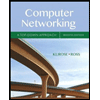
Add a main function to the following python code and organize the program using mainline logic.
def resistance(i,e):
return e/i
#Compute the total resistance as per the given formula
def parallel_resistance(r1,r2,r3):
return 1.0/((1.0/r1)+(1.0/r2)+(1.0/r3))
#Compute the series resistance
def series_resistance(r1,r2,r3):
return r1+r2+r3
#Read the voltage
e1=float(input('Voltage 1 : '))
e2=float(input('Voltage 2 : '))
e3=float(input('Voltage 3 : '))
#Read the current
i1=float(input('Current 1 : '))
i2=float(input('Current 2 : '))
i3=float(input('Current 3 : '))
#Compute the resistance
r1=resistance(i1,e1)
r2=resistance(i2,e2)
r3=resistance(i3,e3)
#Compute the resistance in parallel Circuit
rp=parallel_resistance(r1,r2,r3)
#Compute the resistance in series Circuit
rs=series_resistance(r1,r2,r3)
#Display the result
print('Resistance in Parallel Circuit : {0:.2f}'.format(rp))
print('Resistance in Series Circuit : {0:.2f}'.format(rs))

Step by stepSolved in 3 steps with 2 images

- Python programming for the following:arrow_forwardProgram using Python (see pic) Given code: def print_multiples(a,b,x): #Base Case/s #Add conditions here for base case/s if True : return 0 #Recursive Case/s #Add conditions here for recursive case/s else: return print_multiples(a, b, x) a = int(input("Please enter a: "))print(a)b = int(input("Please enter b: "))x = int(input("Please enter x: ")) print_multiples(a,b,x)arrow_forwardWrite a Python program that calculates the area and the circumferenceof a circle whose radius is described by the equation:r=√(x−h)2+(y−k)2+(z−m)2-------------------(1)Your code must have a main( ) and a getValues(center, point) functions.1.The main function reads data from an input file, which has theformat shown below. Each line in the file starts with a serialnumber followed by h, k, m, x, y, and then z (see Fig. 1). 2.For each sphere, the function getValues(center, point) receivestwo lists center (containing h,k, and m) and point (containing x,y, and z). Then it returns a list containing the radius, surfacearea and volume of the sphere use Equation 2 and 3 below. area=4πr2----------------------------(2)volume=43πr3----------------(3)3.This list is received by the main function and it is written it in an output file.4.In addition to that, your main function will count how many spheres have a surface areas less than 200 units2.Note: You program must be general and works for any…arrow_forward
- thumb_down Step 1 looking for a simple python script with an explanation of how -(for loops) work. provide code and explanation using requirements below,. Write a program that prints all the factors of a given number and checks whether it is a prime number. The program Asks user for a positive number strictly between 1 and 5000 (both exclusive). Validates that the number is within the range, if not, prompts the user to enter the number again, until user enters a valid number. Prints all the factors, and the total count of factors. (Using either a for loop or a while loop, checks all the numbers less than the input number to see if it is a factor. A number k is a factor of n if n % k == 0 ). Next checks and reports whether the input number is a prime number. ( A prime number is only divisible by two factors : 1 and itself)arrow_forwardwrite a python code that decodes the coloured bands on a resistor.Each colour band printed on a resistor has a corresponding numeric value, as shown in the table below: Colour Numeric value black 0 brown 1 red 2 orange 3 yellow 4 green 5 blue 6 violet 7 grey 8 white 9 To calculate the total resistance value, the following formula should be used: resistance=(10a+b)⋅10^c where a, b, and c are the numeric values of the first, second, and third colour bands respectively. For example, let's say that the colour bands on a resistor are red-green-orange. In this case, a=2, b=5, and c=3 (using the table). Hence the resistance value is 25000 ohms: resistance=(10*2+5) x 10^3=25000 ohms Your task is to write a program which asks the user to input the three colour bands. The program should then calculate and output the resistance value indicated by the bands. As part of your solution, you must define and use a function named colour_to_number. This function should take…arrow_forwardWrite algorithm to find the final acceleration of a car by taking time initial and final velocity from user. (Note: Final Velocity = Initial Velocity + Acceleration * time) Note : language is C++arrow_forward
- For this question you must design and implement a postcondition loop in Python that will display the following numbers (one on each line) to the terminal: 29, 41, 53, 65, 77, 89, 101, 113, 125, 137, 149, 161, 173, 185, 197, 209, 221, 233, 245, and 257. Although a counter-controlled loop is ideal for this task, you are not permitted to use a FOR loop in your program - you must use a postcondition loop that has been implemented using WHILE instead.Furthermore, your postcondition loop must be implemented using a Boolean flag variable.arrow_forwardExercise 1: Determine the number of times that the for loop is executed for each of the following problems. A. for (int k = 3; k = 10; k) { // statements; } E. for (int time = 10; time >= 5; time++) { //statements; } F. What is the value of count after the nested for loops are executed? int count(0); for (int k = -1; k 0; k++) { count++;arrow_forwardRewrite the two print statements in the code using format printing to show the result in two decimal places . def resistance(i,e): return e/i #Compute the total resistance as per the given formula def parallel_resistance(r1,r2,r3): return 1.0/((1.0/r1)+(1.0/r2)+(1.0/r3)) #Compute the series resistance def series_resistance(r1,r2,r3): return r1+r2+r3 #Read the voltage e1=float(input('Voltage 1 : '))e2=float(input('Voltage 2 : '))e3=float(input('Voltage 3 : ')) #Read the currenti1=float(input('Current 1 : '))i2=float(input('Current 2 : '))i3=float(input('Current 3 : ')) #Compute the resistance r1=resistance(i1,e1)r2=resistance(i2,e2)r3=resistance(i3,e3) #Compute the resistance in parallel Circuitrp=parallel_resistance(r1,r2,r3) #Compute the resistance in series Circuitrs=series_resistance(r1,r2,r3) #Display the result print('Resistance in Parallel Circuit : {0:.2f}'.format(rp))print('Resistance in Series Circuit : {0:.2f}'.format(rs)) Expert Solution…arrow_forward
- Rewrite the following code in python after removing all syntax error(s). Underline each correction done in the code def sum( s = for i in Range(1, c+1 s=s+ return print(sum(5 )si)0c)(sum(5)arrow_forwardMust show it in Python: Please show step by step with comments. Please show it in simplest form. Input and Output must match with the Question Please go through the Question very carefully.arrow_forwardpython:Evaluate the expression 12 % 7arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
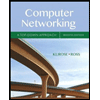
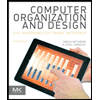
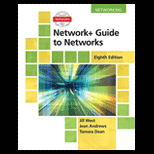
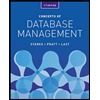
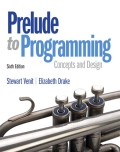
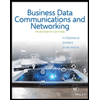