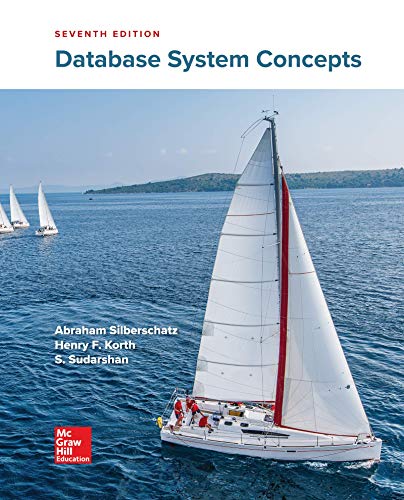
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Need help completing the rest of this code

Transcribed Image Text:2. Code a top-down recursive solution for the classic Fibonacci Sequence
(starting from 0) using dynamic programming with heap memory for storage.
![fibonacci top-down solution (memoization)
0(n) time complexity
Recursive Function
step 1: if n < 2 return the data at position n
step 2: else, if a solution for n has not been stored in a[n]
recurse fibo(n-1) + fibo (n-2) and store the answer into a[n]
step 3: return the data at position n in the array
*/
int fibonacci(int n, long long *a){
if(n == 0){
return 0;
}
if(n == 1){
return 1;
}
else(n=!-1)
return fibonacci(n-1) + fibonacci(n-2);
}
int main (){
// step 1: create a dynamic array of 50 integers.
// step 2: populate the first two indicies of the array with 0 and 1
// step 3: populate the rest of the array with the value -1
// step 4: call the fibonacci sequence for various values of n
int *a = new int[50]{0, 1};
cout <« endl;
cout << "fib(0): " << fibonacci(0) < endl;
cout << "fib(6): " << fibonacci(6) << endl;
" < fibonacci(8) << endl;
<« fibonacci(15) << endl;
cout << "fib(20): " << fibonacci(20) << endl;
cout << "fib(37): " << fibonacci(37) << endl;
cout << "fib(8):
cout << "fib(15):
cout <« endl;
delete [] a;
return 0;
}](https://content.bartleby.com/qna-images/question/c45072c9-820f-4d06-bb43-63ea77dc776c/fa49955e-b109-4bd2-820e-d4a9c6f096d4/jqplwyn.png)
Transcribed Image Text:fibonacci top-down solution (memoization)
0(n) time complexity
Recursive Function
step 1: if n < 2 return the data at position n
step 2: else, if a solution for n has not been stored in a[n]
recurse fibo(n-1) + fibo (n-2) and store the answer into a[n]
step 3: return the data at position n in the array
*/
int fibonacci(int n, long long *a){
if(n == 0){
return 0;
}
if(n == 1){
return 1;
}
else(n=!-1)
return fibonacci(n-1) + fibonacci(n-2);
}
int main (){
// step 1: create a dynamic array of 50 integers.
// step 2: populate the first two indicies of the array with 0 and 1
// step 3: populate the rest of the array with the value -1
// step 4: call the fibonacci sequence for various values of n
int *a = new int[50]{0, 1};
cout <« endl;
cout << "fib(0): " << fibonacci(0) < endl;
cout << "fib(6): " << fibonacci(6) << endl;
" < fibonacci(8) << endl;
<« fibonacci(15) << endl;
cout << "fib(20): " << fibonacci(20) << endl;
cout << "fib(37): " << fibonacci(37) << endl;
cout << "fib(8):
cout << "fib(15):
cout <« endl;
delete [] a;
return 0;
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Language is C++arrow_forwardIn this lab, you use what you have learned about parallel arrays to complete a partially completed C++ program. The program should: Either print the name and price for a coffee add-in from the Jumpin’ Jive Coffee Shop Or it should print the message Sorry, we do not carry that. Read the problem description carefully before you begin. The file provided for this lab includes the necessary variable declarations and input statements. You need to write the part of the program that searches for the name of the coffee add-in(s) and either prints the name and price of the add-in or prints the error message if the add-in is not found. Comments in the code tell you where to write your statements. Instructions Study the prewritten code to make sure you understand it. Write the code that searches the array for the name of the add-in ordered by the customer. Write the code that prints the name and price of the add-in or the error message, and then write the code that prints the cost of the total…arrow_forwardIn the expression 48:(14*3), are the brackets necessary? What happens when you type 48:14*3?arrow_forward
- C++ HELP In this lab, you're going to be working with partially filled arrays that are parallel with each other. That means that the row index in multiple arrays identifies different pieces of data for the same person. This is a simple payroll system that just calculates gross pay given a set of employees, hours worked for the week and hourly rate. Parallel Arrays First, you have to define several arrays in your main program employee names for each employee hourly pay rate for each employee total hours worked for each employee gross pay for each employee You can use a global SIZE of 50 to initialize these arrays Second, you will need a two dimension (2-D) array to record the hours worked each day for an employee. The number of rows for the 2-D array should be the same as the arrays above since each row corresponds to an employee. The number of columns represents days of the week (7 last I looked) Functions Needed In this lab, you must read in the employee names first because this…arrow_forwardWhat would be the missing line of codes?arrow_forwardhelp me solve this in c++ please Write a program that asks the user to enter a list of numbers from 1 to 9 in random order, creates and displays the corresponding 3 by 3 square, and determines whether the resulting square is a Lo Shu Magic Square. Notes Create the square by filling the numbers entered from left to right, top to bottom. Input validation - Do not accept numbers outside the range. Do not accept repeats. Must use two-dimensional arrays in the implementation. Functional decomposition — Program should rely on functions that are consistent with the algorithm.arrow_forward
- Please code in python Kiki is making “Happy National Pizza Day” (February 9th) cards for all of her friends. She’s a bit disappointed with the cards not looking special enough, so she decides to add glitter to them to make them look more special. However, she’s short on glitter, so she decides to add glitter selectively. She uses a box with N*N divided sections to store her cards. Each section is huge and each card is small, so she can put multiple cards in a given section and they will never overlap. We model the way that she organizes the cards and adds glitter using two commands: Command 1: 1 x y: add a card to the box in section (x, y). Command n: 2 x1 y1 x2 y2: Add one unit of glitter to each of the cards in the sections from (x1, y2) to (x2, y2). Help Kiki determine the total number of units of glitter that she placed on the cards. Input The first line will contain N (1 <= N <= 500) and C (1 <= C <= 500 ), the square size of the card storage box…arrow_forward18. Given the following C code, what is the value of scores[5]? int scores[10] [95,82);arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
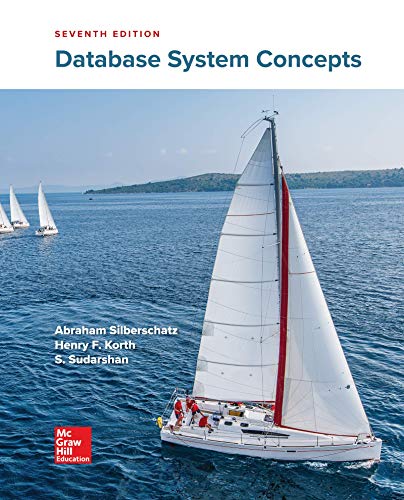
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
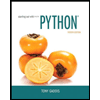
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
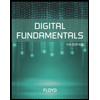
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
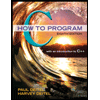
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
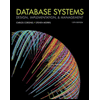
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
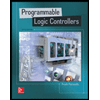
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education