Assignment #6 - Array Practice Due: Wed, Nov 16 Objective To gain practice with arrays and common array algorithms, as well as the use of array parameters in functions. Task This assignment will consist of writing several functions that manipulate arrays or access data from arrays, as well as a test program that will allow interactive testing of the array functions. Part 1: Functions Write the following functions. Each one takes in an integer array as a parameter, and other necessary parameters are returns are described. Make sure the parameters are in the order specified. Make sure to use the const qualifier on the array parameter on any function where it is appropriate. A sample CALL is given for each function. • Insert Write a function called Insert that takes in four parameters: o an integer array o the size of the array o the new value to be inserted into the array o the index at which to insert the new value This function should insert a a new value into the array, at the specified index. Note that this means that other values have to "move" to make room. The last value in the array will just disappear from the array. If the index is out of bounds for the array, abort the function with no change made to the array. This function does not return a value. Sample call: // Suppose the array "list" is {2, 4, 6, 8, 10, 12} Insert(list, 6, 100, 3); // insert the value 100 at index 3. // "list" is now {2, 4, 6, 100, 8, 10} • Delete Write a function called Delete that takes in three parameters: o an integer array o the size of the array o the index of the item to delete This function should delete the value at the given index. The remaining items in the array will need to shift over to fill the "empty" slot. The last item in the array (now vacant) should be set to 0. If the given index is out of bounds for the array, abort the function without deleting anything. This function does not return a value. Sample call: // Suppose the array "list" is {2, 4, 6, 8, 10, 12} Delete (list, 6, 2); // delete the value at index 2. // "list" is now {2, 4, 8, 10, 12, 0}
Assignment #6 - Array Practice Due: Wed, Nov 16 Objective To gain practice with arrays and common array algorithms, as well as the use of array parameters in functions. Task This assignment will consist of writing several functions that manipulate arrays or access data from arrays, as well as a test program that will allow interactive testing of the array functions. Part 1: Functions Write the following functions. Each one takes in an integer array as a parameter, and other necessary parameters are returns are described. Make sure the parameters are in the order specified. Make sure to use the const qualifier on the array parameter on any function where it is appropriate. A sample CALL is given for each function. • Insert Write a function called Insert that takes in four parameters: o an integer array o the size of the array o the new value to be inserted into the array o the index at which to insert the new value This function should insert a a new value into the array, at the specified index. Note that this means that other values have to "move" to make room. The last value in the array will just disappear from the array. If the index is out of bounds for the array, abort the function with no change made to the array. This function does not return a value. Sample call: // Suppose the array "list" is {2, 4, 6, 8, 10, 12} Insert(list, 6, 100, 3); // insert the value 100 at index 3. // "list" is now {2, 4, 6, 100, 8, 10} • Delete Write a function called Delete that takes in three parameters: o an integer array o the size of the array o the index of the item to delete This function should delete the value at the given index. The remaining items in the array will need to shift over to fill the "empty" slot. The last item in the array (now vacant) should be set to 0. If the given index is out of bounds for the array, abort the function without deleting anything. This function does not return a value. Sample call: // Suppose the array "list" is {2, 4, 6, 8, 10, 12} Delete (list, 6, 2); // delete the value at index 2. // "list" is now {2, 4, 8, 10, 12, 0}
C++ for Engineers and Scientists
4th Edition
ISBN:9781133187844
Author:Bronson, Gary J.
Publisher:Bronson, Gary J.
Chapter7: Arrays
Section: Chapter Questions
Problem 6PP: (Numerical) a. Define an array with a maximum of 20 integer values, and fill the array with numbers...
