
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
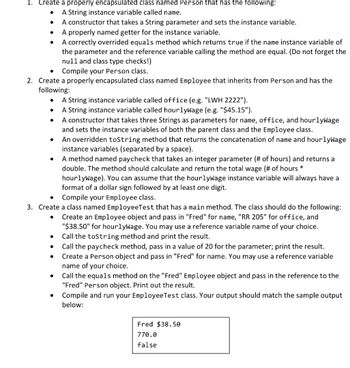
Transcribed Image Text:1. Create a properly encapsulated class named Person that has the following:
A String instance variable called name.
A constructor that takes a String parameter and sets the instance variable.
A properly named getter for the instance variable.
A correctly overrided equals method which returns true if the name instance variable of
the parameter and the reference variable calling the method are equal. (Do not forget the
null and class type checks!)
Compile your Person class.
2. Create a properly encapsulated class named Employee that inherits from Person and has the
following:
●
●
● A String instance variable called office (e.g. "LWH 2222").
A String instance variable called hourlywage (e.g. "$45.15").
A constructor that takes three Strings as parameters for name, office, and hourlyWage
and sets the instance variables of both the parent class and the Employee class.
An overridden toString method that returns the concatenation of name and hourlyWage
instance variables (separated by a space).
A method named paycheck that takes an integer parameter (# of hours) and returns a
double. The method should calculate and return the total wage (# of hours *
hourlyWage). You can assume that the hourlywage instance variable will always have a
format of a dollar sign followed by at least one digit.
Compile your Employee class.
3. Create a class named EmployeeTest that has a main method. The class should do the following:
Create an Employee object and pass in "Fred" for name, "RR 205" for office, and
"$38.50" for hourlyWage. You may use a reference variable name of your choice.
Call the toString method and print the result.
Call the paycheck method, pass in a value of 20 for the parameter; print the result.
Create a Person object and pass in "Fred" for name. You may use a reference variable
name of your choice.
●
●
●
●
Call the equals method on the "Fred" Employee object and pass in the reference to the
"Fred" Person object. Print out the result.
Compile and run your Employee Test class. Your output should match the sample output
below:
Fred $38.50
770.0
false
Expert Solution

arrow_forward
Step 1
Here's the code for each class:
- Person class:
public class Person {
private String name;
public Person(String name) {
this.name = name;
}
public String getName() {
return name;
}
@Override
public boolean equals(Object obj) {
if (this == obj) return true;
if (obj == null || getClass() != obj.getClass()) return false;
Person person = (Person) obj;
return name.equals(person.name);
}
}
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Explain Abstract Model and System-Level Computing Model?arrow_forwardDesign a class called Date that has integer data members to store month , day, and year. The class should have two constructors; a default constructor that accepts no parameters, and a three-parameter constructor that allows the date to be set by the programmer. If the user creates a Date object without passing any arguments, or if any of the values passed are invalid, the default values of 1, 1, 2001 (i.e., January 1, 2001) should be used. The class should have three (3) member functions to print the date in the following formats: 3/15/16 March 15, 2016 15 March 2016 Demonstrate the class by writing a program that uses it. Be sure your program only accepts reasonable values for month and day. The month should be between 1 and 12. The day should be between 1 and the number of days in the selected month. I expect to see all uses of the constructors that you made, including the error checking. I also expect to see all uses of the 3 output functions in your program. You are responsible…arrow_forwardCreate a class called Complex for performing arithmetic with complex numbers. Complex numbers have the form realPart + imaginaryPart * i where i is -1 Write a program to test your class. Use floating-point variables to represent the private data of the class. Provide a constructor that enables an object of this class to be initialized when it is declared. Provide a no-argument constructor with default values in case no initializers are provided. Provide public methods that perform the following operations: a) Add two Complex numbers: The real parts are added together and the imaginary parts are added together. b) Subtract two Complex numbers: The real part of the right operand is subtracted from the real part of the left operand, and the imaginary part of the right operand is sub- tracted from the imaginary part of the left operand. c) Print Complex numbers in the form (a, b), where a is the real part and b is the imaginary part.arrow_forward
- dayType Class We will be working on a project that designs a class calendarType, so that a client program can use this class to print a calendar for any month starting Jan 1. 1900. An example of the calendar for September 2019 is: September 2019 Sun Mon Tue Wed Thu Fri Sat 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 We will develop several classes which will work together to create a calendar. The first of these classes is called dayType which will manipulate a day of the week. The class dayType will store a day, such as Sun for Sunday. The class should be able to perform the following operations on an object of type dayType: a. Set the day. b. Print the day. c. Return the day. d. Return the next day. e.…arrow_forwardWhich of the statements are true about the class Magazine? 01: class Magazine { 02: public: 03: Magazine (int maxSize = 100); 04: void add (Article a); 05: int numArticles() const; 06: Article getArticle (int i); 07: private: 08: Article* array; 09: int numberOfArticles; 10: }; The compiler will provide a destructor for this class. It has a default constructor It is const-correct. It has a copy constructor The programmer should provide an assignment operatorarrow_forward1. For methods parameters are specified in the method definition, arguments are provided during a method call arguments are specified in the method definition, parameters are provided during a method call arguments override parameters parameters encapsulate arguments 2 Local variables are not automatically initialized. True False 3 Composition refers to an 'is-a' relationship. True False 4 Class constructors must be declared with a return type equal to the class. True False 5 Object instances are created using the “new” keyword. True Falsearrow_forward
