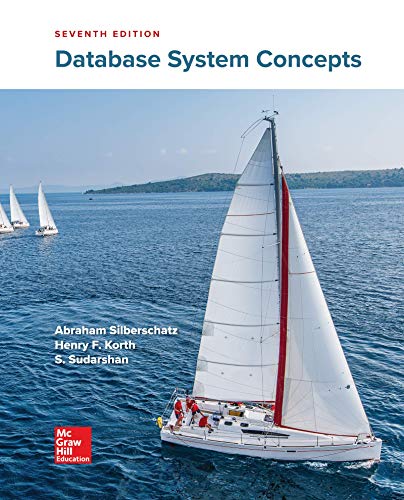
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Concept explainers
Question
Write dry run of following program (java-oop)
public class Movie {
private String rating; private int ID; private String title;
public Movie() {
rating = ""; ID = 0; title = "";
7/3
}
public Movie(String aRating, int aID, String aTitle) {
rating = aRating; ID = aID;
title = aTitle;
}
public String getRating() {
return rating; }
public void setRating(String aRating) {
}
rating = aRating;
public int getID() {
return ID; }
public void setID(int aID) {
ID = aID; }
public String getTitle() {
return title; }
public void setTitle(String aTitle) {
title = aTitle; }
public double calcLateFees(int days) {
return 2.0 * days;
}
public boolean equals(Object obj) {
if(obj == null)
return false;
else if(getClass() != obj.getClass())
{
} }
Movie other = (Movie)obj;
return (rating.equals(other.rating) && ID == other.ID && title.equals(other.title));
return false; else
public String toString()
8/3
} }
{
return "\nMPAA Rating: " + rating + "\nID Number: " + ID + "\nMovie Title: " + title;
// Action.java
public class Action extends Movie {
public Action() {
super(); }
public Action(String aRating, int aID, String aTitle) {
}
super(aRating, aID, aTitle);
public double calcLateFees(int days) {
return 3.0 * days;
} }
// Comedy.java
public class Comedy extends Movie {
public Comedy() {
super(); }
public Comedy(String aRating, int aID, String aTitle) {
super(aRating, aID, aTitle);
}
public double calcLateFees(int days) {
return 2.5 * days;
} }
// Drama.java
public class Drama extends Movie {
public Drama() {
super(); }
} }
// MovieTest.java
public Drama(String aRating, int aID, String aTitle) {
super(aRating, aID, aTitle);
}
public double calcLateFees(int days) {
return 2.0 * days;
9/3
public class MovieTest {
public static void main(String[] args) {
Movie movie =
new Movie("PG-13", 3691, "Norm of the North"); Action action = new Action("G", 2587, "Eye In The Sky");
Comedy comedy = new Comedy("R", 7989, "Kung Fu Panda 3"); Drama drama =
new Drama("PG-13", 4563, "Batman v Superman");
System.out.println(movie);
System.out.println("Late Fee: $" + movie.calcLateFees(6));
System.out.println(action); System.out
.println("Late Fee: $" + action.calcLateFees(6));
} }
System.out.println(comedy); System.out
.println("Late Fee: $" + comedy.calcLateFees(6));
System.out.println(drama);
System.out.println("Late Fee: $" + drama.calcLateFees(6));
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution
Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- class Player { protected: string name; double weight; double height; public: Player(string n, double w, double h) { name = n; weight = w; height = h; } string getName() const { return name; } virtual void printStats() const = 0; }; class BasketballPlayer : public Player { private: int fieldgoals; int attempts; public: BasketballPlayer(string n, double w, double h, int fg, int a) : Player(n, w, h) { fieldgoals = fg; attempts = a; } void printStats() const { cout << name << endl; cout << "Weight: " << weight; cout << " Height: " << height << endl; cout << "FG: " << fieldgoals; cout << " attempts: " << attempts; cout << " Pct: " << (double) fieldgoals / attempts << endl; } }; a. What does = 0 after function printStats() do? b. Would the following line in main() compile: Player p; -- why or why not? c. Could…arrow_forwardQ1. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { balance = balance + amount ; } // modified toString() method public String toString() { return "Account: " + actNum + "\tName: " + nameOnAct + "\tBalance: " + amount ; } } Q2. amount the same, y, n, why? public class CheckingAct { private String actNum; private String nameOnAct; private int balance; . . . . public void processDeposit( int amount ) { // scope of amount starts here balance = balance + amount ; // scope of amount ends here } public void processCheck( int amount ) { // scope of amount starts here int charge; incrementUse(); if ( balance < 100000 ) charge = 15; else charge = 0; balance = balance - amount - charge ; // scope of amount ends here }…arrow_forwardpublic class ItemToPurchase { private String itemName; private int itemPrice; private int itemQuantity; // Default constructor public ItemToPurchase() { itemName = "none"; itemPrice = 0; itemQuantity = 0; } // Mutator for itemName public void setName(String itemName) { this.itemName = itemName; } // Accessor for itemName public String getName() { return itemName; } // Mutator for itemPrice public void setPrice(int itemPrice) { this.itemPrice = itemPrice; } // Accessor for itemPrice public int getPrice() { return itemPrice; } // Mutator for itemQuantity public void setQuantity(int itemQuantity) { this.itemQuantity = itemQuantity; } // Accessor for itemQuantity public int getQuantity() { return itemQuantity; }} import java.util.Scanner; public class ShoppingCartPrinter { public static void main(String[] args) { Scanner scnr = new…arrow_forward
