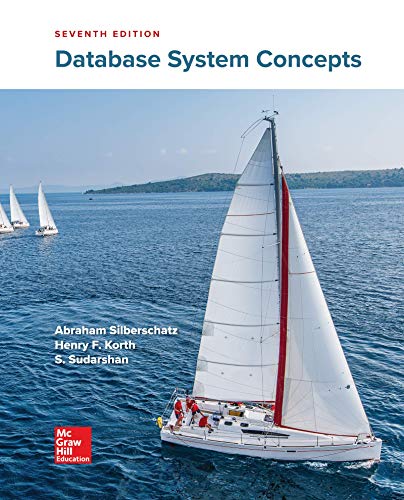
Concept explainers
C++ Programming Question 1:
Hi, need help with review question 1 if possible. Thanks.
Main Function:
int main() {
cout << endl;
IntArr a{5};
for(int i=0; i<5; i++) { a.push_back((i+1)*5); }
cout << "Array size: " << a.getSize() << endl;
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] << " "; }
cout << endl << endl;
a.push_back(30);
a.push_back(35);
cout << "Array size: " << a.getSize() << endl;
IntArr b = a;
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] << " "; }
cout << "\nPArray B: ";
for(int i=0; i<b.getSize(); i++) { cout << b[i] << " "; }
cout << endl << endl;
a.pop_back();
cout << "Array size: " << a.getSize() << endl;
b = a;
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] << " "; }
cout << "\nArray B: ";
for(int i=0; i<b.getSize(); i++) { cout << b[i] << " "; }
cout << endl << endl;
cout << endl;
return 0;
}
![1. Implement a class IntArr using dynamic memory.
a. data members:
capacity: maximum number of elements in the array
size: current number of elements in the array
array: a pointer to a dynamic array of integers
b. constructors:
default constructor: capacity and size are 0, array pointer is nullptr
user constructor: create a dynamic array of the specified size
c. overloaded operators:
subscript operator: return an element or exits if illegal index
d. "the big three":
copy constructor: construct an IntArr object using deep copy
assignment overload operator: deep copy from one object to another
destructor: destroys an object without creating a memory leak
e. grow function: "grow" the array to twice its capacity
f. push_back function: add a new integer to the end of the array
g. pop_back function: remove the last element in the array
h. getSize function: return the current size of the array (not capacity)
i. Use the provided main function (next page) and output example
Notes
a. grow function replaces the existing array with a new array
b. subscript operator should be a const member function
c. ensure that deep copy is used where applicable
d. push_back and pop_back functions require the grow function
e. push_back and pop_back functions should update array size (not capacity)
main:
int main() {
cout << endl;
}
IntArr a{5};
for(int i=0; i<5; i++)
{ a.push_back((i+1)*5); }
cout << "Array size: " << a.getSize() << endl;
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) { cout << a[i] << " '; }
cout << endl << endl;
a.push_back(30);
a.push_back(35);
cout << "Array size: " << a.getSize() << endl;
IntArr b = a;
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) {
cout << "\nPArray B: ";
for(int i=0; i<b.getSize(); i++) {
cout << endl << endl;
cout << "Array A: ";
for(int i=0; i<a.getSize(); i++) {
cout << "\nArray B: ";
for(int i=0; i<b.getSize(); i++) {
cout << endl << endl;
a.pop_back();
cout << "Array size: " << a.getSize() << endl;
b = a;
cout << endl;
return 0;
cout << a[i] << " "; }
Output:
cout << b[i] << " "; }
cout << a[i] << " "; }
cout << b[i] << " "; }
Array ze:
Array A: 5 10 15 20 25
Array size: 7
Array A: 5 10 15 20 25 30 35
PArray B: 5 10 15 20 25 30 35
Array size: 6
Array A: 5 10 15 20 25 30
Array B: 5 10 15 20 25 30](https://content.bartleby.com/qna-images/question/f0f5add9-d3dc-434f-a8cb-02542b3c567b/f57e34c2-0e08-422c-bf1e-1cc8e37a944a/wg5zkb_thumbnail.png)

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 5 images

- C++ Programming Redesign your class myArray using class templates so that the class can be used in any application that requires arrays to process data. #include <iostream>#include "myArray.h" using namespace std; int main(){myArray list1(5);myArray list2(5); int i; cout << "list1 : ";for (i = 0; i < 5; i++)cout << list1[i] << " ";cout << endl; cout << "Enter 5 integers: ";for (i = 0; i < 5; i++)cin >> list1[i];cout << endl; cout << "After filling list1: "; for (i = 0; i < 5; i++)cout << list1[i] << " ";cout << endl; list2 = list1;cout << "list2 : ";for (i = 0; i < 5; i++)cout << list2[i] << " ";cout << endl; cout << "Enter 3 elements: "; for (i = 0; i < 3; i++)cin >> list1[i];cout << endl; cout << "First three elements of list1: ";for (i = 0; i < 3; i++)cout << list1[i] << " ";cout << endl; myArray list3(-2, 6); cout <<…arrow_forward1. Implement a class IntArr using dynamic memory. a. data members: capacity: maximum number of elements in the array size: current number of elements in the array array: a pointer to a dynamic array of integers b. constructors: default constructor: capacity and size are 0, array pointer is nullptr user constructor: create a dynamic array of the specified size c. overloaded operators: subscript operator: return an element or exits if illegal index d. "the big three": copy constructor: construct an IntArr object using deep copy assignment overload operator: deep copy from one object to another destructor: destroys an object without creating a memory leak e. grow function: "grow" the array to twice its capacity f. push_back function: add a new integer to the end of the array g. pop_back function: remove the last element in the array h. getSize function: return the current size of the array (not capacity) i. Use the provided main function (next page) and output example Notes a. grow…arrow_forwardC++ complete and create magical square #include <iostream> using namespace std; class Vec {public:Vec(){ }int size(){return this->sz;} int capacity(){return this->cap;} void reserve( int n ){// TODO:// (0) check the n should be > size, otherwise// ignore this action.if ( n > sz ){// (1) create a new int array which size is n// and get its addressint *newarr = new int[n];// (2) use for loop to copy the old array to the// new array // (3) update the variable to the new address // (4) delete old arraydelete[] oldarr; } } void push_back( int v ){// TODO: if ( sz == cap ){cap *= 2; reserve(cap);} // complete others } int at( int idx ){ } private:int *arr;int sz = 0;int cap = 0; }; int main(){Vec v;v.reserve(10);v.push_back(3);v.push_back(2);cout << v.size() << endl; // 2cout << v.capacity() << endl; // 10v.push_back(3);v.push_back(2);v.push_back(3);v.push_back(4);v.push_back(3);v.push_back(7);v.push_back(3);v.push_back(8);v.push_back(2);cout…arrow_forward
- C++ need help with part d to g only Assume the Product structure is declared as follows: struct Product { string description; // Product description int partNum; // Part number double cost; // Product cost }; (c) Declare a dynamic array of Products of size 5 and name it ”items”. Then initilize it with user input values. (d) Write a print function (not as a member of the struct) and pass a pointer to the pointer that points to the array (double pointer) and print all the items of the array in that function. (e) Define a max function (not as a member of the struct) that gets an array of items as an input and returns a pointer to the max element of the array. (f) Declare a 3 by 3 two dimensional dynamic array and populate it. (g) Define an output function that takes a stream object and a pointer to a 2D array as arguments and outputs data members of objects in format of 3*3 table into the given stream. Test your function both with an output file…arrow_forwardC PROGRAMMING LANGUAGEarrow_forward#include <iostream>using namespace std;double median(int *, int);int get_mode(int *, int);int *create_array(int);void getinfo(int *, int);void sort(int [], int);double average(int *, int);int getrange(int *,int);int main(){ int *dyn_array; int students; int mode,i,range;float avrg;do{cout << "How many students will you enter? ";cin >> students;}while ( students <= 0 );dyn_array = create_array( students );getinfo(dyn_array, students);cout<<"\nThe array is:\n";for(i=0;i<students;i++)cout<<"student "<<i+1<<" saw "<<*(dyn_array+i)<<" movies.\n";sort(dyn_array, students);cout << "\nthe median is "<<median(dyn_array, students) << endl;cout << "the average is "<<average(dyn_array, students) << endl;mode = get_mode(dyn_array, students);if (mode == -1)cout << "no mode.\n";elsecout << "The mode is " << mode << endl;cout<<"The range of movies seen is…arrow_forward