- Step 1: Extend the ItemToPurchase class per the following specifications: Private fields string itemDescription - Initialized in default constructor to "none" Parameterized constructor to assign item name, item description, item price, and item quantity. Public member methods setDescription() mutator & getDescription() accessor printItemCost() - Outputs the item name followed by the quantity, price, and subtotal printItemDescription() - Outputs the item name and description Ex. of PrintItemCost() output: Bottled Water 10 @ $1 = $10 Ex. of PrintItemDescription() output: Bottled Water: Deer Park, 12 oz. ShoppingCart.java - Class definition ShoppingCartManager.java - Contains main() method Build the ShoppingCart class with the following specifications. Private fields String customerName - Initialized in default constructor to "none" String currentDate - Initialized in default constructor to "January 1, 2016" ArrayList cartItems Default constructor Parameterized…arrow_forward1- Create a hierarchy of Java classes as follows: MyRectangle is_a MyShape; MyOval is a MyShape. Class MyPoint: Class MyPoint is used by class MyShape to define the reference point, p(x, y), of the Java display coordinate system, as well as by all subclasses in the class hierarchy to define the points stipulated in the subclass definition. The class utilizes a color of enum reference type MyColor, and includes appropriate class constructors and methods. The class also includes draw and toString methods, as well as methods that perform point related operations, including but not limited to, shift of point position, distance to the origin and to another point, angle [in degrees] with the x-axis of the line extending from this point to another point. Enum MyColor: Enum MyColor is used by class MyShape and all subclasses in the class hierarchy to define the colors of the shapes. The enum reference type defines a set of constant colors by their red, green, blue, and opacity, components,…arrow_forwardA complex number has the form a+bi , can be expressed as the ordered pair of real numbers (a,b). The class represents the real and imaginary parts as double precision values. Provide a constructor that enables an object of this class to be initialized when it is instantiated. The constructor should contain default values. Provide Public member functions for each of the following arithmetic’s functions (addition – subtraction – multiplication – division), a complex absolute value operation, printing the number in the form (a,b), printing the real part , printing the imaginary part and final overload the == operator to allow comparisons of two complex numbers. Include any additional operations that you think would be useful for a complex number class (c++)arrow_forward
- Construct a Time class containing integer data members seconds, minutes, and hours. Have the class contain two constructors: The first should be a default constructor having the prototype Time(int, int, int), which uses default values of 0 for each data member. The second constructor should accept a long integer representing a total number of seconds and disassemble the long integer into hours, minutes, and seconds. The final member method should display the class data members.arrow_forwarda) Create an enumeration named Departments that contain four sets of values FINANCE, HR, IT, and MARKETING.b) Create a class named PersonDetails that contains fields for a person’s first name (String), last name (String), and address (String). The class haveA constructor that accepts parameters for each field and Get methods for each field.c) Create a class named Employee that contains fields for an employee ID (int), Social Security number (String), personal information (Person object), department (Departments type), and annual salary (double). The class haveA constructor that requires values for each data field.Get methods for each of the data fields.Set methods for each of the data fields.d) Create an application/class named EmployeeSort that allows a user to enter values for an array of 5 Employee objects (create an array of Employee type that will save 5 employee objects). Prompt a user for the data needed to create an employee object. Continue to 2prompt the user for Social…arrow_forwardCreate an abstract class called Movie. The class should declare the following variables: an instance variable that describes the title - String an instance variable that describes the rating - double an instance variable that describes the director - String an instance variable that describes the genre - String an instance variable that describes the year – integer an instance variable that describes the language – String an instance variable that describes the ticket price - double Create the getter and setter methods for each instance variable except setter for ticket price and getter for genre. Create the necessary constructors. Include an abstract method setTicketPrice(double ticketPrice) to determine the ticket price for a movie. Include an abstract method getGenre() to return the genre of the movie. Provide a toString() method that returns the information stored in the above variables. Create three subclasses called ThrillerMovie, ComedyMovie, and ChildrenMovie. These…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
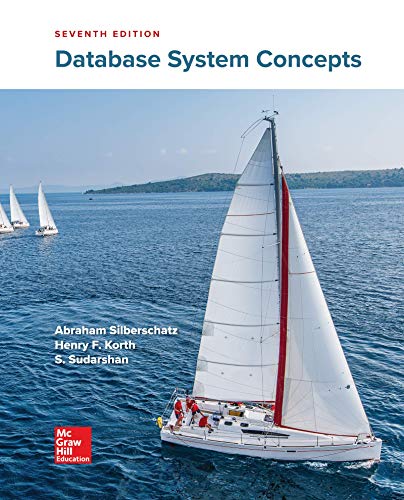
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
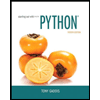
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
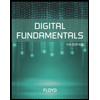
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
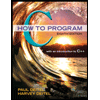
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
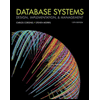
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
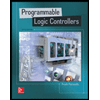
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education