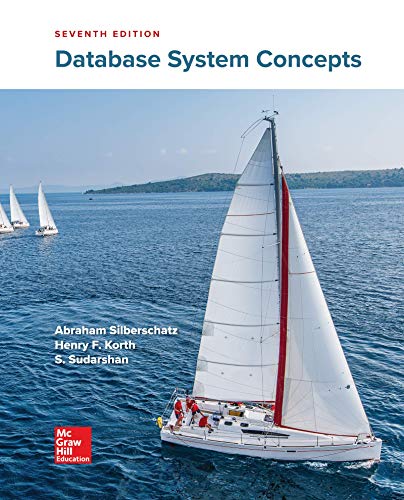
Answer the prompt with given main method:
Code:
import java.awt.*;
public class TestRandomWalker {
public static final int STEPS = 500;
public static void main(String[] args) {
RandomWalker walker = new RandomWalker(); // instantiate Walker object
DrawingPanel panel = new DrawingPanel(500, 500);
Graphics g = panel.getGraphics();
// advanced features -- center and zoom in the image
panel.getGraphics().translate(250, 250);
panel.getGraphics().scale(4, 4);
// make the walker walk, and draw its movement
int prevX = walker.getX(); // initialize Walker display
int prevY = walker.getY();
for (int i = 1; i <= STEPS; i++) {
g.setColor(Color.BLACK);
g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display
walker.move(); // move Walker 1 step
prevX = walker.getX(); // update x value
prevY = walker.getY(); // update y value
g.setColor(Color.RED);
g.drawLine(prevX, prevY, walker.getX(), walker.getY()); // update Walker display
int steps = walker.getSteps(); // record Walker steps
if (steps % 10 == 0) {
System.out.println(steps + " steps");
}
panel.sleep(100);
}
}
}
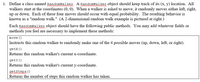

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- What is displayed by the following code segment? public class Widget { private int v1, v2; public Widget(int a, int b){v1 = a; v2 = b;} public int getTot(){return v1 + v2;} public void update(){v1 = v1+v2;} public String toString(){return ""+v1+","+v2;} %3D public static void main(String[] args) { Widget w = new Widget(2, 3); int tot = w.getTot(); w.update(); System.out.println(w + " %3D %3D %3D + tot);arrow_forwardWrite a Rectangle class. Its constructor should accept length and width as a parameter. It should have methods that return its length, width, perimeter, and area. (Use Java) Show a demonstration where you create two separate rectangles.arrow_forwardWhat is the exact output of this program?arrow_forward
- Develop a coding solution to perform the sorting per day (there are 7 days on which CSS perform sorting per day). For each of the days develop a class called CSSSorting, and for each day develop a method, something like: a. public static void CSSSortMonday(int[] values), b. public static void CSSSortTuesday(int[] values), c. public static void CSSSortWednesday(int[] values), d. public static void CSSSortThursday(int[] values) e. public static void CSSSortFriday(int[] values), f. public static void CSSSortSaturday(int[] values), g. public static void CSSSortSunday(int[] values) In each of these methods implement how you think the sorting should take place based on the each of the offered solutions/libraries.arrow_forwardHow many constructors does the following class have? widget weight: double - color: int + Widget(): + Widget (double, int) : + getWeight(): double +getColor() : int + setWeight (double): void + setColor (int) : void + displayInfo() : voidarrow_forwardThe weight of an object can be described by two integers: pounds and ounces (where 16 ounces equals one pound). Class model is as follows: public class Weight { private int pounds; private int ounces; public Weight(int p, int o) ( pounds=p+o/16: ounces = 0% 16: } Implement a method called subtract, which subtracts one weight to another. i.e. Weight w1 = new Weight(10.5): Weight w2 = new Weight(5.7): Weight w3= w1.subtract(w2):arrow_forward
- Please add the code after this one. The code has to be in java eclipse. Please add comments in what you are doing. I'll appreciate it public class Computer { private String ModelNumber; private String BrandName; private String manufacturingDate; private int NumberOfCores; //Constructor a public Computer() {} //Construct a computer object with model, BrandName, NumberOfCoresComputer (String ModelNumber, String BrandName, String manufacturingDate, int NumberOfCores){ this.ModelNumber=ModelNumber; this.BrandName=BrandName; this.manufacturingDate=manufacturingDate; this.NumberOfCores=NumberOfCores;}//generate getters/setterspublic String getModel() { return ModelNumber;} public void setModel(String ModelNumber) { ModelNumber = ModelNumber;} public String getBrandName() { return BrandName;} public void setBrandName(String brandName) { BrandName = brandName;} public String getManufacturingDate() { return manufacturingDate;} public void setManufacturingDate(String…arrow_forward8arrow_forwardConsider the following class definitions: public class Thing {public void string_method(String word) {word = word.substring(word.length() - 1);System.out.print(word);} }public class Thing2 extends Thing {public void string_method(String word) {super.string_method(word);System.out.print(word.substring(0,1));}} The following code segment appears in a class other than Thing or Thing2 Thing2 thing = new Thing2();thing.string_method("Tacky"); What is printed as a result of running the code segment? Group of answer choices yT yy Index Out of Bounds Error TT TackyTackyarrow_forward
- Create a UML class diagram of the application illustrating class hierarchy, collaboration, and the content of each class. There is only one class. There is a main method that calls four methods. I am not sure if I made the UML Class diagram correct. import java.util.Random; // import Random packageimport java.util.Scanner; // import Scanner Package public class SortArray{ // class name public static void main(String[] args) { //main method Scanner scanner = new Scanner(System.in); // creates object of the Scanner classint[] array = initializeArray(scanner); System.out.print("Array of randomly generated values: ");displayArray(array); // Prints unsorted array sortDescending(array); // sort the array in descending orderSystem.out.print("Values in desending order: ");displayArray(array); // prints the sorted array, should be in descending ordersortAscending(array); // sort the array in ascending orderSystem.out.print("Values in asending order: ");displayArray(array); // prints the…arrow_forwardJava programarrow_forward2-7 Given the code below, which lines are valid and invalid.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
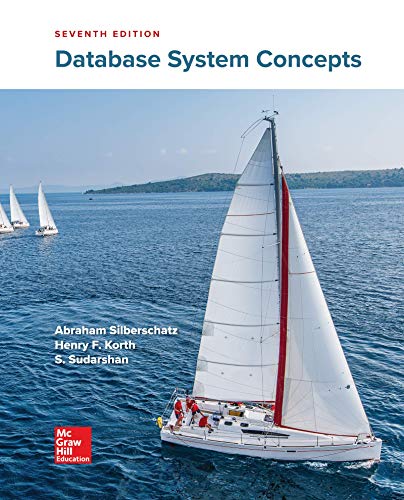
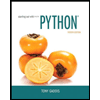
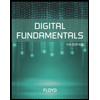
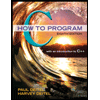
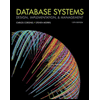
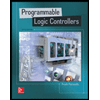