- struct remove_from_front_of_vector { // Function takes no parameters, removes the book at the front of a vector, // and returns nothing. void operator()(const Book& unused) { (/// TO-DO (12) ||||| // // // Write the lines of code to remove the book at the front of "my_vector". // // Remember, attempting to remove an element from an empty data structure is // a logic error. Include code to avoid that. //// END-TO-DO (12) /||, } std::vector& my_vector; };arrow_forwardc++ language using addition, not get_sum function with this pseudocode: Function Main Declare Integer Array ages [ ] Declare integer total assign numbers = [ ] assign total = sum(ages) output "Sum: " & total end fucntion sum(Integer Array array) declare integer total declare integer index assign total = 0 for index = 0 to size(array) -1 assign total = total + array[ ] end return integer totalarrow_forwardclass Main { // this function will return the number elements in the given range public static int getCountInRange(int[] array, int lower, int upper) { int count = 0; // to count the numbers // this loop will count the numbers in the range for (int i = 0; i < array.length; i++) { // if element is in the range if (array[i] >= lower && array[i] <= upper) count++; } return count; } public static void main(String[] args) { // array int array[] = {1,2,3,4,5,6,7,8,9,0}; // ower and upper range int lower = 1, upper = 9; // throwing an exception…arrow_forward
- Activity 1 This program firstly initializes an object of struct Cirele with values and then updates those using pointers. The program uses structure notation once and pointer notation another afterwards. #include #include #define MIN 1 #define MAX 30 struct Circle { float xCoord; float yCoord; float radius; }; /* prototypes */ float getRandomNumber (int min, int max); void printByValue(struct Circle cirobj); void setValue(float *target, float newValue); void printByReference(struct Circle *cirObj); int main(void) { /* Declare a struct circle and name it circleobject */ struct Circle circleObject; /* Fill circleObject's members with random numbers */ circleobject.xCoord = getRandomNumber(MIN, MAX); circleobject.yCoord = getRandomNumber (MIN, MAX); circleobject.radius = getRandomNumber (MIN, MAX); /* Printing values of the circle by calling function print by value */ printByValue(circleobject); /* update values of circle one by one */ setValue (&circleobject.xCoord, getRandomNumber(MIN,…arrow_forwardInstructions: Turn all instances of classes into pointers. You will also need to combine the player and vector into one vector objects and fix all issues this causes. #ifndef ITEM_H #define ITEM_H class Item { public: Item() {}; enum class Type { sword, armor, shield, numTypes }; Item(Type classification, int bonusValue); Type getClassification() const; int getBonusValue() const; private: Type classification{ Type::numTypes }; int bonusValue{ 0 }; }; std::ostream& operator<< (std::ostream& o, const Item& src); bool operator< (const Item& srcL, const Item& srcR); int& operator+=(int& srcL, const Item& srcR); #endif // !ITEM_H #ifndef MONSTER_H #define MONSTER_H #include "Object.h" class Player; class Monster : public Object { public: Monster() {}; Monster(const Player& player); void update(Player& player, std::vector& monsters) override; int attack() const override; void defend(int damage) override; void print(std::ostream& o)…arrow_forwarduse c++ Programming language Write a program that creates a two dimensional array initialized with test data. Use any data type you wish . The program should have following functions: .getAverage: This function should accept a two dimensional array as its argument and return the average of each row (each student have their average) and each column (class test average) all the values in the array. .getRowTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a row in the array. The function should return the total of the values in the specified row. .getColumnTotal: This function should accept a two dimensional array as its first argument and an integer as its second argument. The second argument should be the subscript of a column in the array. The function should return the total of the values in the specified column. .getHighestInRow: This function should accept a two…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
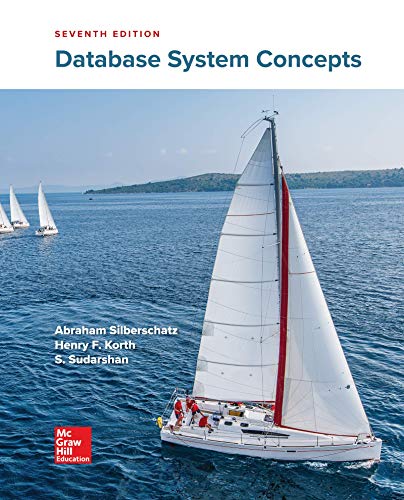
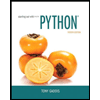
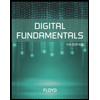
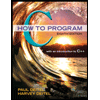
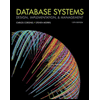
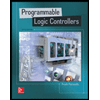