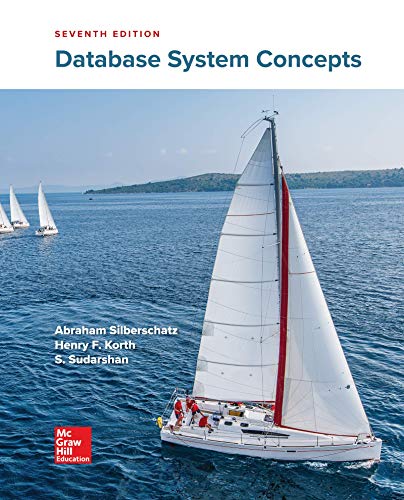
Concept explainers
C++ Given code
#include <
#include <iostream>
#include <algorithm>
using namespace std;
// The puzzle will always have exactly 20 columns
const int numCols = 20;
// Searches the entire puzzle, but may use helper functions to implement logic
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords);
// Printer function that outputs a vector
void printVector(const vector <string> &v);
// Example of one potential helper function.
// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,
// int rowStart, int colStart)
int main()
{
int numRows, numWords;
// grab the array row dimension and amount of words
cin >> numRows >> numWords;
// declare a 2D array
char puzzle[numRows][numCols];
// TODO: fill the 2D array via input
// read the puzzle in from the input file using cin
// create a 1D array for wods
string wordBank[numWords];
// TODO: fill the wordBank through input using cin
// set up discovery vector
vector <string> discovered;
// Search for Words
searchPuzzle(puzzle, wordBank, discovered, numRows, numWords);
// Sort the results
sort(discovered.begin(), discovered.end());
// Print vector of discovered words
printVector(discovered);
return 0;
}
// TODO: implement searchPuzzle and any helper functions you want
void searchPuzzle(const char puzzle[][numCols], const string wordBank[],
vector <string> &discovered, int numRows, int numWords)
{
}
// TODO: implement printVector
void printVector(const vector <string> &v)
{
}
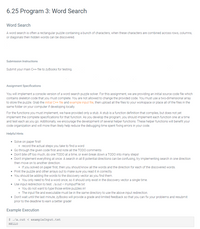
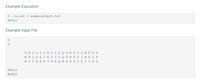

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps

- 23. In the C++ language write a program to find the product of all the elements present in the array of integers given below. int numArr[5] = {16,4, 8, 7, 12};arrow_forwardc++ programming Initialize a string array named person with your first, middle andlast name as separate elements. Print the middle name from the array. Iterate to print all values of the array. Output Example WilliamJohn William Henryarrow_forwardinitial c++ file/starter code: #include <vector>#include <iostream>#include <algorithm> using namespace std; // The puzzle will always have exactly 20 columnsconst int numCols = 20; // Searches the entire puzzle, but may use helper functions to implement logicvoid searchPuzzle(const char puzzle[][numCols], const string wordBank[],vector <string> &discovered, int numRows, int numWords); // Printer function that outputs a vectorvoid printVector(const vector <string> &v);// Example of one potential helper function.// bool searchPuzzleToTheRight(const char puzzle[][numCols], const string &word,// int rowStart, int colStart) int main(){int numRows, numWords; // grab the array row dimension and amount of wordscin >> numRows >> numWords;// declare a 2D arraychar puzzle[numRows][numCols];// TODO: fill the 2D array via input// read the puzzle in from the input file using cin // create a 1D array for wodsstring wordBank[numWords];// TODO:…arrow_forward
- #include <stdio.h>#include <stdlib.h>#include <time.h> struct treeNode { struct treeNode* leftPtr; int data; struct treeNode* rightPtr;}; typedef struct treeNode TreeNode;typedef TreeNode* TreeNodePtr; void insertNode(TreeNodePtr* treePtr, int value);void inOrder(TreeNodePtr treePtr);void preOrder(TreeNodePtr treePtr);void postOrder(TreeNodePtr treePtr); int main(void) { TreeNodePtr rootPtr = NULL; srand(time(NULL)); puts("The numbers being placed in the tree are:"); for (unsigned int i = 1; i <= 10; ++i) { int item = rand() % 15; printf("%3d", item); insertNode(&rootPtr, item); } puts("\n\nThe preOrder traversal is: "); preOrder(rootPtr); puts("\n\nThe inOrder traversal is: "); inOrder(rootPtr); puts("\n\nThe postOrder traversal is: "); postOrder(rootPtr);} void insertNode(TreeNodePtr* treePtr, int value) { if (*treePtr == NULL) { *treePtr = malloc(sizeof(TreeNode)); if…arrow_forward#include <iostream>#include <vector>using namespace std; void PrintVectors(vector<int> numsList) { unsigned int i; for (i = 0; i < numsList.size(); ++i) { cout << numsList.at(i) << " "; } cout << endl;} int main() { vector<int> numsList; int userInput; int