- class temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; I need help writing the definition of the member function set so the instance varialbes are set according to the parameters. I also need help in writing the definition of the member function manipulation that returns a decimal with: the value of the description as "rectangle", returns first * second; if the value of description is "circle", it returns the area of the circle with radius first; if the value of the description is "cylinder", it returns the volume of the cylinder with radius first and height second; otherwise, it returns with the value -1.arrow_forwardC++ programmingarrow_forwardConver the class To UML diagram class Clint { private String accNumber; private String holderName; private long currBalance; public Clint() { currBalance = 0; } public Clint(String acc, String name, long bal) { accNumber = acc; holderName = name; currBalance = bal; } Scanner sc = new Scanner(System.in); // deposit money into the bank account by given amount amt public void deposit(long amt) { currBalance += amt; } // if amount is not given ask the user for the amount public void deposit() { System.out.print("Enter Amount(to deposit): $"); long amt = sc.nextLong(); currBalance += amt; } // withdraw money from the bank account public void withdraw(long amt) { if (currBalance >= amt) { currBalance -= amt; } else { System.out.println("Insufficient funds!"); } } // if amt is not given ask the user for the amount public…arrow_forward
- int getUpperScore(){ int upperScore = 0; for(int i = ONES; i <= SIXES; i++){ upperScore += score[i]; } return upperScore + getBonusScore(); } //Returns the score of the upper part of the board int getLowerScore(){ int lowerScore = 0; for(int i = THREE_OF_KIND; i <= YAHTZEE; i++){ lowerScore += score[i]; } return lowerScore; } //Returns the bonus points int getBonusScore(){ const int UPPER_SCORES_THRESHOLD = 63; const int BONUS_POINTS = 35; if(getUpperScore() >= UPPER_SCORES_THRESHOLD){ return BONUS_POINTS; } return 0; } //Returns the total score int getTotalScore(){ return getUpperScore() + getLowerScore(); } Test 10: Check upper board and having bonus correctly (0/3) failedarrow_forwardPython language Write a initializer method for the class Test. It has 2 parameters/field variables: correct and totalarrow_forwardC# List the differences between CommissionEmployee class and BasePlusCommissionEmployee class public class BasePlusCommissionEmployee { public string FirstName { get; } public string LastName { get; } public string SocialSecurityNumber { get; } private decimal grossSales; private decimal commissionRate; private decimal baseSalary; public BasePlusCommissionEmployee(string firstName, string lastName, string socialSecurityNumber, decimal grossSales, decimal commissionRate, decimal baseSalary) { FirstName = firstName; LastName = lastName; SocialSecurityNumber = socialSecurityNumber; GrossSales = grossSales; CommissionRate = commissionRate; BaseSalary = baseSalary; } public decimal GrossSales { get { return grossSales; } set { if (value < 0) // validation { throw new ArgumentOutOfRangeException(nameof(value),…arrow_forward
- public class Plant { protected String plantName; protected String plantCost; public void setPlantName(String userPlantName) { plantName = userPlantName; } public String getPlantName() { return plantName; } public void setPlantCost(String userPlantCost) { plantCost = userPlantCost; } public String getPlantCost() { return plantCost; } public void printInfo() { System.out.println(" Plant name: " + plantName); System.out.println(" Cost: " + plantCost); }} public class Flower extends Plant { private boolean isAnnual; private String colorOfFlowers; public void setPlantType(boolean userIsAnnual) { isAnnual = userIsAnnual; } public boolean getPlantType(){ return isAnnual; } public void setColorOfFlowers(String userColorOfFlowers) { colorOfFlowers = userColorOfFlowers; } public String getColorOfFlowers(){ return colorOfFlowers; } @Override public void printInfo(){…arrow_forwardclass temporary { public: void set(string, double, double); void print(); double manipulate(); void get(string&, double&, double&); void setDescription(string); void setFirst(double); void setSecond(double); string getDescription() const; double getFirst()const; double getSecond()const; temporary(string = "", double = 0.0, double = 0.0); private: string description; double first; double second; }; What are the members and functions in this example? I need help identifying how the members and functions work to answer the questions below. I am having troubles understanding this concept. I am learning constructors and deconstructors along with inline and hiding information. I feel lost trying to answer these questions. Can I get direction on how to proceed? Write the definition of the member function set so that the instance variables are set according to the parameters. Write the definition of the member function manipulate that returns a decimal number as follows: If the…arrow_forwardQuestion 37 public static void main(String[] args) { Dog[] dogs = { new Dog(), new Dog()}; for(int i = 0; i >>"+decision()); } class Counter { private static int count; public static void inc() { count++;} public static int getCount() {return count;} } class Dog extends Counter{ public Dog(){} public void wo(){inc();} } class Cat extends Counter{ public Cat(){} public void me(){inc();} } The Correct answer: Nothing is output O 2 woofs and 5 mews O 2 woofs and 3 mews O 5 woofs and 5 mews Oarrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
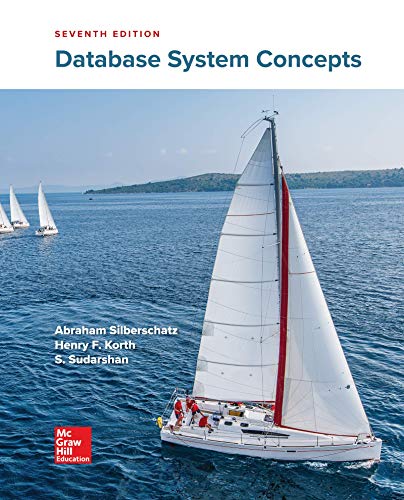
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
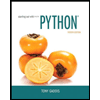
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
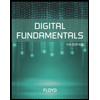
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
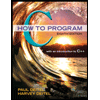
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
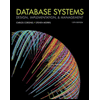
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
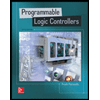
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education