1. Write a program to produce an array of integer random numbers. Your program should find out from the user how many numbers to store. It should then generate and store that many random integers (the random numbers must be between 1 and 999 inclusive). The program should then determine the smallest number, the largest number, and the average of all the numbers stored in the array. Finally, it should print out all the numbers on the screen, five numbers to a line with spaces in between. Once the contents of the array have been printed to screen, display the smallest number, largest number, and average determined previously. You should ensure that your program design in modular. The Random class of Java library (java.util.Random) implements a random number generator. To generate random numbers, you construct an object of q the class Random, and then use the method nextInt(n) which returns a number between 0 (inclusive) and n (exclusive). Eg: ICT167 Principles of Computer Science 2 import java.util.Random; ..... Random generator = new Random(); ..... //generate a random number between 0 and 99 (inclusive) int nextRand = generator.nextInt(100); Alternatively use Math.random(), which returns a random value in the range 0.0 to 1.0 (then adjust to the correct range)
1. Write a program to produce an array of integer random numbers. Your
program should find out from the user how many numbers to store. It should
then generate and store that many random integers (the random numbers must
be between 1 and 999 inclusive). The program should then determine the
smallest number, the largest number, and the average of all the numbers stored
in the array. Finally, it should print out all the numbers on the screen, five
numbers to a line with spaces in between. Once the contents of the array have
been printed to screen, display the smallest number, largest number, and
average determined previously. You should ensure that your program design in
modular.
The Random class of Java library (java.util.Random) implements a random
number generator. To generate random numbers, you construct an object of q
the class Random, and then use the method nextInt(n) which returns a number
between 0 (inclusive) and n (exclusive). Eg:
ICT167 Principles of Computer Science
2
import java.util.Random;
.....
Random generator = new Random();
.....
//generate a random number between 0 and 99 (inclusive)
int nextRand = generator.nextInt(100);
Alternatively use Math.random(), which returns a random value
in the range 0.0 to 1.0 (then adjust to the correct range)
2. Create a UML diagram to help design the class described in exercise 3 below.
Do this exercise before you attempt to code the solution. Think about what
instance variables will be required to describe a Baby class object; should they
be private or public? Determine what class methods are required; should they
be private or public?
3. Write Java code for a Baby class. A Baby has a name of type String and an age
of type integer.
Supply two constructors: one will be the default constructor, that just sets
default values for the name and age; the second constructor will take two
parameters, a string to set the name and an integer to set the age. Also, supply
methods for setting the name, setting the age, getting the name and getting the
age.
The class should not contain I/O methods; input of values to the instance
variables must be done with a set method or constructor, output of values from
the instance variables must be done with get methods. The set method for the
name instance variable should ensure that the input is not empty or contain
whitespaces (otherwise set a default value). The set method for the age
instance variable should validate the input to be between 1 and 4 inclusive
(otherwise set a default value).
Give Java code for an equals method for the Baby class. Babies count as
being the same (i.e. equal) if their names and their ages are exactly identical
(names should not be case sensitive). The method will take a Baby type
parameter and use the calling object (thus comparing these two objects via
name and age); it should return Boolean - true or false as appropriate.
Remember, if comparing Strings, you must use String comparison methods.
4. Test your Baby class by writing a client program which uses an array to store
information about 4 babies. That is, each of the four elements of the array
must store a Baby object.
If you have an array for baby names and another array for baby ages,
then you have missed the point of the exercise and therefore not met
the requirement of this exercise.
ICT167 Principles of Computer Science
3
A Baby class object stores the required information about a Baby. So
each Baby object will have its own relevant information, and thus each
object must be stored in one element of the array.
The client program should:
a. Enter details for each baby (name and age) and thus populate the
Baby array
b. Output the details of each baby from the array (name and age)
c. Calculate and display the average age of all babies in the array
d. Determine whether any two babies in the array are the same
As the required information for these tasks is stored in the Baby array, you
will need to use a loop to access each array element (and use the dot notation
to access the appropriate set and get methods to assign/retrieve the
information)

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

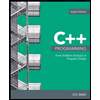
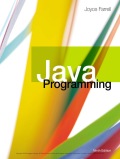
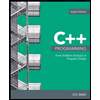
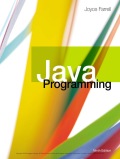