main.py Load default template.. 1 # The word_mult() function takes two inputs. 2 # It should first confirm that they are both string types. 3 # 4 # If they are both strings, compute the product of the two string lengths. 5 # Then print the following: 6 # "Both and are strings." 7 # "The product of their lengths is ." 8 # Then return 10 # If they are not both strings, print the following: 11 # "Incorrect argument type found. Expected strings." 12 # Then return False 13 14 def word_mult(word1, word2): '''Insert code here!'' 15 16 17 18
String Character Multiplier
Learning Objectives
In this lab, you will:
- create a function according to the specifications
- check on the type
- practice if/else statement
Instructions
In this lab, we will practice writing functions that both display (ie: print) and return values. This will all be done in the word_mult() function below.
Given two inputs, write a function word_mult(word1, word2) that will:
- Confirm both word1 and word2 are of type str
- If they are both strings, compute the product of the two string lengths. Then print the following:
and return <product of string lengths>.
- If they are not both strings, print the following:
Then return False.
Hint: Remember, printing and returning a value are different. You can print strings on-screen with the command print(<string>) and return a function's output with return.
# The word_mult() function takes two inputs.
# It should first confirm that they are both string types.
#
# If they are both strings, compute the product of the two string lengths.
# Then print the following:
# "Both <string 1> and <string 2> are strings."
# "The product of their lengths is <product of string lengths>."
# Then return <product of string lengths>
#
# If they are not both strings, print the following:
# "Incorrect argument type found. Expected strings."
# Then return False
def word_mult(word1, word2):
'''Insert code here!'''
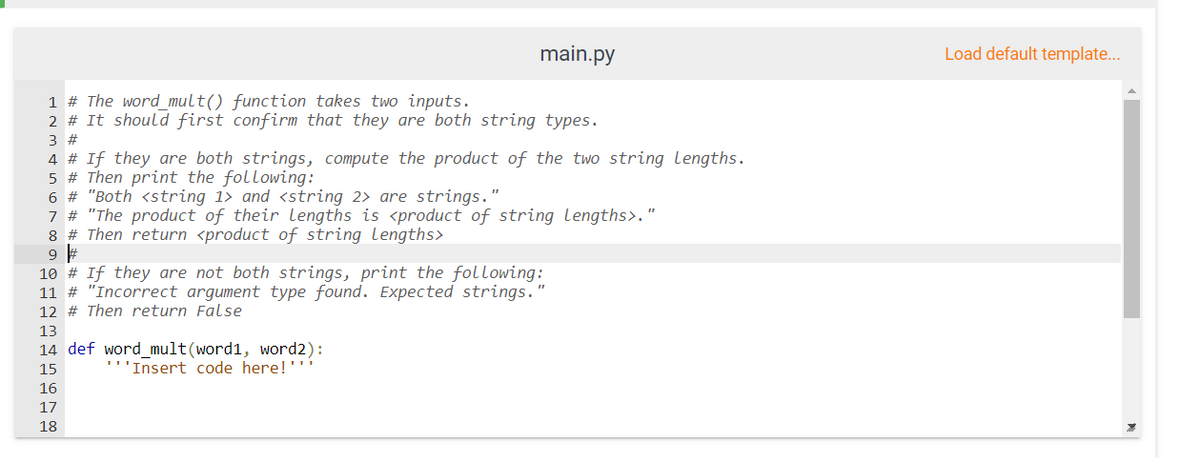

- First, we will enter the two strings.
- Then we will pass both the strings to function word_mult().
- This function will calculate the product of the length of both the strings and return it.
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

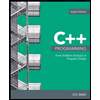
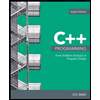