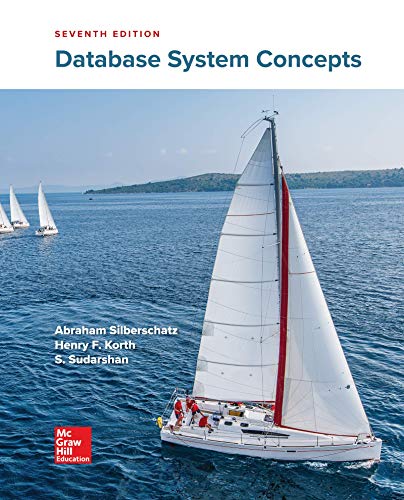
Concept explainers
Introduction The Object Oriented software model is designed to create a new data type by using the Class structure. Therefore the various operations which define a data type, such as the common operators, assignment, etc., have a software architecture available to create those operations and data types. Assignment Description This assignment is an exercise in creating a complete class definition. A standard way to do that is to use a math data type. Here we define a Rational Number data type in a class called “Rational”. The distinctive feature of this type is the data is kept as numerator and denominator throughout all operations; there is no floating point representation used. The standard math operations, addition, subtraction, etc., are implemented, including the iostream overload. The student will not only come to understand what is required for a complete representation of such a type, but also learn about the internal
#ifndef RATIONAL_NUMBER_H_ #define RATIONAL_NUMBER_H_ #include class Rational { public: Rational(); Rational(int n,int d); Rational(const Rational&); // copy constructor ~Rational() {} void Set(int n,int d); void Get(int& n,int& d) const; bool isValid() const; void Set(const char*); Rational& operator= (const Rational &); // assignment operator bool operator== (const Rational &) const; bool operator!= (const Rational &) const; bool operator> (const Rational &) const; bool operator< (const Rational &) const; bool operator>= (const Rational &) const; bool operator<= (const Rational &) const; Rational& operator+= (int); Rational& operator-= (int); Rational operator+ (int) const; Rational operator- (int) const; Rational operator++ (int); Rational& operator++ (); Rational operator-- (int); Rational& operator-- (); Rational& operator+= (const Rational&); Rational& operator-= (const Rational&); Rational operator+ (const Rational&) const; Rational operator- (const Rational&) const; friend Rational operator+ (int,const Rational&); friend Rational operator- (int,const Rational&); friend std::ostream& operator<< (std::ostream&,const Rational &); const char* toString() const; operator const char*() { return toString(); } private: int n,d; // numerator, denominator }; #endif
i need full correct c++ code

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 1 images

- Describe the scope of class.arrow_forwardDefine a Course base class with the following attributes: number - course number title - course title Define a print_info() method in Course that displays the course number and title. Also define a derived class OfferedCourse with the additional attributes: instructor_name - instructor name location - class location class_time - class timearrow_forward*Initial Class Diagram is attached below Refine the UML class diagram so that it demonstrates the following: Classes (using the complex class symbols), Associations (with multiplicities AND association names), Generalizations (if any), and Compositions (if any) Focus ONLY on the following activities within the system: Start a Trip, Reserve a Vehicle, Make a Parking Reservation, Make a Charging Session for a Personal Vehicle, End a Trip or Charging Session, and Submit a Support Ticket for a Vehicle or Station Add the primary key necessary to support any relationships that have a multiplicity of "1" as an attribute in the corresponding classarrow_forward
- USE C++ PLEASE Q#1 Using the concept of classes, constructors and data structure (arrays or any under data structure) implement a detailed University and student enrollment system. Each department of university has a separate class and perform every functionality with in a class. Create a main class where methods of other classes will be called.arrow_forwardWrite UML Class Diagram that illustrates the following program: 1) Person class The person class should include the following data fields; • Name • ID number • birth date • home address • phone number Be sure to include get and set methods for each data field. 2) Course class The course class should contain the following fields: • ID Number • Name College (Business, Engineering, Nursing, etc) • An Array of person objects . 3) University class The University class should contain the data fields for the university, such as: • University name • Federal ID number • Address • Array / ArrayList of course class objects • Array / ArrayList of person class objects Extended Classes: 4 & 5) Extend the Person class into Student class and Employee class 6) Extend the Employee class into Instructor class and Staff class 7 & 8) Extend the Student class into graduate Student and undergrad Student class 9) Registration Class ---> The Registration class creates transactions to link each student with…arrow_forwardProgramming Exercise 10-1arrow_forward
- Using C# language: Programming PLO-2 Measured: Design, implement, and evaluate computer solutions utilizing structured and object-oriented programming methodologies. Design a class named Contractor. The class should keep the following information: Contractor name Contractor number Contractor start date Write one or more constructors and the appropriate accessor and mutator functions for the class.arrow_forwardThe approach to analysis modelling in which we focus on class definitions and class relationships is structured analysis object-oriented analysis top down functional design none of the abovearrow_forwardWhat are the three responsibilities that come with working with classes that include member variables that are pointers and for which you are responsible?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
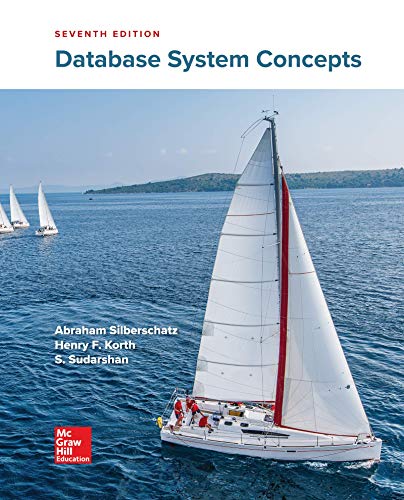
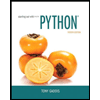
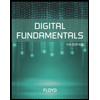
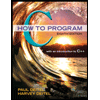
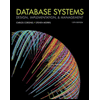
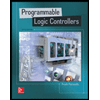