i; for (i = 0; i < 3; ++i) { cin >> userInput; numsList.push_back(userInput); } numsList.erase(numsList.begin()+1); numsList.insert(numsList.begin()+1, 102); numsList.insert(numsList.begin()+1, 100); PrintVectors(numsList); return 0;} Not all tests passed clearTesting with inputs: 33 200 10 Output differs. See highlights below. Special character legend Your output 33 100 102 10 Expected output 100 33 102 10 clearTesting with inputs: 6 7 8 Output differs. See highlights below. Special character legend Your output 6 100 102 8 Expected output 100 6 102 8 Not sure what I did wrong but the the 33…arrow_forward# dates and times with lubridateinstall.packages("nycflights13") library(tidyverse)library(lubridate)library(nycflights13) Qustion: Create a function called date_quarter that accepts any vector of dates as its input and then returns the corresponding quarter for each date Examples: “2019-01-01” should return “Q1” “2011-05-23” should return “Q2” “1978-09-30” should return “Q3” Etc. Use the flight's data set from the nycflights13 package to test your function by creating a new column called quarter using mutate()arrow_forward
- #include <iostream>#include <cstdlib>#include <time.h>#include <chrono> using namespace std::chrono;using namespace std; void randomVector(int vector[], int size){ for (int i = 0; i < size; i++) { //ToDo: Add Comment vector[i] = rand() % 100; }} int main(){ unsigned long size = 100000000; srand(time(0)); int *v1, *v2, *v3; //ToDo: Add Comment auto start = high_resolution_clock::now(); //ToDo: Add Comment v1 = (int *) malloc(size * sizeof(int *)); v2 = (int *) malloc(size * sizeof(int *)); v3 = (int *) malloc(size * sizeof(int *)); randomVector(v1, size); randomVector(v2, size); //ToDo: Add Comment for (int i = 0; i < size; i++) { v3[i] = v1[i] + v2[i]; } auto stop = high_resolution_clock::now(); //ToDo: Add Comment auto duration = duration_cast<microseconds>(stop - start); cout << "Time taken by function: " << duration.count()…arrow_forwardİn C Language pls help #include <stdio.h>#include <stdlib.h>#include <string.h> typedef struct ArrayList_s{ void **list; int size; int capacity; int delta_capacity;} ArrayList_t, *ArrayList; ArrayList arraylist_create(int capacity, int delta_capacity){ ArrayList l; l = (ArrayList)malloc(sizeof(ArrayList_t)); if (l != NULL) { l->list = (void **)malloc(capacity * sizeof(void *)); if (l->list != NULL) { l->size = 0; l->capacity = capacity; l->delta_capacity = delta_capacity; } else { free(l); l = NULL; } } return l;} void arraylist_destroy(ArrayList l){ free(l->list); free(l);} int arraylist_isempty(ArrayList l){ return l->size == 0;} int arraylist_isfull(ArrayList l){ return l->size == l->capacity;} int arraylist_set(ArrayList l, void *e, int index, void **replaced){ if (!arraylist_isfull(l)) {…arrow_forward#include #include using namespace std; void PrintSize(vector numsList) { cout intList (3); PrintSize(intList); cin >> currval; while (currval >= 0) { } Type the program's output intList.push_back(currval); cin >> currVal; PrintSize(intList); intList.clear(); PrintSize(intList); return 0; 1 se mice with camScanner Check Next Input 1234-1 Outputarrow_forward
- Game of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward#include using namespace std; struct ListNode { string data; ListNode *next; }; int main() { ListNode *p, *list; list = new ListNode; list->data = "New York"; p new ListNode; p->data = "Boston"; list->next = p; p->next = new ListNode; p->next->data = "Houston"; p->next->next = nullptr; // new code goes here Which of the following code correctly deletes the node with value "Boston" from the list when added at point of insertion indicated above? O list->next = p; delete p; O p = list->next; %3D list->next = p->next; delete p; p = list->next; list = p->next; delete p; O None of these O p = list->next; %3D list->next = p; %3D delete p;arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
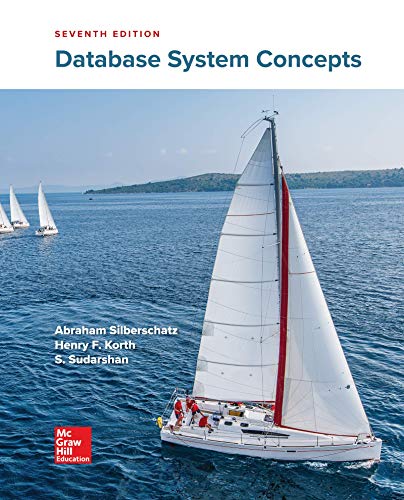
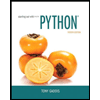
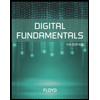
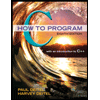
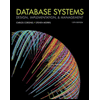
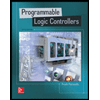