Related questions
Question
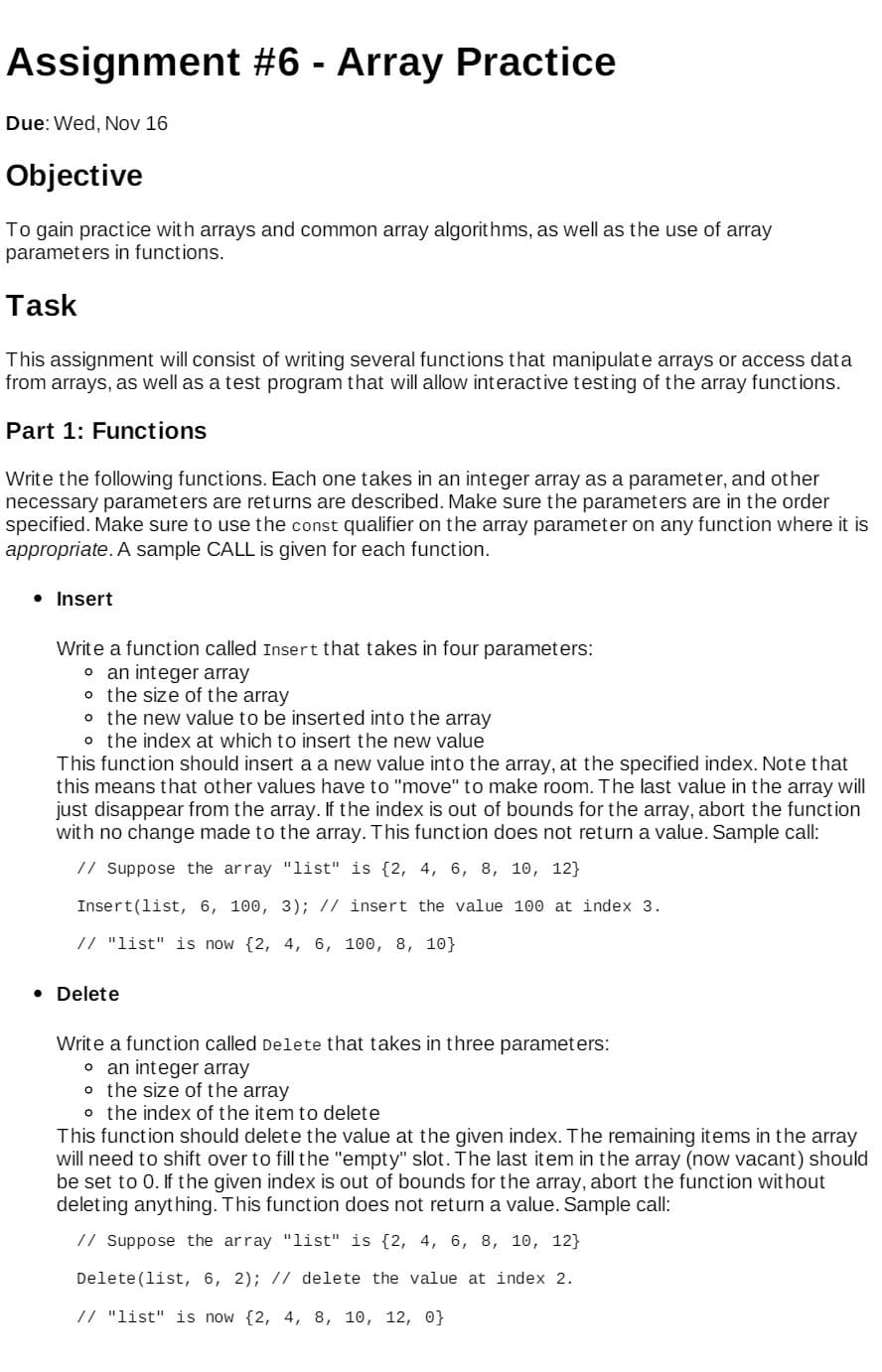
Transcribed Image Text:Assignment #6 - Array Practice
Due: Wed, Nov 16
Objective
To gain practice with arrays and common array algorithms, as well as the use of array
parameters in functions.
Task
This assignment will consist of writing several functions that manipulate arrays or access data
from arrays, as well as a test program that will allow interactive testing of the array functions.
Part 1: Functions
Write the following functions. Each one takes in an integer array as a parameter, and other
necessary parameters are returns are described. Make sure the parameters are in the order
specified. Make sure to use the const qualifier on the array parameter on any function where it is
appropriate. A sample CALL is given for each function.
• Insert
Write a function called Insert that takes in four parameters:
o an integer array
o the size of the array
o the new value to be inserted into the array
o the index at which to insert the new value
This function should insert a a new value into the array, at the specified index. Note that
this means that other values have to "move" to make room. The last value in the array will
just disappear from the array. If the index is out of bounds for the array, abort the function
with no change made to the array. This function does not return a value. Sample call:
// Suppose the array "list" is {2, 4, 6, 8, 10, 12}
Insert(list, 6, 100, 3); // insert the value 100 at index 3.
// "list" is now {2, 4, 6, 100, 8, 10}
• Delete
Write a function called Delete that takes in three parameters:
o an integer array
o the size of the array
o the index of the item to delete
This function should delete the value at the given index. The remaining items in the array
will need to shift over to fill the "empty" slot. The last item in the array (now vacant) should
be set to 0. If the given index is out of bounds for the array, abort the function without
deleting anything. This function does not return a value. Sample call:
// Suppose the array "list" is {2, 4, 6, 8, 10, 12}
Delete (list, 6, 2); // delete the value at index 2.
// "list" is now {2, 4, 8, 10, 12, 0}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
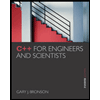
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
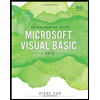
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
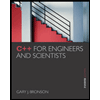
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
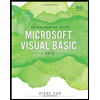
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning