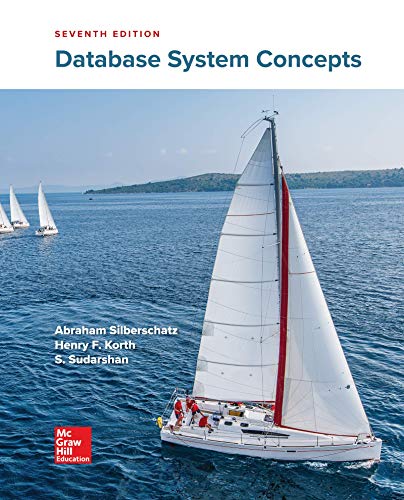
Q1. Within your Jupyter Notebook, write the code for a Python function called
def parseWeatherByYear(year) :
This function will parse an html page containing weather for an entire year of data for the city of Toronto.
The html pages containing weather data can be downloaded from: https://www.extremeweatherwatch.com/cities/toronto/year-2023
The file to parse for this lab however can be downloaded here: https://matrix.senecacollege.ca/~danny.abesdris/prg550.232/labs/lab6/torontoWeather.2023.html
The html file itself contains markers as where to begin parsing the data to extract. The 3 pieces of data that must be extracted consist of the high and low temperatures (in degrees Celsius) as well as the amount of precipitation (in cm) for every day so far in the current year (2023).
A series of lines containing where to begin extracting data is listed below:
<td><div class='width-130'><a href='/cities/toronto/day/january-1'>January 1</a></div></td>
<td class='text-right temp40'>5.0</td>
<td class='text-right temp30'>2.7</td>
<td class='text-right rainsnow1'>0.15</td>
</tr>
Notice the marker in the lines above:
/cities/toronto/day/month-n
In the example above, the data to extract would be: 5.0, 2.7, and 0.15. The extraction can be achieved in several ways, but a carefully structured regular expression (using the match.group( ) directive as well as the re.S and re.M flags) is recommended for speed and simplicity. The trick here is to match text up to the point where the data begins (as groups) and then forming another regular expression that matches the data (again as a group).

Step by stepSolved in 3 steps

- NO REGEX OR ANYTHING COMPLICATED PLEASE.. USE BASIC APPROACH Create a program in Python that reads data from Breakfast Menu (https://www.w3schools.com/xml/simple.xml) and builds parallel arrays for the menu items, with each array containing the menu item name, description, calories, and price, respectively. After reading the data and building the arrays, display the menu items similar to the following: name - description - calories - priceAt the bottom, display the total number of items on the menu, the average number of calories per item, and the average price per item similar to: 0 items - 0 average calories - $0.00 average price You may either read the page using Internet processing methods, or you may download and save the page and then read the data from the saved file. You must process the data using string functions (no XML libraries). must use separate subroutines/functions/methods to implement each type of processing, and include error handling for missing or invalid…arrow_forwardNEED HELP DEBUGGING THE FOLLOW JS CODE JS CODE /* JavaScript 7th Edition Chapter 3 Project 03-05 Application to generate a horizontal bar chart Author: Date: Filename: project03-05.js*/ // Array of phone models sold by the companylet phones = ("Photon 6E", "Photon 6X", "Photon 7E", "Photon 7X", "Photon 8P"); // Units sold in the previous quarterlet sales = (10281, 12255, 25718, 21403, 16142); // Variable to calculate total saleslet totalSales = 0; // Use the forEach() method to sum the sales for each phone model and add it to the totalSales variablesales.forEach(addtoTotal); // For loop to generate bar chart of phone salesfor (let i = 1; i <= phones.length; i++) { let barChart = ""; // Variable to store HTML code for table cells used to create bar chart // Calculate the percent of total sales for a particular phone model let barPercent = 100*sales/totalSales; let cellTag; // Variable that will define the class of td…arrow_forwardFrom the image attached, using C not C++, provide the code, as well as the required output. NOTE: Do not hard code, as all inputs are required for the output.arrow_forward
- Please help me with these question. I am having trouble understanding what to do. Please use HTML, CSS, and JavaScript Thank youarrow_forwardplease use python.arrow_forwardIn Python code: Using this website: https://en.wikipedia.org/wiki/Greenhouse_gasCreate a named tuple to contain each Gas Concentration data for these columns: Gas,Pre-1750,Recent,Absolute increase since 1750,Percentage increase since 1750. Design a class to hold this named tuple and add necessary dunders to support initialization, string conversion, sorting by a specific column (like 'Recent' for example) and searching.arrow_forward
- JQuery loading Consider following code $(function(){ // jQuery methods go here... }); What is the purpose of this code with respect to JQuery ? O This is to prevent any jQuery code from running before the document is finished loading (is ready). O This is to load the jQuery and to run this as soon as page start before loading. This is to load the function so we can run jQuery codearrow_forwardIn c++, Using STL containers, components, and algorithms show all data from the text file movies.txt. Please make sure the list updates once the user adds a new movie to the text file. Console Movies program COMMAND MENU show - user is able to see all available movies from movies.txt command: show The Adventures of a Babysitter $10 Barney $25 Larry the Car Man $15 Dora and the missing map $15 Ceasar and the Lunch Lady $10 Suzie and the Bad Kids $13.95 command: add cout << "What movie do you want to add?"; cin >> BeeHive $12; cout << "Movie added"; command:show The Adventures of a Babysitter $10 Barney $25 Larry the Car Man $15 Dora and the missing map $15 Ceasar and the Lunch Lady $10 Suzie and the Bad Kids $13.95 Beehive $12 movies.txt with all possible movies: The Adventures of a Babysitter $10 Barney $25 Larry the Car Man $15 Dora and the missing map $15 Ceasar and the Lunch Lady $10 Suzie and the Bad Kids $13.95arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
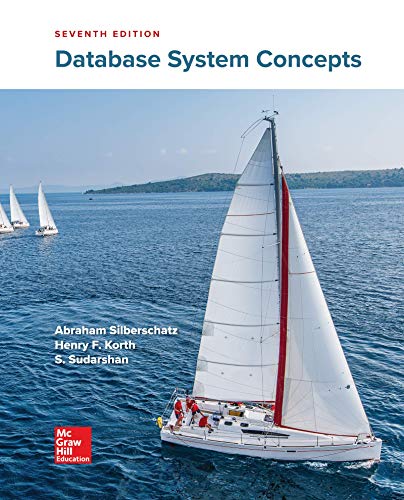
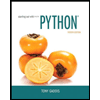
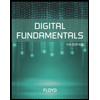
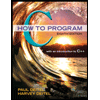
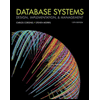
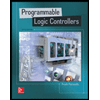