Hello, I am having trouble with my c++ homework. Implement and grow a dynamic array using pointer arithmetic. a) Use the provided main function (see below). b) Implement a populate function which stores values from 0 to size into the array p using pointer arithmetic to access array locations. c) Implement a print function which prints the values of the array p using pointer arithmetic. d) Implement a printMemory function which prints the memory addresses of all elements in array p using pointer arithmetic. e) Implement a grow function which resizes the existing array from the initial size to a new size using pointer arithmetic. f) Verify via the output that the new array is a distinct memory space from the original array.
Hello, I am having trouble with my c++ homework.
Implement and grow a dynamic array using pointer arithmetic.
a) Use the provided main function (see below).
b) Implement a populate function which stores values from 0 to size into the array p using pointer arithmetic to access array locations.
c) Implement a print function which prints the values of the array p using pointer arithmetic.
d) Implement a printMemory function which prints the memory addresses of all elements in array p using pointer arithmetic.
e) Implement a grow function which resizes the existing array from the initial size to a new size using pointer arithmetic.
f) Verify via the output that the new array is a distinct memory space from the original array.
Main: Output Example:
Use the following main function to test your program. (cannot change the int main provided)
int main( ) {
cout << endl;
int size, newSize;
cout << "Enter a size: ";
cin >> size;
cout << endl;
int *p = new int[size]();
cout << "Original: " << endl;
populate(p, size);
print(p, size);
printMemory(p, size);
cout << endl;
cout << "Enter a new size: ";
cin >> newSize;
cout << endl;
p = grow(p, size, newSize);
cout << "After grow: " << endl;
print(p, newSize);
printMemory(p, newSize);
cout << endl;
return 0;
}
![Main:
Output Example:
int main( ) {
Enter a size: 5
cout << endl;
Original:
int size, newsize;
0 12 3 4
cout << "Enter a size: ";
Ox7f970bd04080
cin >> size;
Ox7f970bd04084
Ox7f970bd04088
cout << endl;
Ox7f970bd0408c
int +p = new int [size]):
cout << "Original: " << endl;
Ox7f970bd04090
populate(p, size);
print(p, size);
printMenory(p, size);
Enter a new size:
cout << endl;
Inside grow:
0 1 2
Ox7f970bd040a0
cout << "Enter a new size: ";
cin >> newSize;
Ox7f970bd040a4
Ox7f970bd040a8
cout « endl;
p = grow(p, size, newsize);
cout <« "After grow:
print(p, newSize);
printMenory(p, newSize);
« endl;
After grow:
0 1 2
Ox7f970bd040a0
cout << endl;
Ox7f970bd040a4
Ox7f970bd040a8
return e;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F86bfc21f-7304-4d6a-8ae3-de059a5180e7%2F1d447373-9dee-48ee-9d1c-3efe35d10c17%2Ftjgfa3_processed.png&w=3840&q=75)

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

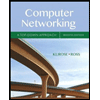
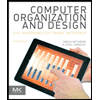
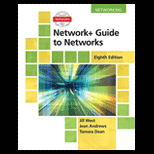
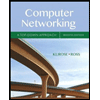
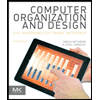
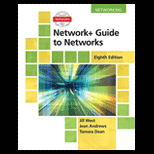
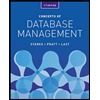
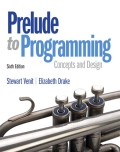
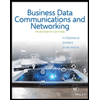