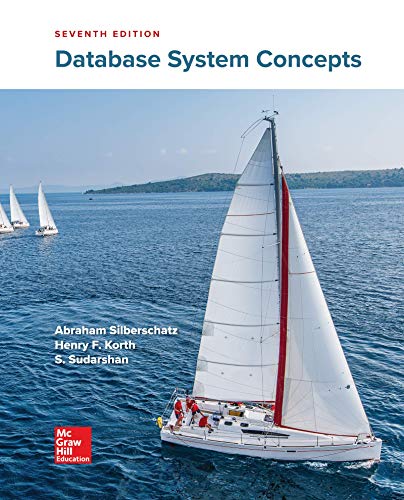
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
thumb_up100%
![1. Implement and grow a dynamic array using pointer arithmetic.
a) Use the provided main function (see below).
b) Implement a populate function which stores values from 0 to size
into the array p using pointer arithmetic to access array locations.
c) Implement a print function which prints the values of the array
p using pointer arithmetic.
d) Implement a printMemory function which prints the memory addresses
of all elements in array p using pointer arithmetic.
e) Implement a grow function which resizes the existing array from
the initial size to a new size using pointer arithmetic.
f) Verify via the output that the new array is a distinct memory space
from the original array.
Main
Output Example
int main( ) {
cout <« endl;
Enter a size: 3
int size, newSize;
cout <« "Enter a size: ";
cin >> size;
original:
0 1 2
0x7fc2ald0000
0x7fc2a1d00004
cout <« endl;
0x7fc2ald00008
int *p = new int[size]();
cout <« "Original:
populate(p, size);
print(p, size);
printMemory(p, size);
<< endl;
Enter a new size: 5
Inside grOw :
0 1 2 0 0
0x7fc2ale00000
0x7fc2ale@0004
0x7fc2 ale00008
0x7fc2ale0000c
cout <« endl;
cout « "Enter a new size: ";
cin >> newSize;
cout << endl;
0x7fc2ale00010
grow(p, size, newSize);
cout <« "After grow:
print(p, newSize);
printMemory(p, newSize);
After gr ow:
0 1 2 0 0
0x7fc2 ale00000
0x7fc2ale00004
0x7fc2ale00008
0x7fc2ale0000c
0x7fc2a1e00010
« endl;
cout « endl;
return 0;](https://content.bartleby.com/qna-images/question/c45072c9-820f-4d06-bb43-63ea77dc776c/584562f5-f1ca-4ad8-810d-11c8b8d9f201/oztti7c.png)
Transcribed Image Text:1. Implement and grow a dynamic array using pointer arithmetic.
a) Use the provided main function (see below).
b) Implement a populate function which stores values from 0 to size
into the array p using pointer arithmetic to access array locations.
c) Implement a print function which prints the values of the array
p using pointer arithmetic.
d) Implement a printMemory function which prints the memory addresses
of all elements in array p using pointer arithmetic.
e) Implement a grow function which resizes the existing array from
the initial size to a new size using pointer arithmetic.
f) Verify via the output that the new array is a distinct memory space
from the original array.
Main
Output Example
int main( ) {
cout <« endl;
Enter a size: 3
int size, newSize;
cout <« "Enter a size: ";
cin >> size;
original:
0 1 2
0x7fc2ald0000
0x7fc2a1d00004
cout <« endl;
0x7fc2ald00008
int *p = new int[size]();
cout <« "Original:
populate(p, size);
print(p, size);
printMemory(p, size);
<< endl;
Enter a new size: 5
Inside grOw :
0 1 2 0 0
0x7fc2ale00000
0x7fc2ale@0004
0x7fc2 ale00008
0x7fc2ale0000c
cout <« endl;
cout « "Enter a new size: ";
cin >> newSize;
cout << endl;
0x7fc2ale00010
grow(p, size, newSize);
cout <« "After grow:
print(p, newSize);
printMemory(p, newSize);
After gr ow:
0 1 2 0 0
0x7fc2 ale00000
0x7fc2ale00004
0x7fc2ale00008
0x7fc2ale0000c
0x7fc2a1e00010
« endl;
cout « endl;
return 0;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

Knowledge Booster
Similar questions
- // Description: Write a function Partition (f, l, p, a) // which partitions array a into two parts, left and right. // Elements of a in the left part should be smaller than p, // and the elements of a great than or eqaul to p should be in the right part. // F should set to the first element of a partition of array a to be partioned, // and l is the left element of a to be subjected to the partition. In C++ please, must include partition and swap functionsarrow_forwarda) Write the definition of a subroutine that swaps the first and the last members of an array of 10double-words. Note that the array is pointed by SI.b) Write the definition of another subroutine, which multiplies the least significant nibble and the most significant nibble of AX register. The result is to be saved into AL.arrow_forwardAHPA #11: Changing Grades * * Create a C function (switcher) that will receive a pointer to the finalExams array, using only pointers look for D scores and boost them to C scores. (ouput should be same as picture)arrow_forward
- 1-Write python code that implements an indexed array and an associative array (dictionary). 2- Use examples to explain the two main problems with Pointers 3- What is the output of the following Python code: x=3 y="5" print (x+y)arrow_forwardDESIGN YOUR OWN SETTING Task: Devise your own setting for storing and searching the data in an array of non-negative integers redundantly. You may just describe the setting without having to give an explicit algorithm to explain the process by which data is stored. You should explain how hardware failures can be detected in your method. Once you have described the setting, include the following to your answer: Write a pseudocode function to describe an algorithm where the stored data can be searched for a value key: if the data is found, its location in the original array should be returned; -1 should be returned if the data is not found; -2 should be returned if there is a data storage error Include a short commentary explaining why your pseudocode works Describe the worst-case and best-case inputs to your search algorithm Derive the worst-case and best-case running times for the search algorithm Derive the Theta notation for the worst-case and best-case running timesarrow_forwardNeed help with this debuggin exercise. 1.) Assume the following main module is in a program that includes the binarySearch function. Why doesn't the pseudocode in the main module work? //This program uses the binarySearch function to search for a //name in the array. This program assumes the binarySearch //function has alreday been defined. Modulemain() Constant Integer SIZE= 5 Declare String names[SIZE]= "Zack", "James", "Pam", "Marc", "Susan" Declare String searchName Declare Integer index Display "Enter a name to search for." Input searchName Set index = binarySearch(names, searchName, SIZE) If index != - 1 Then Display searchName, " was found." Else Display searchName, " was NOT found." End IF End Module All coding is in Python.arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
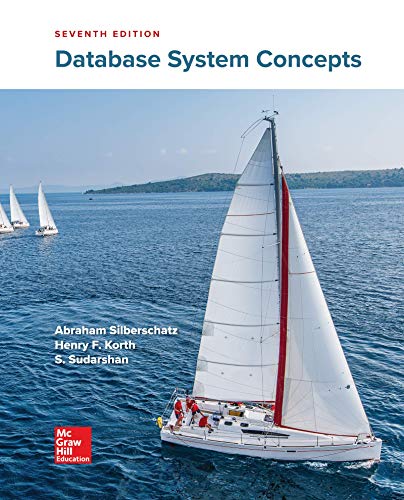
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
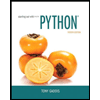
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
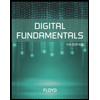
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
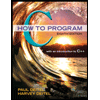
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
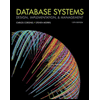
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
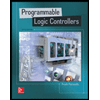
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education